Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Shared / MS / Utility / Trace.cs / 3 / Trace.cs
/****************************************************************************\ * * File: Trace.cs * * Description: * Implements ETW tracing for Avalon Managed Code * * History: * 7/10/2002: Curtis Dutton: Created * * Copyright (C) 2001 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Collections; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Threading; using System.Diagnostics; using System.Security; #pragma warning disable 1634, 1691 //disable warnings about unknown pragmas #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif namespace MS.Utility { #region Trace ////// These are the standard event types. Higher byte values can be used to define custom event /// types within a given event class GUID. /// [FriendAccessAllowed] // Built into Base, also used by Core and Framework internal static class EventType { internal const byte Info = 0x00; // Information or Point Event internal const byte StartEvent = 0x01; // Start of an activity internal const byte EndEvent = 0x02; // End of an activity internal const byte Checkpoint = 0x08; // Checkpoint Event } ////// This enum is provided to allow users of the trace APIs to avoid passing a full 128-bit /// GUID to our APIs simply to no-op because the event tracing is disabled. /// They are resolved to true GUIDs in EventTrace.GuidFromId. /// [FriendAccessAllowed] internal enum EventTraceGuidId: byte { // // Event GUIDs // DISPATCHERDISPATCHGUID, DISPATCHERPOSTGUID, DISPATCHERABORTGUID, DISPATCHERPROMOTEGUID, DISPATCHERIDLEGUID, LAYOUTPASSGUID, MEASUREGUID, ARRANGEGUID, ONRENDERGUID, MEDIACREATEVISUALGUID, HWNDMESSAGEGUID, RENDERHANDLERGUID, ANIMRENDERHANDLERGUID, MEDIARENDERGUID, POSTRENDERGUID, PERFFREQUENCYGUID, PRECOMPUTESCENEGUID, COMPILESCENEGUID, UPDATEREALIZATIONSGUID, UIRESPONSEGUID, COMMITCHANNELGUID, UCENOTIFYPRESENTGUID, SCHEDULERENDERGUID, PARSEBAMLGUID, PARSEXMLGUID, PARSEXMLINITIALIZEDGUID, PARSEFEFCREATEINSTANCEGUID, PARSESTYLEINSTANTIATEVISUALTREEGUID, PARSEREADERCREATEINSTANCEGUID, PARSEREADERCREATEINSTANCEFROMTYPEGUID, APPCTORGUID, APPRUNGUID, TIMEMANAGERTICKGUID, GENERICSTRINGGUID, FONTCACHELIMITGUID, DRXOPENPACKAGEGUID, DRXREADSTREAMGUID, DRXGETSTREAMGUID, DRXPAGEVISIBLEGUID, DRXPAGELOADEDGUID, DRXINVALIDATEVIEWGUID, DRXLINEDOWNGUID, DRXPAGEDOWNGUID, DRXPAGEJUMPGUID, DRXLAYOUTGUID, DRXINSTANTIATEDGUID, DRXSTYLECREATEDGUID, DRXFINDGUID, DRXZOOMGUID, DRXENSUREOMGUID, DRXGETPAGEGUID, DRXTREEFLATTENGUID, DRXALPHAFLATTENGUID, DRXGETDEVMODEGUID, DRXSTARTDOCGUID, DRXENDDOCGUID, DRXSTARTPAGEGUID, DRXENDPAGEGUID, DRXCOMMITPAGEGUID, DRXCONVERTFONTGUID, DRXCONVERTIMAGEGUID, DRXSAVEXPSGUID, DRXLOADPRIMITIVEGUID, DRXSAVEPAGEGUID, DRXSERIALIZATIONGUID, PROPERTYVALIDATIONGUID, PROPERTYINVALIDATIONGUID, PROPERTYPARENTCHECKGUID, RESOURCEFINDGUID, RESOURCECACHEVALUEGUID, RESOURCECACHENULLGUID, RESOURCECACHEMISSGUID, RESOURCESTOCKGUID, RESOURCEBAMLASSEMBLYGUID, CREATESTICKYNOTEGUID, DELETETEXTNOTEGUID, DELETEINKNOTEGUID, CREATEHIGHLIGHTGUID, CLEARHIGHLIGHTGUID, LOADANNOTATIONSGUID, UNLOADANNOTATIONSGUID, ADDANNOTATIONGUID, DELETEANNOTATIONGUID, GETANNOTATIONBYIDGUID, GETANNOTATIONBYLOCGUID, GETANNOTATIONSGUID, SERIALIZEANNOTATIONGUID, DESERIALIZEANNOTATIONGUID, UPDATEANNOTATIONWITHSNCGUID, UPDATESNCWITHANNOTATIONGUID, ANNOTATIONTEXTCHANGEDGUID, ANNOTATIONINKCHANGEDGUID, ADDATTACHEDSNGUID, REMOVEATTACHEDSNGUID, ADDATTACHEDHIGHLIGHTGUID, REMOVEATTACHEDHIGHLIGHTGUID, ADDATTACHEDMHGUID, REMOVEATTACHEDMHGUID, PRESENTATIONHOSTGUID, HOSTINGGUID, NAVIGATIONGUID, SPLASHSCREENGUID, } ////// This event tracing class provides a windows client interface to the /// TraceProvider interface. Events are logged through the EventProvider /// object, using the following format: /// EventTrace.EventProvider.TraceEvent(TRACEGUID, EVENT_TYPE, OPERATION_TYPE, [DATA,]*); /// /// TRACEGUID - Differentiates the format of the record placed in the ETL log file. /// EVENT_TYPE - Event types are defined in TraceProvider.cs /// EventType.Info - Information or point event. /// EventType.StartEvent - Start of operation. /// EventType.EndEvent - End of operation. /// EventType.Checkpoint - Checkpoint within bounded operation. This might be used /// to indicate transfer of control from one resource to another. /// OPERATION_TYPE - Avalon operation. /// DATA - Optional additional data logged with the TRACEGUID. /// /// Example: /// if (EventTrace.IsEnabled(EventTrace.Flags.{flag}, EventTrace.Level.{level})) /// EventTrace.EventProvider.TraceEvent(EventTrace.DISPATCHERDISPATCHGUID, EventType.StartEvent, EventTrace.DISPATCHERDISPATCH, item.GetType()); /// {...} /// if (EventTrace.IsEnabled(EventTrace.Flags.{flag}, EventTrace.Level.{level})) /// EventTrace.EventProvider.TraceEvent(EventTrace.DISPATCHERDISPATCHGUID, EventType.EndEvent, EventTrace.DISPATCHERDISPATCH); /// /// //CASRemoval:[SecurityPermission(SecurityAction.LinkDemand, Unrestricted=true)] [FriendAccessAllowed] sealed internal class EventTrace { ////// This class has only static methods, so use a private default constructor to /// prevent instances from being created /// private EventTrace() { } ////// This is the provider class that actually provides the event tracing /// functionality. /// static readonly internal TraceProvider EventProvider; static internal void NormalTraceEvent(EventTraceGuidId guidId, byte evType) { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.normal)) { EventTrace.EventProvider.TraceEvent(GuidFromId(guidId), evType); } } // These are browser-hosting events coming from managed code. // PresentationHost has its own set of events. See src\Host\Shared\Tracing.hxx. internal enum HostingEvent : byte { DocObjHostCreated = 10, IBHSRunStart = 11, IBHSRunEnd = 12, XappLauncherAppStartup = 13, XappLauncherAppExit = 14, DocObjHostRunApplicationStart = 15, DocObjHostRunApplicationEnd = 16, ClickOnceActivationStart = 17, ClickOnceActivationEnd = 18, DocObjHostInitAppProxyStart = 19, DocObjHostInitAppProxyEnd = 20, AppProxyCtor = 30, RootBrowserWindowSetupStart = 31, RootBrowserWindowSetupEnd = 32, AppProxyRunStart = 33, AppProxyRunEnd = 34, AppDomainManagerCctor = 40, ApplicationActivatorCreateInstanceStart = 41, ApplicationActivatorCreateInstanceEnd = 42, DetermineApplicationTrustStart = 43, DetermineApplicationTrustEnd = 44, FirstTimeActivation = 50, GetDownloadPageStart = 51, GetDownloadPageEnd = 52, DownloadDeplManifestStart = 53, DownloadDeplManifestEnd = 54, AssertAppRequirementsStart = 55, AssertAppRequirementsEnd = 56, DownloadApplicationStart = 57, DownloadApplicationEnd = 58, DownloadProgressUpdate = 59, XappLauncherAppNavigated = 60, StartingFontCacheServiceStart = 61, StartingFontCacheServiceEnd = 62, UpdateBrowserCommandsStart = 70, UpdateBrowserCommandsEnd = 71, PostShutdown = 80, AbortingActivation = 81, }; internal enum NavigationEvent : byte { NavigationStart = 10, NavigationAsyncWorkItem = 11, NavigationWebResponseReceived = 12, NavigationLaunchBrowser = 13, NavigationEnd = 14, NavigationContentRendered = 15, NavigationPageFunctionReturn = 16, }; internal enum SplashScreenEvent : byte { LoadAndDecodeImageStart = 10, LoadAndDecodeImageEnd = 11, CreateWindow = 12, DestroyWindow = 13, } ////// This method resolves a Guid from an EventTraceGuidId. This indirection allows clients /// to avoid passing a full 128 bit value around in the case where tracing is a no-op, and it /// also causes this ~10k method to be loaded only when tracing is enabled. /// internal static Guid GuidFromId(EventTraceGuidId guidId) { switch (guidId) { // WClientUIContextDispatch+ (UInt32 Priority, String Operation) // WClientUIContextDispatch- // {2481a374-999f-4ad2-9f22-6b7c8e2a5db0} case EventTraceGuidId.DISPATCHERDISPATCHGUID: return new Guid(0x2481a374, 0x999f, 0x4ad2, 0x9f, 0x22, 0x6b, 0x7c, 0x8e, 0x2a, 0x5d, 0xb0); // WClientUIContextPost (UInt32 Priority, String Operation) // {76287aef-f674-4061-a60a-76f95550efeb} case EventTraceGuidId.DISPATCHERPOSTGUID: return new Guid(0x76287aef, 0xf674, 0x4061, 0xa6, 0xa, 0x76, 0xf9, 0x55, 0x50, 0xef, 0xeb); // WClientUIContextAbort (UInt32 Priority, String Operation) // {39404da9-413f-4581-a0a1-4715168b5ad8} case EventTraceGuidId.DISPATCHERABORTGUID: return new Guid(0x39404da9, 0x413f, 0x4581, 0xa0, 0xa1, 0x47, 0x15, 0x16, 0x8b, 0x5a, 0xd8); // WClientUIContextPromote (UInt32 Priority, String Operation) // {632d4e9e-b988-4b32-ab2a-b37aa34927ee} case EventTraceGuidId.DISPATCHERPROMOTEGUID: return new Guid(0x632d4e9e, 0xb988, 0x4b32, 0xab, 0x2a, 0xb3, 0x7a, 0xa3, 0x49, 0x27, 0xee); // WClientUIContextIdle // {c626ebef-0780-487f-81d7-38d3f0a6f05e} case EventTraceGuidId.DISPATCHERIDLEGUID: return new Guid(0xc626ebef, 0x780, 0x487f, 0x81, 0xd7, 0x38, 0xd3, 0xf0, 0xa6, 0xf0, 0x5e); // WClientLayout+ (Int32 Id) // WClientLayout- // {a3edb710-21fc-4f91-97f4-ac2b0df1c20f} case EventTraceGuidId.LAYOUTPASSGUID: return new Guid(0xa3edb710, 0x21fc, 0x4f91, 0x97, 0xf4, 0xac, 0x2b, 0xd, 0xf1, 0xc2, 0xf); // WClientMeasure+ (Int32 Id) // WClientMeasure- // {3005e67b-129c-4ced-bcaa-91d7d73b1544} case EventTraceGuidId.MEASUREGUID: return new Guid(0x3005e67b, 0x129c, 0x4ced, 0xbc, 0xaa, 0x91, 0xd7, 0xd7, 0x3b, 0x15, 0x44); // WClientArrange+ (Int32 Id) // WClientArrange- // {4b0ef3d1-0cbb-4847-b98f-16408e7e83f3} case EventTraceGuidId.ARRANGEGUID: return new Guid(0x4b0ef3d1, 0xcbb, 0x4847, 0xb9, 0x8f, 0x16, 0x40, 0x8e, 0x7e, 0x83, 0xf3); // WClientOnrender+ (Int32 Id) // WClientOnrender- // {3A475CEF-0E2A-449b-986E-EFFF5D6260E7} case EventTraceGuidId.ONRENDERGUID: return new Guid(0x3a475cef, 0xe2a, 0x449b, 0x98, 0x6e, 0xef, 0xff, 0x5d, 0x62, 0x60, 0xe7); // WClientCreateVisual (Int32 Id, Int64 