Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / Diagnostics / TextWriterTraceListener.cs / 1 / TextWriterTraceListener.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Diagnostics { using System; using System.IO; using System.Text; using System.Security.Permissions; using System.Runtime.InteropServices; using System.IO.Ports; using Microsoft.Win32; using System.Runtime.Versioning; ////// [HostProtection(Synchronization=true)] public class TextWriterTraceListener : TraceListener { internal TextWriter writer; String fileName = null; ///Directs tracing or debugging output to /// a ///or to a , /// such as or . /// public TextWriterTraceListener() { } ///Initializes a new instance of the ///class with /// /// as the output recipient. /// public TextWriterTraceListener(Stream stream) : this(stream, string.Empty) { } ///Initializes a new instance of the ///class, using the /// stream as the recipient of the debugging and tracing output. /// public TextWriterTraceListener(Stream stream, string name) : base(name) { if (stream == null) throw new ArgumentNullException("stream"); this.writer = new StreamWriter(stream); } ///Initializes a new instance of the ///class with the /// specified name and using the stream as the recipient of the debugging and tracing output. /// public TextWriterTraceListener(TextWriter writer) : this(writer, string.Empty) { } ///Initializes a new instance of the ///class using the /// specified writer as recipient of the tracing or debugging output. /// public TextWriterTraceListener(TextWriter writer, string name) : base(name) { if (writer == null) throw new ArgumentNullException("writer"); this.writer = writer; } ///Initializes a new instance of the ///class with the /// specified name and using the specified writer as recipient of the tracing or /// debugging /// output. /// [ResourceExposure(ResourceScope.Machine)] public TextWriterTraceListener(string fileName) { this.fileName = fileName; } ///[To be supplied.] ////// [ResourceExposure(ResourceScope.Machine)] public TextWriterTraceListener(string fileName, string name) : base(name) { this.fileName = fileName; } ///[To be supplied.] ////// public TextWriter Writer { get { EnsureWriter(); return writer; } set { writer = value; } } ///Indicates the text writer that receives the tracing /// or debugging output. ////// public override void Close() { if (writer != null) writer.Close(); writer = null; } ///Closes the ///so that it no longer /// receives tracing or debugging output. /// /// protected override void Dispose(bool disposing) { if (disposing) this.Close(); } ////// public override void Flush() { if (!EnsureWriter()) return; writer.Flush(); } ///Flushes the output buffer for the ///. /// public override void Write(string message) { if (!EnsureWriter()) return; if (NeedIndent) WriteIndent(); writer.Write(message); } ///Writes a message /// to this instance's ///. /// public override void WriteLine(string message) { if (!EnsureWriter()) return; if (NeedIndent) WriteIndent(); writer.WriteLine(message); NeedIndent = true; } private static Encoding GetEncodingWithFallback(Encoding encoding) { // Clone it and set the "?" replacement fallback Encoding fallbackEncoding = (Encoding)encoding.Clone(); fallbackEncoding.EncoderFallback = EncoderFallback.ReplacementFallback; fallbackEncoding.DecoderFallback = DecoderFallback.ReplacementFallback; return fallbackEncoding; } // This uses a machine resource, scoped by the fileName variable. [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] internal bool EnsureWriter() { bool ret = true; if (writer == null) { ret = false; if (fileName == null) return ret; // StreamWriter by default uses UTF8Encoding which will throw on invalid encoding errors. // This can cause the internal StreamWriter's state to be irrecoverable. It is bad for tracing // APIs to throw on encoding errors. Instead, we should provide a "?" replacement fallback // encoding to substitute illegal chars. For ex, In case of high surrogate character // D800-DBFF without a following low surrogate character DC00-DFFF // NOTE: We also need to use an encoding that does't emit BOM whic is StreamWriter's default Encoding noBOMwithFallback = GetEncodingWithFallback(new UTF8Encoding(false)); // To support multiple appdomains/instances tracing to the same file, // we will try to open the given file for append but if we encounter // IO errors, we will prefix the file name with a unique GUID value // and try one more time string fullPath = Path.GetFullPath(fileName); string dirPath = Path.GetDirectoryName(fullPath); string fileNameOnly = Path.GetFileName(fullPath); for (int i=0; i<2; i++) { try { writer = new StreamWriter(fullPath, true, noBOMwithFallback, 4096); ret = true; break; } catch (IOException ) { // Should we do this only for ERROR_SHARING_VIOLATION? //if (InternalResources.MakeErrorCodeFromHR(Marshal.GetHRForException(ioexc)) == InternalResources.ERROR_SHARING_VIOLATION) { fileNameOnly = Guid.NewGuid().ToString() + fileNameOnly; fullPath = Path.Combine(dirPath, fileNameOnly); continue; } catch (UnauthorizedAccessException ) { //ERROR_ACCESS_DENIED, mostly ACL issues break; } catch (Exception ) { break; } } if (!ret) { // Disable tracing to this listener. Every Write will be nop. // We need to think of a central way to deal with the listener // init errors in the future. The default should be that we eat // up any errors from listener and optionally notify the user fileName = null; } } return ret; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Writes a message /// to this instance's ///followed by a line terminator. The /// default line