Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / Floater.cs / 1305600 / Floater.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Floater element. // //--------------------------------------------------------------------------- using System.ComponentModel; using MS.Internal; using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS restrictions namespace System.Windows.Documents { ////// Floater element /// public class Floater : AnchoredBlock { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Static ctor. Initializes property metadata. /// static Floater() { DefaultStyleKeyProperty.OverrideMetadata(typeof(Floater), new FrameworkPropertyMetadata(typeof(Floater))); } ////// Initialized the new instance of a Floater /// public Floater() : this(null, null) { } ////// Initialized the new instance of a Floater specifying a Block added /// to a Floater as its first child. /// /// /// Block added as a first initial child of the Floater. /// public Floater(Block childBlock) : this(childBlock, null) { } ////// Creates a new Floater instance. /// /// /// Optional child of the new Floater, may be null. /// /// /// Optional position at which to insert the new Floater. May /// be null. /// public Floater(Block childBlock, TextPointer insertionPosition) : base(childBlock, insertionPosition) { } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// DependencyProperty for public static readonly DependencyProperty HorizontalAlignmentProperty = FrameworkElement.HorizontalAlignmentProperty.AddOwner( typeof(Floater), new FrameworkPropertyMetadata( HorizontalAlignment.Stretch, FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// /// public HorizontalAlignment HorizontalAlignment { get { return (HorizontalAlignment)GetValue(HorizontalAlignmentProperty); } set { SetValue(HorizontalAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty WidthProperty = DependencyProperty.Register( "Width", typeof(double), typeof(Floater), new FrameworkPropertyMetadata( Double.NaN, FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsValidWidth)); ///property. /// /// The Width property specifies the width of the element. /// [TypeConverter(typeof(LengthConverter))] public double Width { get { return (double)GetValue(WidthProperty); } set { SetValue(WidthProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidWidth(object o) { double width = (double)o; double maxWidth = Math.Min(1000000, PTS.MaxPageSize); if (Double.IsNaN(width)) { // Default value of width is NaN return true; } if (width < 0 || width > maxWidth) { return false; } return true; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
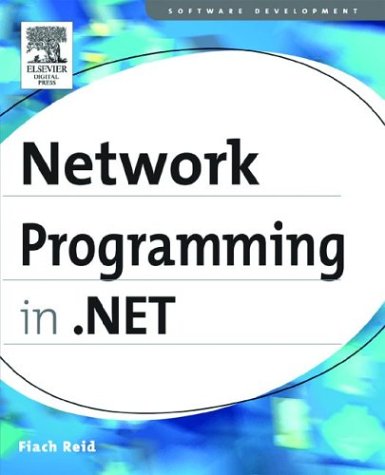
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlHierarchicalDataSourceView.cs
- WS2007FederationHttpBinding.cs
- ConfigXmlAttribute.cs
- InfoCardX509Validator.cs
- TransferRequestHandler.cs
- XmlAutoDetectWriter.cs
- KeyProperty.cs
- EditingCoordinator.cs
- Random.cs
- TextTreeDeleteContentUndoUnit.cs
- CleanUpVirtualizedItemEventArgs.cs
- WorkflowInstanceAbortedRecord.cs
- Queue.cs
- SecurityRuntime.cs
- StorageEndPropertyMapping.cs
- KeyManager.cs
- OleDbParameter.cs
- WrappedKeySecurityToken.cs
- Timer.cs
- StringUtil.cs
- TemplatedWizardStep.cs
- MetadataArtifactLoaderFile.cs
- ItemsControl.cs
- ListViewUpdateEventArgs.cs
- BrushConverter.cs
- ConfigXmlComment.cs
- ProfilePropertySettings.cs
- ControlTemplate.cs
- DbDataReader.cs
- RoleGroup.cs
- PropertyToken.cs
- TypeExtension.cs
- OutputScope.cs
- FindCriteriaApril2005.cs
- SettingsContext.cs
- ObjectReferenceStack.cs
- XmlCharCheckingWriter.cs
- TracedNativeMethods.cs
- ConsoleCancelEventArgs.cs
- URLIdentityPermission.cs
- EntityContainerEmitter.cs
- Code.cs
- UTF32Encoding.cs
- SourceSwitch.cs
- SocketManager.cs
- Message.cs
- ToolStripItemCollection.cs
- MetadataPropertyAttribute.cs
- WebPartDisplayModeEventArgs.cs
- PersonalizationStateQuery.cs
- ChildrenQuery.cs
- Vector3DAnimationBase.cs
- EventMappingSettings.cs
- InternalBufferOverflowException.cs
- HttpCacheVary.cs
- StylusButton.cs
- StrokeCollectionDefaultValueFactory.cs
- DesignTimeVisibleAttribute.cs
- FontResourceCache.cs
- AmbientProperties.cs
- FixedTextView.cs
- TransportBindingElementImporter.cs
- CodeCommentStatement.cs
- BackoffTimeoutHelper.cs
- AttachedPropertyMethodSelector.cs
- XmlSerializerSection.cs
- RetrieveVirtualItemEventArgs.cs
- QilList.cs
- TransactionManager.cs
- PropertyGridEditorPart.cs
- Int64Animation.cs
- PolicyException.cs
- StoreContentChangedEventArgs.cs
- SettingsBase.cs
- RegexTree.cs
- MaskedTextProvider.cs
- SerializationHelper.cs
- ValueUtilsSmi.cs
- StreamInfo.cs
- UserPreferenceChangedEventArgs.cs
- DynamicDiscoveryDocument.cs
- EmptyQuery.cs
- Rect3D.cs
- OdbcReferenceCollection.cs
- ZipPackagePart.cs
- TabItemWrapperAutomationPeer.cs
- HashMembershipCondition.cs
- HostedElements.cs
- ProfileService.cs
- ParallelLoopState.cs
- ResourceManagerWrapper.cs
- GeneralTransform3DTo2DTo3D.cs
- DynamicPropertyReader.cs
- ContextMenu.cs
- DoubleCollectionValueSerializer.cs
- FormViewDeleteEventArgs.cs
- EntityObject.cs
- NotifyInputEventArgs.cs
- IsolatedStorageFilePermission.cs
- PropertyInformation.cs