Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Objects / SqlClient / SqlFunctions.cs / 1305376 / SqlFunctions.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //// This code was generated by a tool. // Generation date and time : 5/1/2009 13:50:44.5127069 // // Changes to this file will be lost if the code is regenerated. // // @owner [....] // @backupOwner [....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Objects; using System.Data.Objects.DataClasses; using System.Linq; using System.Linq.Expressions; using System.Reflection; namespace System.Data.Objects.SqlClient { ////// Contains function stubs that expose SqlServer methods in Linq to Entities. /// public static class SqlFunctions { ////// Proxy for the function SqlServer.CHECKSUM_AGG /// [EdmFunction("SqlServer", "CHECKSUM_AGG")] public static System.Int32? ChecksumAggregate(IEnumerablearg) { ObjectQuery objectQuerySource = arg as ObjectQuery ; if (objectQuerySource != null) { return ((IQueryable)objectQuerySource).Provider.Execute (Expression.Call((MethodInfo)MethodInfo.GetCurrentMethod(),Expression.Constant(arg))); } throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } /// /// Proxy for the function SqlServer.CHECKSUM_AGG /// [EdmFunction("SqlServer", "CHECKSUM_AGG")] public static System.Int32? ChecksumAggregate(IEnumerablearg) { ObjectQuery objectQuerySource = arg as ObjectQuery ; if (objectQuerySource != null) { return ((IQueryable)objectQuerySource).Provider.Execute (Expression.Call((MethodInfo)MethodInfo.GetCurrentMethod(),Expression.Constant(arg))); } throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } /// /// Proxy for the function SqlServer.ASCII /// [EdmFunction("SqlServer", "ASCII")] public static System.Int32? Ascii(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHAR /// [EdmFunction("SqlServer", "CHAR")] public static System.String Char(System.Int32? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int32? CharIndex(System.String toSearch, System.String target) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int32? CharIndex(System.Byte[] toSearch, System.Byte[] target) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int32? CharIndex(System.String toSearch, System.String target, System.Int32? startLocation) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int32? CharIndex(System.Byte[] toSearch, System.Byte[] target, System.Int32? startLocation) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int64? CharIndex(System.String toSearch, System.String target, System.Int64? startLocation) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int64? CharIndex(System.Byte[] toSearch, System.Byte[] target, System.Int64? startLocation) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DIFFERENCE /// [EdmFunction("SqlServer", "DIFFERENCE")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "string")] public static System.Int32? Difference(System.String string1, System.String string2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.NCHAR /// [EdmFunction("SqlServer", "NCHAR")] public static System.String NChar(System.Int32? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.PATINDEX /// [EdmFunction("SqlServer", "PATINDEX")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "string")] public static System.Int32? PatIndex(System.String stringPattern, System.String target) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.QUOTENAME /// [EdmFunction("SqlServer", "QUOTENAME")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "string")] public static System.String QuoteName(System.String stringArg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.QUOTENAME /// [EdmFunction("SqlServer", "QUOTENAME")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "string")] public static System.String QuoteName(System.String stringArg, System.String quoteCharacter) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.REPLICATE /// [EdmFunction("SqlServer", "REPLICATE")] public static System.String Replicate(System.String target, System.Int32? count) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SOUNDEX /// [EdmFunction("SqlServer", "SOUNDEX")] public static System.String SoundCode(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SPACE /// [EdmFunction("SqlServer", "SPACE")] public static System.String Space(System.Int32? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Double? number) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Decimal? number) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Double? number, System.Int32? length) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Decimal? number, System.Int32? length) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Double? number, System.Int32? length, System.Int32? decimalArg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Decimal? number, System.Int32? length, System.Int32? decimalArg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STUFF /// [EdmFunction("SqlServer", "STUFF")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "string")] public static System.String Stuff(System.String stringInput, System.Int32? start, System.Int32? length, System.String stringReplacement) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.UNICODE /// [EdmFunction("SqlServer", "UNICODE")] public static System.Int32? Unicode(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ACOS /// [EdmFunction("SqlServer", "ACOS")] public static System.Double? Acos(System.Double? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ACOS /// [EdmFunction("SqlServer", "ACOS")] public static System.Double? Acos(System.Decimal? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ASIN /// [EdmFunction("SqlServer", "ASIN")] public static System.Double? Asin(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ASIN /// [EdmFunction("SqlServer", "ASIN")] public static System.Double? Asin(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ATAN /// [EdmFunction("SqlServer", "ATAN")] public static System.Double? Atan(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ATAN /// [EdmFunction("SqlServer", "ATAN")] public static System.Double? Atan(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ATN2 /// [EdmFunction("SqlServer", "ATN2")] public static System.Double? Atan2(System.Double? arg1, System.Double? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ATN2 /// [EdmFunction("SqlServer", "ATN2")] public static System.Double? Atan2(System.Decimal? arg1, System.Decimal? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.COS /// [EdmFunction("SqlServer", "COS")] public static System.Double? Cos(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.COS /// [EdmFunction("SqlServer", "COS")] public static System.Double? Cos(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.COT /// [EdmFunction("SqlServer", "COT")] public static System.Double? Cot(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.COT /// [EdmFunction("SqlServer", "COT")] public static System.Double? Cot(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DEGREES /// [EdmFunction("SqlServer", "DEGREES")] public static System.Int32? Degrees(System.Int32? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DEGREES /// [EdmFunction("SqlServer", "DEGREES")] public static System.Int64? Degrees(System.Int64? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DEGREES /// [EdmFunction("SqlServer", "DEGREES")] public static System.Decimal? Degrees(System.Decimal? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DEGREES /// [EdmFunction("SqlServer", "DEGREES")] public static System.Double? Degrees(System.Double? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.EXP /// [EdmFunction("SqlServer", "EXP")] public static System.Double? Exp(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.EXP /// [EdmFunction("SqlServer", "EXP")] public static System.Double? Exp(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.LOG /// [EdmFunction("SqlServer", "LOG")] public static System.Double? Log(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.LOG /// [EdmFunction("SqlServer", "LOG")] public static System.Double? Log(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.LOG10 /// [EdmFunction("SqlServer", "LOG10")] public static System.Double? Log10(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.LOG10 /// [EdmFunction("SqlServer", "LOG10")] public static System.Double? Log10(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.PI /// [EdmFunction("SqlServer", "PI")] public static System.Double? Pi() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RADIANS /// [EdmFunction("SqlServer", "RADIANS")] public static System.Int32? Radians(System.Int32? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RADIANS /// [EdmFunction("SqlServer", "RADIANS")] public static System.Int64? Radians(System.Int64? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RADIANS /// [EdmFunction("SqlServer", "RADIANS")] public static System.Decimal? Radians(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RADIANS /// [EdmFunction("SqlServer", "RADIANS")] public static System.Double? Radians(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RAND /// [EdmFunction("SqlServer", "RAND")] public static System.Double? Rand() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RAND /// [EdmFunction("SqlServer", "RAND")] public static System.Double? Rand(System.Int32? seed) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIGN /// [EdmFunction("SqlServer", "SIGN")] public static System.Int32? Sign(System.Int32? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIGN /// [EdmFunction("SqlServer", "SIGN")] public static System.Int64? Sign(System.Int64? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIGN /// [EdmFunction("SqlServer", "SIGN")] public static System.Decimal? Sign(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIGN /// [EdmFunction("SqlServer", "SIGN")] public static System.Double? Sign(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIN /// [EdmFunction("SqlServer", "SIN")] public static System.Double? Sin(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIN /// [EdmFunction("SqlServer", "SIN")] public static System.Double? Sin(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SQRT /// [EdmFunction("SqlServer", "SQRT")] public static System.Double? SquareRoot(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SQRT /// [EdmFunction("SqlServer", "SQRT")] public static System.Double? SquareRoot(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SQUARE /// [EdmFunction("SqlServer", "SQUARE")] public static System.Double? Square(System.Double? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SQUARE /// [EdmFunction("SqlServer", "SQUARE")] public static System.Double? Square(System.Decimal? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.TAN /// [EdmFunction("SqlServer", "TAN")] public static System.Double? Tan(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.TAN /// [EdmFunction("SqlServer", "TAN")] public static System.Double? Tan(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEADD /// [EdmFunction("SqlServer", "DATEADD")] public static System.DateTime? DateAdd(System.String datePartArg, System.Double? number, System.DateTime? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEADD /// [EdmFunction("SqlServer", "DATEADD")] public static System.TimeSpan? DateAdd(System.String datePartArg, System.Double? number, System.TimeSpan? time) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEADD /// [EdmFunction("SqlServer", "DATEADD")] public static System.DateTimeOffset? DateAdd(System.String datePartArg, System.Double? number, System.DateTimeOffset? dateTimeOffsetArg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEADD /// [EdmFunction("SqlServer", "DATEADD")] public static System.DateTime? DateAdd(System.String datePartArg, System.Double? number, System.String date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTime? startDate, System.DateTime? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTimeOffset? startDate, System.DateTimeOffset? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.TimeSpan? startDate, System.TimeSpan? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.String startDate, System.DateTime? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.String startDate, System.DateTimeOffset? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.String startDate, System.TimeSpan? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.TimeSpan? startDate, System.String endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTime? startDate, System.String endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTimeOffset? startDate, System.String endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.String startDate, System.String endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.TimeSpan? startDate, System.DateTime? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.TimeSpan? startDate, System.DateTimeOffset? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTime? startDate, System.TimeSpan? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTimeOffset? startDate, System.TimeSpan? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTime? startDate, System.DateTimeOffset? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTimeOffset? startDate, System.DateTime? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATENAME /// [EdmFunction("SqlServer", "DATENAME")] public static System.String DateName(System.String datePartArg, System.DateTime? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATENAME /// [EdmFunction("SqlServer", "DATENAME")] public static System.String DateName(System.String datePartArg, System.String date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATENAME /// [EdmFunction("SqlServer", "DATENAME")] public static System.String DateName(System.String datePartArg, System.TimeSpan? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATENAME /// [EdmFunction("SqlServer", "DATENAME")] public static System.String DateName(System.String datePartArg, System.DateTimeOffset? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEPART /// [EdmFunction("SqlServer", "DATEPART")] public static System.Int32? DatePart(System.String datePartArg, System.DateTime? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEPART /// [EdmFunction("SqlServer", "DATEPART")] public static System.Int32? DatePart(System.String datePartArg, System.DateTimeOffset? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEPART /// [EdmFunction("SqlServer", "DATEPART")] public static System.Int32? DatePart(System.String datePartArg, System.String date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEPART /// [EdmFunction("SqlServer", "DATEPART")] public static System.Int32? DatePart(System.String datePartArg, System.TimeSpan? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.GETDATE /// [EdmFunction("SqlServer", "GETDATE")] public static System.DateTime? GetDate() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.GETUTCDATE /// [EdmFunction("SqlServer", "GETUTCDATE")] public static System.DateTime? GetUtcDate() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.Boolean? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.DateTime? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.TimeSpan? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.DateTimeOffset? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.Byte[] arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.Guid? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Boolean? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Double? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Decimal? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.String arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTime? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.TimeSpan? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTimeOffset? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Byte[] arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Guid? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Boolean? arg1, System.Boolean? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Double? arg1, System.Double? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Decimal? arg1, System.Decimal? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.String arg1, System.String arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTime? arg1, System.DateTime? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.TimeSpan? arg1, System.TimeSpan? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTimeOffset? arg1, System.DateTimeOffset? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Byte[] arg1, System.Byte[] arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Guid? arg1, System.Guid? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Boolean? arg1, System.Boolean? arg2, System.Boolean? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Double? arg1, System.Double? arg2, System.Double? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Decimal? arg1, System.Decimal? arg2, System.Decimal? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.String arg1, System.String arg2, System.String arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTime? arg1, System.DateTime? arg2, System.DateTime? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTimeOffset? arg1, System.DateTimeOffset? arg2, System.DateTimeOffset? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.TimeSpan? arg1, System.TimeSpan? arg2, System.TimeSpan? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Byte[] arg1, System.Byte[] arg2, System.Byte[] arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Guid? arg1, System.Guid? arg2, System.Guid? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CURRENT_TIMESTAMP /// [EdmFunction("SqlServer", "CURRENT_TIMESTAMP")] public static System.DateTime? CurrentTimestamp() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CURRENT_USER /// [EdmFunction("SqlServer", "CURRENT_USER")] public static System.String CurrentUser() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.HOST_NAME /// [EdmFunction("SqlServer", "HOST_NAME")] public static System.String HostName() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.USER_NAME /// [EdmFunction("SqlServer", "USER_NAME")] public static System.String UserName(System.Int32? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.USER_NAME /// [EdmFunction("SqlServer", "USER_NAME")] public static System.String UserName() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ISNUMERIC /// [EdmFunction("SqlServer", "ISNUMERIC")] public static System.Int32? IsNumeric(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ISDATE /// [EdmFunction("SqlServer", "ISDATE")] public static System.Int32? IsDate(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //// This code was generated by a tool. // Generation date and time : 5/1/2009 13:50:44.5127069 // // Changes to this file will be lost if the code is regenerated. // // @owner [....] // @backupOwner [....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Objects; using System.Data.Objects.DataClasses; using System.Linq; using System.Linq.Expressions; using System.Reflection; namespace System.Data.Objects.SqlClient { ////// Contains function stubs that expose SqlServer methods in Linq to Entities. /// public static class SqlFunctions { ////// Proxy for the function SqlServer.CHECKSUM_AGG /// [EdmFunction("SqlServer", "CHECKSUM_AGG")] public static System.Int32? ChecksumAggregate(IEnumerablearg) { ObjectQuery objectQuerySource = arg as ObjectQuery ; if (objectQuerySource != null) { return ((IQueryable)objectQuerySource).Provider.Execute (Expression.Call((MethodInfo)MethodInfo.GetCurrentMethod(),Expression.Constant(arg))); } throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } /// /// Proxy for the function SqlServer.CHECKSUM_AGG /// [EdmFunction("SqlServer", "CHECKSUM_AGG")] public static System.Int32? ChecksumAggregate(IEnumerablearg) { ObjectQuery objectQuerySource = arg as ObjectQuery ; if (objectQuerySource != null) { return ((IQueryable)objectQuerySource).Provider.Execute (Expression.Call((MethodInfo)MethodInfo.GetCurrentMethod(),Expression.Constant(arg))); } throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } /// /// Proxy for the function SqlServer.ASCII /// [EdmFunction("SqlServer", "ASCII")] public static System.Int32? Ascii(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHAR /// [EdmFunction("SqlServer", "CHAR")] public static System.String Char(System.Int32? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int32? CharIndex(System.String toSearch, System.String target) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int32? CharIndex(System.Byte[] toSearch, System.Byte[] target) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int32? CharIndex(System.String toSearch, System.String target, System.Int32? startLocation) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int32? CharIndex(System.Byte[] toSearch, System.Byte[] target, System.Int32? startLocation) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int64? CharIndex(System.String toSearch, System.String target, System.Int64? startLocation) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHARINDEX /// [EdmFunction("SqlServer", "CHARINDEX")] public static System.Int64? CharIndex(System.Byte[] toSearch, System.Byte[] target, System.Int64? startLocation) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DIFFERENCE /// [EdmFunction("SqlServer", "DIFFERENCE")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "string")] public static System.Int32? Difference(System.String string1, System.String string2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.NCHAR /// [EdmFunction("SqlServer", "NCHAR")] public static System.String NChar(System.Int32? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.PATINDEX /// [EdmFunction("SqlServer", "PATINDEX")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "string")] public static System.Int32? PatIndex(System.String stringPattern, System.String target) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.QUOTENAME /// [EdmFunction("SqlServer", "QUOTENAME")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "string")] public static System.String QuoteName(System.String stringArg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.QUOTENAME /// [EdmFunction("SqlServer", "QUOTENAME")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "string")] public static System.String QuoteName(System.String stringArg, System.String quoteCharacter) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.REPLICATE /// [EdmFunction("SqlServer", "REPLICATE")] public static System.String Replicate(System.String target, System.Int32? count) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SOUNDEX /// [EdmFunction("SqlServer", "SOUNDEX")] public static System.String SoundCode(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SPACE /// [EdmFunction("SqlServer", "SPACE")] public static System.String Space(System.Int32? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Double? number) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Decimal? number) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Double? number, System.Int32? length) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Decimal? number, System.Int32? length) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Double? number, System.Int32? length, System.Int32? decimalArg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STR /// [EdmFunction("SqlServer", "STR")] public static System.String StringConvert(System.Decimal? number, System.Int32? length, System.Int32? decimalArg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.STUFF /// [EdmFunction("SqlServer", "STUFF")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "string")] public static System.String Stuff(System.String stringInput, System.Int32? start, System.Int32? length, System.String stringReplacement) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.UNICODE /// [EdmFunction("SqlServer", "UNICODE")] public static System.Int32? Unicode(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ACOS /// [EdmFunction("SqlServer", "ACOS")] public static System.Double? Acos(System.Double? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ACOS /// [EdmFunction("SqlServer", "ACOS")] public static System.Double? Acos(System.Decimal? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ASIN /// [EdmFunction("SqlServer", "ASIN")] public static System.Double? Asin(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ASIN /// [EdmFunction("SqlServer", "ASIN")] public static System.Double? Asin(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ATAN /// [EdmFunction("SqlServer", "ATAN")] public static System.Double? Atan(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ATAN /// [EdmFunction("SqlServer", "ATAN")] public static System.Double? Atan(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ATN2 /// [EdmFunction("SqlServer", "ATN2")] public static System.Double? Atan2(System.Double? arg1, System.Double? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ATN2 /// [EdmFunction("SqlServer", "ATN2")] public static System.Double? Atan2(System.Decimal? arg1, System.Decimal? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.COS /// [EdmFunction("SqlServer", "COS")] public static System.Double? Cos(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.COS /// [EdmFunction("SqlServer", "COS")] public static System.Double? Cos(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.COT /// [EdmFunction("SqlServer", "COT")] public static System.Double? Cot(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.COT /// [EdmFunction("SqlServer", "COT")] public static System.Double? Cot(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DEGREES /// [EdmFunction("SqlServer", "DEGREES")] public static System.Int32? Degrees(System.Int32? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DEGREES /// [EdmFunction("SqlServer", "DEGREES")] public static System.Int64? Degrees(System.Int64? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DEGREES /// [EdmFunction("SqlServer", "DEGREES")] public static System.Decimal? Degrees(System.Decimal? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DEGREES /// [EdmFunction("SqlServer", "DEGREES")] public static System.Double? Degrees(System.Double? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.EXP /// [EdmFunction("SqlServer", "EXP")] public static System.Double? Exp(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.EXP /// [EdmFunction("SqlServer", "EXP")] public static System.Double? Exp(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.LOG /// [EdmFunction("SqlServer", "LOG")] public static System.Double? Log(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.LOG /// [EdmFunction("SqlServer", "LOG")] public static System.Double? Log(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.LOG10 /// [EdmFunction("SqlServer", "LOG10")] public static System.Double? Log10(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.LOG10 /// [EdmFunction("SqlServer", "LOG10")] public static System.Double? Log10(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.PI /// [EdmFunction("SqlServer", "PI")] public static System.Double? Pi() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RADIANS /// [EdmFunction("SqlServer", "RADIANS")] public static System.Int32? Radians(System.Int32? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RADIANS /// [EdmFunction("SqlServer", "RADIANS")] public static System.Int64? Radians(System.Int64? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RADIANS /// [EdmFunction("SqlServer", "RADIANS")] public static System.Decimal? Radians(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RADIANS /// [EdmFunction("SqlServer", "RADIANS")] public static System.Double? Radians(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RAND /// [EdmFunction("SqlServer", "RAND")] public static System.Double? Rand() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.RAND /// [EdmFunction("SqlServer", "RAND")] public static System.Double? Rand(System.Int32? seed) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIGN /// [EdmFunction("SqlServer", "SIGN")] public static System.Int32? Sign(System.Int32? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIGN /// [EdmFunction("SqlServer", "SIGN")] public static System.Int64? Sign(System.Int64? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIGN /// [EdmFunction("SqlServer", "SIGN")] public static System.Decimal? Sign(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIGN /// [EdmFunction("SqlServer", "SIGN")] public static System.Double? Sign(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIN /// [EdmFunction("SqlServer", "SIN")] public static System.Double? Sin(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SIN /// [EdmFunction("SqlServer", "SIN")] public static System.Double? Sin(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SQRT /// [EdmFunction("SqlServer", "SQRT")] public static System.Double? SquareRoot(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SQRT /// [EdmFunction("SqlServer", "SQRT")] public static System.Double? SquareRoot(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SQUARE /// [EdmFunction("SqlServer", "SQUARE")] public static System.Double? Square(System.Double? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.SQUARE /// [EdmFunction("SqlServer", "SQUARE")] public static System.Double? Square(System.Decimal? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.TAN /// [EdmFunction("SqlServer", "TAN")] public static System.Double? Tan(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.TAN /// [EdmFunction("SqlServer", "TAN")] public static System.Double? Tan(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEADD /// [EdmFunction("SqlServer", "DATEADD")] public static System.DateTime? DateAdd(System.String datePartArg, System.Double? number, System.DateTime? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEADD /// [EdmFunction("SqlServer", "DATEADD")] public static System.TimeSpan? DateAdd(System.String datePartArg, System.Double? number, System.TimeSpan? time) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEADD /// [EdmFunction("SqlServer", "DATEADD")] public static System.DateTimeOffset? DateAdd(System.String datePartArg, System.Double? number, System.DateTimeOffset? dateTimeOffsetArg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEADD /// [EdmFunction("SqlServer", "DATEADD")] public static System.DateTime? DateAdd(System.String datePartArg, System.Double? number, System.String date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTime? startDate, System.DateTime? