Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / Hosting / AppDomainFactory.cs / 2 / AppDomainFactory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * AppDomain factory -- creates app domains on demand from ISAPI * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.Hosting { using System.Collections; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Text; using System.Web; using System.Web.Util; // // IAppDomainFactory / AppDomainFactory are obsolete and stay public // only to avoid breaking changes. // // The new code uses IAppManagerAppDomainFactory / AppAppManagerDomainFactory // ///[ComImport, Guid("e6e21054-a7dc-4378-877d-b7f4a2d7e8ba"), System.Runtime.InteropServices.InterfaceTypeAttribute(System.Runtime.InteropServices.ComInterfaceType.InterfaceIsIUnknown)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public interface IAppDomainFactory { #if !FEATURE_PAL // FEATURE_PAL does not enable COM [return: MarshalAs(UnmanagedType.Interface)] Object Create( [In, MarshalAs(UnmanagedType.BStr)] String module, [In, MarshalAs(UnmanagedType.BStr)] String typeName, [In, MarshalAs(UnmanagedType.BStr)] String appId, [In, MarshalAs(UnmanagedType.BStr)] String appPath, [In, MarshalAs(UnmanagedType.BStr)] String strUrlOfAppOrigin, [In, MarshalAs(UnmanagedType.I4)] int iZone); #else // !FEATURE_PAL [return: MarshalAs(UnmanagedType.Error)] Object Create( [In, MarshalAs(UnmanagedType.Error)] String module, [In, MarshalAs(UnmanagedType.Error)] String typeName, [In, MarshalAs(UnmanagedType.Error)] String appId, [In, MarshalAs(UnmanagedType.Error)] String appPath, [In, MarshalAs(UnmanagedType.Error)] String strUrlOfAppOrigin, [In, MarshalAs(UnmanagedType.I4)] int iZone); #endif // FEATURE_PAL } /// /// ///[To be supplied.] ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class AppDomainFactory : IAppDomainFactory { private AppManagerAppDomainFactory _realFactory; [SecurityPermission(SecurityAction.Demand, Unrestricted=true)] [AspNetHostingPermission(SecurityAction.Demand, Level=AspNetHostingPermissionLevel.Minimal)] public AppDomainFactory() { _realFactory = new AppManagerAppDomainFactory(); } /* * Creates an app domain with an object inside */ #if !FEATURE_PAL // FEATURE_PAL does not enable COM [return: MarshalAs(UnmanagedType.Interface)] #endif // !FEATURE_PAL public Object Create(String module, String typeName, String appId, String appPath, String strUrlOfAppOrigin, int iZone) { return _realFactory.Create(appId, appPath); } } // // The new code -- IAppManagerAppDomainFactory / AppAppManagerDomainFactory // /// [ComImport, Guid("02998279-7175-4d59-aa5a-fb8e44d4ca9d"), System.Runtime.InteropServices.InterfaceTypeAttribute(System.Runtime.InteropServices.ComInterfaceType.InterfaceIsIUnknown)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public interface IAppManagerAppDomainFactory { #if !FEATURE_PAL // FEATURE_PAL does not enable COM [return: MarshalAs(UnmanagedType.Interface)] #else // !FEATURE_PAL Object Create(String appId, String appPath); #endif // !FEATURE_PAL Object Create([In, MarshalAs(UnmanagedType.BStr)] String appId, [In, MarshalAs(UnmanagedType.BStr)] String appPath); void Stop(); } /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class AppManagerAppDomainFactory : IAppManagerAppDomainFactory { private ApplicationManager _appManager; [SecurityPermission(SecurityAction.Demand, Unrestricted=true)] [AspNetHostingPermission(SecurityAction.Demand, Level=AspNetHostingPermissionLevel.Minimal)] public AppManagerAppDomainFactory() { _appManager = ApplicationManager.GetApplicationManager(); _appManager.Open(); } /* * Creates an app domain with an object inside */ #if FEATURE_PAL // FEATURE_PAL does not enable COM [return: MarshalAs(UnmanagedType.Error)] #else // FEATURE_PAL [return: MarshalAs(UnmanagedType.Interface)] #endif // FEATURE_PAL public Object Create(String appId, String appPath) { try { // // Fill app a