Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Protocol / ProtocolState.cs / 1 / ProtocolState.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file contains the class that represents all state for a particular // instance of the WS-AT protocol. This includes configuration and tracing // settings, all listeners, hash tables for transactions, etc. using System; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.ServiceModel; using System.ServiceModel.Channels; using System.Threading; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.InputOutput; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Recovery; using Microsoft.Transactions.Wsat.StateMachines; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.Protocol { // This class holds all protocol state accumulated during the lifetime of a provider class ProtocolState { Guid processId = Guid.NewGuid(); public Guid ProcessId { get { return processId; } } ProtocolVersion protocolVersion; public ProtocolVersion ProtocolVersion { get { return this.protocolVersion; } } TransactionManager tm; public TransactionManager TransactionManager { get { return tm; } } TransactionManagerReceive tmReceive; public TransactionManagerReceive TransactionManagerReceive { get { return tmReceive; } } TransactionManagerSend tmSend; public TransactionManagerSend TransactionManagerSend { get { return tmSend; } } Configuration config; public Configuration Config { get { return config; } } StateContainer allStates; public StateContainer States { get { return allStates; } } static PerformanceCounterHolder perfCounters = new PerformanceCounterHolder(); public PerformanceCounterHolder Perf { get { return perfCounters; } } LookupTables lookupTables; public LookupTables Lookup { get { return this.lookupTables; } } TimerManager timerManager; public TimerManager TimerManager { get { return timerManager; } } // We use the relative timespan from startup for all our time-dependent operations static Stopwatch clock = Stopwatch.StartNew(); public TimeSpan ElapsedTime { get { return clock.Elapsed; } } // // Listeners // CoordinationService coordination; ActivationCoordinator activationCoordinator; RegistrationCoordinator registrationCoordinator; RegistrationParticipant registrationParticipant; CompletionCoordinator completionCoordinator; TwoPhaseCommitCoordinator twoPhaseCommitCoordinator; TwoPhaseCommitParticipant twoPhaseCommitParticipant; ICoordinationListener activationCoordinatorListener; ICoordinationListener registrationCoordinatorListener; ICoordinationListener completionCoordinatorListener; ICoordinationListener twoPhaseCommitCoordinatorListener; ICoordinationListener twoPhaseCommitParticipantListener; public ActivationCoordinator ActivationCoordinator { get { return this.activationCoordinator; } } public RegistrationCoordinator RegistrationCoordinator { get { return this.registrationCoordinator; } } public RegistrationParticipant RegistrationParticipant { get { return this.registrationParticipant; } } public CompletionCoordinator CompletionCoordinator { get { return this.completionCoordinator; } } public TwoPhaseCommitCoordinator TwoPhaseCommitCoordinator { get { return this.twoPhaseCommitCoordinator; } } public TwoPhaseCommitParticipant TwoPhaseCommitParticipant { get { return this.twoPhaseCommitParticipant; } } public ICoordinationListener RegistrationCoordinatorListener { get { return this.registrationCoordinatorListener; } } public ICoordinationListener CompletionCoordinatorListener { get { return this.completionCoordinatorListener; } } public ICoordinationListener TwoPhaseCommitCoordinatorListener { get { return this.twoPhaseCommitCoordinatorListener; } } public ICoordinationListener TwoPhaseCommitParticipantListener { get { return this.twoPhaseCommitParticipantListener; } } public CoordinationService Service { get { return this.coordination; } } DebugTracingEventSink debugTrace = new DebugTracingEventSink(); public DebugTracingEventSink DebugTraceSink { get { return this.debugTrace; } } Faults faults; public Faults Faults { get { return this.faults; } } FaultSender faultsender; public FaultSender FaultSender { get { return this.faultsender; } } // // Recovery // QueuerecoveryQueue = new Queue (); bool recovering; public bool Recovering { get { return this.recovering; } } object recoveryLock = new object(); LogEntrySerialization serializer; public LogEntrySerialization LogEntrySerialization { get { return this.serializer; } } public ProtocolState(TransactionManager transactionManager, ProtocolVersion protocolVersion) { // version this.protocolVersion = protocolVersion; // PPL state this.tm = transactionManager; // Config try { this.config = new Configuration(this); } catch (ConfigurationProviderException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new PluggableProtocolException(e.Message, e)); } this.lookupTables = new LookupTables(this); this.tmReceive = new TransactionManagerReceive(this); this.tmSend = new TransactionManagerSend(this); this.timerManager = new TimerManager(this); // Recovery this.recovering = true; this.serializer = new LogEntrySerialization(this); this.allStates = new StateContainer(this); // Faults this.faults = Faults.Version(protocolVersion); this.faultsender = new FaultSender(this); // Listeners this.activationCoordinator = new ActivationCoordinator(this); this.registrationCoordinator = new RegistrationCoordinator(this); this.registrationParticipant = new RegistrationParticipant(this); this.completionCoordinator = new CompletionCoordinator(this); this.twoPhaseCommitCoordinator = new TwoPhaseCommitCoordinator(this); this.twoPhaseCommitParticipant = new TwoPhaseCommitParticipant(this); } public void Start() { DebugTrace.TraceEnter(this, "Start"); DebugTrace.TraceLeave(this, "Start"); } public void Stop() { DebugTrace.TraceEnter(this, "Stop"); StopListeners(); DebugTrace.TraceLeave(this, "Stop"); } void CleanupOnFailure() { StopListeners(); } void StopListeners() { DebugTrace.TraceEnter(this, "StopListeners"); if (this.twoPhaseCommitCoordinatorListener != null) { this.twoPhaseCommitCoordinatorListener.Stop(); this.twoPhaseCommitCoordinatorListener = null; } if (this.twoPhaseCommitParticipantListener != null) { this.twoPhaseCommitParticipantListener.Stop(); this.twoPhaseCommitParticipantListener = null; } if (this.completionCoordinatorListener != null) { this.completionCoordinatorListener.Stop(); this.completionCoordinatorListener = null; } if (this.registrationCoordinatorListener != null) { this.registrationCoordinatorListener.Stop(); this.registrationCoordinatorListener = null; } if (this.activationCoordinatorListener != null) { this.activationCoordinatorListener.Stop(); this.activationCoordinatorListener = null; } if (this.coordination != null) { this.coordination.Cleanup(); this.coordination = null; } DebugTrace.TraceLeave(this, "StopListeners"); } // // Recovery // public void RecoveryBeginning() { DebugTrace.TraceEnter(this, "RecoveryBeginning"); if (this.config.NetworkEndpointsEnabled) { try { this.coordination = new CoordinationService(config.PortConfiguration, this.protocolVersion); this.activationCoordinatorListener = this.coordination.Add(this.activationCoordinator); this.registrationCoordinatorListener = this.coordination.Add(this.registrationCoordinator); this.completionCoordinatorListener = this.coordination.Add(this.completionCoordinator); this.twoPhaseCommitCoordinatorListener = this.coordination.Add(this.twoPhaseCommitCoordinator); this.twoPhaseCommitParticipantListener = this.coordination.Add(this.twoPhaseCommitParticipant); } catch (MessagingInitializationException e) { if (DebugTrace.Error) DebugTrace.Trace(TraceLevel.Error, "Error initializing CoordinationService: {0}", e); // This is largely a no-op, but it's good practice to call anyway CleanupOnFailure(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new PluggableProtocolException(e.Message, e)); } catch (Exception e) { if (DebugTrace.Error) DebugTrace.Trace(TraceLevel.Error, "Unknown exception initializing CoordinationService: {0}", e); // This is largely a no-op, but it's good practice to call anyway CleanupOnFailure(); throw; } } // Note - we don't start our listeners until recovery completes DebugTrace.TraceLeave(this, "RecoveryBeginning"); } public