Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / IO / Packaging / PackageRelationshipCollection.cs / 1 / PackageRelationshipCollection.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a class for representing a PackageRelationshipCollection. This is a part of the // MMCF Packaging Layer. // // Details: // This class handles serialization to/from relationship parts, creation of those parts // and offers methods to create, delete and enumerate relationships. // // History: // 01/03/2004: SarjanaS: Initial creation. // 03/17/2004: BruceMac: Initial implementation // 04/26/2004: Sarjanas: Refactored some of the code to InternalRelationshipCollection.cs // and added support for filtered relationships // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; // For Exception strings - SRID using System.Collections.Generic; using System.Diagnostics; // For Debug.Assert using MS.Internal.IO.Packaging; namespace System.IO.Packaging { ////// Collection of all the relationships corresponding to a given source PackagePart /// public class PackageRelationshipCollection : IEnumerable{ //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region IEnumerable /// /// Returns an enumerator for all the relationships for a PackagePart /// ///IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// /// Returns an enumerator over all the relationships for a PackagePart /// ///public IEnumerator GetEnumerator() { List .Enumerator relationshipsEnumerator = _relationships.GetEnumerator(); if (_filter == null) return relationshipsEnumerator; else return new FilteredEnumerator(relationshipsEnumerator, _filter); } #endregion //------------------------------------------------------ // // Internal Members // //----------------------------------------------------- #region Internal Members /// /// Constructor /// ///For use by PackagePart internal PackageRelationshipCollection(InternalRelationshipCollection relationships, string filter) { Debug.Assert(relationships != null, "relationships parameter cannot be null"); _relationships = relationships; _filter = filter; } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ // None //----------------------------------------------------- // // Private Members // //------------------------------------------------------ #region Private Members private InternalRelationshipCollection _relationships; private string _filter; #endregion #region Private Class #region FilteredEnumerator Class ////// Internal class for the FilteredEnumerator /// private sealed class FilteredEnumerator : IEnumerator{ #region Constructor /// /// Constructs a FilteredEnumerator /// /// /// internal FilteredEnumerator(IEnumeratorenumerator, string filter) { Debug.Assert((enumerator != null), "Enumerator cannot be null"); Debug.Assert(filter != null, "PackageRelationship filter string cannot be null"); // Look for empty string or string with just spaces Debug.Assert(filter.Trim() != String.Empty, "RelationshipType filter cannot be empty string or a string with just spaces"); _enumerator = enumerator; _filter = filter; } #endregion Constructor #region IEnumerator Methods /// /// This method keeps moving the enumerator the the next position till /// a relationship is found with the matching Name /// ///Bool indicating if the enumerator successfully moved to the next position bool IEnumerator.MoveNext() { while (_enumerator.MoveNext()) { if (RelationshipTypeMatches()) return true; } return false; } ////// Gets the current object in the enumerator /// ///Object IEnumerator.Current { get { return _enumerator.Current; } } /// /// Resets the enumerator to the begining /// void IEnumerator.Reset() { _enumerator.Reset(); } #endregion IEnumerator Methods #region IEnumeratorMembers /// /// Gets the current object in the enumerator /// ///public PackageRelationship Current { get { return _enumerator.Current; } } #endregion IEnumerator Members #region IDisposable Members public void Dispose() { //Most enumerators have dispose as a no-op, we follow the same pattern. _enumerator.Dispose(); } #endregion IDisposable Members #region Private Methods private bool RelationshipTypeMatches() { PackageRelationship r = _enumerator.Current; //Case-sensitive comparison if (String.CompareOrdinal(r.RelationshipType, _filter)==0) return true; else return false; } #endregion Private Methods #region Private Members private IEnumerator _enumerator; private string _filter; #endregion Private Members } #endregion FilteredEnumerator Class #endregion Private Class } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a class for representing a PackageRelationshipCollection. This is a part of the // MMCF Packaging Layer. // // Details: // This class handles serialization to/from relationship parts, creation of those parts // and offers methods to create, delete and enumerate relationships. // // History: // 01/03/2004: SarjanaS: Initial creation. // 03/17/2004: BruceMac: Initial implementation // 04/26/2004: Sarjanas: Refactored some of the code to InternalRelationshipCollection.cs // and added support for filtered relationships // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; // For Exception strings - SRID using System.Collections.Generic; using System.Diagnostics; // For Debug.Assert using MS.Internal.IO.Packaging; namespace System.IO.Packaging { ////// Collection of all the relationships corresponding to a given source PackagePart /// public class PackageRelationshipCollection : IEnumerable{ //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region IEnumerable /// /// Returns an enumerator for all the relationships for a PackagePart /// ///IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// /// Returns an enumerator over all the relationships for a PackagePart /// ///public IEnumerator GetEnumerator() { List .Enumerator relationshipsEnumerator = _relationships.GetEnumerator(); if (_filter == null) return relationshipsEnumerator; else return new FilteredEnumerator(relationshipsEnumerator, _filter); } #endregion //------------------------------------------------------ // // Internal Members // //----------------------------------------------------- #region Internal Members /// /// Constructor /// ///For use by PackagePart internal PackageRelationshipCollection(InternalRelationshipCollection relationships, string filter) { Debug.Assert(relationships != null, "relationships parameter cannot be null"); _relationships = relationships; _filter = filter; } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ // None //----------------------------------------------------- // // Private Members // //------------------------------------------------------ #region Private Members private InternalRelationshipCollection _relationships; private string _filter; #endregion #region Private Class #region FilteredEnumerator Class ////// Internal class for the FilteredEnumerator /// private sealed class FilteredEnumerator : IEnumerator{ #region Constructor /// /// Constructs a FilteredEnumerator /// /// /// internal FilteredEnumerator(IEnumeratorenumerator, string filter) { Debug.Assert((enumerator != null), "Enumerator cannot be null"); Debug.Assert(filter != null, "PackageRelationship filter string cannot be null"); // Look for empty string or string with just spaces Debug.Assert(filter.Trim() != String.Empty, "RelationshipType filter cannot be empty string or a string with just spaces"); _enumerator = enumerator; _filter = filter; } #endregion Constructor #region IEnumerator Methods /// /// This method keeps moving the enumerator the the next position till /// a relationship is found with the matching Name /// ///Bool indicating if the enumerator successfully moved to the next position bool IEnumerator.MoveNext() { while (_enumerator.MoveNext()) { if (RelationshipTypeMatches()) return true; } return false; } ////// Gets the current object in the enumerator /// ///Object IEnumerator.Current { get { return _enumerator.Current; } } /// /// Resets the enumerator to the begining /// void IEnumerator.Reset() { _enumerator.Reset(); } #endregion IEnumerator Methods #region IEnumeratorMembers /// /// Gets the current object in the enumerator /// ///public PackageRelationship Current { get { return _enumerator.Current; } } #endregion IEnumerator Members #region IDisposable Members public void Dispose() { //Most enumerators have dispose as a no-op, we follow the same pattern. _enumerator.Dispose(); } #endregion IDisposable Members #region Private Methods private bool RelationshipTypeMatches() { PackageRelationship r = _enumerator.Current; //Case-sensitive comparison if (String.CompareOrdinal(r.RelationshipType, _filter)==0) return true; else return false; } #endregion Private Methods #region Private Members private IEnumerator _enumerator; private string _filter; #endregion Private Members } #endregion FilteredEnumerator Class #endregion Private Class } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
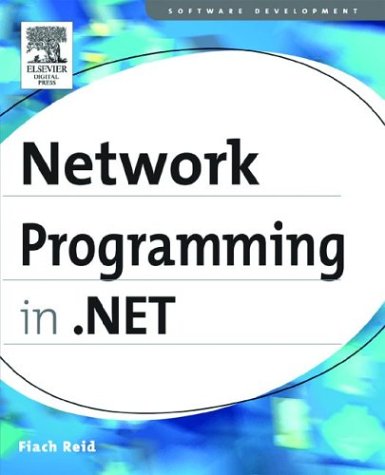
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PathSegment.cs
- VisualTransition.cs
- Events.cs
- Triplet.cs
- ColorInterpolationModeValidation.cs
- EntityDataSourceUtil.cs
- ScriptResourceAttribute.cs
- GeneralTransform3DTo2DTo3D.cs
- WebMessageEncodingElement.cs
- COM2Enum.cs
- ImageInfo.cs
- WorkflowPersistenceService.cs
- BevelBitmapEffect.cs
- StringUtil.cs
- DataGridPageChangedEventArgs.cs
- BackgroundFormatInfo.cs
- SQLRoleProvider.cs
- BrushProxy.cs
- RecognitionResult.cs
- XmlWrappingWriter.cs
- XmlMessageFormatter.cs
- URL.cs
- SchemaElementDecl.cs
- EndEvent.cs
- WsdlInspector.cs
- SspiWrapper.cs
- TerminatorSinks.cs
- HostAdapter.cs
- Bitmap.cs
- Hyperlink.cs
- DoubleIndependentAnimationStorage.cs
- StronglyTypedResourceBuilder.cs
- DetailsViewModeEventArgs.cs
- TaskFileService.cs
- __FastResourceComparer.cs
- unitconverter.cs
- QuestionEventArgs.cs
- ListViewItem.cs
- ConfigurationException.cs
- SmtpNetworkElement.cs
- Cursors.cs
- DbTransaction.cs
- XsltFunctions.cs
- LZCodec.cs
- PropertyPath.cs
- ToolStripProgressBar.cs
- WmlPageAdapter.cs
- EncodingDataItem.cs
- PlacementWorkspace.cs
- IsolatedStorageFileStream.cs
- _DisconnectOverlappedAsyncResult.cs
- TableItemStyle.cs
- ModelServiceImpl.cs
- ValueQuery.cs
- __Filters.cs
- WebPageTraceListener.cs
- RtType.cs
- DispatcherProcessingDisabled.cs
- ObjectViewListener.cs
- KeyboardNavigation.cs
- CodeParameterDeclarationExpressionCollection.cs
- BulletedListEventArgs.cs
- itemelement.cs
- AutomationPeer.cs
- NameTable.cs
- MarkupObject.cs
- RoleManagerSection.cs
- HMACSHA384.cs
- SecurityAlgorithmSuite.cs
- WinHttpWebProxyFinder.cs
- XMLSyntaxException.cs
- ColumnResizeAdorner.cs
- ColorAnimation.cs
- EmptyStringExpandableObjectConverter.cs
- UIAgentAsyncBeginRequest.cs
- DateTimeConverter2.cs
- SQLGuid.cs
- Menu.cs
- Int16.cs
- LogReservationCollection.cs
- GridItemProviderWrapper.cs
- Section.cs
- CLRBindingWorker.cs
- Storyboard.cs
- SQLInt32.cs
- _DigestClient.cs
- DataBoundControlHelper.cs
- COM2Enum.cs
- SQLInt32.cs
- ClientUIRequest.cs
- CodeIterationStatement.cs
- XmlSecureResolver.cs
- WindowsTokenRoleProvider.cs
- InkCanvasSelection.cs
- ZipPackagePart.cs
- XmlSchemaSubstitutionGroup.cs
- NegatedConstant.cs
- HttpDebugHandler.cs
- LinqDataSourceInsertEventArgs.cs
- SqlTypeSystemProvider.cs