Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / IssuanceTokenProviderState.cs / 1 / IssuanceTokenProviderState.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Security { using System.IdentityModel.Claims; using System.IdentityModel.Tokens; using System.ServiceModel; using System.IdentityModel.Policy; using System.Security.Principal; using System.Security.Cryptography.X509Certificates; using System.Collections.Generic; using System.ServiceModel.Channels; using System.ServiceModel.Security.Tokens; using System.Net; using System.Diagnostics; class IssuanceTokenProviderState : IDisposable { bool isNegotiationCompleted = false; GenericXmlSecurityToken serviceToken; string context; EndpointAddress targetAddress; EndpointAddress remoteAddress; public IssuanceTokenProviderState() { } public bool IsNegotiationCompleted { get { return this.isNegotiationCompleted; } } public GenericXmlSecurityToken ServiceToken { get { CheckCompleted(); return this.serviceToken; } } public EndpointAddress TargetAddress { get { return this.targetAddress; } set { this.targetAddress = value; } } public EndpointAddress RemoteAddress { get { return this.remoteAddress; } set { this.remoteAddress = value; } } public string Context { get { return this.context; } set { this.context = value; } } public virtual void Dispose() { } public void SetServiceToken(GenericXmlSecurityToken serviceToken) { if (this.IsNegotiationCompleted) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.NegotiationIsCompleted))); } this.serviceToken = serviceToken; this.isNegotiationCompleted = true; } void CheckCompleted() { if (!this.IsNegotiationCompleted) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.NegotiationIsNotCompleted))); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
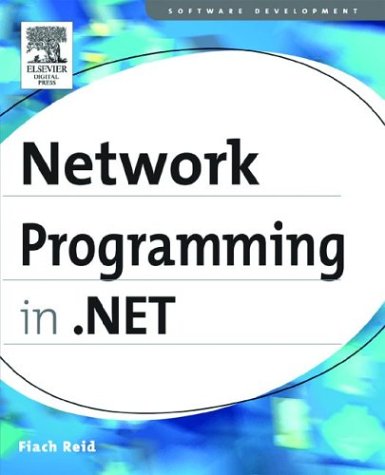
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OSFeature.cs
- ExtensionFile.cs
- CompositeActivityDesigner.cs
- ComponentCollection.cs
- WorkflowItemsPresenter.cs
- TransformPattern.cs
- OleDbParameterCollection.cs
- BuilderPropertyEntry.cs
- ExpressionLexer.cs
- HandleCollector.cs
- GridViewDesigner.cs
- UnionCqlBlock.cs
- TableProviderWrapper.cs
- DataList.cs
- AmbientLight.cs
- BitmapEffectInput.cs
- ImageMap.cs
- Drawing.cs
- HttpGetProtocolReflector.cs
- ApplicationServiceManager.cs
- AjaxFrameworkAssemblyAttribute.cs
- HMACSHA384.cs
- FormatterConverter.cs
- RightNameExpirationInfoPair.cs
- ResourceWriter.cs
- TextAdaptor.cs
- DragCompletedEventArgs.cs
- ProfileService.cs
- HashAlgorithm.cs
- ObjectDataSourceFilteringEventArgs.cs
- SoapIgnoreAttribute.cs
- TreeViewImageKeyConverter.cs
- PointHitTestParameters.cs
- SessionEndedEventArgs.cs
- DesignerActionMethodItem.cs
- RIPEMD160.cs
- PlainXmlDeserializer.cs
- SourceFilter.cs
- Splitter.cs
- XmlSerializerAssemblyAttribute.cs
- ImmutableObjectAttribute.cs
- ScopelessEnumAttribute.cs
- BamlReader.cs
- DrawListViewItemEventArgs.cs
- FragmentQuery.cs
- ProcessHostConfigUtils.cs
- CancellationState.cs
- BaseUriHelper.cs
- NotificationContext.cs
- CodeCompileUnit.cs
- DynamicAttribute.cs
- _Connection.cs
- ExternalCalls.cs
- XPathNode.cs
- Base64Encoder.cs
- IISUnsafeMethods.cs
- BitmapMetadata.cs
- MetaModel.cs
- NameNode.cs
- Ref.cs
- DesignerGenericWebPart.cs
- SqlDataSourceEnumerator.cs
- WebRequestModuleElement.cs
- Source.cs
- JoinElimination.cs
- WindowsFont.cs
- PageThemeBuildProvider.cs
- _SpnDictionary.cs
- TextElementCollection.cs
- WebExceptionStatus.cs
- StandardBindingElement.cs
- NavigationService.cs
- ProfileParameter.cs
- BooleanExpr.cs
- PairComparer.cs
- MouseBinding.cs
- SafeNativeMethods.cs
- Help.cs
- Clock.cs
- PropertyPathConverter.cs
- DBConcurrencyException.cs
- VariantWrapper.cs
- HatchBrush.cs
- WebEventTraceProvider.cs
- OperandQuery.cs
- SystemWebExtensionsSectionGroup.cs
- JobCollate.cs
- PrimitiveDataContract.cs
- InvalidateEvent.cs
- MruCache.cs
- RowUpdatedEventArgs.cs
- WebPartMinimizeVerb.cs
- ButtonBase.cs
- SqlDataSourceView.cs
- CodeTypeParameter.cs
- HttpHandlerAction.cs
- HtmlInputCheckBox.cs
- EntityTemplateUserControl.cs
- Assembly.cs
- ReflectionHelper.cs