Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / PropertyManager.cs / 1 / PropertyManager.cs
namespace System.Windows.Forms { using System; using System.Windows.Forms; using System.Diagnostics.CodeAnalysis; using System.ComponentModel; using System.Collections; ////// /// public class PropertyManager : BindingManagerBase { // PropertyManager class // private object dataSource; private string propName; private PropertyDescriptor propInfo; private bool bound; ///[To be supplied.] ////// /// public override Object Current { get { return this.dataSource; } } private void PropertyChanged(object sender, EventArgs ea) { EndCurrentEdit(); OnCurrentChanged(EventArgs.Empty); } internal override void SetDataSource(Object dataSource) { if (this.dataSource != null && !String.IsNullOrEmpty(this.propName)) { propInfo.RemoveValueChanged(this.dataSource, new EventHandler(PropertyChanged)); propInfo = null; } this.dataSource = dataSource; if (this.dataSource != null && !String.IsNullOrEmpty(this.propName)) { propInfo = TypeDescriptor.GetProperties(dataSource).Find(propName, true); if (propInfo == null) throw new ArgumentException(SR.GetString(SR.PropertyManagerPropDoesNotExist, propName, dataSource.ToString())); propInfo.AddValueChanged(dataSource, new EventHandler(PropertyChanged)); } } ///[To be supplied.] ////// /// public PropertyManager() {} [ SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors") // If the constructor does not set the dataSource // it would be a breaking change. ] internal PropertyManager(Object dataSource) : base(dataSource){} [ SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors") // If the constructor does not set the dataSource // it would be a breaking change. ] internal PropertyManager(Object dataSource, string propName) : base() { this.propName = propName; this.SetDataSource(dataSource); } internal override PropertyDescriptorCollection GetItemProperties(PropertyDescriptor[] listAccessors) { return ListBindingHelper.GetListItemProperties(dataSource, listAccessors); } internal override Type BindType { get { return dataSource.GetType(); } } internal override String GetListName() { return TypeDescriptor.GetClassName(dataSource) + "." + propName; } ///[To be supplied.] ///public override void SuspendBinding() { EndCurrentEdit(); if (bound) { try { bound = false; UpdateIsBinding(); } catch { bound = true; UpdateIsBinding(); throw; } } } /// public override void ResumeBinding() { OnCurrentChanged(new EventArgs()); if (!bound) { try { bound = true; UpdateIsBinding(); } catch { bound = false; UpdateIsBinding(); throw; } } } /// /// /// protected internal override String GetListName(ArrayList listAccessors) { return ""; } ///[To be supplied.] ////// /// public override void CancelCurrentEdit() { IEditableObject obj = this.Current as IEditableObject; if (obj != null) obj.CancelEdit(); PushData(); } ///[To be supplied.] ////// /// public override void EndCurrentEdit() { bool success; PullData(out success); if (success) { IEditableObject obj = this.Current as IEditableObject; if (obj != null) obj.EndEdit(); } } ///[To be supplied.] ////// /// protected override void UpdateIsBinding() { for (int i = 0; i < this.Bindings.Count; i++) this.Bindings[i].UpdateIsBinding(); } ///[To be supplied.] ////// /// internal protected override void OnCurrentChanged(EventArgs ea) { PushData(); if (this.onCurrentChangedHandler != null) this.onCurrentChangedHandler(this, ea); if (this.onCurrentItemChangedHandler != null) this.onCurrentItemChangedHandler(this, ea); } ///[To be supplied.] ////// /// internal protected override void OnCurrentItemChanged(EventArgs ea) { PushData(); if (this.onCurrentItemChangedHandler != null) this.onCurrentItemChangedHandler(this, ea); } internal override object DataSource { get { return this.dataSource; } } internal