Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / TransportBindingElementImporter.cs / 1 / TransportBindingElementImporter.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Xml; using System.ServiceModel; using System.ServiceModel.Description; using System.ServiceModel.Security; using System.Xml.Schema; using System.Collections.ObjectModel; using System.Collections.Generic; using WsdlNS=System.Web.Services.Description; // implemented by Indigo Transports interface ITransportPolicyImport { void ImportPolicy(MetadataImporter importer, PolicyConversionContext policyContext); } public class TransportBindingElementImporter : IWsdlImportExtension, IPolicyImportExtension { void IWsdlImportExtension.BeforeImport(WsdlNS.ServiceDescriptionCollection wsdlDocuments, XmlSchemaSet xmlSchemas, ICollectionpolicy) { WsdlImporter.SoapInPolicyWorkaroundHelper.InsertAdHocTransportPolicy(wsdlDocuments); } void IWsdlImportExtension.ImportContract(WsdlImporter importer, WsdlContractConversionContext context) { } void IWsdlImportExtension.ImportEndpoint(WsdlImporter importer, WsdlEndpointConversionContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } #pragma warning suppress 56506 // [....], these properties cannot be null in this context if (context.Endpoint.Binding == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context.Endpoint.Binding"); } #pragma warning suppress 56506 // [....], CustomBinding.Elements never be null TransportBindingElement transportBindingElement = GetBindingElements(context).Find (); bool transportHandledExternaly = (transportBindingElement != null) && !StateHelper.IsRegisteredTransportBindingElement(importer, context); if (transportHandledExternaly) return; #pragma warning suppress 56506 // [....], these properties cannot be null in this context WsdlNS.SoapBinding soapBinding = (WsdlNS.SoapBinding)context.WsdlBinding.Extensions.Find(typeof(WsdlNS.SoapBinding)); if (soapBinding != null && transportBindingElement == null) { CreateLegacyTransportBindingElement(importer, soapBinding, context); } // Try to import WS-Addressing address from the port if (context.WsdlPort != null) { ImportAddress(context, transportBindingElement); } } static BindingElementCollection GetBindingElements(WsdlEndpointConversionContext context) { Binding binding = context.Endpoint.Binding; BindingElementCollection elements = binding is CustomBinding ? ((CustomBinding)binding).Elements : binding.CreateBindingElements(); return elements; } static CustomBinding ConvertToCustomBinding(WsdlEndpointConversionContext context) { CustomBinding customBinding = context.Endpoint.Binding as CustomBinding; if (customBinding == null) { customBinding = new CustomBinding(context.Endpoint.Binding); context.Endpoint.Binding = customBinding; } return customBinding; } static void ImportAddress(WsdlEndpointConversionContext context, TransportBindingElement transportBindingElement) { EndpointAddress address = context.Endpoint.Address = WsdlImporter.WSAddressingHelper.ImportAddress(context.WsdlPort); if (address != null) { context.Endpoint.Address = address; if (address.Uri.Scheme == Uri.UriSchemeHttps && !(transportBindingElement is HttpsTransportBindingElement)) { BindingElementCollection elements = ConvertToCustomBinding(context).Elements; // replace the http BE with an https one. elements.Remove(transportBindingElement); elements.Add(CreateHttpsFromHttp(transportBindingElement as HttpTransportBindingElement)); } } } static void CreateLegacyTransportBindingElement(WsdlImporter importer, WsdlNS.SoapBinding soapBinding, WsdlEndpointConversionContext context) { // We create a transportBindingElement based on the SoapBinding's Transport TransportBindingElement transportBindingElement = CreateTransportBindingElements(soapBinding.Transport, null); if (transportBindingElement != null) { ConvertToCustomBinding(context).Elements.Add(transportBindingElement); StateHelper.RegisterTransportBindingElement(importer, context); } } static HttpsTransportBindingElement CreateHttpsFromHttp(HttpTransportBindingElement http) { if (http == null) return new