Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / QueryRewriting / FragmentQuery.cs / 1 / FragmentQuery.cs
using System; using System.Diagnostics; using System.Collections.Generic; using System.Text; using System.Data.Common.Utils; using System.Data.Common.Utils.Boolean; using System.Data.Mapping.ViewGeneration.Structures; using System.Data.Metadata.Edm; using System.Linq; using System.Globalization; namespace System.Data.Mapping.ViewGeneration.QueryRewriting { //using QueryKnowledgeBase = KnowledgeBase>; internal class FragmentQuery : ITileQuery { private BoolExpression m_fromVariable; // optional private string m_label; // optional private HashSet m_attributes; private BoolExpression m_condition; public HashSet Attributes { get { return m_attributes; } } public BoolExpression Condition { get { return m_condition; } } public static FragmentQuery Create(BoolExpression fromVariable, CellQuery cellQuery) { BoolExpression whereClause = cellQuery.WhereClause; whereClause = whereClause.MakeCopy(); whereClause.ExpensiveSimplify(); return new FragmentQuery(null /*label*/, fromVariable, new HashSet (cellQuery.GetProjectedMembers()), whereClause); } public static FragmentQuery Create(string label, RoleBoolean roleBoolean, CellQuery cellQuery) { BoolExpression whereClause = cellQuery.WhereClause.Create(roleBoolean); whereClause = BoolExpression.CreateAnd(whereClause, cellQuery.WhereClause); //return new FragmentQuery(label, null /* fromVariable */, new HashSet (cellQuery.GetProjectedMembers()), whereClause); // don't need any attributes whereClause = whereClause.MakeCopy(); whereClause.ExpensiveSimplify(); return new FragmentQuery(label, null /* fromVariable */, new HashSet (), whereClause); } public static FragmentQuery Create(IEnumerable attrs, BoolExpression whereClause) { return new FragmentQuery(null /* no name */, null /* no fromVariable*/, attrs, whereClause); } public static FragmentQuery Create(BoolExpression whereClause) { return new FragmentQuery(null /* no name */, null /* no fromVariable*/, new MemberPath[] {}, whereClause); } internal FragmentQuery(string label, BoolExpression fromVariable, IEnumerable attrs, BoolExpression condition) { m_label = label; m_fromVariable = fromVariable; m_condition = condition; m_attributes = new HashSet (attrs); } public BoolExpression FromVariable { get { return m_fromVariable; } } public string Description { get { string label = m_label; if (label == null && m_fromVariable != null) { label = m_fromVariable.ToString(); } return label; } } public override string ToString() { // attributes StringBuilder b = new StringBuilder(); foreach (MemberPath value in this.Attributes) { if (b.Length > 0) { b.Append(','); } b.Append(value.ToString()); } if (Description != null && Description != b.ToString()) { return String.Format(CultureInfo.InvariantCulture, "{0}: [{1} where {2}]", Description, b, this.Condition); } else { return String.Format(CultureInfo.InvariantCulture, "[{0} where {1}]", b, this.Condition); } } #region Static methods // creates a condition member=value internal static BoolExpression CreateMemberCondition(MemberPath path, CellConstant domainValue, MemberDomainMap domainMap, MetadataWorkspace workspace) { if (domainValue is TypeConstant) { return BoolExpression.CreateLiteral(new OneOfTypeConst(CreateSlot(path, workspace), new CellConstantDomain(domainValue, domainMap.GetDomain(path))), domainMap); } else { return BoolExpression.CreateLiteral(new OneOfScalarConst(CreateSlot(path, workspace), new CellConstantDomain(domainValue, domainMap.GetDomain(path))), domainMap); } } // creates a fake join tree slot so we can construct a BoolExpression [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1806:DoNotIgnoreMethodResults", MessageId = "System.Data.Mapping.ViewGeneration.Structures.ExtentJoinTreeNode")] internal static JoinTreeSlot CreateSlot(MemberPath path, MetadataWorkspace workspace) { IList members = path.Members; if (members.Count > 0) { MemberJoinTreeNode leaf = new