Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / SMSvcHost / System / ServiceModel / Activation / ListenerAdapter.cs / 1 / ListenerAdapter.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.ComponentModel; using System.Diagnostics; using System.Security.Principal; using System.ServiceModel; using System.ServiceModel.Channels; using System.Threading; using System.ServiceModel.Diagnostics; using System.ServiceModel.Activation.Diagnostics; using System.Globalization; using System.Collections.Generic; using TraceUtility = System.ServiceModel.Diagnostics.TraceUtility; class ListenerAdapter : ListenerAdapterBase { const string SiteRootPath = "/"; IActivationService activationService; bool canDispatch; bool isOpen; AutoResetEvent wasConnected; AutoResetEvent cleanupComplete; AppManager appManager; ManualResetEvent initCompleted; WaitCallback closeAllListenerChannelInstancesCallback; WaitCallback launchQueueInstanceCallback; internal ListenerAdapter(IActivationService activationService) : base(activationService.ProtocolName) { this.activationService = activationService; appManager = new AppManager(); } object ThisLock { get { return this; } } internal bool CanDispatch { get { return canDispatch; } } internal override void Open() { Debug.Print("ListenerAdapter[" + protocolName + "]::Open()"); if (!isOpen) { lock (ThisLock) { if (!isOpen) { initCompleted = new ManualResetEvent(false); base.Open(); initCompleted.WaitOne(); initCompleted.Close(); initCompleted = null; isOpen = true; } } } } internal new void Close() { Debug.Print("ListenerAdapter[" + protocolName + "]::Close()"); if (isOpen) { lock (ThisLock) { if (isOpen) { isOpen = false; // When calling Cleanup() in the graceful case, we must wait for all // OnApplicationPoolAllQueueInstancesStopped callbacks to fire before we can stop cleanupComplete = new AutoResetEvent(false); if (!Cleanup(true)) { cleanupComplete.WaitOne(ListenerConstants.ServiceStopTimeout, false); } // base.Close causes WebhostUnregisterProtocol to be called. base.Close(); } } } } bool Cleanup(bool closeInstances) { Debug.Print("ListenerAdapter[" + protocolName + "]::Cleanup()"); canDispatch = false; bool completeSelf = true; if (closeInstances) { ListexistingApps = new List (); List removeApps = new List (); List delayRemoveApps = new List (); lock (appManager) { if (appManager.AppsCount != 0) { // cleanup for activation service stop: tell WAS about it existingApps.AddRange(appManager.Apps.Values); foreach (App app in existingApps) { if (app.MessageQueue.HasStartedQueueInstances) { delayRemoveApps.Add(app); } else { removeApps.Add(app); } } existingApps.Clear(); } } if (removeApps.Count != 0) { foreach (App app in removeApps) { RemoveApp(app); } } if (delayRemoveApps.Count != 0) { foreach (App app in delayRemoveApps) { if (app.PendingAction != null) { app.PendingAction.MergeFromDeletedAction(); } else { // Create a new action app.SetPendingAction(AppAction.CreateDeletedAction()); CloseAllListenerChannelInstances(app); } } completeSelf = false; } } else { lock (appManager) { appManager.Clear(); } } return completeSelf; } void CloseAllListenerChannelInstances(App app) { int hresult = CloseAllListenerChannelInstances(app.AppPool.AppPoolId, app.MessageQueue.ListenerChannelContext.ListenerChannelId); Debug.Print("ListenerAdapter[" + protocolName + "]::CloseAllListenerChannelInstances(" + app.AppKey + ") returned: " + hresult); if (hresult == 0) { if (DiagnosticUtility.ShouldTraceInformation) { ListenerTraceUtility.TraceEvent(TraceEventType.Information, TraceCode.WasCloseAllListenerChannelInstances, this); } } else { if (DiagnosticUtility.ShouldTraceError) { ListenerTraceUtility.TraceEvent(TraceEventType.Error, TraceCode.WasWebHostAPIFailed, new