Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Automation / Peers / GenericRootAutomationPeer.cs / 1 / GenericRootAutomationPeer.cs
using System; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Text; using System.Windows; using System.Windows.Interop; using System.Windows.Media; using System.ComponentModel; using MS.Internal; using MS.Win32; // Used to support the warnings disabled below #pragma warning disable 1634, 1691 namespace System.Windows.Automation.Peers { /// public class GenericRootAutomationPeer : UIElementAutomationPeer { /// public GenericRootAutomationPeer(UIElement owner): base(owner) {} /// override protected string GetClassNameCore() { return "Pane"; } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Pane; } /// ////// Critical: As this accesses Handle /// TreatAsSafe: Returning the Window Title is considered safe - discussed on Automation TA review /// [SecurityCritical,SecurityTreatAsSafe] override protected string GetNameCore() { string name = base.GetNameCore(); if(name == string.Empty) { IntPtr hwnd = this.Hwnd; if(hwnd != IntPtr.Zero) { try { StringBuilder sb = new StringBuilder(512); //This method elevates via SuppressUnmanadegCodeSecurity and throws Win32Exception on GetLastError UnsafeNativeMethods.GetWindowText(new HandleRef(null, hwnd), sb, sb.Capacity); name = sb.ToString(); } // Allow empty catch statements. #pragma warning disable 56502 catch(Win32Exception) {} // Disallow empty catch statements. #pragma warning restore 56502 if (name == null) name = string.Empty; } } return name; } /// ////// Critical as this method accesses critical data. /// TreatAsSafe - window bounds by themselves is considered safe. /// [SecurityCritical, SecurityTreatAsSafe ] override protected Rect GetBoundingRectangleCore() { Rect bounds = new Rect(0,0,0,0); IntPtr hwnd = this.Hwnd; if(hwnd != IntPtr.Zero) { NativeMethods.RECT rc = new NativeMethods.RECT(0,0,0,0); try { //This method elevates via SuppressUnmanadegCodeSecurity and throws Win32Exception on GetLastError SafeNativeMethods.GetWindowRect(new HandleRef(null, hwnd), ref rc); } // Allow empty catch statements. #pragma warning disable 56502 catch(Win32Exception) {} // Disallow empty catch statements. #pragma warning restore 56502 bounds = new Rect(rc.left, rc.top, rc.right - rc.left, rc.bottom - rc.top); } return bounds; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Text; using System.Windows; using System.Windows.Interop; using System.Windows.Media; using System.ComponentModel; using MS.Internal; using MS.Win32; // Used to support the warnings disabled below #pragma warning disable 1634, 1691 namespace System.Windows.Automation.Peers { /// public class GenericRootAutomationPeer : UIElementAutomationPeer { /// public GenericRootAutomationPeer(UIElement owner): base(owner) {} /// override protected string GetClassNameCore() { return "Pane"; } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Pane; } /// ////// Critical: As this accesses Handle /// TreatAsSafe: Returning the Window Title is considered safe - discussed on Automation TA review /// [SecurityCritical,SecurityTreatAsSafe] override protected string GetNameCore() { string name = base.GetNameCore(); if(name == string.Empty) { IntPtr hwnd = this.Hwnd; if(hwnd != IntPtr.Zero) { try { StringBuilder sb = new StringBuilder(512); //This method elevates via SuppressUnmanadegCodeSecurity and throws Win32Exception on GetLastError UnsafeNativeMethods.GetWindowText(new HandleRef(null, hwnd), sb, sb.Capacity); name = sb.ToString(); } // Allow empty catch statements. #pragma warning disable 56502 