void RecoveryComplete() { DebugTrace.TraceEnter(this, "RecoveryComplete"); if (this.config.NetworkEndpointsEnabled) { // This may throw PluggableProtocolException. // E.g., if our port configuration is incorrect StartListeners(); } // Nothing below here should throw lock (this.recoveryLock) { this.recovering = false; // Process any messages we might have queued during recovery ProcessPendingRecoveryEvents(); // We don't need the recovery queue anymore if (this.recoveryQueue.Count != 0) { // When recovery completes, there should be no more entries left in // the queue. If an entry is left in the queue, that is a product bug // that needs to be reported back to Microsoft. DiagnosticUtility.FailFast("Recovery queue should be empty"); } this.recoveryQueue = null; } DebugTrace.TraceLeave(this, "RecoveryComplete"); } void ProcessPendingRecoveryEvents() { DebugTrace.TraceEnter(this, "ProcessPendingRecoveryEvents"); if (this.recovering) { // Make sure that this method isn't called after calling RecoveryComplete. // To do otherwise is a product bug that needs to be reported back to Microsoft. DiagnosticUtility.FailFast("Cannot process recovery events while recovering"); } if (this.recoveryQueue.Count == 0) { DebugTrace.Trace(TraceLevel.Verbose, "No events were queued during recovery"); } else { DebugTrace.Trace(TraceLevel.Verbose, "Processing events queued during recovery"); while (this.recoveryQueue.Count > 0) { SynchronizationEvent e = this.recoveryQueue.Dequeue(); e.Enlistment.StateMachine.Enqueue(e); } } DebugTrace.TraceLeave(this, "ProcessPendingRecoveryEvents"); } public void EnqueueRecoveryReplay(TmReplayEvent e) { if (!this.recovering) { // Make sure that this method isn't called after calling RecoveryComplete. // To do otherwise is a product bug that needs to be reported back to Microsoft. DiagnosticUtility.FailFast("Cannot enqueue recovery event outside of recovery"); } if (DebugTrace.Info) { CoordinatorEnlistment coordinator = e.Coordinator; DebugTrace.TxTrace( TraceLevel.Info, coordinator.EnlistmentId, "Enqueuing recovery replay for coordinator at {0}", Ports.TryGetAddress(coordinator.CoordinatorProxy)); } this.recoveryQueue.Enqueue(e); } public bool TryEnqueueRecoveryOutcome(ParticipantCallbackEvent e) { // This can be called during recovery or after recovery lock (this.recoveryLock) { if (!this.recovering) { return false; } if (DebugTrace.Info) { ParticipantEnlistment participant = e.Participant; DebugTrace.TxTrace( TraceLevel.Info, participant.EnlistmentId, "Queuing recovery outcome {0} for participant at {1}", e, Ports.TryGetAddress(participant.ParticipantProxy)); } recoveryQueue.Enqueue(e); } return true; } void StartListeners() { DebugTrace.TraceEnter(this, "StartListeners"); try { this.twoPhaseCommitCoordinatorListener.Start(); this.twoPhaseCommitParticipantListener.Start(); this.completionCoordinatorListener.Start(); this.registrationCoordinatorListener.Start(); this.activationCoordinatorListener.Start(); } catch (MessagingInitializationException e) { if (DebugTrace.Error) DebugTrace.Trace(TraceLevel.Error, "Error starting a listener: {0}", e); CleanupOnFailure(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new PluggableProtocolException(e.Message, e)); } catch (Exception e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Error); if (DebugTrace.Error) DebugTrace.Trace(TraceLevel.Error, "Unknown exception starting a listener: {0}", e); CleanupOnFailure(); throw; } DebugTrace.TraceLeave(this, "StartListeners"); } // // Proxy creation // public CompletionParticipantProxy TryCreateCompletionParticipantProxy(EndpointAddress to) { try { return this.coordination.CreateCompletionParticipantProxy(to); } catch (CreateChannelFailureException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); if (DebugTrace.Warning) { DebugTrace.Trace( TraceLevel.Warning, "Could not create proxy to completion participant at {0}: {1}", to.Uri, e.Message ); } } return null; } public TwoPhaseCommitParticipantProxy TryCreateTwoPhaseCommitParticipantProxy(EndpointAddress to) { // Handle recovery correctly in situations where we have no network endpoints, // but