HttpsTransportBindingElement(); HttpsTransportBindingElement https = HttpsTransportBindingElement.CreateFromHttpBindingElement(http); return https; } void IPolicyImportExtension.ImportPolicy(MetadataImporter importer, PolicyConversionContext policyContext) { XmlQualifiedName wsdlBindingQName; string transportUri = WsdlImporter.SoapInPolicyWorkaroundHelper.FindAdHocTransportPolicy(policyContext, out wsdlBindingQName); if (transportUri != null && !policyContext.BindingElements.Contains(typeof(TransportBindingElement))) { TransportBindingElement transportBindingElement = CreateTransportBindingElements(transportUri, policyContext); if (transportBindingElement != null) { ITransportPolicyImport transportPolicyImport = transportBindingElement as ITransportPolicyImport; if (transportPolicyImport != null) transportPolicyImport.ImportPolicy(importer, policyContext); policyContext.BindingElements.Add(transportBindingElement); StateHelper.RegisterTransportBindingElement(importer, wsdlBindingQName); } } } static TransportBindingElement CreateTransportBindingElements(string transportUri, PolicyConversionContext policyContext) { TransportBindingElement transportBindingElement = null; // Try and Create TransportBindingElement switch (transportUri) { case TransportPolicyConstants.HttpTransportUri: if (policyContext != null) { WSSecurityPolicy sp = null; ICollection policyCollection = policyContext.GetBindingAssertions(); if (WSSecurityPolicy.TryGetSecurityPolicyDriver(policyCollection, out sp) && sp.ContainsWsspHttpsTokenAssertion(policyCollection)) { HttpsTransportBindingElement httpsBinding = new HttpsTransportBindingElement(); httpsBinding.MessageSecurityVersion = sp.GetSupportedMessageSecurityVersion(SecurityVersion.WSSecurity11); transportBindingElement = httpsBinding; } } if (transportBindingElement == null) { transportBindingElement = new HttpTransportBindingElement(); } break; case TransportPolicyConstants.TcpTransportUri: transportBindingElement = new TcpTransportBindingElement(); break; case TransportPolicyConstants.NamedPipeTransportUri: transportBindingElement = new NamedPipeTransportBindingElement(); break; case TransportPolicyConstants.MsmqTransportUri: transportBindingElement = new MsmqTransportBindingElement(); break; case TransportPolicyConstants.PeerTransportUri: transportBindingElement = new PeerTransportBindingElement(); break; default: // There may be another registered converter that can handle this transport. break; } return transportBindingElement; } static class StateHelper { readonly static object StateBagKey = new object(); static Dictionary GetGeneratedTransportBindingElements(MetadataImporter importer) { object retValue; if (!importer.State.TryGetValue(StateHelper.StateBagKey, out retValue)) { retValue = new Dictionary (); importer.State.Add(StateHelper.StateBagKey, retValue); } return (Dictionary )retValue; } internal static void RegisterTransportBindingElement(MetadataImporter importer, XmlQualifiedName wsdlBindingQName) { GetGeneratedTransportBindingElements(importer)[wsdlBindingQName] = wsdlBindingQName; } internal static void RegisterTransportBindingElement(MetadataImporter importer, WsdlEndpointConversionContext context) { XmlQualifiedName wsdlBindingQName = new XmlQualifiedName(context.WsdlBinding.Name, context.WsdlBinding.ServiceDescription.TargetNamespace); GetGeneratedTransportBindingElements(importer)[wsdlBindingQName] = wsdlBindingQName; } internal static bool IsRegisteredTransportBindingElement(WsdlImporter importer, WsdlEndpointConversionContext context) { XmlQualifiedName key = new XmlQualifiedName(context.WsdlBinding.Name, context.WsdlBinding.ServiceDescription.TargetNamespace); return GetGeneratedTransportBindingElements(importer).ContainsKey(key); } } } static class TransportPolicyConstants { public const string BasicHttpAuthenticationName = "BasicAuthentication"; public const string CompositeDuplex = "CompositeDuplex"; public const string CompositeDuplexNamespace = "http://schemas.microsoft.com/net/2006/06/duplex"; public const string CompositeDuplexPrefix = "cdp"; public const string DigestHttpAuthenticationName = "DigestAuthentication"; public