Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebControls / DetailsViewActionList.cs / 1 / DetailsViewActionList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Web.UI.Design; using System.Web.UI.Design.Util; using System.Web.UI.WebControls; using System.Windows.Forms; ///internal class DetailsViewActionList : DesignerActionList { private DetailsViewDesigner _detailsViewDesigner; private bool _allowDeleting; private bool _allowEditing; private bool _allowInserting; private bool _allowPaging; private bool _allowRemoveField; private bool _allowMoveUp; private bool _allowMoveDown; /// public DetailsViewActionList(DetailsViewDesigner detailsViewDesigner) : base(detailsViewDesigner.Component) { _detailsViewDesigner = detailsViewDesigner; } /// /// Lets the DetailsView designer specify whether the Delete action should be visible/enabled /// internal bool AllowDeleting { get { return _allowDeleting; } set { _allowDeleting = value; } } ////// Lets the DetailsView designer specify whether the Edit action should be visible/enabled /// internal bool AllowEditing { get { return _allowEditing; } set { _allowEditing = value; } } ////// Lets the DetailsView designer specify whether the Insert action should be visible/enabled /// internal bool AllowInserting { get { return _allowInserting; } set { _allowInserting = value; } } ////// Lets the DetailsView designer specify whether the Move Down action should be visible/enabled /// internal bool AllowMoveDown { get { return _allowMoveDown; } set { _allowMoveDown = value; } } ////// Lets the DetailsView designer specify whether the Move Up action should be visible/enabled /// internal bool AllowMoveUp { get { return _allowMoveUp; } set { _allowMoveUp = value; } } ////// Lets the DetailsView designer specify whether the Page action should be visible/enabled /// internal bool AllowPaging { get { return _allowPaging; } set { _allowPaging = value; } } ////// Lets the DetailsView designer specify whether the Remove Field action should be visible/enabled /// internal bool AllowRemoveField { get { return _allowRemoveField; } set { _allowRemoveField = value; } } public override bool AutoShow { get { return true; } set { } } ////// /// Property used by chrome to display the delete checkbox. Called through reflection. /// public bool EnableDeleting { get { return _detailsViewDesigner.EnableDeleting; } set { _detailsViewDesigner.EnableDeleting = value; } } ////// /// Property used by chrome to display the delete checkbox. Called through reflection. /// public bool EnableEditing { get { return _detailsViewDesigner.EnableEditing; } set { _detailsViewDesigner.EnableEditing = value; } } ////// /// Property used by chrome to display the insert checkbox. Called through reflection. /// public bool EnableInserting { get { return _detailsViewDesigner.EnableInserting; } set { _detailsViewDesigner.EnableInserting = value; } } ////// /// Property used by chrome to display the page checkbox. Called through reflection. /// public bool EnablePaging { get { return _detailsViewDesigner.EnablePaging; } set { _detailsViewDesigner.EnablePaging = value; } } ////// /// Handler for the Add new field action. Called through reflection. /// public void AddNewField() { _detailsViewDesigner.AddNewField(); } ////// /// Handler for the edit fields action. Called through reflection. /// public void EditFields() { _detailsViewDesigner.EditFields(); } ////// /// Handler for the move field up action. Called through reflection. /// public void MoveFieldUp() { _detailsViewDesigner.MoveUp(); } ////// /// Handler for the move field down action. Called through reflection. /// public void MoveFieldDown() { _detailsViewDesigner.MoveDown(); } ////// /// Handler for the remove field action. Called through reflection. /// public void RemoveField() { _detailsViewDesigner.RemoveField(); } ///public override DesignerActionItemCollection GetSortedActionItems() { DesignerActionItemCollection items = new DesignerActionItemCollection(); items.Add(new DesignerActionMethodItem(this, "EditFields", SR.GetString(SR.DetailsView_EditFieldsVerb), "Action", SR.GetString(SR.DetailsView_EditFieldsDesc))); items.Add(new