DTC still wants us to recover a participant enlistment. if (!this.config.NetworkEndpointsEnabled) return null; try { return this.coordination.CreateTwoPhaseCommitParticipantProxy(to, null); } catch (CreateChannelFailureException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); if (DebugTrace.Warning) { DebugTrace.Trace( TraceLevel.Warning, "Could not create proxy to 2PC participant at {0}: {1}", to.Uri, e.Message ); } } return null; } public TwoPhaseCommitCoordinatorProxy TryCreateTwoPhaseCommitCoordinatorProxy(EndpointAddress to) { // Handle recovery correctly in situations where we have no network endpoints, // but DTC still wants us to recover a coordinator enlistment. if (!this.config.NetworkEndpointsEnabled) return null; try { return this.coordination.CreateTwoPhaseCommitCoordinatorProxy(to, null); } catch (CreateChannelFailureException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); if (DebugTrace.Warning) { DebugTrace.Trace( TraceLevel.Warning, "Could not create proxy to 2PC coordinator at {0}: {1}", to.Uri, e.Message ); } } return null; } public RegistrationProxy TryCreateRegistrationProxy(EndpointAddress to) { try { return this.coordination.CreateRegistrationProxy(to); } catch (CreateChannelFailureException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); if (DebugTrace.Warning) { DebugTrace.Trace( TraceLevel.Warning, "Could not create proxy to Registration coordinator at {0}: {1}", to.Uri, e.Message ); } } return null; } } // This class contains instances for all states in all state machines class StateContainer { // // Coordinator states // State coordinatorInitializing; public State CoordinatorInitializing { get { return coordinatorInitializing; } } State coordinatorEnlisting; public State CoordinatorEnlisting { get { return coordinatorEnlisting; } } State coordinatorEnlisted; public State CoordinatorEnlisted { get { return coordinatorEnlisted; } } State coordinatorRegisteringBoth; public State CoordinatorRegisteringBoth { get { return coordinatorRegisteringBoth; } } State coordinatorRegisteringDurable; public State CoordinatorRegisteringDurable { get { return coordinatorRegisteringDurable; } } State coordinatorRegisteringVolatile; public State CoordinatorRegisteringVolatile { get { return coordinatorRegisteringVolatile; } } State coordinatorVolatileActive; public State CoordinatorVolatileActive { get { return coordinatorVolatileActive; } } State coordinatorVolatilePreparing; public State CoordinatorVolatilePreparing { get { return coordinatorVolatilePreparing; } } State coordinatorVolatilePreparingRegistering; public State CoordinatorVolatilePreparingRegistering { get { return coordinatorVolatilePreparingRegistering; } } State coordinatorVolatilePreparingRegistered; public State CoordinatorVolatilePreparingRegistered { get { return coordinatorVolatilePreparingRegistered; } } State coordinatorActive; public State CoordinatorActive { get { return coordinatorActive; } } State coordinatorPreparing; public State CoordinatorPreparing { get { return coordinatorPreparing; } } State coordinatorPrepared; public State CoordinatorPrepared { get { return coordinatorPrepared; } } State coordinatorCommitting; public State CoordinatorCommitting { get { return coordinatorCommitting; } } State coordinatorRecovering; public State CoordinatorRecovering { get { return coordinatorRecovering; } } State coordinatorRecovered; public State CoordinatorRecovered { get { return coordinatorRecovered; } } State coordinatorAwaitingEndOfRecovery; public State CoordinatorAwaitingEndOfRecovery { get { return coordinatorAwaitingEndOfRecovery; } } State coordinatorFailedRecovery; public State CoordinatorFailedRecovery { get { return coordinatorFailedRecovery; } } State coordinatorCommitted; public State CoordinatorCommitted { get { return coordinatorCommitted; } } State coordinatorAborted; public State CoordinatorAborted { get { return coordinatorAborted; } } State coordinatorForgotten; public State CoordinatorForgotten { get { return coordinatorForgotten; } } State coordinatorReadOnlyInDoubt; public State CoordinatorReadOnlyInDoubt { get { return