MemberJoinTreeNode(members[members.Count - 1], false, new MemberJoinTreeNode[] { }, workspace); MemberJoinTreeNode node = leaf; for (int i = members.Count - 2; i >= 0; i--) { node = new MemberJoinTreeNode(members[i], false, new MemberJoinTreeNode[] { node }, workspace); } ExtentJoinTreeNode extentNode = new ExtentJoinTreeNode(path.Extent, new MemberJoinTreeNode[] { node }, workspace); return new JoinTreeSlot(leaf); } else { ExtentJoinTreeNode extentNode = new ExtentJoinTreeNode(path.Extent, new MemberJoinTreeNode[] { }, workspace); return new JoinTreeSlot(extentNode); } } internal static IEqualityComparer GetEqualityComparer(FragmentQueryProcessor qp) { return new FragmentQueryEqualityComparer(qp); } //internal static IComparer GetComparer(IEnumerable attributes) //{ // return new FragmentQueryComparer(attributes); //} #endregion #region Equality Comparer // Two queries are "equal" if they project the same set of attributes // and their WHERE clauses are equivalent private class FragmentQueryEqualityComparer : IEqualityComparer { FragmentQueryProcessor _qp; internal FragmentQueryEqualityComparer(FragmentQueryProcessor qp) { _qp = qp; } #region IEqualityComparer Members public bool Equals(FragmentQuery x, FragmentQuery y) { if (!x.Attributes.SetEquals(y.Attributes)) { return false; } return _qp.IsEquivalentTo(x, y); } // Hashing a bit naive: it exploits syntactic properties, // i.e., some semantically equivalent queries may produce different hash codes // But that's fine for usage scenarios in QueryRewriter.cs public int GetHashCode(FragmentQuery q) { int attrHashCode = 0; foreach (MemberPath member in q.Attributes) { attrHashCode ^= MemberPath.EqualityComparer.GetHashCode(member); } int varHashCode = 0; int constHashCode = 0; foreach (OneOfConst oneOf in q.Condition.OneOfConstVariables) { varHashCode ^= MemberPath.EqualityComparer.GetHashCode(oneOf.Slot.MemberPath); foreach (CellConstant constant in oneOf.Values.Values) { constHashCode ^= CellConstant.EqualityComparer.GetHashCode(constant); } } return attrHashCode * 13 + varHashCode * 7 + constHashCode; } #endregion } #endregion //#region Comparer //// queries with largest attribute overlap go first //private class FragmentQueryComparer : IComparer //{ // private HashSet _attributes; // internal FragmentQueryComparer(IEnumerable attributes) // { // _attributes = new HashSet (attributes); // } // #region IComparer Members // public int Compare(FragmentQuery x, FragmentQuery y) // { // HashSet xAttr = new HashSet (x.Attributes); // HashSet yAttr = new HashSet (y.Attributes); // xAttr.IntersectWith(_attributes); // yAttr.IntersectWith(_attributes); // if (xAttr.Count > yAttr.Count) // { // return -1; // } // else if (xAttr.Count < yAttr.Count) // { // return 1; // } // return 0; // } // #endregion //} //#endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Diagnostics; using System.Collections.Generic; using System.Text; using System.Data.Common.Utils; using System.Data.Common.Utils.Boolean; using System.Data.Mapping.ViewGeneration.Structures; using System.Data.Metadata.Edm; using System.Linq; using System.Globalization; namespace System.Data.Mapping.ViewGeneration.QueryRewriting { //using QueryKnowledgeBase = KnowledgeBase >; internal class FragmentQuery : ITileQuery { private BoolExpression m_fromVariable; // optional private string m_label; // optional private HashSet m_attributes; private BoolExpression m_condition; public HashSet Attributes { get { return m_attributes; } } public BoolExpression Condition { get { return m_condition; } } public static FragmentQuery Create(BoolExpression fromVariable, CellQuery cellQuery) { BoolExpression whereClause = cellQuery.WhereClause; whereClause = whereClause.MakeCopy(); whereClause.ExpensiveSimplify(); return new FragmentQuery(null /*label*/, fromVariable, new HashSet (cellQuery.GetProjectedMembers()), whereClause); } public static FragmentQuery Create(string label, RoleBoolean roleBoolean, CellQuery cellQuery) { BoolExpression whereClause = cellQuery.WhereClause.Create(roleBoolean); whereClause = BoolExpression.CreateAnd(whereClause, cellQuery.WhereClause); //return new FragmentQuery(label, null /* fromVariable */, new HashSet (cellQuery.GetProjectedMembers()), whereClause); // don't need any attributes whereClause = whereClause.MakeCopy(); whereClause.ExpensiveSimplify(); return new FragmentQuery(label, null /* fromVariable */, new HashSet (), whereClause); } public static FragmentQuery Create(IEnumerable attrs, BoolExpression whereClause) { return new FragmentQuery(null /* no name */, null /* no fromVariable*/, attrs, whereClause); } public static FragmentQuery Create(BoolExpression whereClause) { return new FragmentQuery(null /* no name */, null /* no fromVariable*/, new MemberPath[] {}, whereClause); } internal FragmentQuery(string label, BoolExpression fromVariable, IEnumerable attrs, BoolExpression condition) { m_label = label; m_fromVariable = fromVariable; m_condition = condition; m_attributes = new HashSet (attrs); } public BoolExpression FromVariable { get { return m_fromVariable; } } public string Description { get { string label = m_label; if (label == null && m_fromVariable != null) { label = m_fromVariable.ToString(); } return label; } } public override string ToString() { // attributes StringBuilder b = new StringBuilder(); foreach (MemberPath value in this.Attributes) { if (b.Length > 0) { b.Append(','); } b.Append(value.ToString()); } if (Description != null && Description != b.ToString()) { return String.Format(CultureInfo.InvariantCulture, "{0}: [{1} where {2}]", Description, b, this.Condition); } else { return String.Format(CultureInfo.InvariantCulture, "[{0} where {1}]", b, this.Condition); } } #region Static methods // creates a condition member=value internal static BoolExpression CreateMemberCondition(MemberPath path, CellConstant domainValue, MemberDomainMap domainMap, MetadataWorkspace workspace) { if (domainValue is TypeConstant) { return BoolExpression.CreateLiteral(new OneOfTypeConst(CreateSlot(path, workspace), new CellConstantDomain(domainValue, domainMap.GetDomain(path))), domainMap); } else { return BoolExpression.CreateLiteral(new OneOfScalarConst(CreateSlot(path, workspace), new CellConstantDomain(domainValue, domainMap.GetDomain(path))), domainMap); } } // creates a fake join tree slot so we can construct a BoolExpression [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1806:DoNotIgnoreMethodResults", MessageId = "System.Data.Mapping.ViewGeneration.Structures.ExtentJoinTreeNode")] internal static JoinTreeSlot CreateSlot(MemberPath path, MetadataWorkspace workspace) { IList members = path.Members; if (members.Count > 0) { MemberJoinTreeNode leaf = new MemberJoinTreeNode(members[members.Count - 1], false, new MemberJoinTreeNode[] { }, workspace); MemberJoinTreeNode node = leaf; for (int i = members.Count - 2; i >= 0; i--) { node = new MemberJoinTreeNode(members[i], false, new MemberJoinTreeNode[] { node }, workspace); } ExtentJoinTreeNode extentNode = new ExtentJoinTreeNode(path.Extent, new MemberJoinTreeNode[] { node }, workspace); return new JoinTreeSlot(leaf); } else { ExtentJoinTreeNode extentNode = new ExtentJoinTreeNode(path.Extent, new MemberJoinTreeNode[] { }, workspace); return new JoinTreeSlot(extentNode); } } internal static IEqualityComparer GetEqualityComparer(FragmentQueryProcessor qp) { return new FragmentQueryEqualityComparer(qp); } //internal static IComparer GetComparer(IEnumerable attributes) //{ // return new FragmentQueryComparer(attributes); //} #endregion #region Equality Comparer // Two queries are "equal" if they project the same set of attributes // and their WHERE clauses are equivalent private class FragmentQueryEqualityComparer : IEqualityComparer { FragmentQueryProcessor _qp; internal FragmentQueryEqualityComparer(FragmentQueryProcessor qp) { _qp = qp; } #region IEqualityComparer Members public bool Equals(FragmentQuery x, FragmentQuery y) { if (!x.Attributes.SetEquals(y.Attributes)) { return false; } return _qp.IsEquivalentTo(x, y); } // Hashing