Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / DataRowIndexBuffer.cs / 1 / DataRowIndexBuffer.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Specialized; using System.Collections.Generic; using System.IO; using System.Runtime.InteropServices; using System.Threading; // // Summary: // Class to manage the collection of IndexObjects that // are bound to a DataRow. // // Remarks: // Should never be invoked. Use DataRow instead. // internal class DataRowIndexBuffer { Dictionary> m_objects; public DataRowIndexBuffer( ) { m_objects = new Dictionary >(); } // // Summary: // Returns the list of names for all indexes that have IndexObjects // associated with them. // // Remarks: // // // Returns: // Copy of the names of all indexes that have IndexObjects // public string[] GetIndexNames() { List keys = new List ( m_objects.Keys ); return keys.ToArray(); } // // Summary: // Returns a pointer to the actual inner list of IndexObjects. // // Remarks: // If you are using the list returned from this method, you should // modify only before saving. // // Paramters: // name: Name of the index you want // // Returns: // The innerlist for a given index. // public List this[ string name ] { get { if( !m_objects.ContainsKey( name ) ) { m_objects.Add( name, new List () ); } return m_objects[ name ]; } } // // Summary: // Clears All index values from all indexes // public void Clear() { m_objects.Clear(); } // // Summary: // Clears all values from a specific index. // // Remarks: // // Paramters: // name: Name of the index you want to clear. public void ClearIndexValues( string name ) { m_objects.Remove( name ); } // // Summary: // Adds a single index object to a specific index. // // Remarks: // // Parameters: // name: Name of the index to add the value to // obj: The index object to add. // public void AddIndexValue( string name, IndexObject obj ) { this[ name ].Add( obj ); } // // Summary: // Adds a range of index objects to a specific index. // // Remarks: // // Parameters: // name: Name of the index to add the value to // objs: The index object array to add. // public void AddIndexValues( string name, IndexObject[] objs ) { this[ name ].AddRange( objs ); } // // Summary: // Set a range of index objects to a specific index. // // Remarks: // This will clear the list before adding all of the values. // // Parameters: // name: Name of the index to add the value to // indexObjects: The index object array to add. // public void SetIndexValues( string name, IndexObject[] indexObjects ) { this[ name ].Clear(); this[ name ].AddRange( indexObjects ); } // // Summary: // Gets the count of index valus in a given index. // // Remarks: // // Paramters: // name: The name of the index to get the count for. // // Returns: // The count of index values for the specified index. // public int GetValueCount( string name ) { if( !m_objects.ContainsKey( name ) ) { return 0; } return m_objects[ name ].Count; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
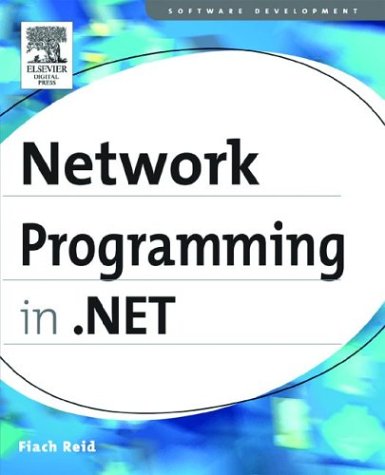
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpModuleCollection.cs
- TextAction.cs
- AnnotationMap.cs
- CodeSnippetStatement.cs
- NetworkCredential.cs
- IntranetCredentialPolicy.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- MultidimensionalArrayItemReference.cs
- XmlDocumentViewSchema.cs
- TextViewSelectionProcessor.cs
- LinkLabel.cs
- SecurityAlgorithmSuiteConverter.cs
- URIFormatException.cs
- TypedTableBaseExtensions.cs
- CellTreeNodeVisitors.cs
- Authorization.cs
- WebPartHeaderCloseVerb.cs
- XXXInfos.cs
- ObjectContextServiceProvider.cs
- ComboBoxAutomationPeer.cs
- Maps.cs
- XmlBindingWorker.cs
- RoleGroupCollection.cs
- TextBoxBase.cs
- XmlIncludeAttribute.cs
- Calendar.cs
- DesignerTextBoxAdapter.cs
- PassportAuthenticationModule.cs
- Ports.cs
- TextBox.cs
- ClipboardProcessor.cs
- Perspective.cs
- HttpsChannelFactory.cs
- DataKeyArray.cs
- Pkcs9Attribute.cs
- SqlConnection.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- XsltContext.cs
- TextEditorMouse.cs
- PositiveTimeSpanValidatorAttribute.cs
- StateRuntime.cs
- EntityContainerEmitter.cs
- EntityDataSourceContextDisposingEventArgs.cs
- IUnknownConstantAttribute.cs
- PtsPage.cs
- Operators.cs
- RelatedCurrencyManager.cs
- IndicCharClassifier.cs
- TreeViewItemAutomationPeer.cs
- ImageSourceConverter.cs
- BinHexDecoder.cs
- RemotingClientProxy.cs
- TaskFileService.cs
- ByteAnimationBase.cs
- ChildrenQuery.cs
- EventLogEntryCollection.cs
- DataPagerFieldCommandEventArgs.cs
- Activator.cs
- ActivityDesignerHelper.cs
- PointCollection.cs
- ExpressionVisitor.cs
- AvtEvent.cs
- DrawingBrush.cs
- HtmlGenericControl.cs
- PersonalizationStateInfo.cs
- ListView.cs
- PolicyValidator.cs
- SQLInt64.cs
- TransactionBridgeSection.cs
- InkCanvasFeedbackAdorner.cs
- SchemaCreator.cs
- HttpResponseInternalWrapper.cs
- MenuItem.cs
- AssertFilter.cs
- SiteMapNodeItemEventArgs.cs
- ComplexType.cs
- ProviderMetadata.cs
- DrawToolTipEventArgs.cs
- OrCondition.cs
- HttpRequest.cs
- AppDomainCompilerProxy.cs
- HMACRIPEMD160.cs
- _CacheStreams.cs
- ReachPrintTicketSerializerAsync.cs
- Vector.cs
- WebPermission.cs
- CapabilitiesState.cs
- GradientStopCollection.cs
- RangeValuePattern.cs
- SendingRequestEventArgs.cs
- PathData.cs
- BeginEvent.cs
- ThreadStaticAttribute.cs
- XmlSchemaException.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- RuntimeHandles.cs
- WmlObjectListAdapter.cs
- SqlNodeTypeOperators.cs
- Privilege.cs
- Unit.cs