override bool IsBinding { get { return (dataSource != null); } } // no op on the propertyManager ///[To be supplied.] ////// /// public override int Position { get { return 0; } set { } } ///[To be supplied.] ///public override int Count { get { return 1; } } // no-op on the propertyManager /// /// /// public override void AddNew() { throw new NotSupportedException(SR.GetString(SR.DataBindingAddNewNotSupportedOnPropertyManager)); } // no-op on the propertyManager ///[To be supplied.] ////// /// public override void RemoveAt(int index) { throw new NotSupportedException(SR.GetString(SR.DataBindingRemoveAtNotSupportedOnPropertyManager)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Windows.Forms { using System; using System.Windows.Forms; using System.Diagnostics.CodeAnalysis; using System.ComponentModel; using System.Collections; ///[To be supplied.] ////// /// public class PropertyManager : BindingManagerBase { // PropertyManager class // private object dataSource; private string propName; private PropertyDescriptor propInfo; private bool bound; ///[To be supplied.] ////// /// public override Object Current { get { return this.dataSource; } } private void PropertyChanged(object sender, EventArgs ea) { EndCurrentEdit(); OnCurrentChanged(EventArgs.Empty); } internal override void SetDataSource(Object dataSource) { if (this.dataSource != null && !String.IsNullOrEmpty(this.propName)) { propInfo.RemoveValueChanged(this.dataSource, new EventHandler(PropertyChanged)); propInfo = null; } this.dataSource = dataSource; if (this.dataSource != null && !String.IsNullOrEmpty(this.propName)) { propInfo = TypeDescriptor.GetProperties(dataSource).Find(propName, true); if (propInfo == null) throw new ArgumentException(SR.GetString(SR.PropertyManagerPropDoesNotExist, propName, dataSource.ToString())); propInfo.AddValueChanged(dataSource, new EventHandler(PropertyChanged)); } } ///[To be supplied.] ////// /// public PropertyManager() {} [ SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors") // If the constructor does not set the dataSource // it would be a breaking change. ] internal PropertyManager(Object dataSource) : base(dataSource){} [ SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors") // If the constructor does not set the dataSource // it would be a breaking change. ] internal PropertyManager(Object dataSource, string propName) : base() { this.propName = propName; this.SetDataSource(dataSource); } internal override PropertyDescriptorCollection GetItemProperties(PropertyDescriptor[] listAccessors) { return ListBindingHelper.GetListItemProperties(dataSource, listAccessors); } internal override Type BindType { get { return dataSource.GetType(); } } internal override String GetListName() { return TypeDescriptor.GetClassName(dataSource) + "." + propName; } ///[To be supplied.] ///public override void SuspendBinding() { EndCurrentEdit(); if (bound) { try { bound = false; UpdateIsBinding(); } catch { bound = true; UpdateIsBinding(); throw; } } } /// public override void ResumeBinding() { OnCurrentChanged(new EventArgs()); if (!bound) { try { bound = true; UpdateIsBinding(); } catch { bound = false; UpdateIsBinding(); throw; } } } /// /// /// protected internal override String GetListName(ArrayList listAccessors) { return ""; } ///[To be supplied.] ////// /// public override void CancelCurrentEdit() { IEditableObject obj = this.Current as IEditableObject; if (obj != null) obj.CancelEdit(); PushData(); } ///[To be supplied.] ////// /// public override void EndCurrentEdit() { bool success; PullData(out success); if (success) { IEditableObject obj = this.Current as IEditableObject; if (obj != null) obj.EndEdit(); } } ///[To be supplied.] ////// /// protected override void UpdateIsBinding() { for (int i = 0; i < this.Bindings.Count; i++) this.Bindings[i].UpdateIsBinding(); } ///[To be supplied.] ////// /// internal protected override void OnCurrentChanged(EventArgs ea) { PushData(); if (this.onCurrentChangedHandler != null) this.onCurrentChangedHandler(this, ea); if (this.onCurrentItemChangedHandler != null) this.onCurrentItemChangedHandler(this, ea); } ///[To be supplied.] ////// /// internal protected override void OnCurrentItemChanged(EventArgs ea) { PushData(); if (this.onCurrentItemChangedHandler != null) this.onCurrentItemChangedHandler(this, ea); } internal override object DataSource { get { return this.dataSource; } } internal override bool IsBinding { get { return (dataSource != null); } } // no op on the propertyManager ///[To be supplied.] ////// /// public override int Position { get { return 0; } set { } } ///[To be supplied.] ///public override int Count { get { return 1; } } // no-op on the propertyManager /// /// /// public override void AddNew() { throw new NotSupportedException(SR.GetString(SR.DataBindingAddNewNotSupportedOnPropertyManager)); } // no-op on the propertyManager ///[To be supplied.] ////// /// public override void RemoveAt(int index) { throw new NotSupportedException(SR.GetString(SR.DataBindingRemoveAtNotSupportedOnPropertyManager)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
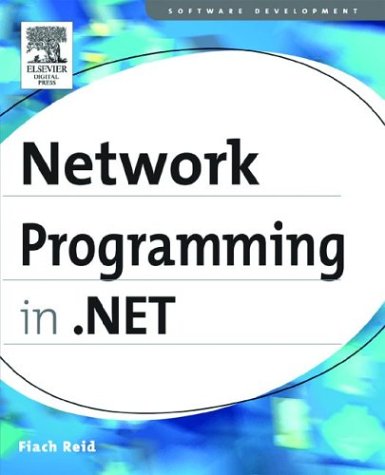
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CommandSet.cs
- DbFunctionCommandTree.cs
- SubqueryRules.cs
- ConfigurationValidatorBase.cs
- FacetChecker.cs
- StoreContentChangedEventArgs.cs
- ToolstripProfessionalRenderer.cs
- RangeBase.cs
- DataBoundControl.cs
- SqlInternalConnectionTds.cs
- DataGridViewCheckBoxColumn.cs
- _SslState.cs
- RepeaterDesigner.cs
- EventLogPermission.cs
- ZipIOFileItemStream.cs
- CodeSnippetCompileUnit.cs
- DesignerCommandAdapter.cs
- QilExpression.cs
- WaitHandleCannotBeOpenedException.cs
- XmlPreloadedResolver.cs
- ServerIdentity.cs
- ToolStripPanelRow.cs
- messageonlyhwndwrapper.cs
- FormsIdentity.cs
- InternalEnumValidatorAttribute.cs
- ListView.cs
- InkCanvasSelection.cs
- InsufficientMemoryException.cs
- XslCompiledTransform.cs
- XmlSchemaComplexType.cs
- ControlHelper.cs
- BindingCompleteEventArgs.cs
- ProxyOperationRuntime.cs
- TextServicesLoader.cs
- ListDependantCardsRequest.cs
- TableCellCollection.cs
- IgnoreSection.cs
- XPathDocumentIterator.cs
- DynamicEndpoint.cs
- ContextQuery.cs
- HyperLinkField.cs
- XslAstAnalyzer.cs
- _NTAuthentication.cs
- TextFormatter.cs
- ComplexPropertyEntry.cs
- CustomAttribute.cs
- ProfilePropertySettingsCollection.cs
- ResXBuildProvider.cs
- EventLogPermissionEntry.cs
- CharAnimationUsingKeyFrames.cs
- CodeGen.cs
- StylusButton.cs
- VariableQuery.cs
- IntSecurity.cs
- ValueUnavailableException.cs
- QilStrConcatenator.cs
- CategoryNameCollection.cs
- ImageCodecInfoPrivate.cs
- TraceSource.cs
- SecurityState.cs
- EntityClassGenerator.cs
- WorkflowOperationContext.cs
- ObjectNotFoundException.cs
- SupportedAddressingMode.cs
- QueryStringParameter.cs
- AssociationEndMember.cs
- AsymmetricKeyExchangeFormatter.cs
- TdsEnums.cs
- StandardCommandToolStripMenuItem.cs
- OutputScope.cs
- EdgeProfileValidation.cs
- TabRenderer.cs
- CodeAttributeDeclaration.cs
- Pair.cs
- TypeInfo.cs
- NonParentingControl.cs
- ConvertersCollection.cs
- Single.cs
- SchemaElementLookUpTable.cs
- SerializationInfoEnumerator.cs
- XmlEntityReference.cs
- TreeView.cs
- HttpListener.cs
- PropertySourceInfo.cs
- ViewGenerator.cs
- DataGridViewElement.cs
- MessageSmuggler.cs
- HttpClientCertificate.cs
- X509AudioLogo.cs
- MultipleFilterMatchesException.cs
- Send.cs
- UnlockCardRequest.cs
- FileDialog.cs
- QuaternionRotation3D.cs
- HtmlShimManager.cs
- SqlFunctionAttribute.cs
- ConfigurationElementCollection.cs
- SymbolPair.cs
- AsyncDataRequest.cs
- SplitterEvent.cs