Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Messaging / TwoPhaseCommitProxy.cs / 1 / TwoPhaseCommitProxy.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Define the interfaces and infrastructure needed to send 2PC messages using System; using System.ServiceModel.Channels; using System.ServiceModel; using System.Transactions; using Microsoft.Transactions.Wsat.Protocol; namespace Microsoft.Transactions.Wsat.Messaging { class TwoPhaseCommitCoordinatorProxy : DatagramProxy { public TwoPhaseCommitCoordinatorProxy(CoordinationService coordination, EndpointAddress to, EndpointAddress from) : base(coordination, to, from) { } public IAsyncResult BeginSendPrepared(AsyncCallback callback, object state) { Message message = new PreparedMessage(this.messageVersion, this.protocolVersion); return BeginSendMessage(message, callback, state); } public IAsyncResult BeginSendReadOnly(AsyncCallback callback, object state) { Message message = new ReadOnlyMessage(this.messageVersion, this.protocolVersion); return BeginSendMessage(message, callback, state); } public IAsyncResult BeginSendCommitted(AsyncCallback callback, object state) { Message message = new CommittedMessage(this.messageVersion, this.protocolVersion); return BeginSendMessage(message, callback, state); } public IAsyncResult BeginSendAborted(AsyncCallback callback, object state) { Message message = new AbortedMessage(this.messageVersion, this.protocolVersion); return BeginSendMessage(message, callback, state); } public IAsyncResult BeginSendRecoverMessage(AsyncCallback callback, object state) { Message message = NotificationMessage.CreateRecoverMessage(this.messageVersion, this.protocolVersion); return BeginSendMessage(message, callback, state); } } class TwoPhaseCommitParticipantProxy : DatagramProxy { public TwoPhaseCommitParticipantProxy (CoordinationService coordination, EndpointAddress to, EndpointAddress from) : base(coordination, to, from) { } public IAsyncResult BeginSendPrepare(AsyncCallback callback, object state) { Message message = new PrepareMessage(this.messageVersion, this.protocolVersion); return BeginSendMessage(message, callback, state); } public IAsyncResult BeginSendCommit(AsyncCallback callback, object state) { Message message = new CommitMessage(this.messageVersion, this.protocolVersion); return BeginSendMessage(message, callback, state); } public IAsyncResult BeginSendRollback(AsyncCallback callback, object state) { Message message = new RollbackMessage(this.messageVersion, this.protocolVersion); return BeginSendMessage(message, callback, state); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
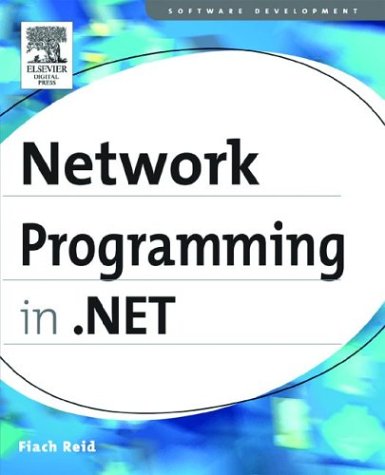
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegexCaptureCollection.cs
- EdmToObjectNamespaceMap.cs
- MissingManifestResourceException.cs
- PropertyOrder.cs
- HandlerWithFactory.cs
- WebPartZoneBase.cs
- SelectionPattern.cs
- BigInt.cs
- ContextItem.cs
- UserPersonalizationStateInfo.cs
- ListBoxChrome.cs
- QilChoice.cs
- FormsIdentity.cs
- CodeCommentStatement.cs
- HorizontalAlignConverter.cs
- HtmlProps.cs
- DbParameterHelper.cs
- AssociationType.cs
- TreeView.cs
- TypeDelegator.cs
- EntityUtil.cs
- XmlArrayItemAttributes.cs
- PrefixQName.cs
- PropertyMetadata.cs
- SqlFunctions.cs
- BindingWorker.cs
- Command.cs
- EntityDataSourceContainerNameItem.cs
- HostingEnvironmentSection.cs
- ByteAnimationUsingKeyFrames.cs
- Brush.cs
- Console.cs
- IdentityReference.cs
- DataBindingExpressionBuilder.cs
- SqlBulkCopyColumnMapping.cs
- GraphicsPathIterator.cs
- PassportAuthenticationEventArgs.cs
- RectangleGeometry.cs
- HttpCachePolicyElement.cs
- LifetimeServices.cs
- SessionIDManager.cs
- WebBrowserPermission.cs
- DbTransaction.cs
- DbConnectionPoolGroup.cs
- NullReferenceException.cs
- UnsafeMethods.cs
- ThicknessConverter.cs
- WebPartMenuStyle.cs
- ChannelTraceRecord.cs
- CommandValueSerializer.cs
- WindowsListViewGroupSubsetLink.cs
- DeviceContexts.cs
- ConsoleCancelEventArgs.cs
- HtmlImage.cs
- PriorityBindingExpression.cs
- OleDbRowUpdatedEvent.cs
- HttpChannelBindingToken.cs
- HttpProcessUtility.cs
- AssemblySettingAttributes.cs
- MobileCapabilities.cs
- BamlRecordWriter.cs
- PositiveTimeSpanValidatorAttribute.cs
- WebPartsSection.cs
- HostedAspNetEnvironment.cs
- ConsoleKeyInfo.cs
- MeasurementDCInfo.cs
- DataPagerFieldCollection.cs
- IndexOutOfRangeException.cs
- FormsAuthenticationEventArgs.cs
- XmlSchema.cs
- TdsParser.cs
- ActivityExecutorOperation.cs
- ProviderUtil.cs
- XPathNodeInfoAtom.cs
- QueryableDataSourceHelper.cs
- Part.cs
- Vector3DCollectionValueSerializer.cs
- HttpCookiesSection.cs
- RegexTree.cs
- PerformanceCounterLib.cs
- TrustLevel.cs
- NativeWindow.cs
- IndexedSelectQueryOperator.cs
- TraceEventCache.cs
- MaskDescriptors.cs
- PageContentAsyncResult.cs
- XPathNavigatorReader.cs
- PerformanceCounterCategory.cs
- SafeRightsManagementHandle.cs
- PageContent.cs
- GPPOINT.cs
- SqlGenerator.cs
- PropertyNames.cs
- ResetableIterator.cs
- RoleManagerEventArgs.cs
- ArgumentDesigner.xaml.cs
- SapiInterop.cs
- Typeface.cs
- LineSegment.cs
- InfoCardAsymmetricCrypto.cs