Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / PeerService.cs / 1 / PeerService.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel.Dispatcher; using System.ServiceModel.Description; using System.Diagnostics; using System.Collections.ObjectModel; using System.Net; using System.ServiceModel.Diagnostics; using System.ServiceModel; // What the connector interface needs to looks like interface IPeerConnectorContract { void Connect (IPeerNeighbor neighbor, ConnectInfo connectInfo); void Disconnect(IPeerNeighbor neighbor, DisconnectInfo disconnectInfo); void Refuse (IPeerNeighbor neighbor, RefuseInfo refuseInfo); void Welcome (IPeerNeighbor neighbor, WelcomeInfo welcomeInfo); } // Implemented by flooder / service uses this to delegate service invocations interface IPeerFlooderContract{ //invoked by the peerservice IAsyncResult OnFloodedMessage(IPeerNeighbor neighbor, TFloodContract floodedInfo, AsyncCallback callback, object state); void EndFloodMessage(IAsyncResult result); void ProcessLinkUtility(IPeerNeighbor neighbor, TLinkContract utilityInfo); } // Class that implements IPeerService contract for incoming neighbor sessions and messages. // WARNING: This class is not synchronized. Expects the using class to synchronize access [ ServiceBehavior( ConcurrencyMode=ConcurrencyMode.Multiple, InstanceContextMode=InstanceContextMode.Single, UseSynchronizationContext=false ) ] class PeerService : IPeerService, IServiceBehavior, IChannelInitializer { public delegate bool ChannelCallback(IClientChannel channel); public delegate IPeerNeighbor GetNeighborCallback(IPeerProxy channel); Binding binding; PeerNodeConfig config; ChannelCallback newChannelCallback; GetNeighborCallback getNeighborCallback; ServiceHost serviceHost; // To listen for incoming neighbor sessions IPeerConnectorContract connector; IPeerFlooderContract flooder; IPeerNodeMessageHandling messageHandler; public PeerService(PeerNodeConfig config, ChannelCallback channelCallback, GetNeighborCallback getNeighborCallback, Dictionary services) : this(config, channelCallback, getNeighborCallback, services, null) { } public PeerService( PeerNodeConfig config, ChannelCallback channelCallback, GetNeighborCallback getNeighborCallback, Dictionary services, IPeerNodeMessageHandling messageHandler) { this.config = config; this.newChannelCallback = channelCallback; DiagnosticUtility.DebugAssert(getNeighborCallback != null, "getNeighborCallback must be passed to PeerService constructor"); this.getNeighborCallback = getNeighborCallback; this.messageHandler = messageHandler; if(services != null) { object reply = null; services.TryGetValue(typeof(IPeerConnectorContract), out reply); connector = reply as IPeerConnectorContract; DiagnosticUtility.DebugAssert(connector != null, "PeerService must be created with a connector implementation"); reply = null; services.TryGetValue(typeof(IPeerFlooderContract ), out reply); flooder = reply as IPeerFlooderContract ; DiagnosticUtility.DebugAssert(flooder != null, "PeerService must be created with a flooder implementation"); } this.serviceHost = new ServiceHost(this); // Add throttling ServiceThrottlingBehavior throttle = new ServiceThrottlingBehavior(); throttle.MaxConcurrentCalls = this.config.MaxPendingIncomingCalls; throttle.MaxConcurrentSessions = this.config.MaxConcurrentSessions; this.serviceHost.Description.Behaviors.Add(throttle); } public