StringTraceRecord("HRESULT", SR.GetString(SR.TraceCodeWasWebHostAPIFailed, "WebhostCloseAllListenerChannelInstances", hresult.ToString(CultureInfo.CurrentCulture))), this, null); } } } protected override void OnApplicationAppPoolChanged(string appKey, string appPoolId) { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationAppPoolChanged(" + appKey + ", " + appPoolId + ")"); try { App app = null; lock (appManager) { // The app might have been removed due to the service shutdown. if (!appManager.Apps.TryGetValue(appKey, out app)) { return; } } if (app.PendingAction != null) { app.PendingAction.MergeFromAppPoolChangedAction(appPoolId); } else { if (app.MessageQueue.HasStartedQueueInstances) { // Create a new action app.SetPendingAction(AppAction.CreateAppPoolChangedAction(appPoolId)); ScheduleClosingListenerChannelInstances(app); } else { CompleteAppPoolChange(app, appPoolId); } } } catch (Exception exception) { HandleUnknownError(exception); } } internal void CompleteAppPoolChange(App app, string appPoolId) { lock (appManager) { AppPool appPool; if (!appManager.AppPools.TryGetValue(appPoolId, out appPool)) { // The AppPool has been removed return; } if (appPool != app.AppPool) { app.AppPool.RemoveApp(app); appPool.AddApp(app); app.OnAppPoolChanged(appPool); } } } protected override void OnApplicationDeleted(string appKey) { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationDeleted(" + appKey + ")"); try { App app = null; lock (appManager) { // The app must exist when this notification is received. app = appManager.Apps[appKey]; } if (app.PendingAction != null) { app.PendingAction.MergeFromDeletedAction(); } else { if (app.MessageQueue.HasStartedQueueInstances) { // Creae a new action app.SetPendingAction(AppAction.CreateDeletedAction()); ScheduleClosingListenerChannelInstances(app); } else { CompleteDeleteApp(app); } } } catch (Exception exception) { HandleUnknownError(exception); } } internal void CompleteDeleteApp(App app) { RemoveApp(app); } void CompleteAppSettingsChanged(App app) { if (app.PendingAction.AppPoolId != null) { CompleteAppPoolChange(app, app.PendingAction.AppPoolId); } if (app.PendingAction.Path != null) { app.Path = app.PendingAction.Path; } if (app.PendingAction.Bindings != null) { RegisterNewBindings(app, app.PendingAction.Bindings); } if (app.PendingAction.RequestsBlocked.HasValue) { app.SetRequestBlocked(app.PendingAction.RequestsBlocked.Value); } } void RemoveApp(App app) { Debug.Print("ListenerAdapter[" + protocolName + "]::RemoveApp(" + app.AppKey + ")"); lock (appManager) { // The App might have been deleted when the AppPool is deleted. if (appManager.Apps.ContainsKey(app.AppKey)) { appManager.DeleteApp(app, false); } } } protected override void OnApplicationBindingsChanged(string appKey, IntPtr bindingsMultiSz, int numberOfBindings) { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationBindingsChanged(" + appKey + ")"); string[] bindings = null; try { bindings = base.ParseBindings(bindingsMultiSz, numberOfBindings); } catch (ArgumentException exception) { if (DiagnosticUtility.ShouldTraceError) { DiagnosticUtility.ExceptionUtility.TraceHandledException(exception, TraceEventType.Error); } // Ignore the binding change if WAS provides wrong bindings. return; } App app = null; lock (appManager) { // The app might have been removed due to the service shutdown. if (!appManager.Apps.TryGetValue(appKey, out app)) { return; } } try { if (app.PendingAction != null) { app.PendingAction.MergeFromBindingChangedAction(bindings); } else { if (app.MessageQueue.HasStartedQueueInstances) { app.SetPendingAction(AppAction.CreateBindingsChangedAction(bindings)); ScheduleClosingListenerChannelInstances(app); } else { RegisterNewBindings(app, bindings); } } } catch (Exception exception) { HandleUnknownError(exception); } } internal void RegisterNewBindings(App app, string[] bindings) { Debug.Print("ListenerAdapter[" + protocolName + "]::RegisterNewBindings(" + app.AppKey + ")"); // we could be smart-er and leave the bindings that have not changed alone app.MessageQueue.UnregisterAll(); bool success = RegisterBindings(app.MessageQueue, app.SiteId, bindings, app.Path); app.OnInvalidBinding(!success); } protected override void OnApplicationCreated(string appKey, string path, int siteId, string appPoolId, IntPtr bindingsMultiSz, int numberOfBindings, bool requestsBlocked) { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationCreated(" + appKey + ", " + path + ", " + siteId + ", " + appPoolId + ", " + requestsBlocked + ")"); string[] bindings = null; try { bindings = base.ParseBindings(bindingsMultiSz, numberOfBindings); } catch (ArgumentException exception) { if (DiagnosticUtility.ShouldTraceError) { DiagnosticUtility.ExceptionUtility.TraceHandledException(exception, TraceEventType.Error); } // Ignore the app if WAS provides wrong bindings. return; } try { bool found = true; App app = null; lock(appManager) { if (!appManager.Apps.TryGetValue(appKey, out app)) { found = false; app = appManager.CreateApp(appKey, path, siteId, appPoolId, requestsBlocked); } } if (found) { DiagnosticUtility.DebugAssert(app.PendingAction != null, "The app should be waiting for AllLCStopped notification."); app.PendingAction.MergeFromCreatedAction(path, siteId, appPoolId, requestsBlocked, bindings); } else { CompleteAppCreation(app, bindings); } } catch (Exception exception) { HandleUnknownError(exception); } } void CompleteAppCreation(App app, string[] bindings) { IActivatedMessageQueue queue = activationService.CreateQueue(this, app); app.RegisterQueue(queue); bool success = RegisterBindings(app.MessageQueue, app.SiteId, bindings, app.Path); app.OnInvalidBinding(!success); } bool RegisterBindings(IActivatedMessageQueue queue, int siteId, string[] bindings, string path) { Debug.Print("ListenerAdapter[" + protocolName + "]::RegisterBindings() bindings#: " + bindings.Length); BaseUriWithWildcard[] baseAddresses = new BaseUriWithWildcard[bindings.Length]; // first make sure all the bindings are valid for this protocol for (int i = 0; i < bindings.Length; i++) { string binding = bindings[i]; int index = binding.IndexOf(':'); string protocol = binding.Substring(0, index); if (string.Compare(this.protocolName, protocol, StringComparison.OrdinalIgnoreCase) != 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException( SR.GetString(SR.LAProtocolMismatch, protocol, path, this.protocolName))); } binding = binding.Substring(index + 1); try { baseAddresses[i] = BaseUriWithWildcard.CreateUri(protocolName, binding, path); Debug.Print("ListenerAdapter[" + protocolName + "]::RegisterBindings() CreateUrlFromBinding(binding: " + binding + " path: " + path + ") returned baseAddress: " + baseAddresses[i]); } catch (UriFormatException exception) { Debug.Print("ListenerAdapter[" + protocolName + "]::RegisterBindings() CreateUrlFromBinding(binding: " + binding + " path: " + path + ") failed with UriFormatException: " + exception.Message); if (DiagnosticUtility.ShouldTraceError) { DiagnosticUtility.ExceptionUtility.TraceHandledException(exception, TraceEventType.Error); } // We only log the event for the site root. if (string.Compare(path, SiteRootPath, StringComparison.OrdinalIgnoreCase) == 0) { DiagnosticUtility.EventLog.LogEvent(TraceEventType.Error, EventLogCategory.ListenerAdapter, EventLogEventId.BindingError, protocol, binding, siteId.ToString(NumberFormatInfo.CurrentInfo), bindings[i], DiagnosticTrace.CreateSourceString(this), exception.ToString()); } return false; } } // now make sure all the bindings can be listened on or roll back for (int i = 0; i < bindings.Length; i++) { ListenerExceptionStatus status = ListenerExceptionStatus.FailedToListen; Exception exception = null; try { status = queue.Register(baseAddresses[i]); Debug.Print("ListenerAdapter[" + protocolName + "]::RegisterBindings() registering baseAddress: " + baseAddresses[i] + " with queue returned: " + status); } catch (Exception ex) { if (DiagnosticUtility.IsFatal(ex)) { throw; } if (DiagnosticUtility.ShouldTraceError) { DiagnosticUtility.ExceptionUtility.TraceHandledException(exception, TraceEventType.Error); } exception = ex; } if (status != ListenerExceptionStatus.Success) { // We only log the event for the site root. if (string.Compare(path, SiteRootPath, StringComparison.OrdinalIgnoreCase) == 0) { DiagnosticUtility.EventLog.LogEvent(TraceEventType.Error, EventLogCategory.ListenerAdapter, EventLogEventId.LAFailedToListenForApp, activationService.ActivationServiceName, protocolName, siteId.ToString(NumberFormatInfo.CurrentInfo), baseAddresses[i].ToString(), status.ToString(), exception == null ? string.Empty : exception.ToString()); } queue.UnregisterAll(); return false; } } return true; } protected override void OnApplicationPoolAllQueueInstancesStopped(string appPoolId, int queueId) { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationPoolAllQueueInstancesStopped(" + appPoolId + ", " + queueId + ")"); try { AppPool appPool = null; lock (appManager) { if (!appManager.AppPools.TryGetValue(appPoolId, out appPool)) { // Ignore this notification if we received OnApplicationPoolDeleted. return; } } IActivatedMessageQueue queue = activationService.FindQueue(queueId); if (queue == null) { // This is the belated notification. Ignore it. return; } DiagnosticUtility.DebugAssert(queue.App.AppPool.AppPoolId == appPoolId, "OnApplicationPoolAllQueueInstancesStopped: unexpected pool id"); queue.OnQueueInstancesStopped(); App app = queue.App; try { if (app.PendingAction.ActionType == AppActionType.Deleted) { CompleteDeleteApp(app); SignalCleanupForNoApps(); } else if (app.PendingAction.ActionType == AppActionType.SettingsChanged) { CompleteAppSettingsChanged(app); } } finally { // Reset the action app.SetPendingAction(null); } } catch (Exception exception) { HandleUnknownError(exception); } } protected override void OnApplicationPoolCanLaunchQueueInstance(string appPoolId, int queueId) { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationPoolCanLaunchQueueInstance(" + appPoolId + ", " + queueId + ")"); try { IActivatedMessageQueue queue = activationService.FindQueue(queueId); if (queue != null) { if (queue.App.AppPool.AppPoolId != appPoolId) { DiagnosticUtility.DebugAssert("OnApplicationPoolCanLaunchQueueInstance: unexpected pool id"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } ScheduleLaunchingQueueInstance(queue); } } catch (Exception exception) { HandleUnknownError(exception); } } protected override void OnApplicationPoolCreated(string appPoolId, SecurityIdentifier sid) { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationPoolCreated(" + appPoolId + ", " + sid + ")"); try { lock (appManager) { appManager.CreateAppPool(appPoolId, sid); } } catch (Exception exception) { HandleUnknownError(exception); } } protected override void OnApplicationPoolDeleted(string appPoolId) { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationPoolDeleted(" + appPoolId + ")"); try { lock(appManager) { appManager.DeleteAppPool(appPoolId); } SignalCleanupForNoApps(); } catch (Exception exception) { HandleUnknownError(exception); } } protected override void OnApplicationPoolIdentityChanged(string appPoolId, SecurityIdentifier sid) { try { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationPoolIdentityChanged(" + appPoolId + ", " + sid + ")"); AppPool appPool = null; lock (appManager) { if (!appManager.AppPools.TryGetValue(appPoolId, out appPool)) { return; } appPool.SetIdentity(sid); } } catch (Exception exception) { HandleUnknownError(exception); } } protected override void OnApplicationPoolStateChanged(string