HWND) // {2dbecf62-51ea-493a-8dd0-4bee1ccbe8aa} case EventTraceGuidId.MEDIACREATEVISUALGUID: return new Guid(0x2dbecf62, 0x51ea, 0x493a, 0x8d, 0xd0, 0x4b, 0xee, 0x1c, 0xcb, 0xe8, 0xaa); // Info (Int32 Id, Int64 HWND, Int32 Msg, Int32 WParam, Int32 LParam) // {4ac79bac-7dfb-4402-a910-fdafe16f29b2} case EventTraceGuidId.HWNDMESSAGEGUID: return new Guid(0x4ac79bac, 0x7dfb, 0x4402, 0xa9, 0x10, 0xfd, 0xaf, 0xe1, 0x6f, 0x29, 0xb2); // WClientRenderHandler+ (Int32 Id) // WClientRenderHandler- // {7723d8b7-488b-4f80-b089-46a4c6aca1c4} case EventTraceGuidId.RENDERHANDLERGUID: return new Guid(0x7723d8b7, 0x488b, 0x4f80, 0xb0, 0x89, 0x46, 0xa4, 0xc6, 0xac, 0xa1, 0xc4); // WClientAnimRenderHandler+ (Int32 Id) // WClientAnimRenderHandler- // {521c1c8d-faaa-435b-ad8c-1d64442bfd70} case EventTraceGuidId.ANIMRENDERHANDLERGUID: return new Guid(0x521c1c8d, 0xfaaa, 0x435b, 0xad, 0x8c, 0x1d, 0x64, 0x44, 0x2b, 0xfd, 0x70); // WClientMediaRender+ (Int32 RenderId, Int64 ExpectedPresentTime) // WClientMediaRender- // {6827e447-0e0e-4b5e-ae81-b79a00ec8349} case EventTraceGuidId.MEDIARENDERGUID: return new Guid(0x6827e447, 0xe0e, 0x4b5e, 0xae, 0x81, 0xb7, 0x9a, 0x0, 0xec, 0x83, 0x49); // WClientPostRender // {fb69cd45-c00d-4c23-9765-69c00344b2c5} case EventTraceGuidId.POSTRENDERGUID: return new Guid(0xfb69cd45, 0xc00d, 0x4c23, 0x97, 0x65, 0x69, 0xc0, 0x03, 0x44, 0xb2, 0xc5); // WClientPerfFrequency (Int64 qpcFrequency, Int64 qpcCurrentTime) // {30EE0097-084C-408b-9038-73BED0479873} case EventTraceGuidId.PERFFREQUENCYGUID: return new Guid(0x30ee0097, 0x84c, 0x408b, 0x90, 0x38, 0x73, 0xbe, 0xd0, 0x47, 0x98, 0x73); // WClientPrecomputeScene+ (Int32 Id) // WClientPrecomputeScene- // {3331420f-7a3b-42b6-8dfe-aabf472801da} case EventTraceGuidId.PRECOMPUTESCENEGUID: return new Guid(0x3331420f, 0x7a3b, 0x42b6, 0x8d, 0xfe, 0xaa, 0xbf, 0x47, 0x28, 0x1, 0xda); // WClientCompileScene+ (Int32 Id) // WClientCompileScene- // {af36fcb5-58e5-48d0-88d0-d8f4dcb56a12} case EventTraceGuidId.COMPILESCENEGUID: return new Guid(0xaf36fcb5, 0x58e5, 0x48d0, 0x88, 0xd0, 0xd8, 0xf4, 0xdc, 0xb5, 0x6a, 0x12); // WClientUpdateRealizations+ // WClientUpdateRealizations- // {22272cfc-de04-4900-af53-3ee8ef1ef426} case EventTraceGuidId.UPDATEREALIZATIONSGUID: return new Guid(0x22272cfc, 0xde04, 0x4900, 0xaf, 0x53, 0x3e, 0xe8, 0xef, 0x1e, 0xf4, 0x26); // WClientUIResponse (Int32 ContextId, Int32 ResponseID) // {ab29585b-4794-4465-91e6-9df5861c88c5} case EventTraceGuidId.UIRESPONSEGUID: return new Guid(0xab29585b, 0x4794, 0x4465, 0x91, 0xe6, 0x9d, 0xf5, 0x86, 0x1c, 0x88, 0xc5); // WClientUICommitBatch (Int32 RenderId) // {f9c0372e-60bd-46c9-bc64-94fe5fd31fe4} case EventTraceGuidId.COMMITCHANNELGUID: return new Guid("f9c0372e-60bd-46c9-bc64-94fe5fd31fe4"); // WClientUceNotifyPresented (Int32 RenderId, long LastPresentTime) // {24cd1476-e145-4e5a-8bfc-50c36bbdf9cc} case EventTraceGuidId.UCENOTIFYPRESENTGUID: return new Guid("24cd1476-e145-4e5a-8bfc-50c36bbdf9cc"); // WClientScheduleRender (Int32 RenderDelay) // {6d5aeaf3-a433-4daa-8b31-d8ae49cf6bd1} case EventTraceGuidId.SCHEDULERENDERGUID: return new Guid("6d5aeaf3-a433-4daa-8b31-d8ae49cf6bd1"); // WClientParseBaml+ // WClientParseBaml- // {8a1e3af5-3a6d-4582-86d1-5901471ebbde} case EventTraceGuidId.PARSEBAMLGUID: return new Guid(0x8a1e3af5, 0x3a6d, 0x4582, 0x86, 0xd1, 0x59, 0x1, 0x47, 0x1e, 0xbb, 0xde); // WClientParseXml+ // WClientParseXml- // {bf86e5bf-3fb4-442f-a34a-b207a3b19c3b} case EventTraceGuidId.PARSEXMLGUID: return new Guid(0xbf86e5bf, 0x3fb4, 0x442f, 0xa3, 0x4a, 0xb2, 0x7, 0xa3, 0xb1, 0x9c, 0x3b); // WClientParseXmlInitialized // {80484707-838b-4328-9f31-5ecbaf25a531} case EventTraceGuidId.PARSEXMLINITIALIZEDGUID: return new Guid(0x80484707, 0x838b, 0x4328, 0x9f, 0x31, 0x5e, 0xcb, 0xaf, 0x25, 0xa5, 0x31); // WClientParseFefCrInst+ // WClientParseFefCrInst- // {f7555161-6c1a-4a12-828d-8492a7699a49} case EventTraceGuidId.PARSEFEFCREATEINSTANCEGUID: return new Guid(0xf7555161, 0x6c1a, 0x4a12, 0x82, 0x8d, 0x84, 0x92, 0xa7, 0x69, 0x9a, 0x49); // WClientParseInstVisTree+ // WClientParseInstVisTree- (String Message) // {a8c3b9c0-562b-4509-becb-a08e481a7273} case EventTraceGuidId.PARSESTYLEINSTANTIATEVISUALTREEGUID: return new Guid(0xa8c3b9c0, 0x562b, 0x4509, 0xbe, 0xcb, 0xa0, 0x8e, 0x48, 0x1a, 0x72, 0x73); // WClientParseRdrCrInst+ // WClientParseRdrCrInst- // {8ba8f51c-0775-4adf-9eed-b1654ca088f5} case EventTraceGuidId.PARSEREADERCREATEINSTANCEGUID: return new Guid(0x8ba8f51c, 0x775, 0x4adf, 0x9e, 0xed, 0xb1, 0x65, 0x4c, 0xa0, 0x88, 0xf5); // WClientParseRdrCrInFTyp+ // WClientParseRdrCrInFTyp- (String Type) // {0da15d58-c3a7-40de-9113-72db0c4a9351} case EventTraceGuidId.PARSEREADERCREATEINSTANCEFROMTYPEGUID: return new Guid(0xda15d58, 0xc3a7, 0x40de, 0x91, 0x13, 0x72, 0xdb, 0xc, 0x4a, 0x93, 0x51); // WClientAppCtor // {f9f048c6-2011-4d0a-812a-23a4a4d801f5} case EventTraceGuidId.APPCTORGUID: return new Guid(0xf9f048c6, 0x2011, 0x4d0a, 0x81, 0x2a, 0x23, 0xa4, 0xa4, 0xd8, 0x1, 0xf5); // WClientAppRun // {08a719d6-ea79-4abc-9799-38eded602133} case EventTraceGuidId.APPRUNGUID: return new Guid(0x8a719d6, 0xea79, 0x4abc, 0x97, 0x99, 0x38, 0xed, 0xed, 0x60, 0x21, 0x33); // WClientTimeManagerTick+ (Int64 TickTime) // WClientTimeManagerTick- // {ea3b4b66-b25f-4e5d-8bd4-ec62bb44583e} case EventTraceGuidId.TIMEMANAGERTICKGUID: return new Guid(0xea3b4b66, 0xb25f, 0x4e5d, 0x8b, 0xd4, 0xec, 0x62, 0xbb, 0x44, 0x58, 0x3e); // WClientString (String Info) // WClientString+ (String Info) // WClientString- (String Info) // {6b3c0258-9ddb-4579-8660-41c3ada25c34} case EventTraceGuidId.GENERICSTRINGGUID: return new Guid(0x6b3c0258, 0x9ddb, 0x4579, 0x86, 0x60, 0x41, 0xc3, 0xad, 0xa2, 0x5c, 0x34); // WClientFontCache // {f3362106-b861-4980-9aac-b1ef0bab75aa} case EventTraceGuidId.FONTCACHELIMITGUID: return new Guid(0xf3362106, 0xb861, 0x4980, 0x9a, 0xac, 0xb1, 0xef, 0xb, 0xab, 0x75, 0xaa); // WClientDRXOpenPackage+ // WClientDRXOpenPackage- // {2b8f75f3-f8f9-4075-b914-5ae853c76276} case EventTraceGuidId.DRXOPENPACKAGEGUID: return new Guid(0x2b8f75f3, 0xf8f9, 0x4075, 0xb9, 0x14, 0x5a, 0xe8, 0x53, 0xc7, 0x62, 0x76); // WClientDRXReadStream+ (Int32 Count) // WClientDRXReadStream- (Int32 Result) // {c2b15025-7812-4e44-8b68-7d734303438a} case EventTraceGuidId.DRXREADSTREAMGUID: return new Guid(0xc2b15025, 0x7812, 0x4e44, 0x8b, 0x68, 0x7d, 0x73, 0x43, 0x3, 0x43, 0x8a); // WClientDRXGetStream+ // WClientDRXGetStream- // {3f4510eb-9ee8-4b80-9ec7-775efeb1ba72} case EventTraceGuidId.DRXGETSTREAMGUID: return new Guid(0x3f4510eb, 0x9ee8, 0x4b80, 0x9e, 0xc7, 0x77, 0x5e, 0xfe, 0xb1, 0xba, 0x72); // WClientDRXPageVisible (Int32 FirstVisiblePage, Int32 LastVisiblePage) // {2ae7c601-0aec-4c99-ba80-2eca712d1b97} case EventTraceGuidId.DRXPAGEVISIBLEGUID: return new Guid(0x2ae7c601, 0xaec, 0x4c99, 0xba, 0x80, 0x2e, 0xca, 0x71, 0x2d, 0x1b, 0x97); // WClientDRXPageLoaded (Int32 PageNumber) // {66028645-e022-4d90-a7bd-a8ccdacdb2e1} case EventTraceGuidId.DRXPAGELOADEDGUID: return new Guid(0x66028645, 0xe022, 0x4d90, 0xa7, 0xbd, 0xa8, 0xcc, 0xda, 0xcd, 0xb2, 0xe1); // WClientDRXInvalidateView // {3be3740f-0a31-4d22-a2a3-4d4b6d3ab899} case EventTraceGuidId.DRXINVALIDATEVIEWGUID: return new Guid(0x3be3740f, 0xa31, 0x4d22, 0xa2, 0xa3, 0x4d, 0x4b, 0x6d, 0x3a, 0xb8, 0x99); // WClientDRXLineDown (Int32 VerticalOffset) // {b67ab12c-29bf-4020-b678-f043925b8235} case EventTraceGuidId.DRXLINEDOWNGUID: return new Guid(0xb67ab12c, 0x29bf, 0x4020, 0xb6, 0x78, 0xf0, 0x43, 0x92, 0x5b, 0x82, 0x35); // WClientDRXPageDown (Int32 VerticalOffset) // {d7cdeb52-5ba3-4e02-b114-385a61e7ba9d} case EventTraceGuidId.DRXPAGEDOWNGUID: return new Guid(0xd7cdeb52, 0x5ba3, 0x4e02, 0xb1, 0x14, 0x38, 0x5a, 0x61, 0xe7, 0xba, 0x9d); // WClientDRXPageJump (Int32 PageNumber, Int32 FirstVisiblePage) // {f068b137-7b09-44a1-84d0-4ff1592e0ac1} case EventTraceGuidId.DRXPAGEJUMPGUID: return new Guid(0xf068b137, 0x7b09, 0x44a1, 0x84, 0xd0, 0x4f, 0xf1, 0x59, 0x2e, 0xa, 0xc1); // WClientDRXLayout+ // WClientDRXLayout- // {34fbea40-0238-498f-b12a-631f5a8ef9a5} case EventTraceGuidId.DRXLAYOUTGUID: return new Guid(0x34fbea40, 0x238, 0x498f, 0xb1, 0x2a, 0x63, 0x1f, 0x5a, 0x8e, 0xf9, 0xa5); // WClientDRXInstantiated // {9de677e1-914a-426c-bcd9-2ccdea3648df} case EventTraceGuidId.DRXINSTANTIATEDGUID: return new Guid(0x9de677e1, 0x914a, 0x426c, 0xbc, 0xd9, 0x2c, 0xcd, 0xea, 0x36, 0x48, 0xdf); // WClientDRXStyleCreated // {69737c35-1636-43be-a352-428ca36d1b2c} case EventTraceGuidId.DRXSTYLECREATEDGUID: return new Guid(0x69737c35, 0x1636, 0x43be, 0xa3, 0x52, 0x42, 0x8c, 0xa3, 0x6d, 0x1b, 0x2c); // WClientDRXFind+ // WClientDRXFind- // {ff8efb74-efaa-424d-9022-ee8d21ad804e} case EventTraceGuidId.DRXFINDGUID: return new Guid(0xff8efb74, 0xefaa, 0x424d, 0x90, 0x22, 0xee, 0x8d, 0x21, 0xad, 0x80, 0x4e); // WClientDRXZoom (Int32 Zoom) // {2e5045a1-8dac-4c90-9995-3260de166c8f} case EventTraceGuidId.DRXZOOMGUID: return new Guid(0x2e5045a1, 0x8dac, 0x4c90, 0x99, 0x95, 0x32, 0x60, 0xde, 0x16, 0x6c, 0x8f); // WClientDRXEnsureOM+ // WClientDRXEnsureOM- // {28e3a8bb-aebb-48e8-86b6-32759b47fcbe} case EventTraceGuidId.DRXENSUREOMGUID: return new Guid(0x28e3a8bb, 0xaebb, 0x48e8, 0x86, 0xb6, 0x32, 0x75, 0x9b, 0x47, 0xfc, 0xbe); // WClientDRXGetPage+ // WClientDRXGetPage- // {a0c17259-c6b1-4850-a9ab-13659fe6dc58} case EventTraceGuidId.DRXGETPAGEGUID: return new Guid(0xa0c17259, 0xc6b1, 0x4850, 0xa9, 0xab, 0x13, 0x65, 0x9f, 0xe6, 0xdc, 0x58); // WClientDRXTreeFlatten+ // WClientDRXTreeFlatten- // {b4557454-212b-4f57-b9ca-2ba9d58273b3} case EventTraceGuidId.DRXTREEFLATTENGUID: return new Guid(0xb4557454, 0x212b, 0x4f57, 0xb9, 0xca, 0x2b, 0xa9, 0xd5, 0x82, 0x73, 0xb3); // WClientDRXAlphaFlatten+ // WClientDRXAlphaFlatten- // {302f02e9-f025-4083-abd5-2ce3aaa9a3cf} case EventTraceGuidId.DRXALPHAFLATTENGUID: return new Guid(0x302f02e9, 0xf025, 0x4083, 0xab, 0xd5, 0x2c, 0xe3, 0xaa, 0xa9, 0xa3, 0xcf); // WClientDRXGetDevMode+ // WClientDRXGetDevMode- // {573ea8dc-db6c-42c0-91f8-964e39cb6a70} case EventTraceGuidId.DRXGETDEVMODEGUID: return new Guid(0x573ea8dc, 0xdb6c, 0x42c0, 0x91, 0xf8, 0x96, 0x4e, 0x39, 0xcb, 0x6a, 0x70); // WClientDRXStartDoc+ // WClientDRXStartDoc- // {f3fba666-fa0f-4487-b846-9f204811bf3d} case EventTraceGuidId.DRXSTARTDOCGUID: return new Guid(0xf3fba666, 0xfa0f, 0x4487, 0xb8, 0x46, 0x9f, 0x20, 0x48, 0x11, 0xbf, 0x3d); // WClientDRXEndDoc+ // WClientDRXEndDoc- // {743dd3cf-bbce-4e69-a4db-85226ec6a445} case EventTraceGuidId.DRXENDDOCGUID: return new Guid(0x743dd3cf, 0xbbce, 0x4e69, 0xa4, 0xdb, 0x85, 0x22, 0x6e, 0xc6, 0xa4, 0x45); // WClientDRXStartPage+ // WClientDRXEndPage- // {5303d552-28ab-4dac-8bcd-0f7d5675a157} case EventTraceGuidId.DRXSTARTPAGEGUID: return new Guid(0x5303d552, 0x28ab, 0x4dac, 0x8b, 0xcd, 0xf, 0x7d, 0x56, 0x75, 0xa1, 0x57); // WClientDRXEndPage+ // WClientDRXEndPage- // {e20fddf4-17a6-4e5f-8693-3dd7cb049422} case EventTraceGuidId.DRXENDPAGEGUID: return new Guid(0xe20fddf4, 0x17a6, 0x4e5f, 0x86, 0x93, 0x3d, 0xd7, 0xcb, 0x4, 0x94, 0x22); // WClientDRXCommitPage+ // WClientDRXCommitPage- // {7d7ee18d-aea5-493f-9ef2-bbdb36fcaa78} case EventTraceGuidId.DRXCOMMITPAGEGUID: return new Guid(0x7d7ee18d, 0xaea5, 0x493f, 0x9e, 0xf2, 0xbb, 0xdb, 0x36, 0xfc, 0xaa, 0x78); // WClientDRXConvertFont+ // WClientDRXConvertFont- // {88fc2d42-b1de-4588-8c3b-dc5bec03a9ac} case EventTraceGuidId.DRXCONVERTFONTGUID: return new Guid(0x88fc2d42, 0xb1de, 0x4588, 0x8c, 0x3b, 0xdc, 0x5b, 0xec, 0x3, 0xa9, 0xac); // WClientDRXConvertImage+ // WClientDRXConvertImage- // {17fddfdc-a1be-43b3-b2ee-f5e89b7b1b26} case EventTraceGuidId.DRXCONVERTIMAGEGUID: return new Guid(0x17fddfdc, 0xa1be, 0x43b3, 0xb2, 0xee, 0xf5, 0xe8, 0x9b, 0x7b, 0x1b, 0x26); // WClientDRXSaveXps+ // WClientDRXSaveXps- // {ba0320d5-2294-4067-8b19-ef9cddad4b1a} case EventTraceGuidId.DRXSAVEXPSGUID: return new Guid(0xba0320d5, 0x2294, 0x4067, 0x8b, 0x19, 0xef, 0x9c, 0xdd, 0xad, 0x4b, 0x1a); // WClientDRXLoadPrimitive+ // WClientDRXLoadPrimitive- // {d0b70c99-450e-4872-a2d4-fbfb1dc797fa} case EventTraceGuidId.DRXLOADPRIMITIVEGUID: return new Guid(0xd0b70c99, 0x450e, 0x4872, 0xa2, 0xd4, 0xfb, 0xfb, 0x1d, 0xc7, 0x97, 0xfa); // WClientDRXSavePage+ // WClientDRXSavePage- // {b0e3e78b-9ac7-473c-8903-b5d212399e3b} case EventTraceGuidId.DRXSAVEPAGEGUID: return new Guid(0xb0e3e78b, 0x9ac7, 0x473c, 0x89, 0x3, 0xb5, 0xd2, 0x12, 0x39, 0x9e, 0x3b); // WClientDRXSerialization+ // WClientDRXSerialization- // {0527276c-d3f4-4293-b88c-ecdf7cac4430} case EventTraceGuidId.DRXSERIALIZATIONGUID: return new Guid(0x527276c, 0xd3f4, 0x4293, 0xb8, 0x8c, 0xec, 0xdf, 0x7c, 0xac, 0x44, 0x30); // WClientPropValidate (Int32 ValidationCount) // WClientPropValidate+ (Int32 Id, String TypeAndName) // WClientPropValidate- (Int32 UsingDefault) // {e0bb1a36-6dc9-459b-ab81-b5da910e5d37} case EventTraceGuidId.PROPERTYVALIDATIONGUID: return new Guid(0xe0bb1a36, 0x6dc9, 0x459b, 0xab, 0x81, 0xb5, 0xda, 0x91, 0xe, 0x5d, 0x37); // WClientPropInvalidate (Int32 InvalidationCount) // WClientPropInvalidate+ (Int32 Id, String TypeAndName) // WClientPropInvalidate- // {61159ef2-501f-452f-8d13-51cd05f23e82} case EventTraceGuidId.PROPERTYINVALIDATIONGUID: return new Guid(0x61159ef2, 0x501f, 0x452f, 0x8d, 0x13, 0x51, 0xcd, 0x5, 0xf2, 0x3e, 0x82); // WClientPropParentCheck (Int32 Id, String TypeAndName) // {831bea07-5a2c-434c-8ef8-7eba41c881fb} case EventTraceGuidId.PROPERTYPARENTCHECKGUID: return new Guid(0x831bea07, 0x5a2c, 0x434c, 0x8e, 0xf8, 0x7e, 0xba, 0x41, 0xc8, 0x81, 0xfb); // WClientResourceFind+ (String Key) // WClientResourceFind- // {228d90d5-7e19-4480-9e56-3af2e90f8da6} case EventTraceGuidId.RESOURCEFINDGUID: return new Guid(0x228d90d5, 0x7e19, 0x4480, 0x9e, 0x56, 0x3a, 0xf2, 0xe9, 0xf, 0x8d, 0xa6); // WClientResourceCacheValue // {3b253e2d-72a5-489e-8c65-56c1e6c859b5} case EventTraceGuidId.RESOURCECACHEVALUEGUID: return new Guid(0x3b253e2d, 0x72a5, 0x489e, 0x8c, 0x65, 0x56, 0xc1, 0xe6, 0xc8, 0x59, 0xb5); // WClientResourceCacheNull // {7866a65b-2f38-43b6-abd2-df433bbca073} case EventTraceGuidId.RESOURCECACHENULLGUID: return new Guid(0x7866a65b, 0x2f38, 0x43b6, 0xab, 0xd2, 0xdf, 0x43, 0x3b, 0xbc, 0xa0, 0x73); // WClientResourceCacheMiss // {0420755f-d416-4f15-939f-3e2cd3fcea23} case EventTraceGuidId.RESOURCECACHEMISSGUID: return new Guid(0x420755f, 0xd416, 0x4f15, 0x93, 0x9f, 0x3e, 0x2c, 0xd3, 0xfc, 0xea, 0x23); // WClientResourceStock (String Key) // {06f0fee4-72dd-4802-bd3d-0985139fa91a} case EventTraceGuidId.RESOURCESTOCKGUID: return new Guid(0x6f0fee4, 0x72dd, 0x4802, 0xbd, 0x3d, 0x9, 0x85, 0x13, 0x9f, 0xa9, 0x1a); // WClientResourceBamlAssembly (String AssemblyName) // {19df4373-6680-4a04-8c77-d2f6809ca703} case EventTraceGuidId.RESOURCEBAMLASSEMBLYGUID: return new Guid(0x19df4373, 0x6680, 0x4a04, 0x8c, 0x77, 0xd2, 0xf6, 0x80, 0x9c, 0xa7, 0x3); // CreateStickyNote+ // CreateStickyNote- // {e3dbffac-1e92-4f48-a65a-c290bd5f5f15} case EventTraceGuidId.CREATESTICKYNOTEGUID: return new Guid(0xe3dbffac, 0x1e92, 0x4f48, 0xa6, 0x5a, 0xc2, 0x90, 0xbd, 0x5f, 0x5f, 0x15); // DeleteTextNote+ // DeleteTextNote- // {7626a2f9-9a61-43a3-b7cc-bb84c2493aa7} case EventTraceGuidId.DELETETEXTNOTEGUID: return new Guid(0x7626a2f9, 0x9a61, 0x43a3, 0xb7, 0xcc, 0xbb, 0x84, 0xc2, 0x49, 0x3a, 0xa7); // DeleteInkNote+ // DeleteInkNote- // {bf7e2a93-9d6a-453e-badb-3f8f60075cf2} case EventTraceGuidId.DELETEINKNOTEGUID: return new Guid(0xbf7e2a93, 0x9d6a, 0x453e, 0xba, 0xdb, 0x3f, 0x8f, 0x60, 0x07, 0x5c, 0xf2); // CreateHighlight+ // CreateHighlight- // {c2a5edb8-ac73-41ef-a943-a8a49fa284b1} case EventTraceGuidId.CREATEHIGHLIGHTGUID: return new Guid(0xc2a5edb8, 0xac73, 0x41ef, 0xa9, 0x43, 0xa8, 0xa4, 0x9f, 0xa2, 0x84, 0xb1); // ClearHighlight+ // ClearHighlight- // {e1a59147-d28d-4c5f-b980-691be2fd4208} case EventTraceGuidId.CLEARHIGHLIGHTGUID: return new Guid(0xe1a59147, 0xd28d, 0x4c5f, 0xb9, 0x80, 0x69, 0x1b, 0xe2, 0xfd, 0x42, 0x08); // LoadAnnotations+ // LoadAnnotations- // {cf3a283e-c004-4e7d-b3b9-cc9b582a4a5f} case EventTraceGuidId.LOADANNOTATIONSGUID: return new Guid(0xcf3a283e, 0xc004, 0x4e7d, 0xb3, 0xb9, 0xcc, 0x9b, 0x58, 0x2a, 0x4a, 0x5f); // UnloadAnnotations+ // UnloadAnnotations- // {1c97b272-9b6e-47b0-a470-fcf425377dcc} case EventTraceGuidId.UNLOADANNOTATIONSGUID: return new Guid(0x1c97b272, 0x9b6e, 0x47b0, 0xa4, 0x70, 0xfc, 0xf4, 0x25, 0x37, 0x7d, 0xcc); // AddAnnotation+ // AddAnnotation- // {8f4b2faa-24d6-4ee2-9935-bbf845f758a2} case EventTraceGuidId.ADDANNOTATIONGUID: return new Guid(0x8f4b2faa, 0x24d6, 0x4ee2, 0x99, 0x35, 0xbb, 0xf8, 0x45, 0xf7, 0x58, 0xa2); // DeleteAnnotation+ // DeleteAnnotation- // {4d832230-952a-4464-80af-aab2ac861703} case EventTraceGuidId.DELETEANNOTATIONGUID: return new Guid(0x4d832230, 0x952a, 0x4464, 0x80, 0xaf, 0xaa, 0xb2, 0xac, 0x86, 0x17, 0x03); // GetAnnotationById+ // GetAnnotationById- // {3d27753f-eb8a-4e75-9d5b-82fba55cded1} case EventTraceGuidId.GETANNOTATIONBYIDGUID: return new Guid(0x3d27753f, 0xeb8a, 0x4e75, 0x9d, 0x5b, 0x82, 0xfb, 0xa5, 0x5c, 0xde, 0xd1); // GetAnnotationByLoc+ // GetAnnotationByLoc- // {741a41bc-8ecd-43d1-a7f1-d2faca7362ef} case EventTraceGuidId.GETANNOTATIONBYLOCGUID: return new Guid(0x741a41bc, 0x8ecd, 0x43d1, 0xa7, 0xf1, 0xd2, 0xfa, 0xca, 0x73, 0x62, 0xef); // GetAnnotations+ // GetAnnotations- // {cd9f6017-7e64-4c61-b9ed-5c2fc8c4d849} case EventTraceGuidId.GETANNOTATIONSGUID: return new Guid(0xcd9f6017, 0x7e64, 0x4c61, 0xb9, 0xed, 0x5c, 0x2f, 0xc8, 0xc4, 0xd8, 0x49); // SerializeAnnotation+ // SerializeAnnotation- // {0148924b-5bea-43e9-b3ed-399ca13b35eb} case EventTraceGuidId.SERIALIZEANNOTATIONGUID: return new Guid(0x0148924b, 0x5bea, 0x43e9, 0xb3, 0xed, 0x39, 0x9c, 0xa1, 0x3b, 0x35, 0xeb); // DeserializeAnnotation+ // DeserializeAnnotation- // {2e32c255-d6db-4de7-9e62-9586377778d5} case EventTraceGuidId.DESERIALIZEANNOTATIONGUID: return new Guid(0x2e32c255, 0xd6db, 0x4de7, 0x9e, 0x62, 0x95, 0x86, 0x37, 0x77, 0x78, 0xd5); // UpdateAnnotationWithSNC+ // UpdateAnnotationWithSNC- // {205e0a58-3c7d-495d-b3ed-18c3fb38923f} case EventTraceGuidId.UPDATEANNOTATIONWITHSNCGUID: return new Guid(0x205e0a58, 0x3c7d, 0x495d, 0xb3, 0xed, 0x18, 0xc3, 0xfb, 0x38, 0x92, 0x3f); // UpdateSNCWithAnnotation+ // UpdateSNCWithAnnotation- // {59c337ce-9cc2-4a86-9bfa-061fe954086b} case EventTraceGuidId.UPDATESNCWITHANNOTATIONGUID: return new Guid(0x59c337ce, 0x9cc2, 0x4a86, 0x9b, 0xfa, 0x06, 0x1f, 0xe9, 0x54, 0x08, 0x6b); // AnnotationTextChanged+ // AnnotationTextChanged- // {8bb912b9-39dd-4208-ad62-be66fe5b7ba5} case EventTraceGuidId.ANNOTATIONTEXTCHANGEDGUID: return new Guid(0x8bb912b9, 0x39dd, 0x4208, 0xad, 0x62, 0xbe, 0x66, 0xfe, 0x5b, 0x7b, 0xa5); // AnnotationInkChanged+ // AnnotationInkChanged- // {1228e154-f171-426e-b672-5ee19b755edf} case EventTraceGuidId.ANNOTATIONINKCHANGEDGUID: return new Guid(0x1228e154, 0xf171, 0x426e, 0xb6, 0x72, 0x5e, 0xe1, 0x9b, 0x75, 0x5e, 0xdf); // AddAttachedSN+ // AddAttachedSN- // {9ca660f6-8d7c-4a90-a92f-74482d9cc1cf} case EventTraceGuidId.ADDATTACHEDSNGUID: return new Guid(0x9ca660f6, 0x8d7c, 0x4a90, 0xa9, 0x2f, 0x74, 0x48, 0x2d, 0x9c, 0xc1, 0xcf); // RemoveAttachedSN+ // RemoveAttachedSN- // {8c4c69f7-1185-46df-a5f5-e31ac7e96c07} case EventTraceGuidId.REMOVEATTACHEDSNGUID: return new Guid(0x8c4c69f7, 0x1185, 0x46df, 0xa5, 0xf5, 0xe3, 0x1a, 0xc7, 0xe9, 0x6c, 0x07); // AddAttachedHighlight+ // AddAttachedHighlight- // {56d2cae5-5ec0-44fb-98c2-453e87a0877b} case EventTraceGuidId.ADDATTACHEDHIGHLIGHTGUID: return new Guid(0x56d2cae5, 0x5ec0, 0x44fb, 0x98, 0xc2, 0x45, 0x3e, 0x87, 0xa0, 0x87, 0x7b); // RemoveAttachedHighlight+ // RemoveAttachedHighlight- // {4c81d490-9004-49d1-87d7-289d53a314ef} case EventTraceGuidId.REMOVEATTACHEDHIGHLIGHTGUID: return new Guid(0x4c81d490, 0x9004, 0x49d1, 0x87, 0xd7, 0x28, 0x9d, 0x53, 0xa3, 0x14, 0xef); // AddAttachedMH+ // AddAttachedMH- // {7ea1d548-ca17-ca17-a1a8-f1857db6302e} case EventTraceGuidId.ADDATTACHEDMHGUID: return new Guid(0x7ea1d548, 0xca17, 0xca17, 0xa1, 0xa8, 0xf1, 0x85, 0x7d, 0xb6, 0x30, 0x2e); // RemoveAttachedMH+ // RemoveAttachedMH- // {296c7961-b975-450b-8975-bf862b6c7159} case EventTraceGuidId.REMOVEATTACHEDMHGUID: return new Guid(0x296c7961, 0xb975, 0x450b, 0x89, 0x75, 0xbf, 0x86, 0x2b, 0x6c, 0x71, 0x59); // Defined in src\Host\Shared\Tracing.