Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / AccessibilityHelperForVista.cs / 1 / AccessibilityHelperForVista.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Text; using Microsoft.Win32; using System.Diagnostics; // Process using System.Collections.Generic; using System.Security.Principal; //WindowsIdentity using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.ComponentModel; using Microsoft.InfoCards; using Microsoft.InfoCards.Diagnostics; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // This class will manage the lifetimes of the accessibility applications // on the InfoCard desktop on the Windows Vista platform. // internal class AccessibilityHelperForVista: IAccessibilityHelper, IDisposable { static readonly string systemPath = Environment.GetFolderPath( Environment.SpecialFolder.System ); static readonly string progfilePath = Environment.GetFolderPath( Environment.SpecialFolder.CommonProgramFiles ); static readonly string baseATPath = @"SOFTWARE\Microsoft\Windows NT\CurrentVersion\Accessibility\ATs"; static readonly string configPath = @"SOFTWARE\Microsoft\Windows NT\CurrentVersion\Accessibility\Session"; Listm_applicationList = new List (); ProcessManager m_manager; bool m_fResetConfigKey = false; int m_sessionId = 0; bool m_fTabletPC = false; // // Summary: // Constructs a new Vista Accessibility helper object. // // Parameters: // fTabletPC - If true, we are running on a tablet. // public AccessibilityHelperForVista( bool fTabletPC ) { m_fTabletPC = fTabletPC; InitializeATAppData(); } // // Summary: // Determines the list of AT applications from the registry. This is the // list we will look for when we are looking for apps to stop on the user // desktop. // private void InitializeATAppData() { // // This runs as system. Go through the list of applications in the LH // registry and determine the names of the applications. // RegistryKey rk = Registry.LocalMachine.OpenSubKey( baseATPath ); foreach( string subKeyName in rk.GetSubKeyNames() ) { RegistryKey subKey = rk.OpenSubKey( subKeyName ); { string exeName = (string) subKey.GetValue( "ATExe" ); if( !String.IsNullOrEmpty( exeName ) ) { m_applicationList.Add( exeName ); } } } } // // Summary: // Stops all the applications on the secure desktop by terminating the // job containing them. // void IAccessibilityHelper.Stop() { // // Stop the applications by simply terminating the job // if( null != m_manager ) { IDT.TraceDebug( "ICARDACCESS:Stopping the AT job" ); m_manager.Dispose(); m_manager = null; } } // // Summary: // Restart the applications on the user default desktop. // Return values: // TRUE - If AT apps need to be started. // FALSE - if no AT apps need to be stared. // // If True, Agent then needs to send WinKey + U to start the AT Apps. // // Params: // userProcessId - Calling user process Id. Used to determine // session Id further down the chain in native code. // userDesktop - Name of the userDesktop. // userIdentity - Identity of the user. // // Remarks: // Attempts to reset the config on the session key for AT apps and then // starts ATBroker.exe. See comments in code for resetting the config key. // bool IAccessibilityHelper.RestartOnUsersDesktop( uint userProcessId, string userDesktop, WindowsIdentity userIdentity ) { IDT.Assert( null == m_manager, "The AT applications must be terminated before they can be restarted" ); if( m_fResetConfigKey ) { // // We stopped apps, change the config key for the session so that // we can force atbroker to restart the AT applications. // When ATBroker is started, it looks at the