appPoolId, bool isEnabled) { try { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationPoolStateChanged(" + appPoolId + ", " + isEnabled + ")"); AppPool appPool = null; lock (appManager) { if (!appManager.AppPools.TryGetValue(appPoolId, out appPool)) { return; } appPool.SetEnabledState(isEnabled); } } catch (Exception exception) { HandleUnknownError(exception); } } protected override void OnApplicationRequestsBlockedChanged(string appKey, bool requestsBlocked) { try { Debug.Print("ListenerAdapter[" + protocolName + "]::OnApplicationRequestsBlockedChanged(" + appKey + ", " + requestsBlocked + ")"); App app = null; lock (appManager) { if (!appManager.Apps.TryGetValue(appKey, out app)) { return; } } if (app.PendingAction != null) { app.PendingAction.MergeFromRequestsBlockedAction(requestsBlocked); } else { app.SetRequestBlocked(requestsBlocked); } } catch (Exception exception) { HandleUnknownError(exception); } } protected override void OnConfigManagerConnected() { Debug.Print("ListenerAdapter[" + protocolName + "]::OnConfigManagerConnected()"); if (DiagnosticUtility.ShouldTraceInformation) { ListenerTraceUtility.TraceEvent(TraceEventType.Information, TraceCode.WasConnected, this); } if (wasConnected != null) { wasConnected.Set(); } } protected override void OnConfigManagerDisconnected(int hresult) { Debug.Print("ListenerAdapter[" + protocolName + "]::OnConfigManagerDisconnected(" + hresult + ") isOpen: " + isOpen); if (!isOpen) { // ignored this call, we are gracefully closing return; } DiagnosticUtility.EventLog.LogEvent(TraceEventType.Warning, EventLogCategory.ListenerAdapter, EventLogEventId.WasDisconnected, hresult.ToString(CultureInfo.InvariantCulture)); // WAS has crashed. Cleanup(false); wasConnected = new AutoResetEvent(false); ThreadPool.UnsafeRegisterWaitForSingleObject(wasConnected, DiagnosticUtility.Utility.ThunkCallback(new WaitOrTimerCallback(WasConnected)), null, ListenerConstants.WasConnectTimeout, true); } void WasConnected(object state, bool timedOut) { Debug.Print("ListenerAdapter[" + protocolName + "]::WasConnected() timedOut: " + timedOut); if (timedOut) { // WAS didn't connect within the timeout give up waiting and stop DiagnosticUtility.EventLog.LogEvent(TraceEventType.Warning, EventLogCategory.ListenerAdapter, EventLogEventId.WasConnectionTimedout); activationService.StopService(); } wasConnected.Close(); wasConnected = null; } protected override void OnConfigManagerInitializationCompleted() { Debug.Print("ListenerAdapter[" + protocolName + "]::OnConfigManagerInitializationCompleted()"); canDispatch = true; ManualResetEvent initCompleted = this.initCompleted; if (initCompleted != null) { initCompleted.Set(); } } internal bool OpenListenerChannelInstance(IActivatedMessageQueue queue) { byte[] queueBlob = queue.ListenerChannelContext.Dehydrate(); Debug.Print("ListenerAdapter[" + protocolName + "]::ListenerAdapter.OpenListenerChannelInstance(appPoolId:" + queue.App.AppPool.AppPoolId + " appKey:" + queue.ListenerChannelContext.AppKey + " queueId:" + queue.ListenerChannelContext.ListenerChannelId + ")"); int hresult = OpenListenerChannelInstance(queue.App.AppPool.AppPoolId, queue.ListenerChannelContext.ListenerChannelId, queueBlob); if (hresult != 0) { if (DiagnosticUtility.ShouldTraceError) { ListenerTraceUtility.TraceEvent(TraceEventType.Error, TraceCode.WasWebHostAPIFailed, new StringTraceRecord("HRESULT", SR.GetString(SR.TraceCodeWasWebHostAPIFailed, "WebhostOpenListenerChannelInstance", hresult.ToString(CultureInfo.CurrentCulture))), this, null); } return false; } return true; } void ScheduleClosingListenerChannelInstances(App app) { if (closeAllListenerChannelInstancesCallback == null) { closeAllListenerChannelInstancesCallback = new WaitCallback(OnCloseAllListenerChannelInstances); } IOThreadScheduler.ScheduleCallback(closeAllListenerChannelInstancesCallback, app); } void