* case EventTraceGuidId.PRESENTATIONHOSTGUID: return new Guid(0xed251760, 0x7bbc, 0x4b25, 0x83, 0x28, 0xcd, 0x7f, 0x27, 0x1f, 0xee, 0x89); // The HOSTINGGUID's record format is: // HostingEvent.xx { [data0, data1, ..] } // {5FF6B585-7FB9-4189-BEB3-54C82CE4D7D1} case EventTraceGuidId.HOSTINGGUID: return new Guid(0x5ff6b585, 0x7fb9, 0x4189, 0xbe, 0xb3,0x54, 0xc8, 0x2c, 0xe4, 0xd7, 0xd1); // The NAVIGATIONGUID's record format is: // NavigationEvent.xx { [data0, data1, ..] } // {6FFB9C25-5C8A-4091-989C-5B596AB286A0} case EventTraceGuidId.NAVIGATIONGUID: return new Guid(0x6ffb9c25, 0x5c8a, 0x4091, 0x98, 0x9c, 0x5b, 0x59, 0x6a, 0xb2, 0x86, 0xa0); // {3256BDD3-A738-4d3a-9FF5-513D7F54FCA6} case EventTraceGuidId.SPLASHSCREENGUID: return new Guid(0x3256bdd3, 0xa738, 0x4d3a, 0x9f, 0xf5, 0x51, 0x3d, 0x7f, 0x54, 0xfc, 0xa6); default: return new Guid(); } } ////// The users specifies the type of information they want logged by /// passing in one of these flags when enabling tracing. This may /// change as we get more guidelines from the ETW team. /// [Flags] internal enum Flags:int { debugging = 0x00000001, performance = 0x00000002, /// Performance analysis stress = 0x00000004, security = 0x00000008, uiautomation = 0x00000010, response = 0x00000020, /// Response time tracing (benchmarks) trace = 0x00000040, PerToolSupport = 0x00000080, all = 0x7FFFFFFF } ////// Calls to the TraceEvent API specify a level of detail, which the user /// selects when enabling tracing. Setting the detail level to a higher /// level increases the number of events logged. A higher level of /// tracing includes the lower level tracing events. This is used with /// the flags to enable the right set of events. /// internal enum Level: byte { fatal = 1, // Events signalling fatal errors error = 2, // Events that should never happen warning = 3, // Infrequent events that indicate a potential problem normal = 4, // Normal level of tracing verbose = 5, // Additional information } ////// Callers use this to check if they should be logging. /// //[CodeAnalysis("AptcaMethodsShouldOnlyCallAptcaMethods")] //Tracking Bug: 29647 static internal bool IsEnabled(Flags flag, Level level) { if (EventProvider != null && ((uint)level <= EventProvider.Level) && EventProvider.IsEnabled && Convert.ToBoolean((uint)flag & EventProvider.Flags)) { return true; } else { return false; } } static internal bool IsEnabled(Flags flags) { return IsEnabled(flags, Level.normal); } ////// Internal operations associated with initializing the event provider and /// monitoring the Dispatcher and input components. /// ////// Critical: This calls critical code in TraceProvider /// TreatAsSafe: it generates the GUID that is passed into the TraceProvider /// {a42c77db-874f-422e-9b44-6d89fe2bd3e5} /// [SecurityCritical, SecurityTreatAsSafe] static EventTrace() { EventProvider = null; EventProvider = new TraceProvider("WindowsClientProvider", new Guid(0xa42c77db, 0x874f, 0x422e, 0x9b,0x44, 0x6d,0x89,0xfe,0x2b,0xd3,0xe5)); } } #if DEBUG_CLR_MEM ////// This class is taken from the CLRProfiler documentation to provide a windows client interface to /// the CLRProfiler functionality. Events are logged through the CLRProfiler It is documented in the /// CLRProfiler doc by Peter Sollich, the author of the CLRProfiler. /// /// The comments can be used in the timeline view in the CLRProfiler to determine heap usage and /// allocation behavior of sections of program execution delimited by the comments. Comments can /// be used to define allocation patterns and track object lifetimes via the CLRProfiler. /// ///sealed internal class CLRProfilerControl { [DllImport("ProfilerOBJ.dll", CharSet=CharSet.Unicode)] private static extern void LogComment(string comment); [DllImport("ProfilerOBJ.dll")] private static extern bool GetAllocationLoggingActive(); [DllImport("ProfilerOBJ.dll")] private static extern void SetAllocationLoggingActive(bool active); [DllImport("ProfilerOBJ.dll")] private static extern bool GetCallLoggingActive(); [DllImport("ProfilerOBJ.dll")] private static extern void SetCallLoggingActive(bool active); [DllImport("ProfilerOBJ.dll")] private static extern bool DumpHeap(uint timeOut); private static bool _processIsUnderCLRProfiler; private enum CLRGCStateEnum { Unset = -1, None = 0, DumpHeap = 1, Finalizers = 2} private static CLRGCStateEnum _CLRGCMode = CLRGCStateEnum.Unset; /// /// The level of logging we will do, some marks are performance, some are verbose. /// public enum CLRLogState { Unset = -1, None = 0, Performance = 1, Verbose = 2 } private static CLRLogState _CLRLogLevel = CLRLogState.Unset; ////// Static constructor to get things set up. /// static CLRProfilerControl() { try { // If AllocationLoggingActive does something, // this implies ProfilerOBJ.dll is attached // and initialized properly. bool active = GetAllocationLoggingActive(); SetAllocationLoggingActive(!active); _processIsUnderCLRProfiler = active != GetAllocationLoggingActive(); SetAllocationLoggingActive(active); // Default CLRGCMode to be done at logging comments _CLRGCMode = CLRGCStateEnum.None; // Default logging level is performance _CLRLogLevel = CLRLogState.Performance; } // If logging isn't on then CLRProfilerControl should do nothing #pragma warning disable 6502 catch (DllNotFoundException) { return; } #pragma warning restore 6502 } ////// Write the text as a comment in the log, appears as a green vertical line in the Timeline view. /// The setting of CLRGCMode determines what other actions at done BEFORE the mark. /// internal static void CLRLogWriteLine(string comment) { if (_processIsUnderCLRProfiler) { // Force a full GC heap rundown if (CLRAllocationLoggingActive && (_CLRGCMode >= CLRGCStateEnum.DumpHeap)) { CLRDumpHeap(); } LogComment(comment); } } ////// Write the text as a comment in the log, appears as a green vertical line in the Timeline view. /// The setting of CLRGCMode determines what other actions at done BEFORE the mark. /// internal static void CLRLogWriteLine(string format, params object[] args) { if (_processIsUnderCLRProfiler) { // Force a full GC heap rundown if (CLRAllocationLoggingActive && (_CLRGCMode >= CLRGCStateEnum.DumpHeap)) { CLRDumpHeap(); } LogComment(string.Format(System.Globalization.CultureInfo.CurrentCulture, format, args)); } } ////// Logging level /// public static CLRLogState CLRLoggingLevel { get { return _CLRLogLevel; } set { _CLRLogLevel = value; } } ////// Allocation logging active? /// internal static bool CLRAllocationLoggingActive { get { if (_processIsUnderCLRProfiler) { // Check this every time in case it gets turned on/off return GetAllocationLoggingActive(); } else return false; } set { if (_processIsUnderCLRProfiler) { SetAllocationLoggingActive(value); } } } ////// Is call logging active? /// internal static bool CLRCallLoggingActive { get { if (_processIsUnderCLRProfiler) { return GetCallLoggingActive(); } else { return false; } } set { if (_processIsUnderCLRProfiler) { SetCallLoggingActive(value); } } } ////// Dump the CLR Heap. If the CLRGCMode is CLRGCState.Finalizers we will /// force the finalizers to be run as well. /// internal static void CLRDumpHeap() { if (_processIsUnderCLRProfiler) { // Wait for finalizers so that we get an accurate value for live GC heap // objects when the comment is logged. if (_CLRGCMode >= CLRGCStateEnum.Finalizers) { // GC.Collect(2); GC.WaitForPendingFinalizers(); } // Timeout of 60 milliseconds. if (!DumpHeap(60*1000)) { throw new Exception("Failure to dump heap"); } } } ////// Is this process running under the CLRProfiler? /// internal static bool ProcessIsUnderCLRProfiler { get { return _processIsUnderCLRProfiler; } } } #endif // DEBUG_CLR_MEM #endregion Trace } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: Trace.cs * * Description: * Implements ETW tracing for Avalon Managed Code * * History: * 7/10/2002: Curtis Dutton: Created * * Copyright (C) 2001 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Collections; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Threading; using System.Diagnostics; using System.Security; #pragma warning disable 1634, 1691 //disable warnings about unknown pragmas #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif namespace MS.Utility { #region Trace ////// These are the standard event types. Higher byte values can be used to define custom event /// types within a given event class GUID. /// [FriendAccessAllowed] // Built into Base, also used by Core and Framework internal static class EventType { internal const byte Info = 0x00; // Information or Point Event internal const byte StartEvent = 0x01; // Start of an activity internal const byte EndEvent = 0x02; // End of an activity internal const byte Checkpoint = 0x08; // Checkpoint Event } ////// This enum is provided to allow users of the trace APIs to avoid passing a full 128-bit /// GUID to our APIs simply to no-op because the event tracing