Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Configuration / HttpTransportElement.cs / 1 / HttpTransportElement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Configuration { using System.Configuration; using System.ServiceModel; using System.Globalization; using System.Net; using System.Net.Security; using System.Security.Principal; using System.ServiceModel.Channels; public partial class HttpTransportElement : TransportElement { public HttpTransportElement() { } [ConfigurationProperty(ConfigurationStrings.AllowCookies, DefaultValue = HttpTransportDefaults.AllowCookies)] public bool AllowCookies { get { return (bool)base[ConfigurationStrings.AllowCookies]; } set { base[ConfigurationStrings.AllowCookies] = value; } } [ConfigurationProperty(ConfigurationStrings.AuthenticationScheme, DefaultValue = HttpTransportDefaults.AuthenticationScheme)] [StandardRuntimeEnumValidator(typeof(AuthenticationSchemes))] public AuthenticationSchemes AuthenticationScheme { get {return (AuthenticationSchemes) base[ConfigurationStrings.AuthenticationScheme]; } set {base[ConfigurationStrings.AuthenticationScheme] = value; } } public override Type BindingElementType { get {return typeof(HttpTransportBindingElement); } } [ConfigurationProperty(ConfigurationStrings.BypassProxyOnLocal, DefaultValue = HttpTransportDefaults.BypassProxyOnLocal)] public bool BypassProxyOnLocal { get {return (bool) base[ConfigurationStrings.BypassProxyOnLocal]; } set {base[ConfigurationStrings.BypassProxyOnLocal] = value; } } [ConfigurationProperty(ConfigurationStrings.HostNameComparisonMode, DefaultValue = HttpTransportDefaults.HostNameComparisonMode)] [ServiceModelEnumValidator(typeof(HostNameComparisonModeHelper))] public HostNameComparisonMode HostNameComparisonMode { get { return (HostNameComparisonMode)base[ConfigurationStrings.HostNameComparisonMode]; } set { base[ConfigurationStrings.HostNameComparisonMode] = value; } } [ConfigurationProperty(ConfigurationStrings.KeepAliveEnabled, DefaultValue = HttpTransportDefaults.KeepAliveEnabled)] public bool KeepAliveEnabled { get { return (bool)base[ConfigurationStrings.KeepAliveEnabled]; } set { base[ConfigurationStrings.KeepAliveEnabled] = value; } } [ConfigurationProperty(ConfigurationStrings.MaxBufferSize, DefaultValue = TransportDefaults.MaxBufferSize)] [IntegerValidator(MinValue = 1)] public int MaxBufferSize { get { return (int)base[ConfigurationStrings.MaxBufferSize]; } set { base[ConfigurationStrings.MaxBufferSize] = value; } } [ConfigurationProperty(ConfigurationStrings.ProxyAddress, DefaultValue = HttpTransportDefaults.ProxyAddress)] public Uri ProxyAddress { get {return (Uri) base[ConfigurationStrings.ProxyAddress]; } set {base[ConfigurationStrings.ProxyAddress] = value; } } [ConfigurationProperty(ConfigurationStrings.ProxyAuthenticationScheme, DefaultValue = HttpTransportDefaults.ProxyAuthenticationScheme)] [StandardRuntimeEnumValidator(typeof(AuthenticationSchemes))] public AuthenticationSchemes ProxyAuthenticationScheme { get { return (AuthenticationSchemes)base[ConfigurationStrings.ProxyAuthenticationScheme]; } set { base[ConfigurationStrings.ProxyAuthenticationScheme] = value; } } [ConfigurationProperty(ConfigurationStrings.Realm, DefaultValue = HttpTransportDefaults.Realm)] [StringValidator(MinLength = 0)] public string Realm { get {return (string) base[ConfigurationStrings.Realm]; } set { if (String.IsNullOrEmpty(value)) { value = String.Empty; } base[ConfigurationStrings.Realm] = value; } } [ConfigurationProperty(ConfigurationStrings.TransferMode, DefaultValue = HttpTransportDefaults.TransferMode)] [ServiceModelEnumValidator(typeof(TransferModeHelper))] public TransferMode TransferMode { get { return (TransferMode)base[ConfigurationStrings.TransferMode]; } set { base[ConfigurationStrings.TransferMode] = value; } } [ConfigurationProperty(ConfigurationStrings.UnsafeConnectionNtlmAuthentication, DefaultValue = HttpTransportDefaults.UnsafeConnectionNtlmAuthentication)] public bool UnsafeConnectionNtlmAuthentication { get { return (bool)base[ConfigurationStrings.UnsafeConnectionNtlmAuthentication]; } set { base[ConfigurationStrings.UnsafeConnectionNtlmAuthentication] = value; } } [ConfigurationProperty(ConfigurationStrings.UseDefaultWebProxy, DefaultValue = HttpTransportDefaults.UseDefaultWebProxy)] public bool UseDefaultWebProxy { get { return (bool)base[ConfigurationStrings.UseDefaultWebProxy]; } set { base[ConfigurationStrings.UseDefaultWebProxy] = value; } } public override void ApplyConfiguration(BindingElement bindingElement) { base.ApplyConfiguration(bindingElement); HttpTransportBindingElement binding = (HttpTransportBindingElement)bindingElement; binding.AllowCookies = this.AllowCookies; binding.AuthenticationScheme = this.AuthenticationScheme; binding.BypassProxyOnLocal = this.BypassProxyOnLocal; binding.KeepAliveEnabled = this.KeepAliveEnabled; binding.HostNameComparisonMode = this.HostNameComparisonMode; PropertyInformationCollection propertyInfo = this.ElementInformation.Properties; if (propertyInfo[ConfigurationStrings.MaxBufferSize].ValueOrigin != PropertyValueOrigin.Default) { binding.MaxBufferSize = this.MaxBufferSize; } binding.ProxyAddress = this.ProxyAddress; binding.ProxyAuthenticationScheme = this.ProxyAuthenticationScheme; binding.Realm = this.Realm; binding.TransferMode = this.TransferMode; binding.UnsafeConnectionNtlmAuthentication = this.UnsafeConnectionNtlmAuthentication; binding.UseDefaultWebProxy = this.UseDefaultWebProxy; } public override void CopyFrom(ServiceModelExtensionElement from) { base.CopyFrom(from); HttpTransportElement source = (HttpTransportElement) from; #pragma warning suppress 56506 // [....], base.CopyFrom() validates the argument this.AllowCookies = source.AllowCookies; this.AuthenticationScheme = source.AuthenticationScheme; this.BypassProxyOnLocal = source.BypassProxyOnLocal; this.KeepAliveEnabled = source.KeepAliveEnabled; this.HostNameComparisonMode = source.HostNameComparisonMode; this.MaxBufferSize = source.MaxBufferSize; this.ProxyAddress = source.ProxyAddress; this.ProxyAuthenticationScheme = source.ProxyAuthenticationScheme; this.Realm = source.Realm; this.TransferMode = source.TransferMode; this.UnsafeConnectionNtlmAuthentication = source.UnsafeConnectionNtlmAuthentication; this.UseDefaultWebProxy = source.UseDefaultWebProxy; } protected override TransportBindingElement CreateDefaultBindingElement() { return new HttpTransportBindingElement(); } protected internal override void