Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / SMSvcHost / System / ServiceModel / Activation / ListenerConnectionModeReader.cs / 1 / ListenerConnectionModeReader.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.Collections.Generic; using System.Diagnostics; using System.ServiceModel; using System.ServiceModel.Channels; using System.Threading; using System.ServiceModel.Activation.Diagnostics; delegate void ListenerConnectionModeCallback(ListenerConnectionModeReader connectionModeReader); sealed class ListenerConnectionModeReader : InitialServerConnectionReader { Exception readException; ServerModeDecoder decoder; byte[] buffer; int offset; int size; ListenerConnectionModeCallback callback; static WaitCallback readCallback; byte[] accruedData; TimeoutHelper receiveTimeoutHelper; public ListenerConnectionModeReader(IConnection connection, ListenerConnectionModeCallback callback, ConnectionClosedCallback closedCallback) : base(connection, closedCallback) { this.callback = callback; } public int BufferOffset { get { return offset; } } public int BufferSize { get { return size; } } public long StreamPosition { get { return decoder.StreamPosition; } } public TimeSpan GetRemainingTimeout() { return this.receiveTimeoutHelper.RemainingTime(); } void Complete(Exception e) { // exception will be logged by the caller readException = e; Complete(); } void Complete() { callback(this); } bool ContinueReading() { while (true) { if (size == 0) { if (readCallback == null) { readCallback = new WaitCallback(ReadCallback); } // if we already have buffered some data we need // to accrue it in case we're duping the connection if (buffer != null) { int dataOffset = 0; if (accruedData == null) { accruedData = new byte[offset]; } else { byte[] newAccruedData = new byte[accruedData.Length + offset]; Buffer.BlockCopy(accruedData, 0, newAccruedData, 0, accruedData.Length); dataOffset = this.accruedData.Length; accruedData = newAccruedData; } Buffer.BlockCopy(buffer, 0, accruedData, dataOffset, offset); } if (Connection.BeginRead(0, Connection.AsyncReadBufferSize, GetRemainingTimeout(), readCallback, this) == AsyncReadResult.Queued) { return false; } GetReadResult(); } while (true) { int bytesDecoded = decoder.Decode(buffer, offset, size); if (bytesDecoded > 0) { offset += bytesDecoded; size -= bytesDecoded; } if (decoder.CurrentState == ServerModeDecoder.State.Done) { return true; } if (size == 0) { break; } } } } static void ReadCallback(object state) { ListenerConnectionModeReader reader = (ListenerConnectionModeReader)state; bool completeSelf = false; Exception completionException = null; try { if (reader.GetReadResult()) { completeSelf = reader.ContinueReading(); } } #pragma warning suppress 56500 // [....], transferring exception to caller catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } completeSelf = true; completionException = e; } if (completeSelf) { reader.Complete(completionException); } } bool GetReadResult() { offset = 0; size = Connection.EndRead(); if (size == 0) { if (this.decoder.StreamPosition == 0) // client timed out a cached connection { base.Close(GetRemainingTimeout()); return false; } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(decoder.CreatePrematureEOFException()); } } if (buffer == null) { buffer = Connection.AsyncReadBuffer; } return