Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / IssuerInformation.cs / 1 / IssuerInformation.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Xml; using System.Collections.Generic; using System.Collections; using System.Xml.Serialization; using System.Xml.Schema; using System.IO; using System.Text; using Microsoft.InfoCards.Diagnostics; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; internal class IssuerInformationEntry { string entryName; string entryValue; public string Name { get { return entryName; } } public string Value { get { return entryValue; } } public IssuerInformationEntry( string name, string value ) { entryName = name; entryValue = value; } } // // Summary // Additional information about a managed card issuer // internal class IssuerInformation { Listm_informationEntries; public IssuerInformation() { m_informationEntries = new List (); } // // Summary // Serialize the IssuerInformation object // // Parameter // writer - binary stream conforming to the serialization format supported by this class. // public void Serialize( System.IO.Stream stream ) { // // Setup a BinaryWriter to serialize the bytes of each member to the provided stream // System.IO.BinaryWriter writer = new BinaryWriter( stream, Encoding.Unicode ); writer.Write( m_informationEntries.Count ); if( m_informationEntries.Count > 0 ) { foreach( IssuerInformationEntry entry in m_informationEntries ) { Utility.SerializeString( writer, entry.Name ); Utility.SerializeString( writer, entry.Value ); } } } // // Summary // Read the IssuerInformation object // // Parameter // reader - xml stream reader conforming to the serialization format supported by this class. // public void ReadIssuerInformation( XmlReader reader ) { if( !reader.IsStartElement( XmlNames.WSIdentity07.IssuerInformation, XmlNames.WSIdentity07.Namespace ) ) { throw IDT.ThrowHelperError( new XmlException( SR.GetString( SR.UnexpectedElement ) ) ); } while( reader.Read() ) { if( XmlNames.WSIdentity07.IssuerInformationEntry == reader.LocalName && XmlNames.WSIdentity07.Namespace == reader.NamespaceURI ) { ReadIssuerInformationEntry( reader ); } if( XmlNames.WSIdentity07.IssuerInformation == reader.LocalName && XmlNames.WSIdentity07.Namespace == reader.NamespaceURI && XmlNodeType.EndElement == reader.NodeType ) { return; } } } // // Summary // Read the IssuerInformationEntry object // // Parameter // reader - xml stream reader conforming to the serialization format supported by this class. // public void ReadIssuerInformationEntry( XmlReader reader ) { if( !reader.IsStartElement( XmlNames.WSIdentity07.IssuerInformationEntry, XmlNames.WSIdentity07.Namespace ) ) { throw IDT.ThrowHelperError( new XmlException( SR.GetString( SR.UnexpectedElement ) ) ); } string name = string.Empty; string value = string.Empty; while( reader.Read() ) { if( XmlNames.WSIdentity07.IssuerInformationEntry == reader.LocalName && XmlNames.WSIdentity07.Namespace == reader.NamespaceURI && XmlNodeType.EndElement == reader.NodeType ) { m_informationEntries.Add( new IssuerInformationEntry( name, value ) ); return; } if( XmlNames.WSIdentity07.EntryName == reader.LocalName && XmlNames.WSIdentity07.Namespace == reader.NamespaceURI ) { name = reader.ReadString(); if( string.IsNullOrEmpty( name ) ) { throw IDT.ThrowHelperError( new InvalidCardException() ); } } if( XmlNames.WSIdentity07.EntryValue == reader.LocalName && XmlNames.WSIdentity07.Namespace == reader.NamespaceURI ) { value = reader.ReadString(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
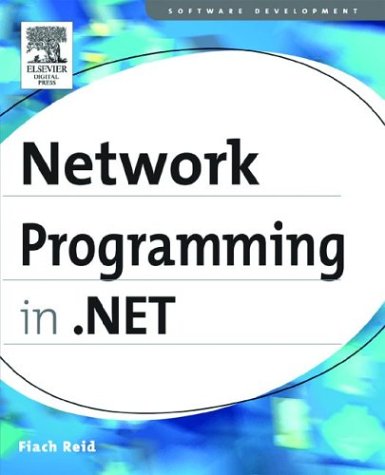
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PackUriHelper.cs
- RadialGradientBrush.cs
- WindowsFormsHost.cs
- AgileSafeNativeMemoryHandle.cs
- FloaterBaseParaClient.cs
- TextOutput.cs
- Encoding.cs
- ListBox.cs
- Camera.cs
- ExceptionUtil.cs
- TokenBasedSet.cs
- ClientSponsor.cs
- HtmlInputCheckBox.cs
- SystemResources.cs
- AxisAngleRotation3D.cs
- SpinLock.cs
- CodeDomDesignerLoader.cs
- FormViewActionList.cs
- TextReader.cs
- HtmlTitle.cs
- EasingKeyFrames.cs
- CompensateDesigner.cs
- ValidationManager.cs
- COM2PictureConverter.cs
- Latin1Encoding.cs
- SimpleHandlerBuildProvider.cs
- HashStream.cs
- X509Chain.cs
- CollectionsUtil.cs
- DataColumnSelectionConverter.cs
- ThreadStaticAttribute.cs
- MouseEventArgs.cs
- MetadataFile.cs
- Wizard.cs
- RemotingSurrogateSelector.cs
- QueryPageSettingsEventArgs.cs
- TaskbarItemInfo.cs
- PointHitTestParameters.cs
- KeyPressEvent.cs
- ByteFacetDescriptionElement.cs
- TypeConverterHelper.cs
- ClientScriptItemCollection.cs
- CurrentTimeZone.cs
- RTLAwareMessageBox.cs
- EncryptedKeyHashIdentifierClause.cs
- XPathItem.cs
- DBCommandBuilder.cs
- PathFigure.cs
- SoundPlayer.cs
- ManualWorkflowSchedulerService.cs
- OleStrCAMarshaler.cs
- NotSupportedException.cs
- ImageAnimator.cs
- ContextMenuStrip.cs
- PerformanceCounter.cs
- DesignTimeData.cs
- MonthCalendar.cs
- SqlNodeAnnotations.cs
- HtmlInputCheckBox.cs
- Subtree.cs
- QilFactory.cs
- DependencyPropertyConverter.cs
- HScrollBar.cs
- ToolStripItemClickedEventArgs.cs
- MailHeaderInfo.cs
- Socket.cs
- Image.cs
- TextElementAutomationPeer.cs
- IxmlLineInfo.cs
- Matrix3DStack.cs
- CompositeActivityCodeGenerator.cs
- HttpGetServerProtocol.cs
- IisTraceListener.cs
- ToolboxComponentsCreatingEventArgs.cs
- XmlExpressionDumper.cs
- MemberAccessException.cs
- GuidelineCollection.cs
- XPathAncestorIterator.cs
- SerializationStore.cs
- XmlSchemaAttributeGroup.cs
- serverconfig.cs
- ResourceExpressionBuilder.cs
- ChildDocumentBlock.cs
- ContentElement.cs
- UniqueEventHelper.cs
- BoundPropertyEntry.cs
- WarningException.cs
- ControlCodeDomSerializer.cs
- MarkupExtensionParser.cs
- AuthorizationRule.cs
- Graphics.cs
- ToolStripPanelCell.cs
- ImageAutomationPeer.cs
- OutputCacheProfileCollection.cs
- QilName.cs
- DataStreamFromComStream.cs
- CacheEntry.cs
- ConditionalExpression.cs
- PerfService.cs
- TreeView.cs