const string DotNetFramingNamespace = FramingEncodingString.NamespaceUri + "/policy"; public const string DotNetFramingPrefix = "msf"; public const string HttpAuthNamespace = "http://schemas.microsoft.com/ws/06/2004/policy/http"; public const string HttpAuthPrefix = "http"; public const string HttpTransportUri = "http://schemas.xmlsoap.org/soap/http"; public const string MsmqBestEffort = "MsmqBestEffort"; public const string MsmqSession = "MsmqSession"; public const string MsmqTransportNamespace = "http://schemas.microsoft.com/ws/06/2004/mspolicy/msmq"; public const string MsmqTransportPrefix = "msmq"; public const string MsmqTransportUri = "http://schemas.microsoft.com/soap/msmq"; public const string MsmqVolatile = "MsmqVolatile"; public const string MsmqAuthenticated = "Authenticated"; public const string MsmqWindowsDomain = "WindowsDomain"; public const string NamedPipeTransportUri = "http://schemas.microsoft.com/soap/named-pipe"; public const string NegotiateHttpAuthenticationName = "NegotiateAuthentication"; public const string NtlmHttpAuthenticationName = "NtlmAuthentication"; public const string PeerTransportUri = "http://schemas.microsoft.com/soap/peer"; public const string ProtectionLevelName = "ProtectionLevel"; public const string RequireClientCertificateName = "RequireClientCertificate"; public const string SslTransportSecurityName = "SslTransportSecurity"; public const string StreamedName = "Streamed"; public const string TcpTransportUri = "http://schemas.microsoft.com/soap/tcp"; public const string WindowsTransportSecurityName = "WindowsTransportSecurity"; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
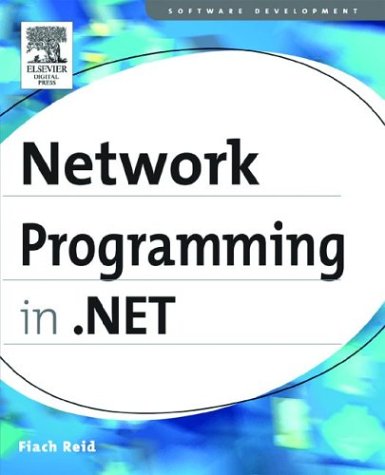
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebBrowserHelper.cs
- Vector3DValueSerializer.cs
- WorkflowMessageEventHandler.cs
- ConstraintManager.cs
- DataGridViewButtonCell.cs
- DataRelation.cs
- StoreContentChangedEventArgs.cs
- XmlCollation.cs
- GroupPartitionExpr.cs
- ClientUtils.cs
- SQlBooleanStorage.cs
- RequestTimeoutManager.cs
- CookieProtection.cs
- XmlSerializerSection.cs
- DataTableReaderListener.cs
- ProfileSettings.cs
- HTTPNotFoundHandler.cs
- ReadOnlyObservableCollection.cs
- SqlBuffer.cs
- PriorityChain.cs
- WebBrowser.cs
- WebPartDescriptionCollection.cs
- DBCommand.cs
- Floater.cs
- PrintDialog.cs
- RoutedEventHandlerInfo.cs
- Transform.cs
- DelegatingHeader.cs
- WebPartDisplayModeCollection.cs
- Security.cs
- FullTextState.cs
- XD.cs
- DebugView.cs
- ScrollContentPresenter.cs
- cookiecontainer.cs
- CultureSpecificCharacterBufferRange.cs
- UserInitiatedNavigationPermission.cs
- ResolveResponseInfo.cs
- WhitespaceReader.cs
- HttpWebResponse.cs
- CommandLineParser.cs
- ToolStripDropDownClosingEventArgs.cs
- CollectionCodeDomSerializer.cs
- TransformConverter.cs
- DataChangedEventManager.cs
- ReflectionTypeLoadException.cs
- EpmCustomContentWriterNodeData.cs
- Pair.cs
- RelationshipNavigation.cs
- DateTimeOffsetAdapter.cs
- CreatingCookieEventArgs.cs
- CompilerParameters.cs
- ListViewHitTestInfo.cs
- TogglePattern.cs
- SqlCacheDependencySection.cs
- JsonWriter.cs
- DbTransaction.cs
- ListBase.cs
- HashRepartitionStream.cs
- MaskDescriptors.cs
- StateItem.cs
- TitleStyle.cs
- UnsafeNativeMethods.cs
- BinaryReader.cs
- ProxyGenerator.cs
- EmptyEnumerable.cs
- InternalsVisibleToAttribute.cs
- DocumentXPathNavigator.cs
- DoubleAnimationUsingKeyFrames.cs
- CharAnimationBase.cs
- XmlIlGenerator.cs
- DataGridColumn.cs
- InputLanguageSource.cs
- RankException.cs
- listviewsubitemcollectioneditor.cs
- ListViewPagedDataSource.cs
- MemberDomainMap.cs
- formatter.cs
- SafeLibraryHandle.cs
- HtmlInputImage.cs
- BitHelper.cs
- XmlAutoDetectWriter.cs
- ObfuscationAttribute.cs
- COM2ExtendedBrowsingHandler.cs
- StateChangeEvent.cs
- ContentType.cs
- Pkcs7Recipient.cs
- IndentedTextWriter.cs
- XmlSchemaInclude.cs
- BrowserCapabilitiesCompiler.cs
- SyntaxCheck.cs
- MemoryStream.cs
- FontConverter.cs
- DataPagerCommandEventArgs.cs
- DbModificationClause.cs
- ComponentRenameEvent.cs
- SQLBoolean.cs
- Form.cs
- ModelFactory.cs
- DesignConnectionCollection.cs