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTimeOffset? startDate, System.DateTimeOffset? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.TimeSpan? startDate, System.TimeSpan? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.String startDate, System.DateTime? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.String startDate, System.DateTimeOffset? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.String startDate, System.TimeSpan? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.TimeSpan? startDate, System.String endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTime? startDate, System.String endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTimeOffset? startDate, System.String endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.String startDate, System.String endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.TimeSpan? startDate, System.DateTime? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.TimeSpan? startDate, System.DateTimeOffset? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTime? startDate, System.TimeSpan? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTimeOffset? startDate, System.TimeSpan? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTime? startDate, System.DateTimeOffset? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEDIFF /// [EdmFunction("SqlServer", "DATEDIFF")] public static System.Int32? DateDiff(System.String datePartArg, System.DateTimeOffset? startDate, System.DateTime? endDate) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATENAME /// [EdmFunction("SqlServer", "DATENAME")] public static System.String DateName(System.String datePartArg, System.DateTime? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATENAME /// [EdmFunction("SqlServer", "DATENAME")] public static System.String DateName(System.String datePartArg, System.String date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATENAME /// [EdmFunction("SqlServer", "DATENAME")] public static System.String DateName(System.String datePartArg, System.TimeSpan? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATENAME /// [EdmFunction("SqlServer", "DATENAME")] public static System.String DateName(System.String datePartArg, System.DateTimeOffset? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEPART /// [EdmFunction("SqlServer", "DATEPART")] public static System.Int32? DatePart(System.String datePartArg, System.DateTime? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEPART /// [EdmFunction("SqlServer", "DATEPART")] public static System.Int32? DatePart(System.String datePartArg, System.DateTimeOffset? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEPART /// [EdmFunction("SqlServer", "DATEPART")] public static System.Int32? DatePart(System.String datePartArg, System.String date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATEPART /// [EdmFunction("SqlServer", "DATEPART")] public static System.Int32? DatePart(System.String datePartArg, System.TimeSpan? date) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.GETDATE /// [EdmFunction("SqlServer", "GETDATE")] public static System.DateTime? GetDate() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.GETUTCDATE /// [EdmFunction("SqlServer", "GETUTCDATE")] public static System.DateTime? GetUtcDate() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.Boolean? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.Double? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.Decimal? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.DateTime? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.TimeSpan? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.DateTimeOffset? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.Byte[] arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.DATALENGTH /// [EdmFunction("SqlServer", "DATALENGTH")] public static System.Int32? DataLength(System.Guid? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Boolean? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Double? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Decimal? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.String arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTime? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.TimeSpan? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTimeOffset? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Byte[] arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Guid? arg1) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Boolean? arg1, System.Boolean? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Double? arg1, System.Double? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Decimal? arg1, System.Decimal? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.String arg1, System.String arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTime? arg1, System.DateTime? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.TimeSpan? arg1, System.TimeSpan? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTimeOffset? arg1, System.DateTimeOffset? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Byte[] arg1, System.Byte[] arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Guid? arg1, System.Guid? arg2) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Boolean? arg1, System.Boolean? arg2, System.Boolean? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Double? arg1, System.Double? arg2, System.Double? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Decimal? arg1, System.Decimal? arg2, System.Decimal? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.String arg1, System.String arg2, System.String arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTime? arg1, System.DateTime? arg2, System.DateTime? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.DateTimeOffset? arg1, System.DateTimeOffset? arg2, System.DateTimeOffset? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.TimeSpan? arg1, System.TimeSpan? arg2, System.TimeSpan? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Byte[] arg1, System.Byte[] arg2, System.Byte[] arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CHECKSUM /// [EdmFunction("SqlServer", "CHECKSUM")] public static System.Int32? Checksum(System.Guid? arg1, System.Guid? arg2, System.Guid? arg3) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CURRENT_TIMESTAMP /// [EdmFunction("SqlServer", "CURRENT_TIMESTAMP")] public static System.DateTime? CurrentTimestamp() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.CURRENT_USER /// [EdmFunction("SqlServer", "CURRENT_USER")] public static System.String CurrentUser() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.HOST_NAME /// [EdmFunction("SqlServer", "HOST_NAME")] public static System.String HostName() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.USER_NAME /// [EdmFunction("SqlServer", "USER_NAME")] public static System.String UserName(System.Int32? arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.USER_NAME /// [EdmFunction("SqlServer", "USER_NAME")] public static System.String UserName() { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ISNUMERIC /// [EdmFunction("SqlServer", "ISNUMERIC")] public static System.Int32? IsNumeric(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } ////// Proxy for the function SqlServer.ISDATE /// [EdmFunction("SqlServer", "ISDATE")] public static System.Int32? IsDate(System.String arg) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_EdmFunctionDirectCall); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
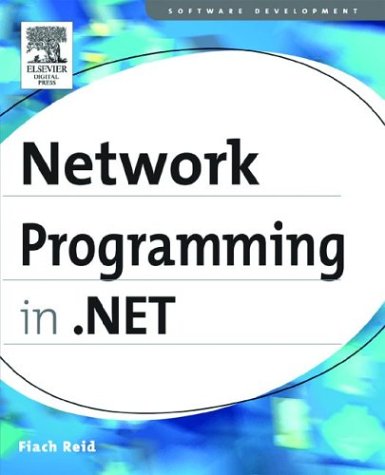
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultMemberAttribute.cs
- XmlSchemaAttribute.cs
- SamlEvidence.cs
- CounterSample.cs
- ProxyDataContractResolver.cs
- SynchronizationHandlesCodeDomSerializer.cs
- OracleConnectionStringBuilder.cs
- _Events.cs
- DirectoryNotFoundException.cs
- SizeConverter.cs
- TabControlEvent.cs
- RepeaterCommandEventArgs.cs
- MediaEntryAttribute.cs
- WebSysDefaultValueAttribute.cs
- FlowNode.cs
- LogArchiveSnapshot.cs
- PartialList.cs
- PackageDigitalSignatureManager.cs
- StaticResourceExtension.cs
- __ConsoleStream.cs
- DataGridPagerStyle.cs
- CodeFieldReferenceExpression.cs
- ResourceReader.cs
- EventManager.cs
- processwaithandle.cs
- ExplicitDiscriminatorMap.cs
- Header.cs
- CodeTypeReferenceCollection.cs
- ClientClassGenerator.cs
- Sequence.cs
- CodeVariableDeclarationStatement.cs
- PenContexts.cs
- FloaterBaseParaClient.cs
- ObjectSecurity.cs
- StoreContentChangedEventArgs.cs
- DivideByZeroException.cs
- XmlDocumentFieldSchema.cs
- TextBoxBase.cs
- dtdvalidator.cs
- COM2FontConverter.cs
- KeyGestureConverter.cs
- CheckBoxBaseAdapter.cs
- PerformanceCounterPermissionEntry.cs
- WebMessageEncoderFactory.cs
- HostingEnvironmentSection.cs
- X509ChainElement.cs
- SafeSystemMetrics.cs
- TransformFinalBlockRequest.cs
- shaperfactoryquerycacheentry.cs
- TcpSocketManager.cs
- AsymmetricKeyExchangeFormatter.cs
- XmlException.cs
- GeneralTransform3DCollection.cs
- FrameworkElement.cs
- _LocalDataStoreMgr.cs
- RoleManagerSection.cs
- InvalidProgramException.cs
- NameSpaceEvent.cs
- WinFormsSpinner.cs
- UnsafeNativeMethods.cs
- ToolZone.cs
- ResourceDisplayNameAttribute.cs
- SqlInternalConnectionTds.cs
- WebPartCloseVerb.cs
- DnsPermission.cs
- DataGridViewCellEventArgs.cs
- EventProviderTraceListener.cs
- DictionaryEditChange.cs
- OleDbConnection.cs
- QueryInterceptorAttribute.cs
- _SslStream.cs
- DbDataAdapter.cs
- SqlAggregateChecker.cs
- HScrollProperties.cs
- SqlRetyper.cs
- FaultFormatter.cs
- IndentedWriter.cs
- WinEventWrap.cs
- FontFamily.cs
- RemotingClientProxy.cs
- SmiMetaData.cs
- HttpBrowserCapabilitiesWrapper.cs
- CodeIterationStatement.cs
- TraceSource.cs
- ModelUIElement3D.cs
- _SafeNetHandles.cs
- UpdateExpressionVisitor.cs
- Page.cs
- TaskFormBase.cs
- RectAnimationClockResource.cs
- MbpInfo.cs
- Symbol.cs
- RequestQueryProcessor.cs
- DataBindEngine.cs
- FixUp.cs
- UnsettableComboBox.cs
- odbcmetadatacolumnnames.cs
- PersonalizationAdministration.cs
- UserMapPath.cs
- ItemMap.cs