coordinatorReadOnlyInDoubt; } } State coordinatorInitializationFailed; public State CoordinatorInitializationFailed { get { return coordinatorInitializationFailed; } } // // Completion states // State completionInitializing; public State CompletionInitializing { get { return completionInitializing; } } State completionCreating; public State CompletionCreating { get { return completionCreating; } } State completionCreated; public State CompletionCreated { get { return completionCreated; } } State completionActive; public State CompletionActive { get { return completionActive; } } State completionCommitting; public State CompletionCommitting { get { return completionCommitting; } } State completionAborting; public State CompletionAborting { get { return completionAborting; } } State completionCommitted; public State CompletionCommitted { get { return completionCommitted; } } State completionAborted; public State CompletionAborted { get { return completionAborted; } } State completionInitializationFailed; public State CompletionInitializationFailed { get { return completionInitializationFailed; } } // // Subordinate states // State subordinateInitializing; public State SubordinateInitializing { get { return subordinateInitializing; } } State subordinateRegistering; public State SubordinateRegistering { get { return subordinateRegistering; } } State subordinateActive; public State SubordinateActive { get { return subordinateActive; } } State subordinateFinished; public State SubordinateFinished { get { return subordinateFinished; } } // // Durable states // State durableRegistering; public State DurableRegistering { get { return durableRegistering; } } State durableActive; public State DurableActive { get { return durableActive; } } State durableUnregistered; public State DurableUnregistered { get { return durableUnregistered; } } State durablePreparing; public State DurablePreparing { get { return durablePreparing; } } State durablePrepared; public State DurablePrepared { get { return durablePrepared; } } State durableCommitting; public State DurableCommitting { get { return durableCommitting; } } State durableRecovering; public State DurableRecovering { get { return durableRecovering; } } State durableRejoined; public State DurableRejoined { get { return durableRejoined; } } State durableRecoveryAwaitingCommit; public State DurableRecoveryAwaitingCommit { get { return durableRecoveryAwaitingCommit; } } State durableRecoveryReceivedCommit; public State DurableRecoveryReceivedCommit { get { return durableRecoveryReceivedCommit; } } State durableRecoveryAwaitingRollback; public State DurableRecoveryAwaitingRollback { get { return durableRecoveryAwaitingRollback; } } State durableRecoveryReceivedRollback; public State DurableRecoveryReceivedRollback { get { return durableRecoveryReceivedRollback; } } State durableFailedRecovery; public State DurableFailedRecovery { get { return durableFailedRecovery; } } State durableCommitted; public State DurableCommitted { get { return durableCommitted; } } State durableAborted; public State DurableAborted { get { return durableAborted; } } State durableInDoubt; public State DurableInDoubt { get { return durableInDoubt; } } State durableInitializationFailed; public State DurableInitializationFailed { get { return durableInitializationFailed; } } // // Volatile states // State volatileRegistering; public State VolatileRegistering { get { return volatileRegistering; } } State volatilePhaseZeroActive; public State VolatilePhaseZeroActive { get { return volatilePhaseZeroActive; } } State volatilePhaseZeroUnregistered; public State VolatilePhaseZeroUnregistered { get { return volatilePhaseZeroUnregistered; } } State volatilePhaseOneUnregistered; public State VolatilePhaseOneUnregistered { get { return volatilePhaseOneUnregistered; } } State volatilePrePreparing; public State VolatilePrePreparing { get { return volatilePrePreparing; } } State volatilePrePrepared; public State VolatilePrePrepared { get { return volatilePrePrepared; } } State volatilePrepared; public State VolatilePrepared { get { return volatilePrepared; } } State