is disabled. /// They are resolved to true GUIDs in EventTrace.GuidFromId. /// [FriendAccessAllowed] internal enum EventTraceGuidId: byte { // // Event GUIDs // DISPATCHERDISPATCHGUID, DISPATCHERPOSTGUID, DISPATCHERABORTGUID, DISPATCHERPROMOTEGUID, DISPATCHERIDLEGUID, LAYOUTPASSGUID, MEASUREGUID, ARRANGEGUID, ONRENDERGUID, MEDIACREATEVISUALGUID, HWNDMESSAGEGUID, RENDERHANDLERGUID, ANIMRENDERHANDLERGUID, MEDIARENDERGUID, POSTRENDERGUID, PERFFREQUENCYGUID, PRECOMPUTESCENEGUID, COMPILESCENEGUID, UPDATEREALIZATIONSGUID, UIRESPONSEGUID, COMMITCHANNELGUID, UCENOTIFYPRESENTGUID, SCHEDULERENDERGUID, PARSEBAMLGUID, PARSEXMLGUID, PARSEXMLINITIALIZEDGUID, PARSEFEFCREATEINSTANCEGUID, PARSESTYLEINSTANTIATEVISUALTREEGUID, PARSEREADERCREATEINSTANCEGUID, PARSEREADERCREATEINSTANCEFROMTYPEGUID, APPCTORGUID, APPRUNGUID, TIMEMANAGERTICKGUID, GENERICSTRINGGUID, FONTCACHELIMITGUID, DRXOPENPACKAGEGUID, DRXREADSTREAMGUID, DRXGETSTREAMGUID, DRXPAGEVISIBLEGUID, DRXPAGELOADEDGUID, DRXINVALIDATEVIEWGUID, DRXLINEDOWNGUID, DRXPAGEDOWNGUID, DRXPAGEJUMPGUID, DRXLAYOUTGUID, DRXINSTANTIATEDGUID, DRXSTYLECREATEDGUID, DRXFINDGUID, DRXZOOMGUID, DRXENSUREOMGUID, DRXGETPAGEGUID, DRXTREEFLATTENGUID, DRXALPHAFLATTENGUID, DRXGETDEVMODEGUID, DRXSTARTDOCGUID, DRXENDDOCGUID, DRXSTARTPAGEGUID, DRXENDPAGEGUID, DRXCOMMITPAGEGUID, DRXCONVERTFONTGUID, DRXCONVERTIMAGEGUID, DRXSAVEXPSGUID, DRXLOADPRIMITIVEGUID, DRXSAVEPAGEGUID, DRXSERIALIZATIONGUID, PROPERTYVALIDATIONGUID, PROPERTYINVALIDATIONGUID, PROPERTYPARENTCHECKGUID, RESOURCEFINDGUID, RESOURCECACHEVALUEGUID, RESOURCECACHENULLGUID, RESOURCECACHEMISSGUID, RESOURCESTOCKGUID, RESOURCEBAMLASSEMBLYGUID, CREATESTICKYNOTEGUID, DELETETEXTNOTEGUID, DELETEINKNOTEGUID, CREATEHIGHLIGHTGUID, CLEARHIGHLIGHTGUID, LOADANNOTATIONSGUID, UNLOADANNOTATIONSGUID, ADDANNOTATIONGUID, DELETEANNOTATIONGUID, GETANNOTATIONBYIDGUID, GETANNOTATIONBYLOCGUID, GETANNOTATIONSGUID, SERIALIZEANNOTATIONGUID, DESERIALIZEANNOTATIONGUID, UPDATEANNOTATIONWITHSNCGUID, UPDATESNCWITHANNOTATIONGUID, ANNOTATIONTEXTCHANGEDGUID, ANNOTATIONINKCHANGEDGUID, ADDATTACHEDSNGUID, REMOVEATTACHEDSNGUID, ADDATTACHEDHIGHLIGHTGUID, REMOVEATTACHEDHIGHLIGHTGUID, ADDATTACHEDMHGUID, REMOVEATTACHEDMHGUID, PRESENTATIONHOSTGUID, HOSTINGGUID, NAVIGATIONGUID, SPLASHSCREENGUID, } ////// This event tracing class provides a windows client interface to the /// TraceProvider interface. Events are logged through the EventProvider /// object, using the following format: /// EventTrace.EventProvider.TraceEvent(TRACEGUID, EVENT_TYPE, OPERATION_TYPE, [DATA,]*); /// /// TRACEGUID - Differentiates the format of the record placed in the ETL log file. /// EVENT_TYPE - Event types are defined in TraceProvider.cs /// EventType.Info - Information or point event. /// EventType.StartEvent - Start of operation. /// EventType.EndEvent - End of operation. /// EventType.Checkpoint - Checkpoint within bounded operation. This might be used /// to indicate transfer of control from one resource to another. /// OPERATION_TYPE - Avalon operation. /// DATA - Optional additional data logged with the TRACEGUID. /// /// Example: /// if (EventTrace.IsEnabled(EventTrace.Flags.{flag}, EventTrace.Level.{level})) /// EventTrace.EventProvider.TraceEvent(EventTrace.DISPATCHERDISPATCHGUID, EventType.StartEvent, EventTrace.DISPATCHERDISPATCH, item.GetType()); /// {...} /// if (EventTrace.IsEnabled(EventTrace.Flags.{flag}, EventTrace.Level.{level})) /// EventTrace.EventProvider.TraceEvent(EventTrace.DISPATCHERDISPATCHGUID, EventType.EndEvent, EventTrace.DISPATCHERDISPATCH); /// /// //CASRemoval:[SecurityPermission(SecurityAction.LinkDemand, Unrestricted=true)] [FriendAccessAllowed] sealed internal class EventTrace { ////// This class has only static methods, so use a private default constructor to /// prevent instances from being created /// private EventTrace() { } ////// This is the provider class that actually provides the event tracing /// functionality. /// static readonly internal TraceProvider EventProvider; static internal void NormalTraceEvent(EventTraceGuidId guidId, byte evType) { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.normal)) { EventTrace.EventProvider.TraceEvent(GuidFromId(guidId), evType); } } // These are browser-hosting events coming from managed code. // PresentationHost has its own set of events. See src\Host\Shared\Tracing.hxx. internal enum HostingEvent : byte { DocObjHostCreated = 10, IBHSRunStart = 11, IBHSRunEnd = 12, XappLauncherAppStartup = 13, XappLauncherAppExit = 14, DocObjHostRunApplicationStart = 15, DocObjHostRunApplicationEnd = 16, ClickOnceActivationStart = 17, ClickOnceActivationEnd = 18, DocObjHostInitAppProxyStart = 19, DocObjHostInitAppProxyEnd = 20, AppProxyCtor = 30, RootBrowserWindowSetupStart = 31, RootBrowserWindowSetupEnd = 32, AppProxyRunStart = 33, AppProxyRunEnd = 34, AppDomainManagerCctor = 40, ApplicationActivatorCreateInstanceStart = 41, ApplicationActivatorCreateInstanceEnd = 42, DetermineApplicationTrustStart = 43, DetermineApplicationTrustEnd = 44, FirstTimeActivation = 50, GetDownloadPageStart = 51, GetDownloadPageEnd = 52, DownloadDeplManifestStart = 53, DownloadDeplManifestEnd = 54, AssertAppRequirementsStart = 55, AssertAppRequirementsEnd = 56, DownloadApplicationStart = 57, DownloadApplicationEnd = 58, DownloadProgressUpdate = 59, XappLauncherAppNavigated = 60, StartingFontCacheServiceStart = 61, StartingFontCacheServiceEnd = 62, UpdateBrowserCommandsStart = 70, UpdateBrowserCommandsEnd = 71, PostShutdown = 80, AbortingActivation = 81, }; internal enum NavigationEvent : byte { NavigationStart = 10, NavigationAsyncWorkItem = 11, NavigationWebResponseReceived = 12, NavigationLaunchBrowser = 13, NavigationEnd = 14, NavigationContentRendered = 15, NavigationPageFunctionReturn = 16, }; internal enum SplashScreenEvent : byte { LoadAndDecodeImageStart = 10, LoadAndDecodeImageEnd = 11, CreateWindow = 12, DestroyWindow = 13, } ////// This method resolves a Guid from an EventTraceGuidId. This indirection allows clients /// to avoid passing a full 128 bit value around in the case where tracing is a no-op, and it /// also causes this ~10k method to be loaded only when tracing is enabled. /// internal static Guid GuidFromId(EventTraceGuidId guidId) { switch (guidId) { // WClientUIContextDispatch+ (UInt32 Priority, String Operation) // WClientUIContextDispatch- // {2481a374-999f-4ad2-9f22-6b7c8e2a5db0} case EventTraceGuidId.DISPATCHERDISPATCHGUID: return new Guid(0x2481a374, 0x999f, 0x4ad2, 0x9f, 0x22, 0x6b, 0x7c, 0x8e, 0x2a, 0x5d, 0xb0); // WClientUIContextPost (UInt32 Priority, String Operation) // {76287aef-f674-4061-a60a-76f95550efeb} case EventTraceGuidId.DISPATCHERPOSTGUID: return new Guid(0x76287aef, 0xf674, 0x4061, 0xa6, 0xa, 0x76, 0xf9, 0x55, 0x50, 0xef, 0xeb); // WClientUIContextAbort (UInt32 Priority, String Operation) // {39404da9-413f-4581-a0a1-4715168b5ad8} case EventTraceGuidId.DISPATCHERABORTGUID: return new Guid(0x39404da9, 0x413f, 0x4581, 0xa0, 0xa1, 0x47, 0x15, 0x16, 0x8b, 0x5a, 0xd8); // WClientUIContextPromote (UInt32 Priority, String Operation) // {632d4e9e-b988-4b32-ab2a-b37aa34927ee} case EventTraceGuidId.DISPATCHERPROMOTEGUID: return new Guid(0x632d4e9e, 0xb988, 0x4b32, 0xab, 0x2a, 0xb3, 0x7a, 0xa3, 0x49, 0x27, 0xee); // WClientUIContextIdle // {c626ebef-0780-487f-81d7-38d3f0a6f05e} case EventTraceGuidId.DISPATCHERIDLEGUID: return new Guid(0xc626ebef, 0x780, 0x487f, 0x81, 0xd7, 0x38, 0xd3, 0xf0, 0xa6, 0xf0, 0x5e); // WClientLayout+ (Int32 Id) // WClientLayout- // {a3edb710-21fc-4f91-97f4-ac2b0df1c20f} case EventTraceGuidId.LAYOUTPASSGUID: return new Guid(0xa3edb710, 0x21fc, 0x4f91, 0x97, 0xf4, 0xac, 0x2b, 0xd, 0xf1, 0xc2, 0xf); // WClientMeasure+ (Int32 Id) // WClientMeasure- // {3005e67b-129c-4ced-bcaa-91d7d73b1544} case EventTraceGuidId.MEASUREGUID: return new Guid(0x3005e67b, 0x129c, 0x4ced, 0xbc, 0xaa, 0x91, 0xd7, 0xd7, 0x3b, 0x15, 0x44); // WClientArrange+ (Int32 Id) // WClientArrange- // {4b0ef3d1-0cbb-4847-b98f-16408e7e83f3} case EventTraceGuidId.ARRANGEGUID: return new Guid(0x4b0ef3d1, 0xcbb, 0x4847, 0xb9, 0x8f, 0x16, 0x40, 0x8e, 0x7e, 0x83, 0xf3); // WClientOnrender+ (Int32 Id) // WClientOnrender- // {3A475CEF-0E2A-449b-986E-EFFF5D6260E7} case EventTraceGuidId.ONRENDERGUID: return new Guid(0x3a475cef, 0xe2a, 0x449b, 0x98, 0x6e, 0xef, 0xff, 0x5d, 0x62, 0x60, 0xe7); // WClientCreateVisual (Int32 Id, Int64 HWND) // {2dbecf62-51ea-493a-8dd0-4bee1ccbe8aa} case EventTraceGuidId.MEDIACREATEVISUALGUID: return new Guid(0x2dbecf62, 0x51ea, 0x493a, 0x8d, 0xd0, 0x4b, 0xee, 0x1c, 0xcb, 0xe8, 0xaa); // Info (Int32 Id, Int64 HWND, Int32 Msg, Int32 WParam, Int32 LParam) // {4ac79bac-7dfb-4402-a910-fdafe16f29b2} case EventTraceGuidId.HWNDMESSAGEGUID: return new Guid(0x4ac79bac, 0x7dfb, 0x4402, 0xa9, 0x10, 0xfd, 0xaf, 0xe1, 0x6f, 0x29, 0xb2); // WClientRenderHandler+ (Int32 Id) // WClientRenderHandler- // {7723d8b7-488b-4f80-b089-46a4c6aca1c4} case EventTraceGuidId.RENDERHANDLERGUID: return new Guid(0x7723d8b7, 0x488b, 0x4f80, 0xb0, 0x89, 0x46, 0xa4, 0xc6, 0xac, 0xa1, 0xc4); // WClientAnimRenderHandler+ (Int32 Id) // WClientAnimRenderHandler- // {521c1c8d-faaa-435b-ad8c-1d64442bfd70} case EventTraceGuidId.ANIMRENDERHANDLERGUID: return new Guid(0x521c1c8d, 0xfaaa, 0x435b, 0xad, 0x8c, 0x1d, 0x64, 0x44, 0x2b, 0xfd, 0x70); // WClientMediaRender+ (Int32 RenderId, Int64 ExpectedPresentTime) // WClientMediaRender- // {6827e447-0e0e-4b5e-ae81-b79a00ec8349} case EventTraceGuidId.MEDIARENDERGUID: return new Guid(0x6827e447, 0xe0e, 0x4b5e, 0xae, 0x81, 0xb7, 0x9a, 0x0, 0xec, 0x83, 0x49); // WClientPostRender // {fb69cd45-c00d-4c23-9765-69c00344b2c5} case EventTraceGuidId.POSTRENDERGUID: return new Guid(0xfb69cd45, 0xc00d, 0x4c23, 0x97, 0x65, 