true; } public FramingMode GetConnectionMode() { if (readException != null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(readException); } return decoder.Mode; } public void StartReading(TimeSpan timeout, ItemDequeuedCallback connectionDequeuedCallback) { this.receiveTimeoutHelper = new TimeoutHelper(timeout); this.decoder = new ServerModeDecoder(); this.ConnectionDequeuedCallback = connectionDequeuedCallback; bool completeSelf; try { completeSelf = ContinueReading(); } #pragma warning suppress 56500 // [....], transferring exception to caller catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } // exception will be logged by the caller this.readException = e; completeSelf = true; } if (completeSelf) { Complete(); } } public byte[] AccruedData { get { return this.accruedData; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
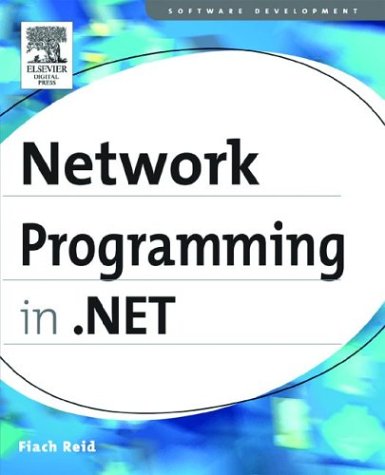
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValueOfAction.cs
- SerializationInfoEnumerator.cs
- MultiPartWriter.cs
- WebBrowserDesigner.cs
- OrderedDictionary.cs
- Main.cs
- PreservationFileWriter.cs
- RenderCapability.cs
- TransformedBitmap.cs
- TileBrush.cs
- FloaterBaseParaClient.cs
- XpsImageSerializationService.cs
- Int16KeyFrameCollection.cs
- TypeBuilder.cs
- InternalPolicyElement.cs
- WS2007HttpBinding.cs
- ControlParser.cs
- RedirectionProxy.cs
- Message.cs
- Drawing.cs
- PassportAuthenticationModule.cs
- SrgsText.cs
- HttpSessionStateBase.cs
- DataGridViewComponentPropertyGridSite.cs
- AnnouncementSendsAsyncResult.cs
- WorkflowMarkupSerializationException.cs
- VideoDrawing.cs
- DataGridViewCellCollection.cs
- RoutedEventValueSerializer.cs
- LingerOption.cs
- FamilyTypefaceCollection.cs
- StartUpEventArgs.cs
- IPPacketInformation.cs
- TextStore.cs
- KeyToListMap.cs
- NativeWindow.cs
- LocationEnvironment.cs
- DocumentNUp.cs
- Win32Exception.cs
- EntityViewGenerator.cs
- PopupEventArgs.cs
- Pair.cs
- _NegoStream.cs
- NativeMethods.cs
- CallSiteBinder.cs
- RegexRunner.cs
- PartialArray.cs
- SimpleWorkerRequest.cs
- Activator.cs
- RestrictedTransactionalPackage.cs
- UnsafeNativeMethods.cs
- XmlSchemaComplexContent.cs
- DocumentOrderQuery.cs
- ObjectContextServiceProvider.cs
- DataServiceRequestException.cs
- StringConcat.cs
- InstalledFontCollection.cs
- Empty.cs
- ParallelForEach.cs
- ArcSegment.cs
- TemplateApplicationHelper.cs
- StringOutput.cs
- OutputScopeManager.cs
- CrossAppDomainChannel.cs
- WinFormsSecurity.cs
- WindowsAuthenticationModule.cs
- ExpandableObjectConverter.cs
- ToolBarPanel.cs
- PrePrepareMethodAttribute.cs
- BuildManagerHost.cs
- ReadOnlyHierarchicalDataSource.cs
- InheritanceRules.cs
- HttpInputStream.cs
- FrameworkElement.cs
- XmlSchemaComplexContentExtension.cs
- StylusCaptureWithinProperty.cs
- RequestCachingSection.cs
- XPathNodePointer.cs
- mansign.cs
- TableParaClient.cs
- ValueUtilsSmi.cs
- DataTemplateSelector.cs
- XmlChildEnumerator.cs
- ActivityMarkupSerializer.cs
- TokenBasedSet.cs
- SplitterPanel.cs
- InvalidContentTypeException.cs
- Vector3DCollection.cs
- SamlDelegatingWriter.cs
- mediapermission.cs
- PaginationProgressEventArgs.cs
- ZipIORawDataFileBlock.cs
- WmlSelectionListAdapter.cs
- TableCellAutomationPeer.cs
- SByteStorage.cs
- DBConnection.cs
- Renderer.cs
- FontFamily.cs
- processwaithandle.cs
- WCFBuildProvider.cs