volatileCommitting; public State VolatileCommitting { get { return volatileCommitting; } } State volatileAborting; public State VolatileAborting { get { return volatileAborting; } } State volatileCommitted; public State VolatileCommitted { get { return volatileCommitted; } } State volatileAborted; public State VolatileAborted { get { return volatileAborted; } } State volatileInDoubt; public State VolatileInDoubt { get { return volatileInDoubt; } } State volatileInitializationFailed; public State VolatileInitializationFailed { get { return volatileInitializationFailed; } } // // Transaction context states // State transactionContextInitializing; public State TransactionContextInitializing { get { return transactionContextInitializing; } } State transactionContextInitializingCoordinator; public State TransactionContextInitializingCoordinator { get { return transactionContextInitializingCoordinator; } } State transactionContextActive; public State TransactionContextActive { get { return transactionContextActive; } } State transactionContextFinished; public State TransactionContextFinished { get { return transactionContextFinished; } } public StateContainer (ProtocolState state) { // Coordinator states coordinatorInitializing = new CoordinatorInitializing(state); coordinatorEnlisting = new CoordinatorEnlisting(state); coordinatorEnlisted = new CoordinatorEnlisted(state); coordinatorRegisteringBoth = new CoordinatorRegisteringBoth(state); coordinatorRegisteringDurable = new CoordinatorRegisteringDurable(state); coordinatorRegisteringVolatile = new CoordinatorRegisteringVolatile(state); coordinatorVolatileActive = new CoordinatorVolatileActive(state); coordinatorVolatilePreparing = new CoordinatorVolatilePreparing(state); coordinatorVolatilePreparingRegistering = new CoordinatorVolatilePreparingRegistering(state); coordinatorVolatilePreparingRegistered = new CoordinatorVolatilePreparingRegistered(state); coordinatorActive = new CoordinatorActive(state); coordinatorPreparing = new CoordinatorPreparing(state); coordinatorPrepared = new CoordinatorPrepared(state); coordinatorCommitting = new CoordinatorCommitting(state); coordinatorRecovering = new CoordinatorRecovering(state); coordinatorRecovered = new CoordinatorRecovered(state); coordinatorAwaitingEndOfRecovery = new CoordinatorAwaitingEndOfRecovery(state); coordinatorFailedRecovery = new CoordinatorFailedRecovery(state); coordinatorCommitted = new CoordinatorCommitted(state); coordinatorAborted = new CoordinatorAborted(state); coordinatorForgotten = new CoordinatorForgotten(state); coordinatorReadOnlyInDoubt = new CoordinatorReadOnlyInDoubt(state); coordinatorInitializationFailed = new CoordinatorInitializationFailed(state); // Completion states completionInitializing = new CompletionInitializing(state); completionCreating = new CompletionCreating(state); completionCreated = new CompletionCreated(state); completionActive = new CompletionActive(state); completionCommitting = new CompletionCommitting(state); completionAborting = new CompletionAborting(state); completionCommitted = new CompletionCommitted(state); completionAborted = new CompletionAborted(state); completionInitializationFailed = new CompletionInitializationFailed(state); // Subordinate states subordinateInitializing = new SubordinateInitializing(state); subordinateRegistering = new SubordinateRegistering(state); subordinateActive = new SubordinateActive(state); subordinateFinished = new SubordinateFinished(state); // Durable states durableRegistering = new DurableRegistering(state); durableActive = new DurableActive(state); durableUnregistered = new DurableUnregistered(state); durablePreparing = new DurablePreparing(state); durablePrepared = new DurablePrepared(state); durableCommitting = new DurableCommitting(state); durableRecovering = new DurableRecovering(state); durableRejoined = new DurableRejoined(state); durableRecoveryAwaitingCommit = new DurableRecoveryAwaitingCommit(state); durableRecoveryReceivedCommit = new DurableRecoveryReceivedCommit(state); durableRecoveryAwaitingRollback = new DurableRecoveryAwaitingRollback(state); durableRecoveryReceivedRollback = new DurableRecoveryReceivedRollback(state); durableFailedRecovery = new DurableFailedRecovery(state); durableCommitted = new DurableCommitted(state); durableAborted = new DurableAborted(state); durableInDoubt = new DurableInDoubt(state); durableInitializationFailed = new DurableInitializationFailed(state); // Volatile states volatileRegistering = new VolatileRegistering(state); volatilePhaseZeroActive = new VolatilePhaseZeroActive(state); volatilePhaseZeroUnregistered = new VolatilePhaseZeroUnregistered(state); volatilePhaseOneUnregistered = new VolatilePhaseOneUnregistered(state); volatilePrePreparing = new VolatilePrePreparing(state); volatilePrePrepared = new VolatilePrePrepared(state); volatilePrepared = new VolatilePrepared(state); volatileCommitting = new VolatileCommitting(state); volatileAborting = new VolatileAborting(state); volatileCommitted = new VolatileCommitted(state); volatileAborted = new VolatileAborted(state); volatileInDoubt = new VolatileInDoubt(state); volatileInitializationFailed = new VolatileInitializationFailed(state); // Transaction context states transactionContextInitializing = new TransactionContextInitializing(state); transactionContextInitializingCoordinator = new TransactionContextInitializingCoordinator(state); transactionContextActive = new TransactionContextActive(state); transactionContextFinished = new TransactionContextFinished(state); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
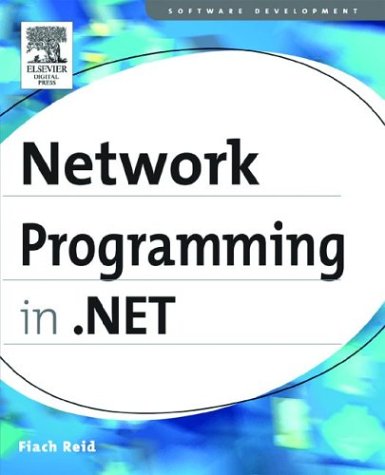
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigXmlDocument.cs
- PageThemeCodeDomTreeGenerator.cs
- UserControlBuildProvider.cs
- PngBitmapDecoder.cs
- DbConnectionInternal.cs
- UnicastIPAddressInformationCollection.cs
- OleDbStruct.cs
- PassportAuthenticationModule.cs
- InvalidCommandTreeException.cs
- TdsParserSafeHandles.cs
- MarkerProperties.cs
- IPCCacheManager.cs
- DataBoundControl.cs
- TimelineGroup.cs
- RequiredFieldValidator.cs
- DataColumnChangeEvent.cs
- MLangCodePageEncoding.cs
- ContextDataSourceView.cs
- Message.cs
- Asn1IntegerConverter.cs
- DrawingAttributes.cs
- BitmapImage.cs
- InterleavedZipPartStream.cs
- wmiprovider.cs
- ConsumerConnectionPointCollection.cs
- StyleXamlParser.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- translator.cs
- BCLDebug.cs
- SqlMethods.cs
- BaseParser.cs
- NavigationPropertyAccessor.cs
- ScriptBehaviorDescriptor.cs
- Pkcs7Recipient.cs
- XmlCompatibilityReader.cs
- BooleanAnimationUsingKeyFrames.cs
- ResourceContainer.cs
- FrameAutomationPeer.cs
- BamlRecordWriter.cs
- DecoderFallbackWithFailureFlag.cs
- RegexWriter.cs
- EventDescriptorCollection.cs
- UdpTransportBindingElement.cs
- QueryResults.cs
- ActiveXHelper.cs
- ResXResourceWriter.cs
- CodeVariableDeclarationStatement.cs
- PackagingUtilities.cs
- InvokeWebServiceDesigner.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- ObjectListSelectEventArgs.cs
- AVElementHelper.cs
- InvokePatternIdentifiers.cs
- DataGridViewSelectedColumnCollection.cs
- DynamicQueryableWrapper.cs
- NativeMethods.cs
- CopyCodeAction.cs
- XamlReader.cs
- DataTableReader.cs
- EntityDataSourceChangingEventArgs.cs
- DataSvcMapFile.cs
- LoginView.cs
- ArgumentOutOfRangeException.cs
- Int32Rect.cs
- FormViewRow.cs
- Converter.cs
- InvalidStoreProtectionKeyException.cs
- SafeFindHandle.cs
- RadioButton.cs
- ExceptionHelpers.cs
- ProfilePropertySettings.cs
- ComplexPropertyEntry.cs
- ImageFormatConverter.cs
- COM2EnumConverter.cs
- _Win32.cs
- TypeConverter.cs
- ControlPropertyNameConverter.cs
- MemoryMappedViewAccessor.cs
- ListViewInsertionMark.cs
- KnownBoxes.cs
- SignerInfo.cs
- SerializationHelper.cs
- ClrProviderManifest.cs
- DocumentEventArgs.cs
- ObjectDataSourceMethodEventArgs.cs
- RoleManagerEventArgs.cs
- DispatcherExceptionEventArgs.cs
- DiscoveryEndpointValidator.cs
- EventSource.cs
- ActivityExecutionFilter.cs
- GridViewHeaderRowPresenter.cs
- _FtpDataStream.cs
- WindowsListViewScroll.cs
- DataSourceGroupCollection.cs
- BitmapMetadata.cs
- PrintControllerWithStatusDialog.cs
- FrameworkElement.cs
- Calendar.cs
- ExpressionReplacer.cs
- ReferenceTypeElement.cs