Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / textformatting / NumberSubstitution.cs / 1 / NumberSubstitution.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: NumberSubstituion.cs // // Contents: Number substitution related types // // Spec: [....]/sites/Avalon/Specs/Cultural%20digit%20substitution.htm // // Created: 1-7-2005 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Globalization; using System.ComponentModel; using System.Windows; using MS.Internal.FontCache; // for HashFn using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; // Allow suppression of presharp warnings #pragma warning disable 1634, 1691 namespace System.Windows.Media { ////// The NumberSubstitution class specifies how numbers in text /// are to be displayed. /// public class NumberSubstitution { ////// Initializes a NumberSubstitution object with default values. /// public NumberSubstitution() { _source = NumberCultureSource.Text; _cultureOverride = null; _substitution = NumberSubstitutionMethod.AsCulture; } ////// Initializes a NumberSubstitution object with explicit values. /// /// Specifies how the number culture is determined. /// Number culture if NumberCultureSource.Override is specified. /// Type of number substitution to perform. public NumberSubstitution( NumberCultureSource source, CultureInfo cultureOverride, NumberSubstitutionMethod substitution) { _source = source; _cultureOverride = ThrowIfInvalidCultureOverride(cultureOverride); _substitution = substitution; } ////// The CultureSource property specifies how the culture for numbers /// is determined. The default value is NumberCultureSource.Text, /// which means the number culture is the culture of the text run. /// public NumberCultureSource CultureSource { get { return _source; } set { if ((uint)value > (uint)NumberCultureSource.Override) throw new InvalidEnumArgumentException("CultureSource", (int)value, typeof(NumberCultureSource)); _source = value; } } ////// If the CultureSource == NumberCultureSource.Override, this /// property specifies the number culture. A value of null is interpreted /// as US-English. The default value is null. If CultureSource != /// NumberCultureSource.Override, this property is ignored. /// [TypeConverter(typeof(System.Windows.CultureInfoIetfLanguageTagConverter))] public CultureInfo CultureOverride { get { return _cultureOverride; } set { _cultureOverride = ThrowIfInvalidCultureOverride(value); } } ////// Helper function to throw an exception if invalid value is specified for /// CultureOverride property. /// /// Culture to validate. ///The value of the culture parameter. private static CultureInfo ThrowIfInvalidCultureOverride(CultureInfo culture) { if (!IsValidCultureOverride(culture)) { throw new ArgumentException(SR.Get(SRID.SpecificNumberCultureRequired)); } return culture; } ////// Determines whether the specific culture is a valid value for the /// CultureOverride property. /// /// Culture to validate. ///Returns true if it's a valid CultureOverride, false if not. private static bool IsValidCultureOverride(CultureInfo culture) { // Null culture override is OK, but otherwise it must be a specific culture. return (culture == null) || !(culture.IsNeutralCulture || culture.Equals(CultureInfo.InvariantCulture)); } ////// Weakly typed validation callback for CultureOverride dependency property. /// /// CultureInfo object to validate; the type is assumed /// to be CultureInfo as the type is validated by the property engine. ///Returns true if it's a valid culture, false if not. private static bool IsValidCultureOverrideValue(object value) { return IsValidCultureOverride((CultureInfo)value); } ////// Specifies the type of number substitution to perform, if any. /// public NumberSubstitutionMethod Substitution { get { return _substitution; } set { if ((uint)value > (uint)NumberSubstitutionMethod.Traditional) throw new InvalidEnumArgumentException("Substitution", (int)value, typeof(NumberSubstitutionMethod)); _substitution = value; } } ////// DP For CultureSource /// public static readonly DependencyProperty CultureSourceProperty = DependencyProperty.RegisterAttached( "CultureSource", typeof(NumberCultureSource), typeof(NumberSubstitution)); ////// Setter for NumberSubstitution DependencyProperty /// public static void SetCultureSource(DependencyObject target, NumberCultureSource value) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CultureSourceProperty, value); } ////// Getter for NumberSubstitution DependencyProperty /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static NumberCultureSource GetCultureSource(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (NumberCultureSource)(target.GetValue(CultureSourceProperty)); } ////// DP For CultureOverride /// public static readonly DependencyProperty CultureOverrideProperty = DependencyProperty.RegisterAttached( "CultureOverride", typeof(CultureInfo), typeof(NumberSubstitution), null, // default property metadata new ValidateValueCallback(IsValidCultureOverrideValue) ); ////// Setter for NumberSubstitution DependencyProperty /// public static void SetCultureOverride(DependencyObject target, CultureInfo value) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CultureOverrideProperty, value); } ////// Getter for NumberSubstitution DependencyProperty /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] [TypeConverter(typeof(System.Windows.CultureInfoIetfLanguageTagConverter))] public static CultureInfo GetCultureOverride(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (CultureInfo)(target.GetValue(CultureOverrideProperty)); } ////// DP For Substitution /// public static readonly DependencyProperty SubstitutionProperty = DependencyProperty.RegisterAttached( "Substitution", typeof(NumberSubstitutionMethod), typeof(NumberSubstitution)); ////// Setter for NumberSubstitution DependencyProperty /// public static void SetSubstitution(DependencyObject target, NumberSubstitutionMethod value) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(SubstitutionProperty, value); } ////// Getter for NumberSubstitution DependencyProperty /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static NumberSubstitutionMethod GetSubstitution(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (NumberSubstitutionMethod)(target.GetValue(SubstitutionProperty)); } ////// Computes hash code for this object. /// ///A 32-bit signed integer hash code. public override int GetHashCode() { int hash = HashFn.HashMultiply((int)_source) + (int)_substitution; if (_cultureOverride != null) hash = HashFn.HashMultiply(hash) + _cultureOverride.GetHashCode(); return HashFn.HashScramble(hash); } ////// Checks whether this object is equal to another NumberSubstitution object. /// /// Object to compare with. ///Returns true if the specified object is a NumberSubstitution object with the /// same properties as this object, and false otherwise. public override bool Equals(object obj) { NumberSubstitution sub = obj as NumberSubstitution; // Suppress PRESharp warning that sub can be null; apparently PRESharp // doesn't understand short circuit evaluation of operator &&. #pragma warning disable 6506 return sub != null && _source == sub._source && _substitution == sub._substitution && (_cultureOverride == null ? (sub._cultureOverride == null) : (_cultureOverride.Equals(sub._cultureOverride))); #pragma warning restore 6506 } private NumberCultureSource _source; private CultureInfo _cultureOverride; private NumberSubstitutionMethod _substitution; } ////// Used with the NumberSubstitution class, specifies how the culture for /// numbers in a text run is determined. /// public enum NumberCultureSource { ////// Number culture is TextRunProperties.CultureInfo, i.e., the culture /// of the text run. In markup, this is the xml:lang attribute. /// Text = 0, ////// Number culture is the culture of the current thread, which by default /// is the user default culture. /// User = 1, ////// Number culture is NumberSubstitution.CultureOverride. /// Override = 2 } ////// Value of the NumberSubstitution.Substitituion property, specifies /// the type of number substitution to perform, if any. /// public enum NumberSubstitutionMethod { ////// Specifies that the substitution method should be determined based /// on the number culture's NumberFormat.DigitSubstitution property. /// This is the default value. /// AsCulture = 0, ////// If the number culture is an Arabic or Farsi culture, specifies that /// the digits depend on the context. Either traditional or Latin digits /// are used depending on the nearest preceding strong character or (if /// there is none) the text direction of the paragraph. /// Context = 1, ////// Specifies that code points 0x30-0x39 are always rendered as European /// digits, i.e., no substitution is performed. /// European = 2, ////// Specifies that numbers are rendered using the national digits for /// the number culture, as specified by the culture's NumberFormat /// property. /// NativeNational = 3, ////// Specifies that numbers are rendered using the traditional digits /// for the number culture. For most cultures, this is the same as /// NativeNational. However, NativeNational results in Latin digits /// for some Arabic cultures, whereas this value results in Arabic /// digits for all Arabic cultures. /// Traditional = 4 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
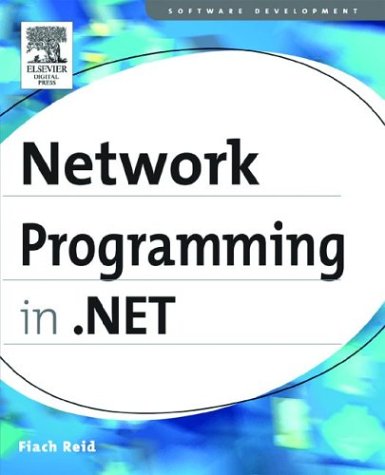
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AddInPipelineAttributes.cs
- XhtmlBasicValidationSummaryAdapter.cs
- ComponentResourceManager.cs
- Int16Converter.cs
- Parsers.cs
- XPathParser.cs
- SchemaImporter.cs
- PermissionRequestEvidence.cs
- TransactionChannel.cs
- GestureRecognitionResult.cs
- AllMembershipCondition.cs
- AutomationPropertyInfo.cs
- SoapTypeAttribute.cs
- VisualStyleInformation.cs
- xmlsaver.cs
- StylusButton.cs
- XmlNullResolver.cs
- IDispatchConstantAttribute.cs
- QuestionEventArgs.cs
- Base64Encoder.cs
- FixedTextSelectionProcessor.cs
- ListViewSortEventArgs.cs
- AssemblyNameProxy.cs
- FilterQuery.cs
- ProcessInputEventArgs.cs
- DummyDataSource.cs
- ListChunk.cs
- AliasedSlot.cs
- BasicKeyConstraint.cs
- ServicePoint.cs
- ViewGenerator.cs
- BasePattern.cs
- NumberFormatter.cs
- IsolatedStoragePermission.cs
- GridErrorDlg.cs
- ControlCachePolicy.cs
- TextSelectionHelper.cs
- MessageSmuggler.cs
- sqlmetadatafactory.cs
- DocumentViewerAutomationPeer.cs
- CapacityStreamGeometryContext.cs
- TransportBindingElement.cs
- EdmFunction.cs
- TextRange.cs
- ParseElement.cs
- InkPresenterAutomationPeer.cs
- DataPointer.cs
- DataSourceHelper.cs
- PanelStyle.cs
- AppSettingsSection.cs
- UrlAuthFailedErrorFormatter.cs
- BinaryParser.cs
- StreamingContext.cs
- ResourceBinder.cs
- MSAAEventDispatcher.cs
- XmlText.cs
- StrokeNodeEnumerator.cs
- TypeRefElement.cs
- RuleEngine.cs
- DocumentApplication.cs
- ThousandthOfEmRealDoubles.cs
- LineSegment.cs
- ListControlConvertEventArgs.cs
- HttpGetServerProtocol.cs
- OleDbMetaDataFactory.cs
- XmlFormatWriterGenerator.cs
- TrackingParticipant.cs
- CodeThrowExceptionStatement.cs
- ObjectRef.cs
- SimpleTextLine.cs
- XmlSchemaException.cs
- ZipIOExtraField.cs
- ObjectPersistData.cs
- SessionStateModule.cs
- DbDataRecord.cs
- FontFaceLayoutInfo.cs
- X509Certificate.cs
- SharedConnectionListener.cs
- Rotation3D.cs
- querybuilder.cs
- DesignerHost.cs
- ToolStripItemCollection.cs
- DataGridLinkButton.cs
- ConfigurationException.cs
- XmlComment.cs
- SizeF.cs
- XmlSchemaAttribute.cs
- WebPartDisplayModeCancelEventArgs.cs
- XmlName.cs
- ConfigurationElement.cs
- MulticastNotSupportedException.cs
- DbCommandDefinition.cs
- TextEditorContextMenu.cs
- MaskedTextBoxTextEditor.cs
- ChtmlCommandAdapter.cs
- DetailsViewModeEventArgs.cs
- XmlSerializableReader.cs
- XmlParserContext.cs
- FontNameConverter.cs
- WebPartMovingEventArgs.cs