Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / AccessibilityHelperForXpWin2k3.cs / 1 / AccessibilityHelperForXpWin2k3.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Text; using Microsoft.Win32; using System.Diagnostics; // Process using System.Runtime.ConstrainedExecution; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Collections.Generic; using System.Security.Principal; //WindowsIdentity using Microsoft.InfoCards; using Microsoft.InfoCards.Diagnostics; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.ComponentModel; // // Summary: // This class will manage the lifetimes of the accessibility applications // on the InfoCard desktop. // internal class AccessibilityHelperForXpWin2k3: IAccessibilityHelper, IDisposable { internal struct DownlevelAtData { public DownlevelAtData( string path, string image, string additional ) { RegistryPath = path; Image = image; AdditionalImage = additional; } public string RegistryPath; public string Image; public string AdditionalImage; }; static readonly string systemPath = Environment.GetFolderPath( Environment.SpecialFolder.System ); static readonly string baseRegistryPath = @"\SOFTWARE\Microsoft\Utility Manager\"; static readonly string keyName = @"Start on locked desktop"; internal static DownlevelAtData[] atApplications = new DownlevelAtData[ 3 ] { new DownlevelAtData( "On-Screen Keyboard", "osk.exe", "msswchx" ), new DownlevelAtData( "Magnifier", "magnify.exe", null ), new DownlevelAtData( "Narrator", "narrator.exe", null ) }; const int OSKIndex = 0; // **** This should always be index of OSK.exe // **** We need this for tablet PC scenarios Listm_restartList = new List (); ProcessManager m_manager; bool m_fTabletPC = false; // // Summary: // Constructs a new XP/Win2k3 Accessibility helper object. // // Parameters: // fTabletPC - If true, we are running on a tablet. // public AccessibilityHelperForXpWin2k3( bool fTabletPC ) { m_fTabletPC = fTabletPC; } void IAccessibilityHelper.Stop() { // // Stop the applications by simply terminating the job // if( null != m_manager ) { IDT.TraceDebug( "ICARDACCESS:Stopping the AT job if any AT apps are still running" ); m_manager.Dispose(); m_manager = null; } } bool IAccessibilityHelper.RestartOnUsersDesktop( uint userProcessId, string userDesktop, WindowsIdentity userIdentity ) { IDT.Assert( null == m_manager, "The AT applications must be terminated before they can be restarted" ); // // Restart the previously running applications // on the user's desktop. // This code be called in a situation where the caller was already // running as LSA so specify false to SystemIdentity constructor. // using( SystemIdentity lsa = new SystemIdentity( false ) ) { foreach( int i in m_restartList ) { // // Start the application on the infocard desktop with the // trusted user token. // string fullpath = Path.Combine( systemPath, atApplications[ i ].Image ); int processId = 0; uint error = NativeMcppMethods.CreateProcessAsUserHelperWrapper( fullpath, "", userProcessId, userDesktop, userIdentity.Name, ref processId ); if( 0 == error ) { IDT.TraceDebug( "ICARDACCESS:{0} restarted", atApplications[ i ].Image ); } else { IDT.TraceDebug( "ICARDACCESS:{0} failed to restart.", atApplications[ i ].Image ); // // Treat a failure to launch an AT application as non-fatal // } } } m_restartList.Clear(); // // This is always false as we do not want the agent to send any // to do anything more. // return false; } void IAccessibilityHelper.RestartOnInfoCardDesktop( uint ATApplicationFlags, SafeNativeHandle hTrustedUserToken, ref string trustedUserSid, string infocardDesktop, int userSessionId, uint userProcessId, WindowsIdentity userIdentity ) { // // I've specified false here (don't throw if already system) since this code // is currently being called on a new thread. Moving forward, the timing issues // associated with launching this should allow this to be called directly after the // desktop is created. ([....]) // using( SystemIdentity lsa = new SystemIdentity( false ) ) { IDT.Assert( null == m_manager, "The AT applications are already started" ); m_restartList.Clear(); bool fOSKStarted = false; string sid = userIdentity.User.Value; for( int i = 0; i < atApplications.Length; i++ ) { using( RegistryKey rk = Registry.Users.OpenSubKey( sid + baseRegistryPath + atApplications[ i ].RegistryPath ) ) { bool imageRunning = false; int? refValue = null; if ( ( null != rk ) && ( RegistryValueKind.DWord == rk.GetValueKind( keyName ) ) ) { refValue = (int) rk.GetValue(keyName); } // // We do need to kill and restart AT applications if we are on tablet PC, // and OSK is running even though it is not configured to run. // if ( (null != refValue && 1 == refValue) || ( m_fTabletPC && OSKIndex == i ) ) { // // Find and Kill the process on the user's desktop // foreach( Process p in Process.GetProcessesByName( atApplications[ i ].Image.Substring( 0, atApplications[ i ].Image.LastIndexOf( '.' ) ) ) ) { imageRunning = false; if( userSessionId == p.SessionId ) { // // Indicate that image was running. We will need // this