a bit naive: it exploits syntactic properties, // i.e., some semantically equivalent queries may produce different hash codes // But that's fine for usage scenarios in QueryRewriter.cs public int GetHashCode(FragmentQuery q) { int attrHashCode = 0; foreach (MemberPath member in q.Attributes) { attrHashCode ^= MemberPath.EqualityComparer.GetHashCode(member); } int varHashCode = 0; int constHashCode = 0; foreach (OneOfConst oneOf in q.Condition.OneOfConstVariables) { varHashCode ^= MemberPath.EqualityComparer.GetHashCode(oneOf.Slot.MemberPath); foreach (CellConstant constant in oneOf.Values.Values) { constHashCode ^= CellConstant.EqualityComparer.GetHashCode(constant); } } return attrHashCode * 13 + varHashCode * 7 + constHashCode; } #endregion } #endregion //#region Comparer //// queries with largest attribute overlap go first //private class FragmentQueryComparer : IComparer //{ // private HashSet _attributes; // internal FragmentQueryComparer(IEnumerable attributes) // { // _attributes = new HashSet (attributes); // } // #region IComparer Members // public int Compare(FragmentQuery x, FragmentQuery y) // { // HashSet xAttr = new HashSet (x.Attributes); // HashSet yAttr = new HashSet (y.Attributes); // xAttr.IntersectWith(_attributes); // yAttr.IntersectWith(_attributes); // if (xAttr.Count > yAttr.Count) // { // return -1; // } // else if (xAttr.Count < yAttr.Count) // { // return 1; // } // return 0; // } // #endregion //} //#endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
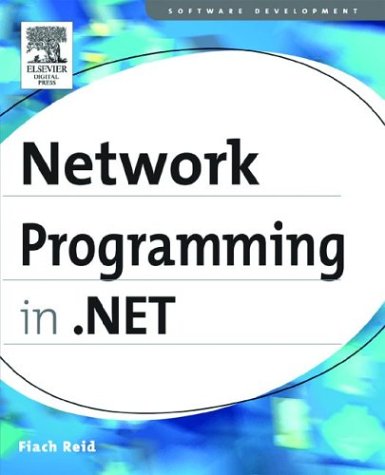
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Binding.cs
- SiteMapNode.cs
- TemplateParser.cs
- EntityModelBuildProvider.cs
- TiffBitmapEncoder.cs
- RuntimeEnvironment.cs
- LineGeometry.cs
- BamlReader.cs
- MenuAutomationPeer.cs
- MetadataSerializer.cs
- DispatcherProcessingDisabled.cs
- DrawingAttributes.cs
- ClickablePoint.cs
- DynamicValueConverter.cs
- OrderedDictionaryStateHelper.cs
- VisualCollection.cs
- RuleSettings.cs
- PackWebResponse.cs
- cookiecontainer.cs
- WebPartConnectionsCancelVerb.cs
- ToolStripItemBehavior.cs
- SqlDataSourceCommandEventArgs.cs
- PowerEase.cs
- FlowDocumentFormatter.cs
- Message.cs
- SBCSCodePageEncoding.cs
- WmlPanelAdapter.cs
- FlowLayoutPanelDesigner.cs
- TabControlCancelEvent.cs
- ChangePasswordAutoFormat.cs
- _ShellExpression.cs
- MemberCollection.cs
- XmlCharCheckingWriter.cs
- Debug.cs
- StateWorkerRequest.cs
- OdbcConnectionString.cs
- SoundPlayer.cs
- HashUtility.cs
- WebBrowser.cs
- XmlSchemaExternal.cs
- EntityContainerAssociationSet.cs
- RawStylusActions.cs
- GridViewSortEventArgs.cs
- DefaultHttpHandler.cs
- TaiwanCalendar.cs
- SqlNodeAnnotation.cs
- FormViewInsertEventArgs.cs
- PasswordTextContainer.cs
- HandlerMappingMemo.cs
- EmptyEnumerable.cs
- DbDataSourceEnumerator.cs
- HyperLinkStyle.cs
- Preprocessor.cs
- RequestQueue.cs
- ValueProviderWrapper.cs
- EventManager.cs
- Nodes.cs
- WhiteSpaceTrimStringConverter.cs
- Button.cs
- SqlParameterCollection.cs
- Classification.cs
- FileNotFoundException.cs
- PipelineComponent.cs
- XmlSerializerVersionAttribute.cs
- Hyperlink.cs
- OperationAbortedException.cs
- StateDesigner.Layouts.cs
- XmlDownloadManager.cs
- PropertiesTab.cs
- RenderingEventArgs.cs
- InvalidWMPVersionException.cs
- XmlSchemaSimpleTypeRestriction.cs
- EntitySetBaseCollection.cs
- DeviceOverridableAttribute.cs
- PolyQuadraticBezierSegment.cs
- DiagnosticsConfiguration.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- WebContext.cs
- _Events.cs
- SizeKeyFrameCollection.cs
- IgnoreFileBuildProvider.cs
- MenuItemAutomationPeer.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- OutputCacheSettings.cs
- ThicknessAnimationUsingKeyFrames.cs
- SequentialOutput.cs
- StructuralType.cs
- Filter.cs
- XmlSchemaType.cs
- ObjectRef.cs
- SchemaElement.cs
- TypeElementCollection.cs
- SystemResourceHost.cs
- ErrorLog.cs
- GeneralTransform2DTo3D.cs
- Page.cs
- CheckoutException.cs
- FileDetails.cs
- ChannelServices.cs
- EntityDataSourceDesigner.cs