Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Configuration / ProfileSection.cs / 1305376 / ProfileSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Security.Permissions; /**/ public sealed class ProfileSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnabled = new ConfigurationProperty("enabled", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDefaultProvider = new ConfigurationProperty("defaultProvider", typeof(string), "AspNetSqlProfileProvider", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProviders = new ConfigurationProperty("providers", typeof(ProviderSettingsCollection), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProfile = new ConfigurationProperty("properties", typeof(RootProfilePropertySettingsCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propInherits = new ConfigurationProperty("inherits", typeof(string), String.Empty, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propAutomaticSaveEnabled = new ConfigurationProperty("automaticSaveEnabled", typeof(bool), true, ConfigurationPropertyOptions.None); static ProfileSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnabled); _properties.Add(_propDefaultProvider); _properties.Add(_propProviders); _properties.Add(_propProfile); _properties.Add(_propInherits); _properties.Add(_propAutomaticSaveEnabled); } private long _recompilationHash; private bool _recompilationHashCached; internal long RecompilationHash { get { if (!_recompilationHashCached) { _recompilationHash = CalculateHash(); _recompilationHashCached = true; } return _recompilationHash; } } private long CalculateHash() { HashCodeCombiner hashCombiner = new HashCodeCombiner(); CalculateProfilePropertySettingsHash(PropertySettings, hashCombiner); if (PropertySettings != null) { foreach (ProfileGroupSettings pgs in PropertySettings.GroupSettings) { hashCombiner.AddObject(pgs.Name); CalculateProfilePropertySettingsHash(pgs.PropertySettings, hashCombiner); } } return hashCombiner.CombinedHash; } private void CalculateProfilePropertySettingsHash( ProfilePropertySettingsCollection settings, HashCodeCombiner hashCombiner) { foreach (ProfilePropertySettings pps in settings) { hashCombiner.AddObject(pps.Name); hashCombiner.AddObject(pps.Type); } } public ProfileSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("automaticSaveEnabled", DefaultValue = true)] public bool AutomaticSaveEnabled { get { return (bool)base[_propAutomaticSaveEnabled]; } set { base[_propAutomaticSaveEnabled] = value; } } [ConfigurationProperty("enabled", DefaultValue = true)] public bool Enabled { get { return (bool)base[_propEnabled]; } set { base[_propEnabled] = value; } } [ConfigurationProperty("defaultProvider", DefaultValue = "AspNetSqlProfileProvider")] [StringValidator(MinLength = 1)] public string DefaultProvider { get { return (string)base[_propDefaultProvider]; } set { base[_propDefaultProvider] = value; } } [ConfigurationProperty("inherits", DefaultValue = "")] public string Inherits { get { return (string)base[_propInherits]; } set { base[_propInherits] = value; } } [ConfigurationProperty("providers")] public ProviderSettingsCollection Providers { get { return (ProviderSettingsCollection)base[_propProviders]; } } // not exposed to the API [ConfigurationProperty("properties")] public RootProfilePropertySettingsCollection PropertySettings { get { return (RootProfilePropertySettingsCollection)base[_propProfile]; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Security.Permissions; /**/ public sealed class ProfileSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnabled = new ConfigurationProperty("enabled", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDefaultProvider = new ConfigurationProperty("defaultProvider", typeof(string), "AspNetSqlProfileProvider", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProviders = new ConfigurationProperty("providers", typeof(ProviderSettingsCollection), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProfile = new ConfigurationProperty("properties", typeof(RootProfilePropertySettingsCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propInherits = new ConfigurationProperty("inherits", typeof(string), String.Empty, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propAutomaticSaveEnabled = new ConfigurationProperty("automaticSaveEnabled", typeof(bool), true, ConfigurationPropertyOptions.None); static ProfileSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnabled); _properties.Add(_propDefaultProvider); _properties.Add(_propProviders); _properties.Add(_propProfile); _properties.Add(_propInherits); _properties.Add(_propAutomaticSaveEnabled); } private long _recompilationHash; private bool _recompilationHashCached; internal long RecompilationHash { get { if (!