Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Dispatcher / ContentTypeSettingClientMessageFormatter.cs / 1 / ContentTypeSettingClientMessageFormatter.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System.Collections; using System.ServiceModel.Channels; using System.ServiceModel; using System.ServiceModel.Description; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Reflection; using System.Xml; using System.ServiceModel.Diagnostics; using System.Net; using System.ServiceModel.Dispatcher; using System.ServiceModel.Web; class ContentTypeSettingClientMessageFormatter : IClientMessageFormatter { IClientMessageFormatter innerFormatter; string outgoingContentType; public ContentTypeSettingClientMessageFormatter(string outgoingContentType, IClientMessageFormatter innerFormatter) { if (outgoingContentType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("outgoingContentType"); } if (innerFormatter == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("innerFormatter"); } this.outgoingContentType = outgoingContentType; this.innerFormatter = innerFormatter; } public object DeserializeReply(Message message, object[] parameters) { return this.innerFormatter.DeserializeReply(message, parameters); } public Message SerializeRequest(MessageVersion messageVersion, object[] parameters) { Message message = this.innerFormatter.SerializeRequest(messageVersion, parameters); if (message != null) { AddRequestContentTypeProperty(message, this.outgoingContentType); } return message; } static void AddRequestContentTypeProperty(Message message, string contentType) { if (message == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("message"); } if (contentType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("contentType"); } if (OperationContext.Current != null && OperationContext.Current.HasOutgoingMessageProperties) { if (string.IsNullOrEmpty(WebOperationContext.Current.OutgoingRequest.ContentType)) { WebOperationContext.Current.OutgoingRequest.ContentType = contentType; } } else { object prop; message.Properties.TryGetValue(HttpRequestMessageProperty.Name, out prop); HttpRequestMessageProperty httpProperty; if (prop != null) { httpProperty = (HttpRequestMessageProperty) prop; } else { httpProperty = new HttpRequestMessageProperty(); message.Properties.Add(HttpRequestMessageProperty.Name, httpProperty); } if (string.IsNullOrEmpty(httpProperty.Headers[HttpRequestHeader.ContentType])) { httpProperty.Headers[HttpRequestHeader.ContentType] = contentType; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
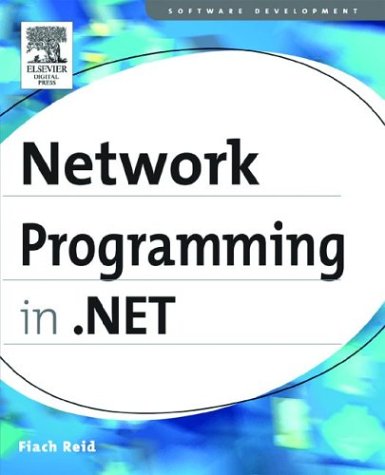
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LogSwitch.cs
- CookieProtection.cs
- DetailsViewDeleteEventArgs.cs
- Config.cs
- DeploymentSection.cs
- XDRSchema.cs
- ConcurrentStack.cs
- MergeFailedEvent.cs
- FontUnitConverter.cs
- DesignerWithHeader.cs
- ModelPerspective.cs
- FileNotFoundException.cs
- CalendarDay.cs
- Matrix.cs
- WebException.cs
- EntityTypeEmitter.cs
- UserNameSecurityTokenParameters.cs
- DbConnectionClosed.cs
- EncryptedPackageFilter.cs
- SafeJobHandle.cs
- RegexCharClass.cs
- NetDataContractSerializer.cs
- MeasureData.cs
- GZipStream.cs
- TypographyProperties.cs
- Graph.cs
- DragCompletedEventArgs.cs
- DbCommandTree.cs
- SimpleHandlerBuildProvider.cs
- MouseGestureValueSerializer.cs
- PopOutPanel.cs
- SortedDictionary.cs
- CommunicationException.cs
- ButtonFlatAdapter.cs
- Tile.cs
- NameValuePair.cs
- BufferedGraphicsManager.cs
- DeleteStoreRequest.cs
- ThreadSafeMessageFilterTable.cs
- ProofTokenCryptoHandle.cs
- CorrelationKey.cs
- TargetParameterCountException.cs
- SamlSubjectStatement.cs
- AppDomainResourcePerfCounters.cs
- FocusWithinProperty.cs
- DataGridParentRows.cs
- DataGridTable.cs
- CapabilitiesPattern.cs
- WebHttpSecurity.cs
- Type.cs
- PrintingPermissionAttribute.cs
- NameTable.cs
- RemotingConfiguration.cs
- EntityTypeEmitter.cs
- MethodInfo.cs
- SafeRightsManagementQueryHandle.cs
- PostBackOptions.cs
- SRGSCompiler.cs
- AnnotationMap.cs
- WmlFormAdapter.cs
- DiscreteKeyFrames.cs
- XmlCharType.cs
- DataSourceCache.cs
- ComboBoxRenderer.cs
- DataGridViewCellValueEventArgs.cs
- DateTime.cs
- StatusBarItemAutomationPeer.cs
- Tokenizer.cs
- ObjectDataSourceDisposingEventArgs.cs
- WSSecurityOneDotOneReceiveSecurityHeader.cs
- DataBoundControl.cs
- QueryException.cs
- PhonemeConverter.cs
- RecognizedPhrase.cs
- AmbientProperties.cs
- CodeTypeReference.cs
- KerberosSecurityTokenProvider.cs
- ClientBuildManager.cs
- ConfigurationLocationCollection.cs
- PrincipalPermission.cs
- WindowsAltTab.cs
- GenericWebPart.cs
- RecognizerInfo.cs
- XamlReader.cs
- HttpRuntimeSection.cs
- TabPanel.cs
- PrimitiveDataContract.cs
- IndentedWriter.cs
- ContentPresenter.cs
- ClientTarget.cs
- WindowsAltTab.cs
- ClientFormsAuthenticationMembershipProvider.cs
- EditorPartCollection.cs
- GridViewColumnHeaderAutomationPeer.cs
- SBCSCodePageEncoding.cs
- NumberSubstitution.cs
- ResponseBodyWriter.cs
- CategoryAttribute.cs
- QilStrConcat.cs
- WorkflowEventArgs.cs