Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / QueryResults.cs / 1305376 / QueryResults.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // QueryResults.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections; using System.Collections.Generic; using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// The QueryResults{T} is a class representing the results of the query. There may /// be different ways the query results can be manipulated. Currently, two ways are /// supported: /// /// 1. Open the query results as a partitioned stream by calling GivePartitionedStream /// and pass a generic action as an argument. /// /// 2. Access individual elements of the results list by calling GetElement(index) and /// ElementsCount. This method of accessing the query results is available only if /// IsIndexible return true. /// ///internal abstract class QueryResults : IList { //------------------------------------------------------------------------------------ // Gets the query results represented as a partitioned stream. Instead of returning // the PartitionedStream, we instead call recipient.Receive (...). That way, // the code that receives the partitioned stream has access to the TKey type. // // Arguments: // recipient - the object that the partitioned stream will be passed to // internal abstract void GivePartitionedStream(IPartitionedStreamRecipient recipient); //----------------------------------------------------------------------------------- // Returns whether the query results are indexible. If this property is true, the // user can call GetElement(index) and ElementsCount. If it is false, both // GetElement(index) and ElementsCount should throw InvalidOperationException. // internal virtual bool IsIndexible { get { return false; } } //----------------------------------------------------------------------------------- // Returns index-th element in the query results // // Assumptions: // IsIndexible returns true // 0 <= index < ElementsCount // internal virtual T GetElement(int index) { Contract.Assert(false, "GetElement property is not supported by non-indexible query results"); throw new NotSupportedException(); } //----------------------------------------------------------------------------------- // Returns the number of elements in the query results // // Assumptions: // IsIndexible returns true // internal virtual int ElementsCount { get { Contract.Assert(false, "ElementsCount property is not supported by non-indexible query results"); throw new NotSupportedException(); } } // // An assortment of methods we need to support in order to implement the IList interface // int IList .IndexOf(T item) { throw new NotSupportedException(); } void IList .Insert(int index, T item) { throw new NotSupportedException(); } void IList .RemoveAt(int index) { throw new NotSupportedException(); } public T this[int index] { get { return GetElement(index); } set { throw new NotSupportedException(); } } void ICollection .Add(T item) { throw new NotSupportedException(); } void ICollection .Clear() { throw new NotSupportedException(); } bool ICollection .Contains(T item) { throw new NotSupportedException(); } void ICollection .CopyTo(T[] array, int arrayIndex) { throw new NotSupportedException(); } public int Count { get { return ElementsCount; } } bool ICollection .IsReadOnly { get { return true; } } bool ICollection .Remove(T item) { throw new NotSupportedException(); } IEnumerator IEnumerable .GetEnumerator() { for (int index = 0; index < Count; index++) { yield return this[index]; } } IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // QueryResults.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections; using System.Collections.Generic; using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// The QueryResults{T} is a class representing the results of the query. There may /// be different ways the query results can be manipulated. Currently, two ways are /// supported: /// /// 1. Open the query results as a partitioned stream by calling GivePartitionedStream /// and pass a generic action as an argument. /// /// 2. Access individual elements of the results list by calling GetElement(index) and /// ElementsCount. This method of accessing the query results is available only if /// IsIndexible return true. /// ///internal abstract class QueryResults : IList { //------------------------------------------------------------------------------------ // Gets the query results represented as a partitioned stream. Instead of returning // the PartitionedStream, we instead call recipient.Receive (...). That way, // the code that receives the partitioned stream has access to the TKey type. // // Arguments: // recipient - the object that the partitioned stream will be