0x69, 0xc0, 0x03, 0x44, 0xb2, 0xc5); // WClientPerfFrequency (Int64 qpcFrequency, Int64 qpcCurrentTime) // {30EE0097-084C-408b-9038-73BED0479873} case EventTraceGuidId.PERFFREQUENCYGUID: return new Guid(0x30ee0097, 0x84c, 0x408b, 0x90, 0x38, 0x73, 0xbe, 0xd0, 0x47, 0x98, 0x73); // WClientPrecomputeScene+ (Int32 Id) // WClientPrecomputeScene- // {3331420f-7a3b-42b6-8dfe-aabf472801da} case EventTraceGuidId.PRECOMPUTESCENEGUID: return new Guid(0x3331420f, 0x7a3b, 0x42b6, 0x8d, 0xfe, 0xaa, 0xbf, 0x47, 0x28, 0x1, 0xda); // WClientCompileScene+ (Int32 Id) // WClientCompileScene- // {af36fcb5-58e5-48d0-88d0-d8f4dcb56a12} case EventTraceGuidId.COMPILESCENEGUID: return new Guid(0xaf36fcb5, 0x58e5, 0x48d0, 0x88, 0xd0, 0xd8, 0xf4, 0xdc, 0xb5, 0x6a, 0x12); // WClientUpdateRealizations+ // WClientUpdateRealizations- // {22272cfc-de04-4900-af53-3ee8ef1ef426} case EventTraceGuidId.UPDATEREALIZATIONSGUID: return new Guid(0x22272cfc, 0xde04, 0x4900, 0xaf, 0x53, 0x3e, 0xe8, 0xef, 0x1e, 0xf4, 0x26); // WClientUIResponse (Int32 ContextId, Int32 ResponseID) // {ab29585b-4794-4465-91e6-9df5861c88c5} case EventTraceGuidId.UIRESPONSEGUID: return new Guid(0xab29585b, 0x4794, 0x4465, 0x91, 0xe6, 0x9d, 0xf5, 0x86, 0x1c, 0x88, 0xc5); // WClientUICommitBatch (Int32 RenderId) // {f9c0372e-60bd-46c9-bc64-94fe5fd31fe4} case EventTraceGuidId.COMMITCHANNELGUID: return new Guid("f9c0372e-60bd-46c9-bc64-94fe5fd31fe4"); // WClientUceNotifyPresented (Int32 RenderId, long LastPresentTime) // {24cd1476-e145-4e5a-8bfc-50c36bbdf9cc} case EventTraceGuidId.UCENOTIFYPRESENTGUID: return new Guid("24cd1476-e145-4e5a-8bfc-50c36bbdf9cc"); // WClientScheduleRender (Int32 RenderDelay) // {6d5aeaf3-a433-4daa-8b31-d8ae49cf6bd1} case EventTraceGuidId.SCHEDULERENDERGUID: return new Guid("6d5aeaf3-a433-4daa-8b31-d8ae49cf6bd1"); // WClientParseBaml+ // WClientParseBaml- // {8a1e3af5-3a6d-4582-86d1-5901471ebbde} case EventTraceGuidId.PARSEBAMLGUID: return new Guid(0x8a1e3af5, 0x3a6d, 0x4582, 0x86, 0xd1, 0x59, 0x1, 0x47, 0x1e, 0xbb, 0xde); // WClientParseXml+ // WClientParseXml- // {bf86e5bf-3fb4-442f-a34a-b207a3b19c3b} case EventTraceGuidId.PARSEXMLGUID: return new Guid(0xbf86e5bf, 0x3fb4, 0x442f, 0xa3, 0x4a, 0xb2, 0x7, 0xa3, 0xb1, 0x9c, 0x3b); // WClientParseXmlInitialized // {80484707-838b-4328-9f31-5ecbaf25a531} case EventTraceGuidId.PARSEXMLINITIALIZEDGUID: return new Guid(0x80484707, 0x838b, 0x4328, 0x9f, 0x31, 0x5e, 0xcb, 0xaf, 0x25, 0xa5, 0x31); // WClientParseFefCrInst+ // WClientParseFefCrInst- // {f7555161-6c1a-4a12-828d-8492a7699a49} case EventTraceGuidId.PARSEFEFCREATEINSTANCEGUID: return new Guid(0xf7555161, 0x6c1a, 0x4a12, 0x82, 0x8d, 0x84, 0x92, 0xa7, 0x69, 0x9a, 0x49); // WClientParseInstVisTree+ // WClientParseInstVisTree- (String Message) // {a8c3b9c0-562b-4509-becb-a08e481a7273} case EventTraceGuidId.PARSESTYLEINSTANTIATEVISUALTREEGUID: return new Guid(0xa8c3b9c0, 0x562b, 0x4509, 0xbe, 0xcb, 0xa0, 0x8e, 0x48, 0x1a, 0x72, 0x73); // WClientParseRdrCrInst+ // WClientParseRdrCrInst- // {8ba8f51c-0775-4adf-9eed-b1654ca088f5} case EventTraceGuidId.PARSEREADERCREATEINSTANCEGUID: return new Guid(0x8ba8f51c, 0x775, 0x4adf, 0x9e, 0xed, 0xb1, 0x65, 0x4c, 0xa0, 0x88, 0xf5); // WClientParseRdrCrInFTyp+ // WClientParseRdrCrInFTyp- (String Type) // {0da15d58-c3a7-40de-9113-72db0c4a9351} case EventTraceGuidId.PARSEREADERCREATEINSTANCEFROMTYPEGUID: return new Guid(0xda15d58, 0xc3a7, 0x40de, 0x91, 0x13, 0x72, 0xdb, 0xc, 0x4a, 0x93, 0x51); // WClientAppCtor // {f9f048c6-2011-4d0a-812a-23a4a4d801f5} case EventTraceGuidId.APPCTORGUID: return new Guid(0xf9f048c6, 0x2011, 0x4d0a, 0x81, 0x2a, 0x23, 0xa4, 0xa4, 0xd8, 0x1, 0xf5); // WClientAppRun // {08a719d6-ea79-4abc-9799-38eded602133} case EventTraceGuidId.APPRUNGUID: return new Guid(0x8a719d6, 0xea79, 0x4abc, 0x97, 0x99, 0x38, 0xed, 0xed, 0x60, 0x21, 0x33); // WClientTimeManagerTick+ (Int64 TickTime) // WClientTimeManagerTick- // {ea3b4b66-b25f-4e5d-8bd4-ec62bb44583e} case EventTraceGuidId.TIMEMANAGERTICKGUID: return new Guid(0xea3b4b66, 0xb25f, 0x4e5d, 0x8b, 0xd4, 0xec, 0x62, 0xbb, 0x44, 0x58, 0x3e); // WClientString (String Info) // WClientString+ (String Info) // WClientString- (String Info) // {6b3c0258-9ddb-4579-8660-41c3ada25c34} case EventTraceGuidId.GENERICSTRINGGUID: return new Guid(0x6b3c0258, 0x9ddb, 0x4579, 0x86, 0x60, 0x41, 0xc3, 0xad, 0xa2, 0x5c, 0x34); // WClientFontCache // {f3362106-b861-4980-9aac-b1ef0bab75aa} case EventTraceGuidId.FONTCACHELIMITGUID: return new Guid(0xf3362106, 0xb861, 0x4980, 0x9a, 0xac, 0xb1, 0xef, 0xb, 0xab, 0x75, 0xaa); // WClientDRXOpenPackage+ // WClientDRXOpenPackage- // {2b8f75f3-f8f9-4075-b914-5ae853c76276} case EventTraceGuidId.DRXOPENPACKAGEGUID: return new Guid(0x2b8f75f3, 0xf8f9, 0x4075, 0xb9, 0x14, 0x5a, 0xe8, 0x53, 0xc7, 0x62, 0x76); // WClientDRXReadStream+ (Int32 Count) // WClientDRXReadStream- (Int32 Result) // {c2b15025-7812-4e44-8b68-7d734303438a} case EventTraceGuidId.DRXREADSTREAMGUID: return new Guid(0xc2b15025, 0x7812, 0x4e44, 0x8b, 0x68, 0x7d, 0x73, 0x43, 0x3, 0x43, 0x8a); // WClientDRXGetStream+ // WClientDRXGetStream- // {3f4510eb-9ee8-4b80-9ec7-775efeb1ba72} case EventTraceGuidId.DRXGETSTREAMGUID: return new Guid(0x3f4510eb, 0x9ee8, 0x4b80, 0x9e, 0xc7, 0x77, 0x5e, 0xfe, 0xb1, 0xba, 0x72); // WClientDRXPageVisible (Int32 FirstVisiblePage, Int32 LastVisiblePage) // {2ae7c601-0aec-4c99-ba80-2eca712d1b97} case EventTraceGuidId.DRXPAGEVISIBLEGUID: return new Guid(0x2ae7c601, 0xaec, 0x4c99, 0xba, 0x80, 0x2e, 0xca, 0x71, 0x2d, 0x1b, 0x97); // WClientDRXPageLoaded (Int32 PageNumber) // {66028645-e022-4d90-a7bd-a8ccdacdb2e1} case EventTraceGuidId.DRXPAGELOADEDGUID: return new Guid(0x66028645, 0xe022, 0x4d90, 0xa7, 0xbd, 0xa8, 0xcc, 0xda, 0xcd, 0xb2, 0xe1); // WClientDRXInvalidateView // {3be3740f-0a31-4d22-a2a3-4d4b6d3ab899} case EventTraceGuidId.DRXINVALIDATEVIEWGUID: return new Guid(0x3be3740f, 0xa31, 0x4d22, 0xa2, 0xa3, 0x4d, 0x4b, 0x6d, 0x3a, 0xb8, 0x99); // WClientDRXLineDown (Int32 VerticalOffset) // {b67ab12c-29bf-4020-b678-f043925b8235} case EventTraceGuidId.DRXLINEDOWNGUID: return new Guid(0xb67ab12c, 0x29bf, 0x4020, 0xb6, 0x78, 0xf0, 0x43, 0x92, 0x5b, 0x82, 0x35); // WClientDRXPageDown (Int32 VerticalOffset) // {d7cdeb52-5ba3-4e02-b114-385a61e7ba9d} case EventTraceGuidId.DRXPAGEDOWNGUID: return new Guid(0xd7cdeb52, 0x5ba3, 0x4e02, 0xb1, 0x14, 0x38, 0x5a, 0x61, 0xe7, 0xba, 0x9d); // WClientDRXPageJump (Int32 PageNumber, Int32 FirstVisiblePage) // {f068b137-7b09-44a1-84d0-4ff1592e0ac1} case EventTraceGuidId.DRXPAGEJUMPGUID: return new Guid(0xf068b137, 0x7b09, 0x44a1, 0x84, 0xd0, 0x4f, 0xf1, 0x59, 0x2e, 0xa, 0xc1); // WClientDRXLayout+ // WClientDRXLayout- // {34fbea40-0238-498f-b12a-631f5a8ef9a5} case EventTraceGuidId.DRXLAYOUTGUID: return new Guid(0x34fbea40, 0x238, 0x498f, 0xb1, 0x2a, 0x63, 0x1f, 0x5a, 0x8e, 0xf9, 0xa5); // WClientDRXInstantiated // {9de677e1-914a-426c-bcd9-2ccdea3648df} case EventTraceGuidId.DRXINSTANTIATEDGUID: return new Guid(0x9de677e1, 0x914a, 0x426c, 0xbc, 0xd9, 0x2c, 0xcd, 0xea, 0x36, 0x48, 0xdf); // WClientDRXStyleCreated // {69737c35-1636-43be-a352-428ca36d1b2c} case EventTraceGuidId.DRXSTYLECREATEDGUID: return new Guid(0x69737c35, 0x1636, 0x43be, 0xa3, 0x52, 0x42, 0x8c, 0xa3, 0x6d, 0x1b, 0x2c); // WClientDRXFind+ // WClientDRXFind- // {ff8efb74-efaa-424d-9022-ee8d21ad804e} case EventTraceGuidId.DRXFINDGUID: return new Guid(0xff8efb74, 0xefaa, 0x424d, 0x90, 0x22, 0xee, 0x8d, 0x21, 0xad, 0x80, 0x4e); // WClientDRXZoom (Int32 Zoom) // {2e5045a1-8dac-4c90-9995-3260de166c8f} case EventTraceGuidId.DRXZOOMGUID: return new Guid(0x2e5045a1, 0x8dac, 0x4c90, 0x99, 0x95, 0x32, 0x60, 0xde, 0x16, 0x6c, 0x8f); // WClientDRXEnsureOM+ // WClientDRXEnsureOM- // {28e3a8bb-aebb-48e8-86b6-32759b47fcbe} case EventTraceGuidId.DRXENSUREOMGUID: return new Guid(0x28e3a8bb, 0xaebb, 0x48e8, 0x86, 0xb6, 0x32, 0x75, 0x9b, 0x47, 0xfc, 0xbe); // WClientDRXGetPage+ // WClientDRXGetPage- // {a0c17259-c6b1-4850-a9ab-13659fe6dc58} case EventTraceGuidId.DRXGETPAGEGUID: return new Guid(0xa0c17259, 0xc6b1, 0x4850, 0xa9, 0xab, 0x13, 0x65, 0x9f, 0xe6, 0xdc, 0x58); // WClientDRXTreeFlatten+ // WClientDRXTreeFlatten- // {b4557454-212b-4f57-b9ca-2ba9d58273b3} case EventTraceGuidId.DRXTREEFLATTENGUID: return new Guid(0xb4557454, 0x212b, 0x4f57, 0xb9, 0xca, 0x2b, 0xa9, 0xd5, 0x82, 0x73, 0xb3); // WClientDRXAlphaFlatten+ // WClientDRXAlphaFlatten- // {302f02e9-f025-4083-abd5-2ce3aaa9a3cf} case EventTraceGuidId.DRXALPHAFLATTENGUID: return new Guid(0x302f02e9, 0xf025, 0x4083, 0xab, 0xd5, 0x2c, 0xe3, 0xaa, 0xa9, 0xa3, 0xcf); // WClientDRXGetDevMode+ // WClientDRXGetDevMode- // {573ea8dc-db6c-42c0-91f8-964e39cb6a70} case EventTraceGuidId.DRXGETDEVMODEGUID: return new Guid(0x573ea8dc, 0xdb6c, 0x42c0, 0x91, 0xf8, 0x96, 0x4e, 0x39, 0xcb, 0x6a, 0x70); // WClientDRXStartDoc+ // WClientDRXStartDoc- // {f3fba666-fa0f-4487-b846-9f204811bf3d} case EventTraceGuidId.DRXSTARTDOCGUID: return new Guid(0xf3fba666, 0xfa0f, 0x4487, 0xb8, 0x46, 0x9f, 0x20, 0x48, 0x11, 0xbf, 0x3d); // WClientDRXEndDoc+ // WClientDRXEndDoc- // {743dd3cf-bbce-4e69-a4db-85226ec6a445} case EventTraceGuidId.DRXENDDOCGUID: return new Guid(0x743dd3cf, 0xbbce, 0x4e69, 0xa4, 0xdb, 0x85, 0x22, 0x6e, 0xc6, 0xa4, 0x45); // WClientDRXStartPage+ // WClientDRXEndPage- // {5303d552-28ab-4dac-8bcd-0f7d5675a157} case EventTraceGuidId.DRXSTARTPAGEGUID: return new Guid(0x5303d552, 0x28ab, 0x4dac, 0x8b, 0xcd, 0xf, 0x7d, 0x56, 0x75, 0xa1, 0x57); // WClientDRXEndPage+ // WClientDRXEndPage- // {e20fddf4-17a6-4e5f-8693-3dd7cb049422} case EventTraceGuidId.DRXENDPAGEGUID: return new Guid(0xe20fddf4, 0x17a6, 0x4e5f, 0x86, 0x93, 0x3d, 0xd7, 0xcb, 0x4, 0x94, 0x22); // WClientDRXCommitPage+ // WClientDRXCommitPage- // {7d7ee18d-aea5-493f-9ef2-bbdb36fcaa78} case