Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / FlowThrottle.cs / 1 / FlowThrottle.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.Diagnostics; using System.ServiceModel.Channels; using System.ServiceModel.Diagnostics; using System.Collections.Generic; using System.Threading; sealed class FlowThrottle { int capacity; int count; object mutex; WaitCallback release; Queue
Link Menu
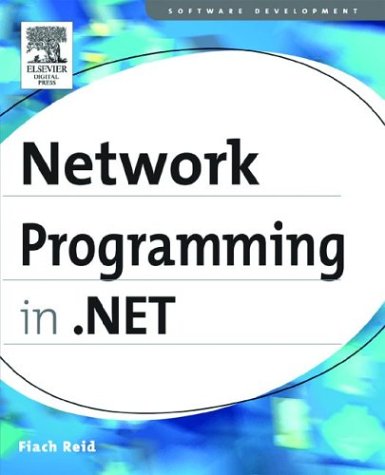
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AtomServiceDocumentSerializer.cs
- ToolTipAutomationPeer.cs
- MouseGestureConverter.cs
- GradientBrush.cs
- ScrollBarRenderer.cs
- Registry.cs
- RtfNavigator.cs
- CharacterMetricsDictionary.cs
- NestedContainer.cs
- DataGridViewCellStateChangedEventArgs.cs
- GrabHandleGlyph.cs
- FocusTracker.cs
- ReadOnlyDictionary.cs
- ConfigXmlReader.cs
- FeatureAttribute.cs
- QuotaExceededException.cs
- DbDataSourceEnumerator.cs
- Peer.cs
- IsolatedStorageFilePermission.cs
- COM2ExtendedBrowsingHandler.cs
- wgx_render.cs
- CodeTryCatchFinallyStatement.cs
- XmlWhitespace.cs
- KeyPressEvent.cs
- XmlReaderDelegator.cs
- XpsTokenContext.cs
- SymLanguageType.cs
- FixedPageAutomationPeer.cs
- PersonalizationProviderCollection.cs
- InvalidDataContractException.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- ProjectedSlot.cs
- CompositeDispatchFormatter.cs
- HotCommands.cs
- MultipartContentParser.cs
- DesignerActionGlyph.cs
- GeneralTransform2DTo3DTo2D.cs
- Quaternion.cs
- PrtCap_Base.cs
- MenuCommands.cs
- LogStream.cs
- GACIdentityPermission.cs
- SafeLocalMemHandle.cs
- NetworkAddressChange.cs
- XmlNamespaceMapping.cs
- FrameworkEventSource.cs
- CodeDomConfigurationHandler.cs
- HelpKeywordAttribute.cs
- CheckBoxBaseAdapter.cs
- MonitoringDescriptionAttribute.cs
- CommandDevice.cs
- DbDataAdapter.cs
- LifetimeServices.cs
- SafeBitVector32.cs
- ToolboxService.cs
- EntityDataSourceWizardForm.cs
- JulianCalendar.cs
- KerberosTicketHashIdentifierClause.cs
- MetafileHeader.cs
- GeometryHitTestParameters.cs
- WebServicesDescriptionAttribute.cs
- SerializableAttribute.cs
- FlowNode.cs
- ScrollBarRenderer.cs
- EdmComplexTypeAttribute.cs
- QueryContinueDragEventArgs.cs
- Typeface.cs
- PKCS1MaskGenerationMethod.cs
- _IPv4Address.cs
- PipelineDeploymentState.cs
- TableLayoutColumnStyleCollection.cs
- PtsCache.cs
- TimeStampChecker.cs
- RuntimeIdentifierPropertyAttribute.cs
- ClassDataContract.cs
- TimeStampChecker.cs
- WebScriptMetadataInstanceContextProvider.cs
- NamedPipeChannelListener.cs
- Message.cs
- HttpModuleCollection.cs
- List.cs
- JournalEntry.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- SimpleHandlerBuildProvider.cs
- OfTypeExpression.cs
- LoadRetryAsyncResult.cs
- DES.cs
- ColorBlend.cs
- CuspData.cs
- ParentQuery.cs
- ServerTooBusyException.cs
- TextEditorSpelling.cs
- AdRotatorDesigner.cs
- MetadataImporter.cs
- TogglePatternIdentifiers.cs
- ResourceReferenceKeyNotFoundException.cs
- IssuedTokenServiceElement.cs
- LogManagementAsyncResult.cs
- ViewStateModeByIdAttribute.cs
- CompositeFontFamily.cs