user data for AT apps // and compares it with the session data. If these do not match, // then it starts the apps indicated in the user data. // RegistryKey rk = Registry.LocalMachine.OpenSubKey( configPath + m_sessionId, true ); string emptyString = ""; rk.SetValue( "Configuration", emptyString ); m_fResetConfigKey = false; // // In this case we want to let the agent know that agent // should signal utility manager for AT apps. // return true; } else { // // No AT apps running, do not signal utility manager. // return false; } } // // Summary: // Restarts the applications on the infocard desktop. First we kill // all the AT apps that are running on the default desktop and then we // launch ATBroker on our secure desktop. AT Broker automatically launches // the required AT applications on our desktop. // NOTE - we need the ATApplicationFlags from the client as the status // maintained in the server may not be the correct one for this session. // This is because we do want to keep AT application status constant for // a given client UI request (but user can update the settings in the middle // of the request). // // Parameters: // userATApplicationFlags - Flag indicating status of AT app support for current session. // hTrustedUserToken - Token with the trusted user SID allowed to be owner. // trustedUserSid - Stringized SID - used in the ACL for the process. // infocardDesktop - Desktop to launch the apps on. // userSessionID - Session ID for the user. // userIdentity - Calling user identity. // void IAccessibilityHelper.RestartOnInfoCardDesktop( uint userATApplicationFlags, SafeNativeHandle hTrustedUserToken, ref string trustedUserSid, string infocardDesktop, int userSessionId, uint userProcessId, WindowsIdentity userIdentity ) { // // I've specified false here (don't throw if already system) since this code // is currently being called on a new thread. Moving forward, the timing issues // associated with launching this should allow this to be called directly after the // desktop is created. ([....]) // using( SystemIdentity lsa = new SystemIdentity( false ) ) { IDT.Assert( null == m_manager, "The AT applications are already started" ); m_fResetConfigKey = false; // // Useful when we want to restart the AT apps on default Desktop. // m_sessionId = userSessionId; string sid = userIdentity.User.Value; // // See if any of the AT apps are runing on the default desktop // and terminate/kill them. // for( int i = 0; i < m_applicationList.Count; i++ ) { string appName = m_applicationList[ i ]; Process[] procs = Process.GetProcessesByName( appName.Substring( 0, appName.LastIndexOf( '.' ) ) ); if (null != procs) { foreach( Process p in procs ) { // // Find and Kill the process on the user's desktop // if( userSessionId == p.SessionId ) { // // If we killed any running applications, on // switching back to the default desktop, we need // to restart them, this flag will let us know if this // is necessary. // m_fResetConfigKey = true; // // Utility will handle exceptions correctly. // if( Utility.KillHelper( p ) ) { IDT.TraceDebug( "ICARDACCESS:Killed {0} on desktop for user {1}", appName, sid ); } break; } } } } if( null == m_manager ) { m_manager = new ProcessManager( userSessionId, trustedUserSid ); } string fullpath = Path.Combine( systemPath, "AtBroker.exe" ); bool fUseElevatedToken; // // Start the ATBroker application on the infocard desktop if AT application // support is enabled with the trusted user token. ATBroker will start all // the necessary AT applications in turn for us. // if( 0 != userATApplicationFlags ) { // // ProcessManager will create the process and keep track of the // process to control the lifetime. // fUseElevatedToken = false; m_manager.AddProcess( hTrustedUserToken, ref trustedUserSid, infocardDesktop, userProcessId, userIdentity, fullpath, "", // no command line fUseElevatedToken ); } // if ATApplications are enabled if ( m_fTabletPC ) { // // Note - wisptis.exe should be started on the private desktop // before tabtip can be started. We cannot start wisptis.exe here // because we need to use ShelLExec to start it. Wisptis.exe requires // UI Label and ShellExec will take care of tht for us. // fullpath = Path.Combine( progfilePath, "microsoft shared\\ink\\tabtip.exe" ); fUseElevatedToken = true; m_manager.AddProcess( hTrustedUserToken, ref trustedUserSid, infocardDesktop, userProcessId, userIdentity, fullpath, "/SeekDesktop:", fUseElevatedToken ); } // tablet PC applications. } } public void Dispose() { if( null != m_manager ) { m_manager.Dispose(); m_manager = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
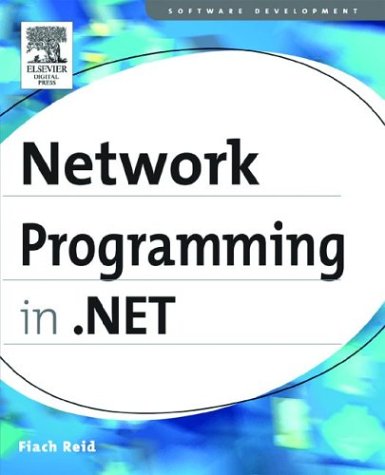
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- App.cs
- Tuple.cs
- ObjectViewFactory.cs
- SqlTransaction.cs
- PersonalizableAttribute.cs
- ConfigurationSectionCollection.cs
- MemberHolder.cs
- EntityConnection.cs
- AnnotationHelper.cs
- VectorAnimationUsingKeyFrames.cs
- ExternalException.cs
- SchemaTypeEmitter.cs
- OLEDB_Util.cs
- MembershipSection.cs
- DataGridCommandEventArgs.cs
- DeviceContexts.cs
- User.cs
- NumericExpr.cs
- EventRoute.cs
- DataGridCaption.cs
- PeerResolverSettings.cs
- StringUtil.cs
- LoginCancelEventArgs.cs
- ExpandCollapsePattern.cs
- MobileUserControl.cs
- ArrayEditor.cs
- BitmapEffectRenderDataResource.cs
- _TransmitFileOverlappedAsyncResult.cs
- DoubleMinMaxAggregationOperator.cs
- CodeVariableDeclarationStatement.cs
- X509Extension.cs
- SemanticBasicElement.cs
- LoginDesignerUtil.cs
- ExtractedStateEntry.cs
- HostingEnvironmentWrapper.cs
- _DigestClient.cs
- TreeViewCancelEvent.cs
- URLMembershipCondition.cs
- XmlTextEncoder.cs
- Itemizer.cs
- XNodeNavigator.cs
- DynamicValueConverter.cs
- DispatcherTimer.cs
- PathGeometry.cs
- DesignerAttribute.cs
- ModifyActivitiesPropertyDescriptor.cs
- ObjectMemberMapping.cs
- RemotingServices.cs
- _DisconnectOverlappedAsyncResult.cs
- ClassHandlersStore.cs
- DataGridCell.cs
- httpstaticobjectscollection.cs
- TypeListConverter.cs
- PermissionListSet.cs
- arc.cs
- NativeMethods.cs
- FileDialogCustomPlacesCollection.cs
- BinaryExpression.cs
- XmlValueConverter.cs
- HttpModule.cs
- DesignerResources.cs
- EntityDataSourceSelectingEventArgs.cs
- AnchoredBlock.cs
- WebConfigurationHostFileChange.cs
- EditingMode.cs
- DocumentSequenceHighlightLayer.cs
- ChildChangedEventArgs.cs
- CqlErrorHelper.cs
- SessionEndingEventArgs.cs
- HandlerFactoryCache.cs
- AlternateViewCollection.cs
- EventDescriptorCollection.cs
- TemplatedWizardStep.cs
- TableRowCollection.cs
- DataSourceConverter.cs
- SmtpClient.cs
- WhitespaceReader.cs
- PresentationSource.cs
- COM2ExtendedTypeConverter.cs
- OnOperation.cs
- GlobalizationSection.cs
- WebCategoryAttribute.cs
- XPathDocumentIterator.cs
- OutputCacheSection.cs
- CodeAttributeDeclarationCollection.cs
- SafeProcessHandle.cs
- DataGridColumn.cs
- ByValueEqualityComparer.cs
- RuleRefElement.cs
- ColorIndependentAnimationStorage.cs
- DesignerForm.cs
- Utility.cs
- DataRelation.cs
- OleDbFactory.cs
- _SslSessionsCache.cs
- StatusBarPanelClickEvent.cs
- DataGridViewHitTestInfo.cs
- TemplateBindingExpression.cs
- TemplateComponentConnector.cs
- FileUtil.cs