_recompilationHashCached) { _recompilationHash = CalculateHash(); _recompilationHashCached = true; } return _recompilationHash; } } private long CalculateHash() { HashCodeCombiner hashCombiner = new HashCodeCombiner(); CalculateProfilePropertySettingsHash(PropertySettings, hashCombiner); if (PropertySettings != null) { foreach (ProfileGroupSettings pgs in PropertySettings.GroupSettings) { hashCombiner.AddObject(pgs.Name); CalculateProfilePropertySettingsHash(pgs.PropertySettings, hashCombiner); } } return hashCombiner.CombinedHash; } private void CalculateProfilePropertySettingsHash( ProfilePropertySettingsCollection settings, HashCodeCombiner hashCombiner) { foreach (ProfilePropertySettings pps in settings) { hashCombiner.AddObject(pps.Name); hashCombiner.AddObject(pps.Type); } } public ProfileSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("automaticSaveEnabled", DefaultValue = true)] public bool AutomaticSaveEnabled { get { return (bool)base[_propAutomaticSaveEnabled]; } set { base[_propAutomaticSaveEnabled] = value; } } [ConfigurationProperty("enabled", DefaultValue = true)] public bool Enabled { get { return (bool)base[_propEnabled]; } set { base[_propEnabled] = value; } } [ConfigurationProperty("defaultProvider", DefaultValue = "AspNetSqlProfileProvider")] [StringValidator(MinLength = 1)] public string DefaultProvider { get { return (string)base[_propDefaultProvider]; } set { base[_propDefaultProvider] = value; } } [ConfigurationProperty("inherits", DefaultValue = "")] public string Inherits { get { return (string)base[_propInherits]; } set { base[_propInherits] = value; } } [ConfigurationProperty("providers")] public ProviderSettingsCollection Providers { get { return (ProviderSettingsCollection)base[_propProviders]; } } // not exposed to the API [ConfigurationProperty("properties")] public RootProfilePropertySettingsCollection PropertySettings { get { return (RootProfilePropertySettingsCollection)base[_propProfile]; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
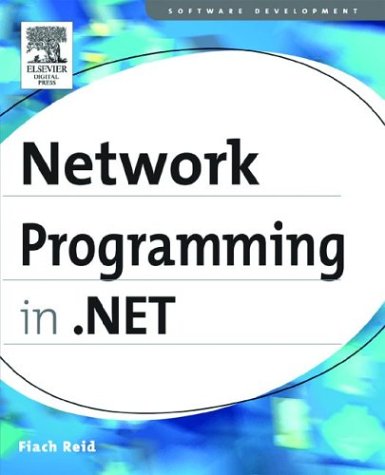
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpStreamMessageEncoderFactory.cs
- StrongNamePublicKeyBlob.cs
- BuildProviderCollection.cs
- TextBoxView.cs
- GetWinFXPath.cs
- SingleResultAttribute.cs
- FilterQueryOptionExpression.cs
- CodePropertyReferenceExpression.cs
- EventProviderTraceListener.cs
- GAC.cs
- HtmlInputPassword.cs
- CompiledRegexRunner.cs
- LoginDesigner.cs
- HostSecurityManager.cs
- Point4DValueSerializer.cs
- WriterOutput.cs
- SpinWait.cs
- CounterNameConverter.cs
- InkCanvasFeedbackAdorner.cs
- StylusPointPropertyId.cs
- AlignmentYValidation.cs
- MembershipPasswordException.cs
- _OSSOCK.cs
- AjaxFrameworkAssemblyAttribute.cs
- PlatformNotSupportedException.cs
- SpecialNameAttribute.cs
- SafeTimerHandle.cs
- RemotingAttributes.cs
- DefaultParameterValueAttribute.cs
- WebSysDefaultValueAttribute.cs
- ReflectTypeDescriptionProvider.cs
- QueryParameter.cs
- DetailsViewRow.cs
- Codec.cs
- OleDbCommandBuilder.cs
- SelectorItemAutomationPeer.cs
- ConditionBrowserDialog.cs
- COSERVERINFO.cs
- MenuItemBinding.cs
- WebErrorHandler.cs
- XPathNodePointer.cs
- ControllableStoryboardAction.cs
- SHA384.cs
- XmlCharCheckingWriter.cs
- DynamicFilter.cs
- PersistenceTypeAttribute.cs
- SafeNativeMethods.cs
- GatewayDefinition.cs
- CatalogZone.cs
- MetadataItem.cs
- HttpCapabilitiesSectionHandler.cs
- LicenseProviderAttribute.cs
- SplineKeyFrames.cs
- AuthenticationManager.cs
- Win32SafeHandles.cs
- HostingEnvironmentWrapper.cs
- DataGridViewColumnConverter.cs
- InternalPolicyElement.cs
- WebRequest.cs
- OleDbConnection.cs
- SqlProvider.cs
- WmfPlaceableFileHeader.cs
- TrackingMemoryStream.cs
- DeferredTextReference.cs
- GestureRecognitionResult.cs
- AuthorizationRuleCollection.cs
- SemaphoreSecurity.cs
- TaskFormBase.cs
- SchemaAttDef.cs
- FileSecurity.cs
- ClientSideQueueItem.cs
- CompilerErrorCollection.cs
- DataGridViewSortCompareEventArgs.cs
- TextDecoration.cs
- PublisherMembershipCondition.cs
- XmlSyndicationContent.cs
- ValidationManager.cs
- TextShapeableCharacters.cs
- WebScriptServiceHost.cs
- MarshalByValueComponent.cs
- XmlSignificantWhitespace.cs
- XmlConvert.cs
- FilterQueryOptionExpression.cs
- UDPClient.cs
- SourceItem.cs
- IpcClientManager.cs
- UIElement3D.cs
- UInt16Storage.cs
- SessionParameter.cs
- ConfigurationCollectionAttribute.cs
- EncodingTable.cs
- JsonCollectionDataContract.cs
- PeerCustomResolverElement.cs
- WindowsFont.cs
- DynamicPropertyReader.cs
- CanonicalFontFamilyReference.cs
- Context.cs
- ServiceReflector.cs
- MetafileEditor.cs
- HealthMonitoringSectionHelper.cs