InitializeFrom(BindingElement bindingElement) { base.InitializeFrom(bindingElement); HttpTransportBindingElement source = (HttpTransportBindingElement)bindingElement; this.AllowCookies = source.AllowCookies; this.AuthenticationScheme = source.AuthenticationScheme; this.BypassProxyOnLocal = source.BypassProxyOnLocal; this.KeepAliveEnabled = source.KeepAliveEnabled; this.HostNameComparisonMode = source.HostNameComparisonMode; this.MaxBufferSize = source.MaxBufferSize; this.ProxyAddress = source.ProxyAddress; this.ProxyAuthenticationScheme = source.ProxyAuthenticationScheme; this.Realm = source.Realm; this.TransferMode = source.TransferMode; this.UnsafeConnectionNtlmAuthentication = source.UnsafeConnectionNtlmAuthentication; this.UseDefaultWebProxy = source.UseDefaultWebProxy; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
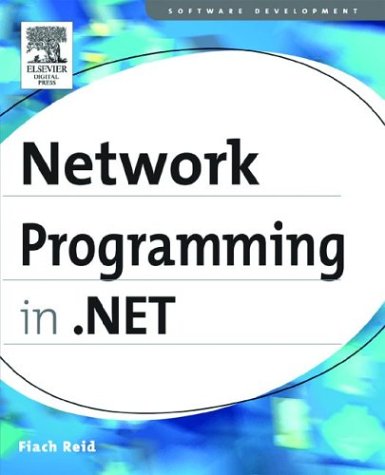
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemberDescriptor.cs
- HMAC.cs
- EntityDataSourceQueryBuilder.cs
- HttpCookieCollection.cs
- ControlIdConverter.cs
- PeerCredential.cs
- SqlFunctionAttribute.cs
- RuntimeHelpers.cs
- NameValueCollection.cs
- KeyFrames.cs
- X509ImageLogo.cs
- TagPrefixAttribute.cs
- Pointer.cs
- SqlBulkCopy.cs
- TableRow.cs
- DBPropSet.cs
- SqlIdentifier.cs
- Button.cs
- Gdiplus.cs
- sqlnorm.cs
- CodeTypeMemberCollection.cs
- OrderedEnumerableRowCollection.cs
- AssemblyHelper.cs
- HttpWebResponse.cs
- _SafeNetHandles.cs
- PageParserFilter.cs
- EventLogEntry.cs
- Console.cs
- URLIdentityPermission.cs
- HtmlControlPersistable.cs
- WindowShowOrOpenTracker.cs
- ExpressionVisitorHelpers.cs
- FlowDocumentScrollViewer.cs
- LinqToSqlWrapper.cs
- IDQuery.cs
- OdbcParameter.cs
- Odbc32.cs
- DataViewManager.cs
- TextDecoration.cs
- ChtmlFormAdapter.cs
- WindowsSolidBrush.cs
- WebPartExportVerb.cs
- ThreadStateException.cs
- HotSpotCollection.cs
- RSAPKCS1SignatureFormatter.cs
- Point3DIndependentAnimationStorage.cs
- Metadata.cs
- EasingKeyFrames.cs
- SystemResourceKey.cs
- UnauthorizedAccessException.cs
- ContractCodeDomInfo.cs
- WebPartConnectionsCloseVerb.cs
- StyleTypedPropertyAttribute.cs
- GraphicsPathIterator.cs
- TextDecorationLocationValidation.cs
- TickBar.cs
- TimerEventSubscriptionCollection.cs
- PlainXmlSerializer.cs
- EncryptedPackageFilter.cs
- WebPartEditorCancelVerb.cs
- RequestResizeEvent.cs
- Select.cs
- DbCommandDefinition.cs
- SimplePropertyEntry.cs
- Int64Converter.cs
- Geometry3D.cs
- MTConfigUtil.cs
- Regex.cs
- SqlCommand.cs
- CommandBinding.cs
- InitializerFacet.cs
- DataGridViewDataErrorEventArgs.cs
- PerformanceCounterLib.cs
- LinkedResource.cs
- TransformGroup.cs
- RadioButtonBaseAdapter.cs
- SchemaNames.cs
- Transform3DGroup.cs
- TextProperties.cs
- EventWaitHandleSecurity.cs
- MatrixIndependentAnimationStorage.cs
- ToolStripContextMenu.cs
- XmlText.cs
- ConfigXmlDocument.cs
- CodeThrowExceptionStatement.cs
- Error.cs
- GridViewActionList.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- _SslState.cs
- Menu.cs
- Helper.cs
- GrammarBuilderRuleRef.cs
- InputEventArgs.cs
- RawUIStateInputReport.cs
- TextBlockAutomationPeer.cs
- InputChannel.cs
- CanonicalFontFamilyReference.cs
- EditorPartDesigner.cs
- NavigationWindowAutomationPeer.cs
- InProcStateClientManager.cs