void Abort() { this.serviceHost.Abort(); } public Binding Binding { get { return this.binding; } } // Create the binding using user specified config. The stacking is // BinaryMessageEncoder/TCP void CreateBinding() { Collection bindingElements = new Collection (); BindingElement security = this.config.SecurityManager.GetSecurityBindingElement(); if(security != null) { bindingElements.Add(security); } TcpTransportBindingElement transport = new TcpTransportBindingElement(); transport.MaxReceivedMessageSize = this.config.MaxReceivedMessageSize; transport.MaxBufferPoolSize = this.config.MaxBufferPoolSize; transport.TeredoEnabled = true; MessageEncodingBindingElement encoder = null; if(messageHandler != null) encoder = messageHandler.EncodingBindingElement; if(encoder == null) { BinaryMessageEncodingBindingElement bencoder = new BinaryMessageEncodingBindingElement(); this.config.ReaderQuotas.CopyTo(bencoder.ReaderQuotas); bindingElements.Add(bencoder); } else { bindingElements.Add(encoder); } bindingElements.Add(transport); this.binding = new CustomBinding(bindingElements); this.binding.ReceiveTimeout = TimeSpan.MaxValue; } // Returns the address that the serviceHost is listening on. public EndpointAddress GetListenAddress() { IChannelListener listener = this.serviceHost.ChannelDispatchers[0].Listener; return new EndpointAddress(listener.Uri, listener.GetProperty ()); } IPeerNeighbor GetNeighbor() { IPeerNeighbor neighbor = (IPeerNeighbor)getNeighborCallback(OperationContext.Current.GetCallbackChannel ()); if (neighbor == null || neighbor.State == PeerNeighborState.Closed) { if (DiagnosticUtility.ShouldTraceWarning) { TraceUtility.TraceEvent(TraceEventType.Warning, TraceCode.PeerNeighborNotFound, new PeerNodeTraceRecord(config.NodeId), this, null, OperationContext.Current.IncomingMessage); } return null; } if (DiagnosticUtility.ShouldTraceVerbose) { PeerNeighborState state = neighbor.State; PeerNodeAddress listenAddr = null; IPAddress connectIPAddr = null; if (state >= PeerNeighborState.Opened && state <= PeerNeighborState.Connected) { listenAddr = config.GetListenAddress(true); connectIPAddr = config.ListenIPAddress; } PeerNeighborTraceRecord record = new PeerNeighborTraceRecord(neighbor.NodeId, this.config.NodeId, listenAddr, connectIPAddr, neighbor.GetHashCode(), neighbor.IsInitiator, state.ToString(), null, null, OperationContext.Current.IncomingMessage.Headers.Action); TraceUtility.TraceEvent(TraceEventType.Verbose, TraceCode.PeerNeighborMessageReceived, record, this, null); } return neighbor; } public void Open(TimeSpan timeout) { // Create the neighbor binding CreateBinding(); this.serviceHost.Description.Endpoints.Clear(); ServiceEndpoint endPoint = this.serviceHost.AddServiceEndpoint(typeof(IPeerService), this.binding, config.GetMeshUri()); endPoint.ListenUri = config.GetSelfUri(); endPoint.ListenUriMode = (this.config.Port > 0) ? ListenUriMode.Explicit : ListenUriMode.Unique; /* Uncomment this to allow the retrieval of metadata using the command: \binaries.x86chk\svcutil http://localhost /t:metadata ServiceMetadataBehavior mex = new ServiceMetadataBehavior(); mex.HttpGetEnabled = true; mex.HttpGetUrl = new Uri("http://localhost"); mex.HttpsGetEnabled = true; mex.HttpsGetUrl = new Uri("https://localhost"); this.serviceHost.Description.Behaviors.Add(mex); */ this.config.SecurityManager.ApplyServiceSecurity(this.serviceHost.Description); this.serviceHost.Open(timeout); if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.PeerServiceOpened, SR.GetString(SR.TraceCodePeerServiceOpened, this.GetListenAddress()), null, null, this); } } // // IContractBehavior and IChannelInitializer implementation. // Used to register for incoming channel notification. // void IServiceBehavior.Validate(ServiceDescription description, ServiceHostBase serviceHost) { } void IServiceBehavior.AddBindingParameters(ServiceDescription description, ServiceHostBase serviceHost, Collection endpoints, BindingParameterCollection parameters) { } void IServiceBehavior.ApplyDispatchBehavior(ServiceDescription description, ServiceHostBase serviceHost) { for (int i=0; i
Link Menu
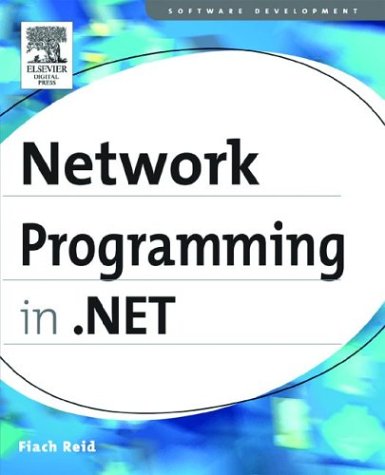
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParameterRefs.cs
- CacheEntry.cs
- Soap12ServerProtocol.cs
- Input.cs
- SHA256Managed.cs
- ObjectPersistData.cs
- DbExpressionVisitor.cs
- SimpleType.cs
- XmlSchemaSimpleContent.cs
- mongolianshape.cs
- VirtualDirectoryMapping.cs
- CounterNameConverter.cs
- MemberAccessException.cs
- MULTI_QI.cs
- ListMarkerSourceInfo.cs
- PropertyDescriptorComparer.cs
- DesignTimeParseData.cs
- ArglessEventHandlerProxy.cs
- IndentedWriter.cs
- BooleanExpr.cs
- ScriptServiceAttribute.cs
- DynamicResourceExtensionConverter.cs
- OracleRowUpdatedEventArgs.cs
- FunctionImportElement.cs
- FixedTextContainer.cs
- Version.cs
- WebPartChrome.cs
- DesignerProperties.cs
- MetafileHeaderEmf.cs
- Types.cs
- SliderAutomationPeer.cs
- TriState.cs
- XpsPackagingPolicy.cs
- XmlAnyAttributeAttribute.cs
- LogoValidationException.cs
- PackageRelationshipSelector.cs
- DrawListViewSubItemEventArgs.cs
- SequenceQuery.cs
- HtmlAnchor.cs
- WebPartManagerInternals.cs
- HashUtility.cs
- XmlSecureResolver.cs
- XmlParserContext.cs
- ValidationEventArgs.cs
- MachineKeyConverter.cs
- MemoryRecordBuffer.cs
- Vector.cs
- MediaElementAutomationPeer.cs
- SqlTypeConverter.cs
- _emptywebproxy.cs
- CommunicationException.cs
- XmlSchemaSet.cs
- __FastResourceComparer.cs
- DataTablePropertyDescriptor.cs
- ValidationPropertyAttribute.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- UIElementIsland.cs
- BookmarkTable.cs
- DataFieldEditor.cs
- StrongNamePublicKeyBlob.cs
- ProviderUtil.cs
- Int16KeyFrameCollection.cs
- FunctionImportElement.cs
- PointConverter.cs
- EntityDesignerUtils.cs
- SecurityTokenException.cs
- AlphabeticalEnumConverter.cs
- AcceptorSessionSymmetricTransportSecurityProtocol.cs
- ReadOnlyHierarchicalDataSourceView.cs
- IncomingWebResponseContext.cs
- DefaultParameterValueAttribute.cs
- KnownColorTable.cs
- DataGridViewRowsRemovedEventArgs.cs
- ChildrenQuery.cs
- ToolStripRenderEventArgs.cs
- TextRunTypographyProperties.cs
- ServicesUtilities.cs
- Configuration.cs
- AnimationTimeline.cs
- HwndStylusInputProvider.cs
- MdiWindowListStrip.cs
- CopyCodeAction.cs
- CodeTypeParameterCollection.cs
- BitmapEffectvisualstate.cs
- HttpCachePolicyElement.cs
- MissingMemberException.cs
- columnmapfactory.cs
- WorkflowView.cs
- HealthMonitoringSectionHelper.cs
- UriTemplateTrieLocation.cs
- DataFormats.cs
- Exceptions.cs
- HttpPostProtocolImporter.cs
- Aggregates.cs
- DataGridViewColumnHeaderCell.cs
- PageTheme.cs
- StrokeCollection.cs
- TouchDevice.cs
- ConfigViewGenerator.cs
- UITypeEditor.cs