Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / HashUtility.cs / 1 / HashUtility.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Security.Cryptography; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Singleton utilty class for hashing data. // Remarks: // This class is currently global. Need to discuss a suitable hosting environment for it // ( per user is probably appropriate ) // internal static class HashUtility { // // Summary: // The String identifier of the alg we use. Do we need to abstract this choice somewhere // public const string HashAlgorithmName = "SHA256"; private static object s_lock = new Object(); private static SHA256Managed s_hasher = new SHA256Managed(); // // Summary: // Gets the BitLength of the current hashing algorithm // public static Int32 HashBitLength { get{ return s_hasher.HashSize; } } // // Summary: // Gets the Buffer Size of the current hashing algorithm // public static Int32 HashBufferLength { get { return HashBitLength / 8; } } // // Summary: // Hashes a given value, and write the value to the output buffer. // // Remarks: // To get the size required for the output buffer, use HashBufferLength // // Parameters: // data: the output data buffer // dataIndex: the index to place the hash in the output data buffer // dataToHash: the input data to hash // public static void SetHashValue( byte[] data, int dataIndex, byte[] dataToHash ) { if( null == dataToHash ) { throw IDT.ThrowHelperArgumentNull( "dataToHash" ); } SetHashValue( data, dataIndex, dataToHash, 0, dataToHash.Length ); } // // Summary: // Hashes a given value, and write the value to the output buffer. // // Remarks: // To get the size required for the output buffer, use HashBufferLength // // Parameters: // data: the output data buffer // dataIndex: the index to place the hash in the output data buffer // dataToHash: the input data to hash // dataToHashIndex: the index in the input to start hashing // dataToHashSize: the size of the data to hash. // public static void SetHashValue( byte[] data, int dataIndex, byte[] dataToHash, int dataToHashIndex, int dataToHashSize ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } if( dataIndex < 0 || dataIndex >= data.Length ) { throw IDT.ThrowHelperError( new ArgumentOutOfRangeException( "dataIndex", dataIndex, SR.GetString( SR.StoreHashUtilityDataOutOfRange ) ) ); } if( null == dataToHash ) { throw IDT.ThrowHelperArgumentNull( "dataToHash" ); } if( dataToHashIndex < 0 || dataToHashIndex > dataToHash.Length ) { throw IDT.ThrowHelperError( new ArgumentOutOfRangeException( "dataToHashIndex", dataToHashIndex, SR.GetString( SR.StoreHashUtilityDataToHashOutOfRange ) ) ); } if( dataToHashSize < 0 || dataToHashSize > ( dataToHash.Length - dataToHashIndex ) ) { throw IDT.ThrowHelperError( new ArgumentOutOfRangeException( "dataToHashIndex", dataToHashIndex, SR.GetString( SR.StoreHashUtilityDataToHashOutOfRange ) ) ); } byte[] newBuffer = null; lock( s_lock ) { newBuffer = s_hasher.ComputeHash( dataToHash, dataToHashIndex, dataToHashSize ); } // // Copy this buffer to the output buffer // Array.Copy( newBuffer, 0, data, dataIndex, newBuffer.Length ); // // Clear this has, as we can't ensure it will // be collected immediatly. // Array.Clear( newBuffer, 0, newBuffer.Length ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
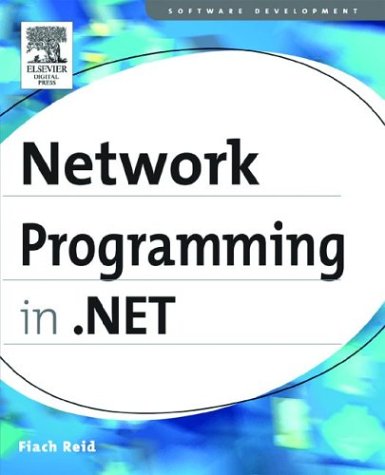
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PasswordDeriveBytes.cs
- Control.cs
- TabRenderer.cs
- DropSource.cs
- StateMachine.cs
- ValueChangedEventManager.cs
- SqlDataSourceSelectingEventArgs.cs
- DateTimeSerializationSection.cs
- XmlValueConverter.cs
- ConfigurationLocationCollection.cs
- ModelVisual3D.cs
- Exceptions.cs
- RequestCachePolicyConverter.cs
- Stack.cs
- CreateUserErrorEventArgs.cs
- DataControlHelper.cs
- AuthenticateEventArgs.cs
- HwndTarget.cs
- DecimalAverageAggregationOperator.cs
- XmlSchemaFacet.cs
- ZipIORawDataFileBlock.cs
- TextEditorCharacters.cs
- ResXResourceReader.cs
- MetadataSerializer.cs
- Command.cs
- ManipulationCompletedEventArgs.cs
- MultiPartWriter.cs
- GeneralTransform3DGroup.cs
- RuntimeArgument.cs
- SqlDataSourceRefreshSchemaForm.cs
- DesignerUtility.cs
- Menu.cs
- TrustLevel.cs
- CellPartitioner.cs
- WebPartZone.cs
- PropertyReferenceExtension.cs
- ExpressionBinding.cs
- XNameConverter.cs
- QueryCacheKey.cs
- Stack.cs
- DbDataReader.cs
- DataBindingList.cs
- CustomWebEventKey.cs
- ExpressionStringBuilder.cs
- SimplePropertyEntry.cs
- StandardOleMarshalObject.cs
- TimestampInformation.cs
- ToolBarOverflowPanel.cs
- List.cs
- XComponentModel.cs
- NoResizeHandleGlyph.cs
- _NegoStream.cs
- UIServiceHelper.cs
- ContentType.cs
- SimpleTypesSurrogate.cs
- DataGridCommandEventArgs.cs
- FixedSOMPageConstructor.cs
- UdpUtility.cs
- PerfCounters.cs
- LinqDataSourceContextEventArgs.cs
- InvalidPropValue.cs
- DrawListViewColumnHeaderEventArgs.cs
- DeferredReference.cs
- TableRowGroup.cs
- PathFigure.cs
- HttpApplication.cs
- ThicknessKeyFrameCollection.cs
- HealthMonitoringSectionHelper.cs
- IntegerValidator.cs
- LifetimeServices.cs
- ApplicationInfo.cs
- EventData.cs
- SerializationSectionGroup.cs
- FieldNameLookup.cs
- OdbcParameterCollection.cs
- InfoCardRequestException.cs
- JournalEntryStack.cs
- KeyboardEventArgs.cs
- NavigationService.cs
- InputEventArgs.cs
- DbConnectionHelper.cs
- ListMarkerSourceInfo.cs
- DirectoryRootQuery.cs
- CopyNodeSetAction.cs
- WebPartDisplayModeCollection.cs
- ObjectDataSourceFilteringEventArgs.cs
- PermissionSetEnumerator.cs
- HtmlToClrEventProxy.cs
- BufferModesCollection.cs
- FlagsAttribute.cs
- SettingsBase.cs
- Peer.cs
- ColorContextHelper.cs
- ConfigDefinitionUpdates.cs
- Instrumentation.cs
- DummyDataSource.cs
- PropertyToken.cs
- ObjectItemCollection.cs
- DetailsViewActionList.cs
- ServicePrincipalNameElement.cs