catch(Win32Exception) {} // Disallow empty catch statements. #pragma warning restore 56502 if (name == null) name = string.Empty; } } return name; } /// ////// Critical as this method accesses critical data. /// TreatAsSafe - window bounds by themselves is considered safe. /// [SecurityCritical, SecurityTreatAsSafe ] override protected Rect GetBoundingRectangleCore() { Rect bounds = new Rect(0,0,0,0); IntPtr hwnd = this.Hwnd; if(hwnd != IntPtr.Zero) { NativeMethods.RECT rc = new NativeMethods.RECT(0,0,0,0); try { //This method elevates via SuppressUnmanadegCodeSecurity and throws Win32Exception on GetLastError SafeNativeMethods.GetWindowRect(new HandleRef(null, hwnd), ref rc); } // Allow empty catch statements. #pragma warning disable 56502 catch(Win32Exception) {} // Disallow empty catch statements. #pragma warning restore 56502 bounds = new Rect(rc.left, rc.top, rc.right - rc.left, rc.bottom - rc.top); } return bounds; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
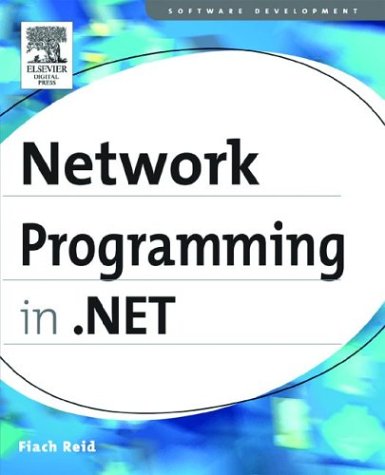
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfileSection.cs
- UserPreferenceChangedEventArgs.cs
- PropertyDescriptor.cs
- PipelineModuleStepContainer.cs
- WebPartEditVerb.cs
- Window.cs
- DataBindEngine.cs
- OdbcPermission.cs
- _AutoWebProxyScriptEngine.cs
- WebPartManager.cs
- WebDisplayNameAttribute.cs
- cookiecontainer.cs
- Mappings.cs
- Action.cs
- WinHttpWebProxyFinder.cs
- ScriptingJsonSerializationSection.cs
- MiniConstructorInfo.cs
- CodeMemberEvent.cs
- XamlPathDataSerializer.cs
- ObfuscateAssemblyAttribute.cs
- WebContext.cs
- BitmapDownload.cs
- VisualProxy.cs
- PointHitTestParameters.cs
- HttpInputStream.cs
- MachineKeySection.cs
- arclist.cs
- ReadOnlyDataSourceView.cs
- BorderSidesEditor.cs
- TextParentUndoUnit.cs
- TextSimpleMarkerProperties.cs
- CmsInterop.cs
- CurrencyManager.cs
- RelationshipConverter.cs
- TcpPortSharing.cs
- UnsafeNativeMethods.cs
- GeneralTransform3DTo2DTo3D.cs
- ProtocolsConfigurationHandler.cs
- RelationshipManager.cs
- ToolStripItemDataObject.cs
- SspiNegotiationTokenAuthenticator.cs
- FontDifferentiator.cs
- AudioSignalProblemOccurredEventArgs.cs
- NeutralResourcesLanguageAttribute.cs
- AutoGeneratedField.cs
- MessageContractMemberAttribute.cs
- TypeSystemHelpers.cs
- OptimalBreakSession.cs
- BatchParser.cs
- ListViewUpdatedEventArgs.cs
- ConfigsHelper.cs
- Win32Native.cs
- CellCreator.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- SerializerDescriptor.cs
- MethodBuilder.cs
- Typeface.cs
- EntryWrittenEventArgs.cs
- ServiceDescriptionSerializer.cs
- QuaternionKeyFrameCollection.cs
- WindowCollection.cs
- ServiceDescriptionContext.cs
- GridItemProviderWrapper.cs
- ReadWriteObjectLock.cs
- InternalRelationshipCollection.cs
- SurrogateEncoder.cs
- WebControlsSection.cs
- SafeRightsManagementSessionHandle.cs
- WmlCommandAdapter.cs
- WebPartDescription.cs
- GroupItemAutomationPeer.cs
- ProfilePropertySettingsCollection.cs
- ToolStripDropDownButton.cs
- HashMembershipCondition.cs
- CodeIdentifiers.cs
- ObjectDataSourceFilteringEventArgs.cs
- DataSetUtil.cs
- SoapCodeExporter.cs
- ContainerParaClient.cs
- DataGridViewLayoutData.cs
- TargetInvocationException.cs
- RowsCopiedEventArgs.cs
- SettingsSavedEventArgs.cs
- TextProperties.cs
- BinaryFormatterWriter.cs
- Compiler.cs
- GeometryCollection.cs
- _TLSstream.cs
- BaseCodePageEncoding.cs
- SystemIPGlobalProperties.cs
- EntityStoreSchemaGenerator.cs
- Material.cs
- Axis.cs
- TextRangeEditLists.cs
- ProvidersHelper.cs
- WebPartActionVerb.cs
- DocumentViewerAutomationPeer.cs
- Subset.cs
- FileDialog.cs
- ObjectDataSourceView.cs