EventTraceGuidId.DRXCOMMITPAGEGUID: return new Guid(0x7d7ee18d, 0xaea5, 0x493f, 0x9e, 0xf2, 0xbb, 0xdb, 0x36, 0xfc, 0xaa, 0x78); // WClientDRXConvertFont+ // WClientDRXConvertFont- // {88fc2d42-b1de-4588-8c3b-dc5bec03a9ac} case EventTraceGuidId.DRXCONVERTFONTGUID: return new Guid(0x88fc2d42, 0xb1de, 0x4588, 0x8c, 0x3b, 0xdc, 0x5b, 0xec, 0x3, 0xa9, 0xac); // WClientDRXConvertImage+ // WClientDRXConvertImage- // {17fddfdc-a1be-43b3-b2ee-f5e89b7b1b26} case EventTraceGuidId.DRXCONVERTIMAGEGUID: return new Guid(0x17fddfdc, 0xa1be, 0x43b3, 0xb2, 0xee, 0xf5, 0xe8, 0x9b, 0x7b, 0x1b, 0x26); // WClientDRXSaveXps+ // WClientDRXSaveXps- // {ba0320d5-2294-4067-8b19-ef9cddad4b1a} case EventTraceGuidId.DRXSAVEXPSGUID: return new Guid(0xba0320d5, 0x2294, 0x4067, 0x8b, 0x19, 0xef, 0x9c, 0xdd, 0xad, 0x4b, 0x1a); // WClientDRXLoadPrimitive+ // WClientDRXLoadPrimitive- // {d0b70c99-450e-4872-a2d4-fbfb1dc797fa} case EventTraceGuidId.DRXLOADPRIMITIVEGUID: return new Guid(0xd0b70c99, 0x450e, 0x4872, 0xa2, 0xd4, 0xfb, 0xfb, 0x1d, 0xc7, 0x97, 0xfa); // WClientDRXSavePage+ // WClientDRXSavePage- // {b0e3e78b-9ac7-473c-8903-b5d212399e3b} case EventTraceGuidId.DRXSAVEPAGEGUID: return new Guid(0xb0e3e78b, 0x9ac7, 0x473c, 0x89, 0x3, 0xb5, 0xd2, 0x12, 0x39, 0x9e, 0x3b); // WClientDRXSerialization+ // WClientDRXSerialization- // {0527276c-d3f4-4293-b88c-ecdf7cac4430} case EventTraceGuidId.DRXSERIALIZATIONGUID: return new Guid(0x527276c, 0xd3f4, 0x4293, 0xb8, 0x8c, 0xec, 0xdf, 0x7c, 0xac, 0x44, 0x30); // WClientPropValidate (Int32 ValidationCount) // WClientPropValidate+ (Int32 Id, String TypeAndName) // WClientPropValidate- (Int32 UsingDefault) // {e0bb1a36-6dc9-459b-ab81-b5da910e5d37} case EventTraceGuidId.PROPERTYVALIDATIONGUID: return new Guid(0xe0bb1a36, 0x6dc9, 0x459b, 0xab, 0x81, 0xb5, 0xda, 0x91, 0xe, 0x5d, 0x37); // WClientPropInvalidate (Int32 InvalidationCount) // WClientPropInvalidate+ (Int32 Id, String TypeAndName) // WClientPropInvalidate- // {61159ef2-501f-452f-8d13-51cd05f23e82} case EventTraceGuidId.PROPERTYINVALIDATIONGUID: return new Guid(0x61159ef2, 0x501f, 0x452f, 0x8d, 0x13, 0x51, 0xcd, 0x5, 0xf2, 0x3e, 0x82); // WClientPropParentCheck (Int32 Id, String TypeAndName) // {831bea07-5a2c-434c-8ef8-7eba41c881fb} case EventTraceGuidId.PROPERTYPARENTCHECKGUID: return new Guid(0x831bea07, 0x5a2c, 0x434c, 0x8e, 0xf8, 0x7e, 0xba, 0x41, 0xc8, 0x81, 0xfb); // WClientResourceFind+ (String Key) // WClientResourceFind- // {228d90d5-7e19-4480-9e56-3af2e90f8da6} case EventTraceGuidId.RESOURCEFINDGUID: return new Guid(0x228d90d5, 0x7e19, 0x4480, 0x9e, 0x56, 0x3a, 0xf2, 0xe9, 0xf, 0x8d, 0xa6); // WClientResourceCacheValue // {3b253e2d-72a5-489e-8c65-56c1e6c859b5} case EventTraceGuidId.RESOURCECACHEVALUEGUID: return new Guid(0x3b253e2d, 0x72a5, 0x489e, 0x8c, 0x65, 0x56, 0xc1, 0xe6, 0xc8, 0x59, 0xb5); // WClientResourceCacheNull // {7866a65b-2f38-43b6-abd2-df433bbca073} case EventTraceGuidId.RESOURCECACHENULLGUID: return new Guid(0x7866a65b, 0x2f38, 0x43b6, 0xab, 0xd2, 0xdf, 0x43, 0x3b, 0xbc, 0xa0, 0x73); // WClientResourceCacheMiss // {0420755f-d416-4f15-939f-3e2cd3fcea23} case EventTraceGuidId.RESOURCECACHEMISSGUID: return new Guid(0x420755f, 0xd416, 0x4f15, 0x93, 0x9f, 0x3e, 0x2c, 0xd3, 0xfc, 0xea, 0x23); // WClientResourceStock (String Key) // {06f0fee4-72dd-4802-bd3d-0985139fa91a} case EventTraceGuidId.RESOURCESTOCKGUID: return new Guid(0x6f0fee4, 0x72dd, 0x4802, 0xbd, 0x3d, 0x9, 0x85, 0x13, 0x9f, 0xa9, 0x1a); // WClientResourceBamlAssembly (String AssemblyName) // {19df4373-6680-4a04-8c77-d2f6809ca703} case EventTraceGuidId.RESOURCEBAMLASSEMBLYGUID: return new Guid(0x19df4373, 0x6680, 0x4a04, 0x8c, 0x77, 0xd2, 0xf6, 0x80, 0x9c, 0xa7, 0x3); // CreateStickyNote+ // CreateStickyNote- // {e3dbffac-1e92-4f48-a65a-c290bd5f5f15} case EventTraceGuidId.CREATESTICKYNOTEGUID: return new Guid(0xe3dbffac, 0x1e92, 0x4f48, 0xa6, 0x5a, 0xc2, 0x90, 0xbd, 0x5f, 0x5f, 0x15); // DeleteTextNote+ // DeleteTextNote- // {7626a2f9-9a61-43a3-b7cc-bb84c2493aa7} case EventTraceGuidId.DELETETEXTNOTEGUID: return new Guid(0x7626a2f9, 0x9a61, 0x43a3, 0xb7, 0xcc, 0xbb, 0x84, 0xc2, 0x49, 0x3a, 0xa7); // DeleteInkNote+ // DeleteInkNote- // {bf7e2a93-9d6a-453e-badb-3f8f60075cf2} case EventTraceGuidId.DELETEINKNOTEGUID: return new Guid(0xbf7e2a93, 0x9d6a, 0x453e, 0xba, 0xdb, 0x3f, 0x8f, 0x60, 0x07, 0x5c, 0xf2); // CreateHighlight+ // CreateHighlight- // {c2a5edb8-ac73-41ef-a943-a8a49fa284b1} case EventTraceGuidId.CREATEHIGHLIGHTGUID: return new Guid(0xc2a5edb8, 0xac73, 0x41ef, 0xa9, 0x43, 0xa8, 0xa4, 0x9f, 0xa2, 0x84, 0xb1); // ClearHighlight+ // ClearHighlight- // {e1a59147-d28d-4c5f-b980-691be2fd4208} case EventTraceGuidId.CLEARHIGHLIGHTGUID: return new Guid(0xe1a59147, 0xd28d, 0x4c5f, 0xb9, 0x80, 0x69, 0x1b, 0xe2, 0xfd, 0x42, 0x08); // LoadAnnotations+ // LoadAnnotations- // {cf3a283e-c004-4e7d-b3b9-cc9b582a4a5f} case EventTraceGuidId.LOADANNOTATIONSGUID: return new Guid(0xcf3a283e, 0xc004, 0x4e7d, 0xb3, 0xb9, 0xcc, 0x9b, 0x58, 0x2a, 0x4a, 0x5f); // UnloadAnnotations+ // UnloadAnnotations- // {1c97b272-9b6e-47b0-a470-fcf425377dcc} case EventTraceGuidId.UNLOADANNOTATIONSGUID: return new Guid(0x1c97b272, 0x9b6e, 0x47b0, 0xa4, 0x70, 0xfc, 0xf4, 0x25, 0x37, 0x7d, 0xcc); // AddAnnotation+ // AddAnnotation- // {8f4b2faa-24d6-4ee2-9935-bbf845f758a2} case EventTraceGuidId.ADDANNOTATIONGUID: return new Guid(0x8f4b2faa, 0x24d6, 0x4ee2, 0x99, 0x35, 0xbb, 0xf8, 0x45, 0xf7, 0x58, 0xa2); // DeleteAnnotation+ // DeleteAnnotation- // {4d832230-952a-4464-80af-aab2ac861703} case EventTraceGuidId.DELETEANNOTATIONGUID: return new Guid(0x4d832230, 0x952a, 0x4464, 0x80, 0xaf, 0xaa, 0xb2, 0xac, 0x86, 0x17, 0x03); // GetAnnotationById+ // GetAnnotationById- // {3d27753f-eb8a-4e75-9d5b-82fba55cded1} case EventTraceGuidId.GETANNOTATIONBYIDGUID: return new Guid(0x3d27753f, 0xeb8a, 0x4e75, 0x9d, 0x5b, 0x82, 0xfb, 0xa5, 0x5c, 0xde, 0xd1); // GetAnnotationByLoc+ // GetAnnotationByLoc- // {741a41bc-8ecd-43d1-a7f1-d2faca7362ef} case EventTraceGuidId.GETANNOTATIONBYLOCGUID: return new Guid(0x741a41bc, 0x8ecd, 0x43d1, 0xa7, 0xf1, 0xd2, 0xfa, 0xca, 0x73, 0x62, 0xef); // GetAnnotations+ // GetAnnotations- // {cd9f6017-7e64-4c61-b9ed-5c2fc8c4d849} case EventTraceGuidId.GETANNOTATIONSGUID: return new Guid(0xcd9f6017, 0x7e64, 0x4c61, 0xb9, 0xed, 0x5c, 0x2f, 0xc8, 0xc4, 0xd8, 0x49); // SerializeAnnotation+ // SerializeAnnotation- // {0148924b-5bea-43e9-b3ed-399ca13b35eb} case EventTraceGuidId.SERIALIZEANNOTATIONGUID: return new Guid(0x0148924b, 0x5bea, 0x43e9, 0xb3, 0xed, 0x39, 0x9c, 0xa1, 0x3b, 0x35, 0xeb); // DeserializeAnnotation+ // DeserializeAnnotation- // {2e32c255-d6db-4de7-9e62-9586377778d5} case EventTraceGuidId.DESERIALIZEANNOTATIONGUID: return new Guid(0x2e32c255, 0xd6db, 0x4de7, 0x9e, 0x62, 0x95, 0x86, 0x37, 0x77, 0x78, 0xd5); // UpdateAnnotationWithSNC+ // UpdateAnnotationWithSNC- // {205e0a58-3c7d-495d-b3ed-18c3fb38923f} case EventTraceGuidId.UPDATEANNOTATIONWITHSNCGUID: return new Guid(0x205e0a58, 0x3c7d, 0x495d, 0xb3, 0xed, 0x18, 0xc3, 0xfb, 0x38, 0x92, 0x3f); // UpdateSNCWithAnnotation+ // UpdateSNCWithAnnotation- // {59c337ce-9cc2-4a86-9bfa-061fe954086b} case EventTraceGuidId.UPDATESNCWITHANNOTATIONGUID: return new Guid(0x59c337ce, 0x9cc2, 0x4a86, 0x9b, 0xfa, 0x06, 0x1f, 0xe9, 0x54, 0x08, 0x6b); // AnnotationTextChanged+ // AnnotationTextChanged- // {8bb912b9-39dd-4208-ad62-be66fe5b7ba5} case EventTraceGuidId.ANNOTATIONTEXTCHANGEDGUID: return new Guid(0x8bb912b9, 0x39dd, 0x4208, 0xad, 0x62, 0xbe, 0x66, 0xfe, 0x5b, 0x7b, 0xa5); // AnnotationInkChanged+ // AnnotationInkChanged- // {1228e154-f171-426e-b672-5ee19b755edf} case EventTraceGuidId.ANNOTATIONINKCHANGEDGUID: return new Guid(0x1228e154, 0xf171, 0x426e, 0xb6, 0x72, 0x5e, 0xe1, 0x9b, 0x75, 0x5e, 0xdf); // AddAttachedSN+ // AddAttachedSN- // {9ca660f6-8d7c-4a90-a92f-74482d9cc1cf} case EventTraceGuidId.ADDATTACHEDSNGUID: return new Guid(0x9ca660f6, 0x8d7c, 0x4a90, 0xa9, 0x2f, 0x74, 0x48, 0x2d, 0x9c, 0xc1, 0xcf); // RemoveAttachedSN+ // RemoveAttachedSN- // {8c4c69f7-1185-46df-a5f5-e31ac7e96c07} case EventTraceGuidId.REMOVEATTACHEDSNGUID: return new Guid(0x8c4c69f7, 0x1185, 0x46df, 0xa5, 0xf5, 0xe3, 0x1a, 0xc7, 0xe9, 0x6c, 0x07); // AddAttachedHighlight+ // AddAttachedHighlight- // {56d2cae5-5ec0-44fb-98c2-453e87a0877b} case EventTraceGuidId.ADDATTACHEDHIGHLIGHTGUID: return new Guid(0x56d2cae5, 0x5ec0, 0x44fb, 0x98, 0xc2, 0x45, 0x3e, 0x87, 0xa0, 0x87, 0x7b); // RemoveAttachedHighlight+ // RemoveAttachedHighlight- // {4c81d490-9004-49d1-87d7-289d53a314ef} case EventTraceGuidId.REMOVEATTACHEDHIGHLIGHTGUID: return new Guid(0x4c81d490, 0x9004, 0x49d1, 0x87, 0xd7, 0x28, 0x9d, 0x53, 0xa3, 0x14, 0xef); // AddAttachedMH+ // AddAttachedMH- // {7ea1d548-ca17-ca17-a1a8-f1857db6302e} case EventTraceGuidId.ADDATTACHEDMHGUID: return new Guid(0x7ea1d548, 0xca17, 0xca17, 0xa1, 0xa8, 0xf1, 0x85, 0x7d, 0xb6, 0x30, 0x2e); // RemoveAttachedMH+ // RemoveAttachedMH- // {296c7961-b975-450b-8975-bf862b6c7159} case EventTraceGuidId.REMOVEATTACHEDMHGUID: return new Guid(0x296c7961, 0xb975, 0x450b, 0x89, 0x75, 0xbf, 0x86, 0x2b, 0x6c, 0x71, 0x59); // Defined in src\Host\Shared\Tracing.