passed to // internal abstract void GivePartitionedStream(IPartitionedStreamRecipient recipient); //----------------------------------------------------------------------------------- // Returns whether the query results are indexible. If this property is true, the // user can call GetElement(index) and ElementsCount. If it is false, both // GetElement(index) and ElementsCount should throw InvalidOperationException. // internal virtual bool IsIndexible { get { return false; } } //----------------------------------------------------------------------------------- // Returns index-th element in the query results // // Assumptions: // IsIndexible returns true // 0 <= index < ElementsCount // internal virtual T GetElement(int index) { Contract.Assert(false, "GetElement property is not supported by non-indexible query results"); throw new NotSupportedException(); } //----------------------------------------------------------------------------------- // Returns the number of elements in the query results // // Assumptions: // IsIndexible returns true // internal virtual int ElementsCount { get { Contract.Assert(false, "ElementsCount property is not supported by non-indexible query results"); throw new NotSupportedException(); } } // // An assortment of methods we need to support in order to implement the IList interface // int IList .IndexOf(T item) { throw new NotSupportedException(); } void IList .Insert(int index, T item) { throw new NotSupportedException(); } void IList .RemoveAt(int index) { throw new NotSupportedException(); } public T this[int index] { get { return GetElement(index); } set { throw new NotSupportedException(); } } void ICollection .Add(T item) { throw new NotSupportedException(); } void ICollection .Clear() { throw new NotSupportedException(); } bool ICollection .Contains(T item) { throw new NotSupportedException(); } void ICollection .CopyTo(T[] array, int arrayIndex) { throw new NotSupportedException(); } public int Count { get { return ElementsCount; } } bool ICollection .IsReadOnly { get { return true; } } bool ICollection .Remove(T item) { throw new NotSupportedException(); } IEnumerator IEnumerable .GetEnumerator() { for (int index = 0; index < Count; index++) { yield return this[index]; } } IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
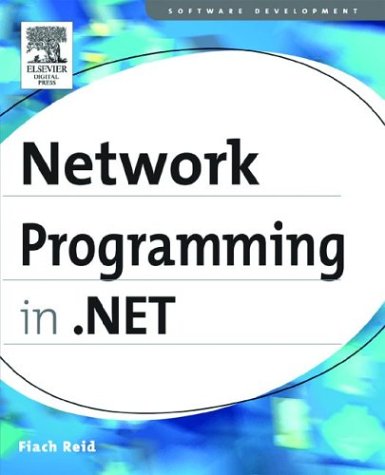
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeArgumentReferenceExpression.cs
- RectangleGeometry.cs
- WebPartVerbCollection.cs
- HttpException.cs
- SimpleType.cs
- Stackframe.cs
- Button.cs
- SqlDataSource.cs
- ClipboardData.cs
- EnumerableCollectionView.cs
- Selection.cs
- SizeIndependentAnimationStorage.cs
- DataGridViewCell.cs
- SspiNegotiationTokenAuthenticatorState.cs
- TransformValueSerializer.cs
- DataGridViewComboBoxColumn.cs
- Context.cs
- UnsafeNetInfoNativeMethods.cs
- Attributes.cs
- SelectionItemPattern.cs
- DictionarySectionHandler.cs
- InvocationExpression.cs
- SafeIUnknown.cs
- SafeProcessHandle.cs
- KeyConverter.cs
- Thickness.cs
- XmlDocumentFragment.cs
- ApplicationDirectoryMembershipCondition.cs
- Header.cs
- LeaseManager.cs
- CodeRegionDirective.cs
- TypeConverterValueSerializer.cs
- PrefixQName.cs
- HtmlInputSubmit.cs
- RenderData.cs
- XsltLoader.cs
- odbcmetadatafactory.cs
- ResourceIDHelper.cs
- Comparer.cs
- EdmTypeAttribute.cs
- _SafeNetHandles.cs
- OneToOneMappingSerializer.cs
- SimplePropertyEntry.cs
- ListenDesigner.cs
- NativeObjectSecurity.cs
- TabControl.cs
- HwndHost.cs
- TextWriter.cs
- Label.cs
- IDataContractSurrogate.cs
- BasePattern.cs
- MergePropertyDescriptor.cs
- DbTransaction.cs
- ValidatorCollection.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- ScriptingProfileServiceSection.cs
- MaskDescriptors.cs
- LinearGradientBrush.cs
- TimerElapsedEvenArgs.cs
- CompilerScope.cs
- XamlTypeWithExplicitNamespace.cs
- WebBrowserContainer.cs
- FunctionCommandText.cs
- ConnectionPoolManager.cs
- QEncodedStream.cs
- XmlMemberMapping.cs
- StatusBarItem.cs
- EventRoute.cs
- MarkupExtensionParser.cs
- ToolTipService.cs
- DiagnosticTraceSource.cs
- CompiledQueryCacheKey.cs
- DBSchemaTable.cs
- SymbolType.cs
- ExpressionVisitorHelpers.cs
- OdbcConnection.cs
- _AutoWebProxyScriptWrapper.cs
- AppSettingsReader.cs
- ObjectListFieldCollection.cs
- HMACSHA1.cs
- DPCustomTypeDescriptor.cs
- EnvironmentPermission.cs
- AudioFormatConverter.cs
- Vector.cs
- FixedHyperLink.cs
- ConstraintManager.cs
- ExtentKey.cs
- LassoSelectionBehavior.cs
- TransformGroup.cs
- AsymmetricAlgorithm.cs
- FrameworkTemplate.cs
- ClientBuildManagerCallback.cs
- SchemaImporterExtensionElementCollection.cs
- IdentityReference.cs
- HelpInfo.cs
- FixedSOMLineRanges.cs
- DocumentGrid.cs
- ObjectReferenceStack.cs
- KoreanLunisolarCalendar.cs
- SymbolDocumentInfo.cs