Dictionary with 'binding rules' -- name value string pairs // for app domain creation // // if (appPath[0] == '.') { System.IO.FileInfo file = new System.IO.FileInfo(appPath); appPath = file.FullName; } if (!StringUtil.StringEndsWith(appPath, '\\')) { appPath = appPath + "\\"; } // Create new app domain via App Manager #if FEATURE_PAL // FEATURE_PAL does not enable IIS-based hosting features throw new NotImplementedException("ROTORTODO"); #else // FEATURE_PAL ISAPIApplicationHost appHost = new ISAPIApplicationHost(appId, appPath, false /*validatePhysicalPath*/); ISAPIRuntime isapiRuntime = (ISAPIRuntime)_appManager.CreateObjectInternal(appId, typeof(ISAPIRuntime), appHost, false /*failIfExists*/, null /*hostingParameters*/); isapiRuntime.StartProcessing(); return new ObjectHandle(isapiRuntime); #endif // FEATURE_PAL } catch (Exception e) { Debug.Trace("internal", "AppDomainFactory::Create failed with " + e.GetType().FullName + ": " + e.Message + "\r\n" + e.StackTrace); throw; } } public void Stop() { // wait for all app domains to go away _appManager.Close(); } internal static String ConstructSimpleAppName(string virtPath) { if (virtPath.Length <= 1) // root? return "root"; else return virtPath.Substring(1).ToLower(CultureInfo.InvariantCulture).Replace('/', '_'); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
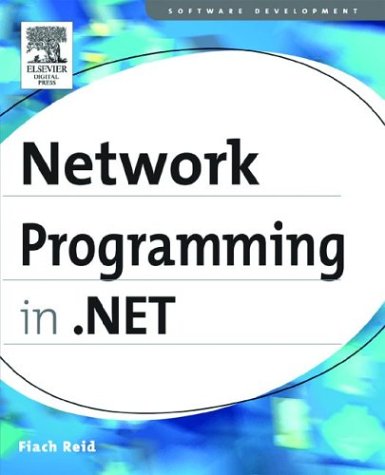
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewButtonCell.cs
- VisualStyleElement.cs
- EventListener.cs
- PrintController.cs
- DataSet.cs
- Baml2006ReaderFrame.cs
- DropShadowBitmapEffect.cs
- NativeMethods.cs
- VisualTreeHelper.cs
- SynchronizationContext.cs
- BrushValueSerializer.cs
- ObjectViewQueryResultData.cs
- TextServicesDisplayAttributePropertyRanges.cs
- MaskInputRejectedEventArgs.cs
- PriorityQueue.cs
- XmlIlVisitor.cs
- XhtmlBasicSelectionListAdapter.cs
- ErrorLog.cs
- SapiAttributeParser.cs
- SessionEndedEventArgs.cs
- WebConfigurationHost.cs
- ParallelForEach.cs
- ContentTextAutomationPeer.cs
- PartBasedPackageProperties.cs
- DateTime.cs
- Quaternion.cs
- SupportingTokenBindingElement.cs
- ReflectPropertyDescriptor.cs
- TagNameToTypeMapper.cs
- SystemException.cs
- ConnectionManagementElement.cs
- CollectionsUtil.cs
- SmtpTransport.cs
- StatusBarDrawItemEvent.cs
- ToolStripSplitButton.cs
- RealProxy.cs
- TextDecorations.cs
- DeviceContext.cs
- CalendarAutoFormat.cs
- ConnectionProviderAttribute.cs
- ProcessRequestArgs.cs
- DesignTimeTemplateParser.cs
- DbParameterCollectionHelper.cs
- BamlResourceSerializer.cs
- AssemblyCollection.cs
- SiteOfOriginPart.cs
- HttpRuntimeSection.cs
- BasicHttpMessageSecurityElement.cs
- UserInitiatedRoutedEventPermission.cs
- ExpandedProjectionNode.cs
- WindowInteropHelper.cs
- Cursor.cs
- BamlRecords.cs
- CodeObject.cs
- ModelUIElement3D.cs
- TCEAdapterGenerator.cs
- FixedDocumentSequencePaginator.cs
- DeviceContexts.cs
- System.Data.OracleClient_BID.cs
- ColorContext.cs
- ZipIOExtraFieldZip64Element.cs
- InteropAutomationProvider.cs
- TriggerBase.cs
- RtType.cs
- WmpBitmapEncoder.cs
- PipelineModuleStepContainer.cs
- NetStream.cs
- WpfWebRequestHelper.cs
- Substitution.cs
- EmptyTextWriter.cs
- XmlQualifiedName.cs
- CachingHintValidation.cs
- BuildResultCache.cs
- InstalledVoice.cs
- CheckBoxPopupAdapter.cs
- FormViewCommandEventArgs.cs
- UrlPath.cs
- AppLevelCompilationSectionCache.cs
- EntityChangedParams.cs
- TypePresenter.xaml.cs
- ReferenceService.cs
- UtilityExtension.cs
- Registry.cs
- ItemList.cs
- NavigationService.cs
- Stackframe.cs
- FunctionMappingTranslator.cs
- StreamSecurityUpgradeAcceptorBase.cs
- CryptoConfig.cs
- SettingsProviderCollection.cs
- Evidence.cs
- Converter.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- BinaryFormatterWriter.cs
- OleDbStruct.cs
- AppDomainManager.cs
- ObjectDataSourceFilteringEventArgs.cs
- EncoderReplacementFallback.cs
- WaitHandleCannotBeOpenedException.cs
- FontEditor.cs