terminator is a carriage return followed by a line feed (\r\n). // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Diagnostics { using System; using System.IO; using System.Text; using System.Security.Permissions; using System.Runtime.InteropServices; using System.IO.Ports; using Microsoft.Win32; using System.Runtime.Versioning; ////// [HostProtection(Synchronization=true)] public class TextWriterTraceListener : TraceListener { internal TextWriter writer; String fileName = null; ///Directs tracing or debugging output to /// a ///or to a , /// such as or . /// public TextWriterTraceListener() { } ///Initializes a new instance of the ///class with /// /// as the output recipient. /// public TextWriterTraceListener(Stream stream) : this(stream, string.Empty) { } ///Initializes a new instance of the ///class, using the /// stream as the recipient of the debugging and tracing output. /// public TextWriterTraceListener(Stream stream, string name) : base(name) { if (stream == null) throw new ArgumentNullException("stream"); this.writer = new StreamWriter(stream); } ///Initializes a new instance of the ///class with the /// specified name and using the stream as the recipient of the debugging and tracing output. /// public TextWriterTraceListener(TextWriter writer) : this(writer, string.Empty) { } ///Initializes a new instance of the ///class using the /// specified writer as recipient of the tracing or debugging output. /// public TextWriterTraceListener(TextWriter writer, string name) : base(name) { if (writer == null) throw new ArgumentNullException("writer"); this.writer = writer; } ///Initializes a new instance of the ///class with the /// specified name and using the specified writer as recipient of the tracing or /// debugging /// output. /// [ResourceExposure(ResourceScope.Machine)] public TextWriterTraceListener(string fileName) { this.fileName = fileName; } ///[To be supplied.] ////// [ResourceExposure(ResourceScope.Machine)] public TextWriterTraceListener(string fileName, string name) : base(name) { this.fileName = fileName; } ///[To be supplied.] ////// public TextWriter Writer { get { EnsureWriter(); return writer; } set { writer = value; } } ///Indicates the text writer that receives the tracing /// or debugging output. ////// public override void Close() { if (writer != null) writer.Close(); writer = null; } ///Closes the ///so that it no longer /// receives tracing or debugging output. /// /// protected override void Dispose(bool disposing) { if (disposing) this.Close(); } ////// public override void Flush() { if (!EnsureWriter()) return; writer.Flush(); } ///Flushes the output buffer for the ///. /// public override void Write(string message) { if (!EnsureWriter()) return; if (NeedIndent) WriteIndent(); writer.Write(message); } ///Writes a message /// to this instance's ///. /// public override void WriteLine(string message) { if (!EnsureWriter()) return; if (NeedIndent) WriteIndent(); writer.WriteLine(message); NeedIndent = true; } private static Encoding GetEncodingWithFallback(Encoding encoding) { // Clone it and set the "?" replacement fallback Encoding fallbackEncoding = (Encoding)encoding.Clone(); fallbackEncoding.EncoderFallback = EncoderFallback.ReplacementFallback; fallbackEncoding.DecoderFallback = DecoderFallback.ReplacementFallback; return fallbackEncoding; } // This uses a machine resource, scoped by the fileName variable. [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] internal bool EnsureWriter() { bool ret = true; if (writer == null) { ret = false; if (fileName == null) return ret; // StreamWriter by default uses UTF8Encoding which will throw on invalid encoding errors. // This can cause the internal StreamWriter's state to be irrecoverable. It is bad for tracing // APIs to throw on encoding errors. Instead, we should provide a "?" replacement fallback // encoding to substitute illegal chars. For ex, In case of high surrogate character // D800-DBFF without a following low surrogate character DC00-DFFF // NOTE: We also need to use an encoding that does't emit BOM whic is StreamWriter's default Encoding noBOMwithFallback = GetEncodingWithFallback(new UTF8Encoding(false)); // To support multiple appdomains/instances tracing to the same file, // we will try to open the given file for append but if we encounter // IO errors, we will prefix the file name with a unique GUID value // and try one more time string fullPath = Path.GetFullPath(fileName); string dirPath = Path.GetDirectoryName(fullPath); string fileNameOnly = Path.GetFileName(fullPath); for (int i=0; i<2; i++) { try { writer = new StreamWriter(fullPath, true, noBOMwithFallback, 4096); ret = true; break; } catch (IOException ) { // Should we do this only for ERROR_SHARING_VIOLATION? //if (InternalResources.MakeErrorCodeFromHR(Marshal.GetHRForException(ioexc)) == InternalResources.ERROR_SHARING_VIOLATION) { fileNameOnly = Guid.NewGuid().ToString() + fileNameOnly; fullPath = Path.Combine(dirPath, fileNameOnly); continue; } catch (UnauthorizedAccessException ) { //ERROR_ACCESS_DENIED, mostly ACL issues break; } catch (Exception ) { break; } } if (!ret) { // Disable tracing to this listener. Every Write will be nop. // We need to think of a central way to deal with the listener // init errors in the future. The default should be that we eat // up any errors from listener and optionally notify the user fileName = null; } } return ret; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Writes a message /// to this instance's ///followed by a line terminator. The /// default line terminator is a carriage return followed by a line feed (\r\n).