information if additional images need to be killed // for this particular AT application. Currnetly only OSK // needs this. // imageRunning = true; m_restartList.Add( i ); // // Utility will handle exceptions correctly. // if( Utility.KillHelper( p ) ) { IDT.TraceDebug( "ICARDACCESS:Killed {0} on desktop for user {1}", atApplications[ i ].Image, sid ); } break; } } // // If we found the image and it has an additionalImage, see if // the additional image is running and kill it. // if( imageRunning && null != atApplications[ i ].AdditionalImage ) { foreach( Process p in Process.GetProcessesByName( atApplications[ i ].AdditionalImage ) ) { if( userSessionId == p.SessionId ) { // // Just kill the process, no need to add to restart list as this // process is never launched directly. // KillHelper will handle exceptions properly. // if( Utility.KillHelper( p ) ) { IDT.TraceDebug( "ICARDACCESS:Added {0} to kill list on desktop for user {1}", atApplications[ i ].Image, sid ); break; } } } } // // Start the application on the infocard desktop with the // trusted user token only if application is set to start // as per the registry and the AT application flags indicate // that AT applications are enabled. // if( null != refValue && 1 == refValue && 0 != ATApplicationFlags ) { string fullpath = Path.Combine( systemPath, atApplications[ i ].Image ); if( null == m_manager ) { m_manager = new ProcessManager( userSessionId, trustedUserSid ); } bool fUseElevatedToken = false; m_manager.AddProcess( hTrustedUserToken, ref trustedUserSid, infocardDesktop, userProcessId, userIdentity, fullpath, "", // no command line fUseElevatedToken ); if( OSKIndex == i ) { fOSKStarted = true; } } else { IDT.TraceDebug( "ICARDACCESS:{0} not launched for user {1}", atApplications[ i ].Image, sid ); } } } } // // Handle the special case of tablet PC. Only start it if // we have not already started it (that would be if AT apps // are enabled and we have OSK turned on). // if( m_fTabletPC && false == fOSKStarted ) { if( null == m_manager ) { m_manager = new ProcessManager( userSessionId, trustedUserSid ); } // // Downlevel we do not have the concept of an elevated token. // bool fUseElevatedToken = false; m_manager.AddProcess( hTrustedUserToken, ref trustedUserSid, infocardDesktop, userProcessId, userIdentity, Path.Combine( systemPath, atApplications[ OSKIndex ].Image ), "", // no command line fUseElevatedToken ); } } } public void Dispose() { if( null != m_manager ) { m_manager.Dispose(); m_manager = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
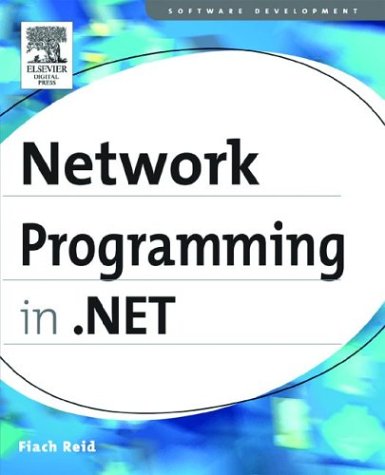
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigXmlCDataSection.cs
- TraceContextRecord.cs
- BitmapEffectRenderDataResource.cs
- ToolboxItem.cs
- SqlMethodAttribute.cs
- TCPListener.cs
- UnmanagedBitmapWrapper.cs
- TraceListeners.cs
- DataComponentGenerator.cs
- QueryConverter.cs
- FixedStringLookup.cs
- CommandField.cs
- CustomCategoryAttribute.cs
- AuthStoreRoleProvider.cs
- Security.cs
- ProtocolsSection.cs
- JavaScriptSerializer.cs
- PtsCache.cs
- ElementMarkupObject.cs
- ICspAsymmetricAlgorithm.cs
- ExtendedPropertyDescriptor.cs
- BlockExpression.cs
- WaitHandle.cs
- ConfigurationSectionGroup.cs
- CornerRadius.cs
- IIS7UserPrincipal.cs
- SerializationFieldInfo.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- WorkflowIdleElement.cs
- RuntimeHelpers.cs
- SmiEventStream.cs
- GridViewSelectEventArgs.cs
- PermissionSetTriple.cs
- Asn1IntegerConverter.cs
- InputScopeAttribute.cs
- AllowedAudienceUriElementCollection.cs
- InputLangChangeEvent.cs
- Renderer.cs
- hebrewshape.cs
- DuplexClientBase.cs
- GeneratedView.cs
- ConfigXmlComment.cs
- DashStyle.cs
- SR.cs
- DataBindEngine.cs
- LassoSelectionBehavior.cs
- WizardStepBase.cs
- SchemaType.cs
- DefaultExpressionVisitor.cs
- MarkedHighlightComponent.cs
- ContentElement.cs
- FlowchartSizeFeature.cs
- IntegerCollectionEditor.cs
- FastEncoderWindow.cs
- MetadataFile.cs
- Translator.cs
- MethodBody.cs
- PerfProviderCollection.cs
- ClassImporter.cs
- EntityStoreSchemaGenerator.cs
- SolidBrush.cs
- SqlServices.cs
- ClusterSafeNativeMethods.cs
- ClientUriBehavior.cs
- UriSection.cs
- LeafCellTreeNode.cs
- WorkflowRuntimeSection.cs
- PKCS1MaskGenerationMethod.cs
- OleDbStruct.cs
- ChannelTerminatedException.cs
- TemplateBindingExtension.cs
- StrokeCollectionDefaultValueFactory.cs
- EncodingConverter.cs
- ColorIndependentAnimationStorage.cs
- MergePropertyDescriptor.cs
- DataTableMappingCollection.cs
- ByteKeyFrameCollection.cs
- Cloud.cs
- LoggedException.cs
- EntitySetBase.cs
- SelectionProviderWrapper.cs
- PeerApplication.cs
- RelativeSource.cs
- ProcessModuleCollection.cs
- ClassDataContract.cs
- RepeatButton.cs
- ControlIdConverter.cs
- ToolStripItemCollection.cs
- RequestTimeoutManager.cs
- TextElementCollectionHelper.cs
- HGlobalSafeHandle.cs
- ByteKeyFrameCollection.cs
- ToolStripGripRenderEventArgs.cs
- ComponentEditorForm.cs
- WebPartVerbCollection.cs
- ComEventsInfo.cs
- Int64Converter.cs
- FunctionDescription.cs
- SoapObjectWriter.cs
- PathGeometry.cs