DesignerActionMethodItem(this, "AddNewField", SR.GetString(SR.DetailsView_AddNewFieldVerb), "Action", SR.GetString(SR.DetailsView_AddNewFieldDesc))); if (AllowMoveUp) { items.Add(new DesignerActionMethodItem(this, "MoveFieldUp", SR.GetString(SR.DetailsView_MoveFieldUpVerb), "Action", SR.GetString(SR.DetailsView_MoveFieldUpDesc))); } if (AllowMoveDown) { items.Add(new DesignerActionMethodItem(this, "MoveFieldDown", SR.GetString(SR.DetailsView_MoveFieldDownVerb), "Action", SR.GetString(SR.DetailsView_MoveFieldDownDesc))); } if (AllowRemoveField) { items.Add(new DesignerActionMethodItem(this, "RemoveField", SR.GetString(SR.DetailsView_RemoveFieldVerb), "Action", SR.GetString(SR.DetailsView_RemoveFieldDesc))); } if (AllowPaging) { items.Add(new DesignerActionPropertyItem("EnablePaging", SR.GetString(SR.DetailsView_EnablePaging), "Behavior", SR.GetString(SR.DetailsView_EnablePagingDesc))); } if (AllowInserting) { items.Add(new DesignerActionPropertyItem("EnableInserting", SR.GetString(SR.DetailsView_EnableInserting), "Behavior", SR.GetString(SR.DetailsView_EnableInsertingDesc))); } if (AllowEditing) { items.Add(new DesignerActionPropertyItem("EnableEditing", SR.GetString(SR.DetailsView_EnableEditing), "Behavior", SR.GetString(SR.DetailsView_EnableEditingDesc))); } if (AllowDeleting) { items.Add(new DesignerActionPropertyItem("EnableDeleting", SR.GetString(SR.DetailsView_EnableDeleting), "Behavior", SR.GetString(SR.DetailsView_EnableDeletingDesc))); } return items; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
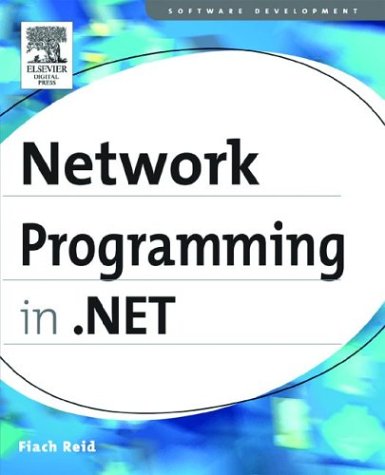
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VoiceChangeEventArgs.cs
- TextParagraphProperties.cs
- PhysicalFontFamily.cs
- TableRow.cs
- DbConvert.cs
- MouseOverProperty.cs
- SqlSelectStatement.cs
- Point3DAnimationBase.cs
- MatrixConverter.cs
- Solver.cs
- DataGridViewImageCell.cs
- SecurityPolicySection.cs
- WebPartCatalogAddVerb.cs
- InternalBufferOverflowException.cs
- CodeDOMProvider.cs
- WorkflowInstance.cs
- TextContainerChangedEventArgs.cs
- DigestTraceRecordHelper.cs
- AddInPipelineAttributes.cs
- ConstrainedDataObject.cs
- RemoteHelper.cs
- Span.cs
- SystemUnicastIPAddressInformation.cs
- TraceContext.cs
- _Win32.cs
- XPathScanner.cs
- AsmxEndpointPickerExtension.cs
- DesignSurfaceManager.cs
- Configuration.cs
- XmlUnspecifiedAttribute.cs
- FixedSOMTableCell.cs
- WebScriptEnablingBehavior.cs
- LinqDataSourceHelper.cs
- DurableErrorHandler.cs
- IriParsingElement.cs
- DrawingContextDrawingContextWalker.cs
- AncillaryOps.cs
- BorderGapMaskConverter.cs
- TextTreeInsertElementUndoUnit.cs
- ClientOptions.cs
- IdnElement.cs
- GridViewCellAutomationPeer.cs
- MaskedTextBoxDesignerActionList.cs
- TableItemPatternIdentifiers.cs
- SpStreamWrapper.cs
- BrowserCapabilitiesFactoryBase.cs
- MetadataCacheItem.cs
- SqlCacheDependencyDatabaseCollection.cs
- DetailsViewDeleteEventArgs.cs
- CompatibleComparer.cs
- util.cs
- VScrollBar.cs
- Pen.cs
- PartialCachingAttribute.cs
- HttpClientCertificate.cs
- XmlSchemaSequence.cs
- SharedStatics.cs
- GestureRecognizer.cs
- PrintEvent.cs
- MediaScriptCommandRoutedEventArgs.cs
- ObjectCacheHost.cs
- XmlSchemaObjectCollection.cs
- NativeMethods.cs
- WorkflowIdleBehavior.cs
- AdapterDictionary.cs
- DataGridBoolColumn.cs
- Point4DValueSerializer.cs
- RegexInterpreter.cs
- InfoCardKeyedHashAlgorithm.cs
- FontEditor.cs
- HtmlToClrEventProxy.cs
- Config.cs
- MemberMemberBinding.cs
- TextContainerChangedEventArgs.cs
- DynamicMethod.cs
- _Win32.cs
- SmtpNetworkElement.cs
- DataAdapter.cs
- FixedTextPointer.cs
- ErrorFormatter.cs
- ViewStateModeByIdAttribute.cs
- PointIndependentAnimationStorage.cs
- SingleObjectCollection.cs
- ExtendedProperty.cs
- ZipFileInfoCollection.cs
- LoginUtil.cs
- ZoneButton.cs
- WindowsAuthenticationModule.cs
- ZipPackage.cs
- RuntimeArgumentHandle.cs
- PairComparer.cs
- NumberFormatter.cs
- TimersDescriptionAttribute.cs
- PauseStoryboard.cs
- AlternationConverter.cs
- XmlResolver.cs
- WindowsAuthenticationModule.cs
- ProvidePropertyAttribute.cs
- WinOEToolBoxItem.cs
- Brush.cs