OnCloseAllListenerChannelInstances(object state) { App app = state as App; DiagnosticUtility.DebugAssert(app != null, "OnCloseAllListenerChannelInstances: app is null"); CloseAllListenerChannelInstances(app); } void ScheduleLaunchingQueueInstance(IActivatedMessageQueue queue) { if (launchQueueInstanceCallback == null) { launchQueueInstanceCallback = new WaitCallback(OnLaunchQueueInstance); } IOThreadScheduler.ScheduleCallback(launchQueueInstanceCallback, queue); } void OnLaunchQueueInstance(object state) { IActivatedMessageQueue queue = state as IActivatedMessageQueue; DiagnosticUtility.DebugAssert(queue != null, "OnLaunchQueueInstance: queue is null"); queue.LaunchQueueInstance(); } void HandleUnknownError(Exception exception) { DiagnosticUtility.EventLog.LogEvent(TraceEventType.Error, EventLogCategory.ListenerAdapter, EventLogEventId.UnknownListenerAdapterError, this.protocolName, exception.ToString()); // We cannot handle this exception and thus have to terminate the process. DiagnosticUtility.InvokeFinalHandler(exception); } void SignalCleanupForNoApps() { if (this.cleanupComplete != null) { lock (appManager) { if (appManager.AppsCount == 0) { // on graceful cleanup we need to unblock Close() when no app is left running this.appManager.Clear(); this.cleanupComplete.Set(); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
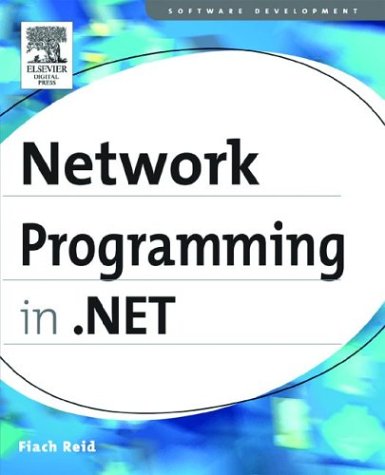
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RefreshPropertiesAttribute.cs
- XdrBuilder.cs
- Cursors.cs
- TogglePatternIdentifiers.cs
- PropertyOverridesDialog.cs
- LateBoundBitmapDecoder.cs
- HyperLinkField.cs
- CodeTypeReference.cs
- InfiniteTimeSpanConverter.cs
- GridViewRowPresenterBase.cs
- ObjectNavigationPropertyMapping.cs
- ListSortDescription.cs
- PrimitiveSchema.cs
- CrossAppDomainChannel.cs
- CommandHelpers.cs
- SqlCacheDependencySection.cs
- SamlAuthorizationDecisionClaimResource.cs
- DbMetaDataColumnNames.cs
- RegexReplacement.cs
- HttpException.cs
- HttpCacheParams.cs
- DuplicateWaitObjectException.cs
- DurationConverter.cs
- SerTrace.cs
- CommonGetThemePartSize.cs
- RegexCode.cs
- XamlWriter.cs
- FormsAuthenticationTicket.cs
- EventSetter.cs
- ColorContext.cs
- CompModSwitches.cs
- ManagedIStream.cs
- X509Utils.cs
- LoginCancelEventArgs.cs
- WebPartExportVerb.cs
- RegexCompilationInfo.cs
- RelationshipEndCollection.cs
- SystemWebSectionGroup.cs
- InputLanguageSource.cs
- EpmTargetPathSegment.cs
- TextBoxAutoCompleteSourceConverter.cs
- Identifier.cs
- SynthesizerStateChangedEventArgs.cs
- XmlAnyAttributeAttribute.cs
- EventProviderWriter.cs
- CollectionsUtil.cs
- InputScopeConverter.cs
- ButtonRenderer.cs
- XmlToDatasetMap.cs
- tabpagecollectioneditor.cs
- AvTraceFormat.cs
- ButtonColumn.cs
- CodeMethodMap.cs
- LocalizableAttribute.cs
- ClientType.cs
- DataSvcMapFile.cs
- PinnedBufferMemoryStream.cs
- SchemaMapping.cs
- RegexStringValidator.cs
- HttpWriter.cs
- RuleEngine.cs
- ButtonRenderer.cs
- SchemaElement.cs
- SubpageParaClient.cs
- OracleException.cs
- _FtpDataStream.cs
- Fault.cs
- Int64.cs
- CodeAccessPermission.cs
- TemplatePagerField.cs
- ForceCopyBuildProvider.cs
- CodeExporter.cs
- HtmlControl.cs
- AttributeCollection.cs
- XmlUnspecifiedAttribute.cs
- AnonymousIdentificationSection.cs
- OAVariantLib.cs
- TreeNodeCollection.cs
- BasicKeyConstraint.cs
- CodeGenerator.cs
- InitializationEventAttribute.cs
- Span.cs
- CompilerState.cs
- MyContact.cs
- WebControlParameterProxy.cs
- XmlToDatasetMap.cs
- ConnectionStringsSection.cs
- TimerElapsedEvenArgs.cs
- PackagePart.cs
- ObjectListField.cs
- ImageMapEventArgs.cs
- TypeSystem.cs
- SettingsSavedEventArgs.cs
- XmlNamespaceManager.cs
- WebPart.cs
- StorageMappingFragment.cs
- TextLine.cs
- ChannelCacheDefaults.cs
- ExtenderProvidedPropertyAttribute.cs
- util.cs