* case EventTraceGuidId.PRESENTATIONHOSTGUID: return new Guid(0xed251760, 0x7bbc, 0x4b25, 0x83, 0x28, 0xcd, 0x7f, 0x27, 0x1f, 0xee, 0x89); // The HOSTINGGUID's record format is: // HostingEvent.xx { [data0, data1, ..] } // {5FF6B585-7FB9-4189-BEB3-54C82CE4D7D1} case EventTraceGuidId.HOSTINGGUID: return new Guid(0x5ff6b585, 0x7fb9, 0x4189, 0xbe, 0xb3,0x54, 0xc8, 0x2c, 0xe4, 0xd7, 0xd1); // The NAVIGATIONGUID's record format is: // NavigationEvent.xx { [data0, data1, ..] } // {6FFB9C25-5C8A-4091-989C-5B596AB286A0} case EventTraceGuidId.NAVIGATIONGUID: return new Guid(0x6ffb9c25, 0x5c8a, 0x4091, 0x98, 0x9c, 0x5b, 0x59, 0x6a, 0xb2, 0x86, 0xa0); // {3256BDD3-A738-4d3a-9FF5-513D7F54FCA6} case EventTraceGuidId.SPLASHSCREENGUID: return new Guid(0x3256bdd3, 0xa738, 0x4d3a, 0x9f, 0xf5, 0x51, 0x3d, 0x7f, 0x54, 0xfc, 0xa6); default: return new Guid(); } } ////// The users specifies the type of information they want logged by /// passing in one of these flags when enabling tracing. This may /// change as we get more guidelines from the ETW team. /// [Flags] internal enum Flags:int { debugging = 0x00000001, performance = 0x00000002, /// Performance analysis stress = 0x00000004, security = 0x00000008, uiautomation = 0x00000010, response = 0x00000020, /// Response time tracing (benchmarks) trace = 0x00000040, PerToolSupport = 0x00000080, all = 0x7FFFFFFF } ////// Calls to the TraceEvent API specify a level of detail, which the user /// selects when enabling tracing. Setting the detail level to a higher /// level increases the number of events logged. A higher level of /// tracing includes the lower level tracing events. This is used with /// the flags to enable the right set of events. /// internal enum Level: byte { fatal = 1, // Events signalling fatal errors error = 2, // Events that should never happen warning = 3, // Infrequent events that indicate a potential problem normal = 4, // Normal level of tracing verbose = 5, // Additional information } ////// Callers use this to check if they should be logging. /// //[CodeAnalysis("AptcaMethodsShouldOnlyCallAptcaMethods")] //Tracking Bug: 29647 static internal bool IsEnabled(Flags flag, Level level) { if (EventProvider != null && ((uint)level <= EventProvider.Level) && EventProvider.IsEnabled && Convert.ToBoolean((uint)flag & EventProvider.Flags)) { return true; } else { return false; } } static internal bool IsEnabled(Flags flags) { return IsEnabled(flags, Level.normal); } ////// Internal operations associated with initializing the event provider and /// monitoring the Dispatcher and input components. /// ////// Critical: This calls critical code in TraceProvider /// TreatAsSafe: it generates the GUID that is passed into the TraceProvider /// {a42c77db-874f-422e-9b44-6d89fe2bd3e5} /// [SecurityCritical, SecurityTreatAsSafe] static EventTrace() { EventProvider = null; EventProvider = new TraceProvider("WindowsClientProvider", new Guid(0xa42c77db, 0x874f, 0x422e, 0x9b,0x44, 0x6d,0x89,0xfe,0x2b,0xd3,0xe5)); } } #if DEBUG_CLR_MEM ////// This class is taken from the CLRProfiler documentation to provide a windows client interface to /// the CLRProfiler functionality. Events are logged through the CLRProfiler It is documented in the /// CLRProfiler doc by Peter Sollich, the author of the CLRProfiler. /// /// The comments can be used in the timeline view in the CLRProfiler to determine heap usage and /// allocation behavior of sections of program execution delimited by the comments. Comments can /// be used to define allocation patterns and track object lifetimes via the CLRProfiler. /// ///sealed internal class CLRProfilerControl { [DllImport("ProfilerOBJ.dll", CharSet=CharSet.Unicode)] private static extern void LogComment(string comment); [DllImport("ProfilerOBJ.dll")] private static extern bool GetAllocationLoggingActive(); [DllImport("ProfilerOBJ.dll")] private static extern void SetAllocationLoggingActive(bool active); [DllImport("ProfilerOBJ.dll")] private static extern bool GetCallLoggingActive(); [DllImport("ProfilerOBJ.dll")] private static extern void SetCallLoggingActive(bool active); [DllImport("ProfilerOBJ.dll")] private static extern bool DumpHeap(uint timeOut); private static bool _processIsUnderCLRProfiler; private enum CLRGCStateEnum { Unset = -1, None = 0, DumpHeap = 1, Finalizers = 2} private static CLRGCStateEnum _CLRGCMode = CLRGCStateEnum.Unset; /// /// The level of logging we will do, some marks are performance, some are verbose. /// public enum CLRLogState { Unset = -1, None = 0, Performance = 1, Verbose = 2 } private static CLRLogState _CLRLogLevel = CLRLogState.Unset; ////// Static constructor to get things set up. /// static CLRProfilerControl() { try { // If AllocationLoggingActive does something, // this implies ProfilerOBJ.dll is attached // and initialized properly. bool active = GetAllocationLoggingActive(); SetAllocationLoggingActive(!active); _processIsUnderCLRProfiler = active != GetAllocationLoggingActive(); SetAllocationLoggingActive(active); // Default CLRGCMode to be done at logging comments _CLRGCMode = CLRGCStateEnum.None; // Default logging level is performance _CLRLogLevel = CLRLogState.Performance; } // If logging isn't on then CLRProfilerControl should do nothing #pragma warning disable 6502 catch (DllNotFoundException) { return; } #pragma warning restore 6502 } ////// Write the text as a comment in the log, appears as a green vertical line in the Timeline view. /// The setting of CLRGCMode determines what other actions at done BEFORE the mark. /// internal static void CLRLogWriteLine(string comment) { if (_processIsUnderCLRProfiler) { // Force a full GC heap rundown if (CLRAllocationLoggingActive && (_CLRGCMode >= CLRGCStateEnum.DumpHeap)) { CLRDumpHeap(); } LogComment(comment); } } ////// Write the text as a comment in the log, appears as a green vertical line in the Timeline view. /// The setting of CLRGCMode determines what other actions at done BEFORE the mark. /// internal static void CLRLogWriteLine(string format, params object[] args) { if (_processIsUnderCLRProfiler) { // Force a full GC heap rundown if (CLRAllocationLoggingActive && (_CLRGCMode >= CLRGCStateEnum.DumpHeap)) { CLRDumpHeap(); } LogComment(string.Format(System.Globalization.CultureInfo.CurrentCulture, format, args)); } } ////// Logging level /// public static CLRLogState CLRLoggingLevel { get { return _CLRLogLevel; } set { _CLRLogLevel = value; } } ////// Allocation logging active? /// internal static bool CLRAllocationLoggingActive { get { if (_processIsUnderCLRProfiler) { // Check this every time in case it gets turned on/off return GetAllocationLoggingActive(); } else return false; } set { if (_processIsUnderCLRProfiler) { SetAllocationLoggingActive(value); } } } ////// Is call logging active? /// internal static bool CLRCallLoggingActive { get { if (_processIsUnderCLRProfiler) { return GetCallLoggingActive(); } else { return false; } } set { if (_processIsUnderCLRProfiler) { SetCallLoggingActive(value); } } } ////// Dump the CLR Heap. If the CLRGCMode is CLRGCState.Finalizers we will /// force the finalizers to be run as well. /// internal static void CLRDumpHeap() { if (_processIsUnderCLRProfiler) { // Wait for finalizers so that we get an accurate value for live GC heap // objects when the comment is logged. if (_CLRGCMode >= CLRGCStateEnum.Finalizers) { // GC.Collect(2); GC.WaitForPendingFinalizers(); } // Timeout of 60 milliseconds. if (!DumpHeap(60*1000)) { throw new Exception("Failure to dump heap"); } } } ////// Is this process running under the CLRProfiler? /// internal static bool ProcessIsUnderCLRProfiler { get { return _processIsUnderCLRProfiler; } } } #endif // DEBUG_CLR_MEM #endregion Trace } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
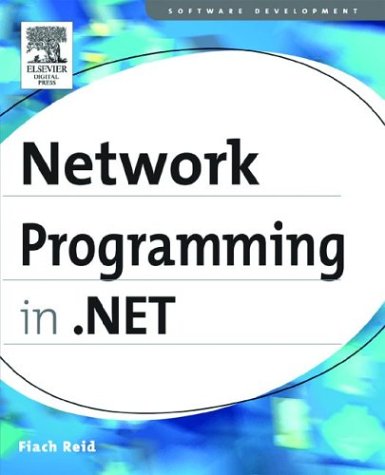
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputLangChangeRequestEvent.cs
- PatternMatcher.cs
- ChangeConflicts.cs
- DetectEofStream.cs
- GeometryValueSerializer.cs
- SQLConvert.cs
- CheckBoxField.cs
- SQLInt64Storage.cs
- ObjectViewListener.cs
- PresentationTraceSources.cs
- BitmapEffectRenderDataResource.cs
- SoapIgnoreAttribute.cs
- InvalidComObjectException.cs
- Crc32.cs
- Stack.cs
- ReadOnlyActivityGlyph.cs
- XomlCompilerHelpers.cs
- DragDeltaEventArgs.cs
- ConnectorSelectionGlyph.cs
- SchemaTableOptionalColumn.cs
- X509CertificateChain.cs
- SequenceDesigner.cs
- AuthenticationServiceManager.cs
- ContentElement.cs
- RewritingPass.cs
- BaseTemplateBuildProvider.cs
- AsyncStreamReader.cs
- ZeroOpNode.cs
- SafeLibraryHandle.cs
- IncrementalReadDecoders.cs
- SemaphoreFullException.cs
- returneventsaver.cs
- BuildManager.cs
- IndexExpression.cs
- SemanticValue.cs
- TcpTransportBindingElement.cs
- CollectionContainer.cs
- GraphicsContext.cs
- InvalidPropValue.cs
- ContentPlaceHolderDesigner.cs
- TextEffect.cs
- TextDecorationCollection.cs
- FileLevelControlBuilderAttribute.cs
- ToolboxComponentsCreatingEventArgs.cs
- EventBuilder.cs
- TraversalRequest.cs
- TransformProviderWrapper.cs
- AddingNewEventArgs.cs
- UserValidatedEventArgs.cs
- ObjectTokenCategory.cs
- SqlHelper.cs
- MessageBodyDescription.cs
- ContextProperty.cs
- StringSource.cs
- TogglePattern.cs
- RecognitionResult.cs
- ImagingCache.cs
- ServiceDescriptionContext.cs
- DiscriminatorMap.cs
- SynchronizedDispatch.cs
- RegexMatch.cs
- HttpRequest.cs
- StructuredTypeInfo.cs
- ConfigurationManager.cs
- ListParagraph.cs
- _DomainName.cs
- CompoundFileDeflateTransform.cs
- DockingAttribute.cs
- MessageVersionConverter.cs
- ListBoxItemWrapperAutomationPeer.cs
- CacheMemory.cs
- FontCacheLogic.cs
- ByeOperationCD1AsyncResult.cs
- HttpProfileBase.cs
- _AutoWebProxyScriptWrapper.cs
- RuntimeCompatibilityAttribute.cs
- FindProgressChangedEventArgs.cs
- Content.cs
- LingerOption.cs
- ProfileBuildProvider.cs
- FixedFindEngine.cs
- ContentOperations.cs
- EdmSchemaAttribute.cs
- FormViewDeleteEventArgs.cs
- FigureHelper.cs
- XmlBaseReader.cs
- XmlSubtreeReader.cs
- FastPropertyAccessor.cs
- VersionConverter.cs
- counter.cs
- ControlsConfig.cs
- RuleSettingsCollection.cs
- DeflateEmulationStream.cs
- XmlEncoding.cs
- ColumnResizeUndoUnit.cs
- ButtonFlatAdapter.cs
- TableCellCollection.cs
- ToolStripComboBox.cs
- ControlSerializer.cs
- SqlFactory.cs