Link Menu
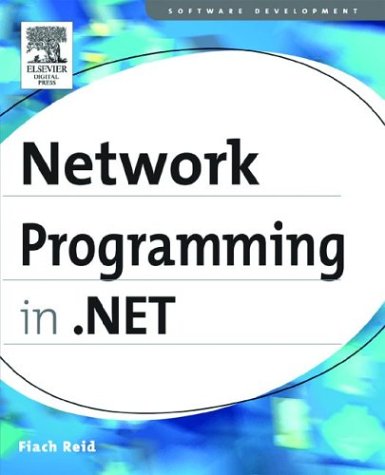
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProcessHost.cs
- RefreshPropertiesAttribute.cs
- CacheDependency.cs
- HtmlInputImage.cs
- ItemList.cs
- TdsParserStateObject.cs
- codemethodreferenceexpression.cs
- AsyncOperation.cs
- LowerCaseStringConverter.cs
- HtmlFormWrapper.cs
- OracleCommand.cs
- Menu.cs
- SplashScreen.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- TextContainerHelper.cs
- ClientType.cs
- SqlMultiplexer.cs
- DbDeleteCommandTree.cs
- ConfigUtil.cs
- Function.cs
- List.cs
- rsa.cs
- WindowsStartMenu.cs
- MessageQueueCriteria.cs
- SmiContext.cs
- IPAddressCollection.cs
- VirtualizedItemPattern.cs
- ListControl.cs
- InstanceDataCollection.cs
- CodePageUtils.cs
- MenuItemCollection.cs
- Condition.cs
- ConnectionPoint.cs
- StoryFragments.cs
- ToolStripRenderEventArgs.cs
- QueryOperator.cs
- IDataContractSurrogate.cs
- NameValuePermission.cs
- SystemColors.cs
- AutomationPropertyInfo.cs
- XPathNodeInfoAtom.cs
- Viewport3DVisual.cs
- NeutralResourcesLanguageAttribute.cs
- SiteMapProvider.cs
- RegistrationProxy.cs
- AppliedDeviceFiltersDialog.cs
- WindowsNonControl.cs
- XsltOutput.cs
- CodeExporter.cs
- ControlCachePolicy.cs
- LinkArea.cs
- SspiSafeHandles.cs
- ImageBrush.cs
- Operators.cs
- TargetConverter.cs
- TemplateBaseAction.cs
- CodePageEncoding.cs
- RawStylusInput.cs
- RotateTransform.cs
- UrlPath.cs
- WizardPanel.cs
- ParameterReplacerVisitor.cs
- ZipIOExtraFieldElement.cs
- DrawingAttributes.cs
- XmlCollation.cs
- TypeBrowser.xaml.cs
- Roles.cs
- TextBoxBase.cs
- DataGridLinkButton.cs
- FunctionDescription.cs
- AssemblyName.cs
- CommandValueSerializer.cs
- WindowsListBox.cs
- ZipIOModeEnforcingStream.cs
- UICuesEvent.cs
- Setter.cs
- KerberosTicketHashIdentifierClause.cs
- DataGridPageChangedEventArgs.cs
- UMPAttributes.cs
- TreeViewImageKeyConverter.cs
- ListenerConnectionDemuxer.cs
- ReadOnlyAttribute.cs
- SoapDocumentMethodAttribute.cs
- WriterOutput.cs
- PluggableProtocol.cs
- AlphabeticalEnumConverter.cs
- ProviderBase.cs
- PathFigure.cs
- ResumeStoryboard.cs
- DoubleAnimationClockResource.cs
- MetadataItemEmitter.cs
- HandleExceptionArgs.cs
- XmlSchemaGroupRef.cs
- CompatibleIComparer.cs
- ClientApiGenerator.cs
- DisposableCollectionWrapper.cs
- PlaceHolder.cs
- BamlLocalizationDictionary.cs
- WMICapabilities.cs
- regiisutil.cs