Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / Sql / SqlFunctionAttribute.cs / 1 / SqlFunctionAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //[....] //[....] //daltudov //[....] //beysims //[....] //vadimt //----------------------------------------------------------------------------- using System; namespace Microsoft.SqlServer.Server { [Serializable] public enum DataAccessKind { None = 0, Read = 1, } [Serializable] public enum SystemDataAccessKind { None = 0, Read = 1, } // sql specific attribute [AttributeUsage(AttributeTargets.Method, AllowMultiple = false, Inherited = false), Serializable] public class SqlFunctionAttribute : System.Attribute { private bool m_fDeterministic; private DataAccessKind m_eDataAccess; private SystemDataAccessKind m_eSystemDataAccess; private bool m_fPrecise; private string m_fName; private string m_fTableDefinition; private string m_FillRowMethodName; public SqlFunctionAttribute() { // default values m_fDeterministic = false; m_eDataAccess = DataAccessKind.None; m_eSystemDataAccess = SystemDataAccessKind.None; m_fPrecise = false; m_fName = null; m_fTableDefinition = null; m_FillRowMethodName = null; } // SqlFunctionAttribute public bool IsDeterministic { get { return m_fDeterministic; } set { m_fDeterministic = value; } } // Deterministic public DataAccessKind DataAccess { get { return m_eDataAccess; } set { m_eDataAccess = value; } } // public bool DataAccessKind public SystemDataAccessKind SystemDataAccess { get { return m_eSystemDataAccess; } set { m_eSystemDataAccess = value; } } // public bool SystemDataAccessKind public bool IsPrecise { get { return m_fPrecise; } set { m_fPrecise = value; } } // Precise public string Name { get { return m_fName; } set { m_fName = value; } } public string TableDefinition { get { return m_fTableDefinition; } set { m_fTableDefinition = value; } } public string FillRowMethodName { get { return m_FillRowMethodName; } set { m_FillRowMethodName = value; } } } // class SqlFunctionAttribute } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
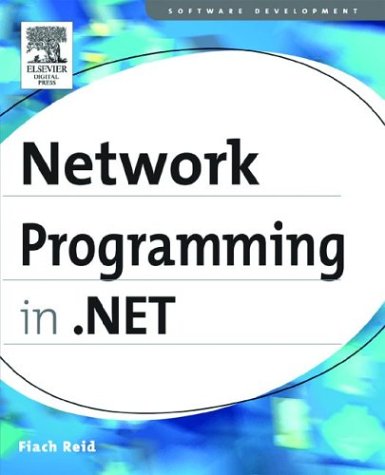
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReaderOutput.cs
- GroupQuery.cs
- _UriTypeConverter.cs
- TextParaLineResult.cs
- SQLInt32.cs
- WebRequest.cs
- SQLInt32Storage.cs
- DataKey.cs
- StructuralCache.cs
- XNameConverter.cs
- SafeRightsManagementSessionHandle.cs
- DataControlButton.cs
- BypassElementCollection.cs
- HttpAsyncResult.cs
- AnimationLayer.cs
- Label.cs
- XmlSchemaSubstitutionGroup.cs
- ExtensionQuery.cs
- SessionStateContainer.cs
- CompositeControl.cs
- ExtractCollection.cs
- MailDefinition.cs
- GenericPrincipal.cs
- InstanceLockedException.cs
- BamlVersionHeader.cs
- Sql8ConformanceChecker.cs
- KeyManager.cs
- RelationshipConstraintValidator.cs
- NullableFloatMinMaxAggregationOperator.cs
- Panel.cs
- ILGenerator.cs
- PreviousTrackingServiceAttribute.cs
- SimpleExpression.cs
- KnownAssembliesSet.cs
- ValuePatternIdentifiers.cs
- HotSpot.cs
- SqlDataAdapter.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- FontUnit.cs
- Parsers.cs
- SqlClientFactory.cs
- DataControlFieldHeaderCell.cs
- SettingsBindableAttribute.cs
- HttpStaticObjectsCollectionBase.cs
- ListViewContainer.cs
- SelectionChangedEventArgs.cs
- TimeoutConverter.cs
- BitmapMetadataBlob.cs
- SchemaEntity.cs
- OdbcErrorCollection.cs
- ArgumentException.cs
- InvalidComObjectException.cs
- AutomationProperties.cs
- XsdDateTime.cs
- Column.cs
- Update.cs
- BCLDebug.cs
- elementinformation.cs
- PageParserFilter.cs
- PaginationProgressEventArgs.cs
- AndCondition.cs
- AssemblyBuilder.cs
- PtsContext.cs
- SectionRecord.cs
- HandlerFactoryCache.cs
- CheckBoxStandardAdapter.cs
- AssemblyName.cs
- ManagementDateTime.cs
- PaintValueEventArgs.cs
- Form.cs
- WebControlParameterProxy.cs
- FtpWebRequest.cs
- ControlParameter.cs
- VideoDrawing.cs
- WebConfigManager.cs
- EventLogReader.cs
- SelectManyQueryOperator.cs
- XmlSchemaObject.cs
- SoapFault.cs
- PauseStoryboard.cs
- SQLUtility.cs
- IntPtr.cs
- OracleConnectionStringBuilder.cs
- LoginName.cs
- HtmlTableCellCollection.cs
- EntityConnectionStringBuilder.cs
- Win32Exception.cs
- Camera.cs
- VectorAnimationUsingKeyFrames.cs
- Keyboard.cs
- Int32CollectionValueSerializer.cs
- ReadOnlyHierarchicalDataSource.cs
- PageThemeCodeDomTreeGenerator.cs
- LoadGrammarCompletedEventArgs.cs
- TraceContextRecord.cs
- CanExecuteRoutedEventArgs.cs
- DataGridTablesFactory.cs
- NavigationWindowAutomationPeer.cs
- PageBorderless.cs
- TypeConverters.cs