Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / RoleManagerSection.cs / 2 / RoleManagerSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.ComponentModel; using System.Web.Security; // for CookieProtection Enum using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class RoleManagerSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnabled = new ConfigurationProperty("enabled", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propUseCookies = new ConfigurationProperty("cacheRolesInCookie", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCookieName = new ConfigurationProperty("cookieName", typeof(string), ".ASPXROLES", StdValidatorsAndConverters.WhiteSpaceTrimStringConverter, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCookieTimeout = new ConfigurationProperty("cookieTimeout", typeof(TimeSpan), TimeSpan.FromMinutes(30.0), StdValidatorsAndConverters.TimeSpanMinutesOrInfiniteConverter, StdValidatorsAndConverters.PositiveTimeSpanValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCookiePath = new ConfigurationProperty("cookiePath", typeof(string), "/", StdValidatorsAndConverters.WhiteSpaceTrimStringConverter, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCookieRequireSSL = new ConfigurationProperty("cookieRequireSSL", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCookieSlidingExpiration = new ConfigurationProperty("cookieSlidingExpiration", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCookieProtection = new ConfigurationProperty("cookieProtection", typeof(CookieProtection), CookieProtection.All, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDefaultProvider = new ConfigurationProperty("defaultProvider", typeof(string), "AspNetSqlRoleProvider", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProviders = new ConfigurationProperty("providers", typeof(ProviderSettingsCollection), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCreatePersistentCookie = new ConfigurationProperty("createPersistentCookie", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDomain = new ConfigurationProperty("domain", typeof(string), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxCachedResults = new ConfigurationProperty("maxCachedResults", typeof(int), 25, ConfigurationPropertyOptions.None); private enum InheritedType { inNeither = 0, inParent = 1, inSelf = 2, inBothSame = 3, inBothDiff = 4, } static RoleManagerSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnabled); _properties.Add(_propUseCookies); _properties.Add(_propCookieName); _properties.Add(_propCookieTimeout); _properties.Add(_propCookiePath); _properties.Add(_propCookieRequireSSL); _properties.Add(_propCookieSlidingExpiration); _properties.Add(_propCookieProtection); _properties.Add(_propDefaultProvider); _properties.Add(_propProviders); _properties.Add(_propCreatePersistentCookie); _properties.Add(_propDomain); _properties.Add(_propMaxCachedResults); } public RoleManagerSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("enabled", DefaultValue = false)] public bool Enabled { get { return (bool)base[_propEnabled]; } set { base[_propEnabled] = value; } } [ConfigurationProperty("createPersistentCookie", DefaultValue = false)] public bool CreatePersistentCookie { get { return (bool)base[_propCreatePersistentCookie]; } set { base[_propCreatePersistentCookie] = value; } } [ConfigurationProperty("cacheRolesInCookie", DefaultValue = false)] public bool CacheRolesInCookie { get { return (bool)base[_propUseCookies]; } set { base[_propUseCookies] = value; } } [ConfigurationProperty("cookieName", DefaultValue = ".ASPXROLES")] [TypeConverter(typeof(WhiteSpaceTrimStringConverter))] [StringValidator(MinLength = 1)] public string CookieName { get { return (string)base[_propCookieName]; } set { base[_propCookieName] = value; } } [ConfigurationProperty("cookieTimeout", DefaultValue = "00:30:00")] [TypeConverter(typeof(TimeSpanMinutesOrInfiniteConverter))] [TimeSpanValidator(MinValueString="00:00:00", MaxValueString=TimeSpanValidatorAttribute.TimeSpanMaxValue)] public TimeSpan CookieTimeout { get { return (TimeSpan)base[_propCookieTimeout]; } set { base[_propCookieTimeout] = value; } } [ConfigurationProperty("cookiePath", DefaultValue = "/")] [TypeConverter(typeof(WhiteSpaceTrimStringConverter))] [StringValidator(MinLength = 1)] public string CookiePath { get { return (string)base[_propCookiePath]; } set { base[_propCookiePath] = value; } } [ConfigurationProperty("cookieRequireSSL", DefaultValue = false)] public bool CookieRequireSSL { get { return (bool)base[_propCookieRequireSSL]; } set { base[_propCookieRequireSSL] = value; } } [ConfigurationProperty("cookieSlidingExpiration", DefaultValue = true)] public bool CookieSlidingExpiration { get { return (bool)base[_propCookieSlidingExpiration]; } set { base[_propCookieSlidingExpiration] = value; } } [ConfigurationProperty("cookieProtection", DefaultValue = CookieProtection.All)] public CookieProtection CookieProtection { get { return (CookieProtection)base[_propCookieProtection]; } set { base[_propCookieProtection] = value; } } [ConfigurationProperty("defaultProvider", DefaultValue = "AspNetSqlRoleProvider")] [TypeConverter(typeof(WhiteSpaceTrimStringConverter))] [StringValidator(MinLength = 1)] public string DefaultProvider { get { return (string)base[_propDefaultProvider]; } set { base[_propDefaultProvider] = value; } } [ConfigurationProperty("providers")] public ProviderSettingsCollection Providers { get { return (ProviderSettingsCollection)base[_propProviders]; } } [ConfigurationProperty("domain")] public string Domain { get { return (string)base[_propDomain]; } set { base[_propDomain] = value; } } [ConfigurationProperty("maxCachedResults", DefaultValue = 25)] public int MaxCachedResults { get { return (int)base[_propMaxCachedResults]; } set { base[_propMaxCachedResults] = value; } } } // class RoleManagerSection } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
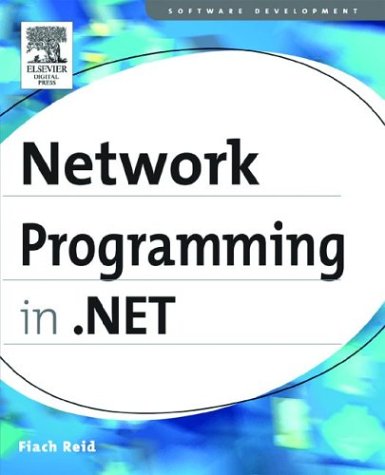
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectContext.cs
- DataListItem.cs
- TextViewBase.cs
- HighContrastHelper.cs
- HttpConfigurationContext.cs
- XslException.cs
- BaseDataBoundControl.cs
- TextParagraphCache.cs
- DataGridColumn.cs
- PerformanceCounterPermission.cs
- CapabilitiesRule.cs
- RegexWriter.cs
- ByteConverter.cs
- ImageField.cs
- initElementDictionary.cs
- CalendarDateChangedEventArgs.cs
- ObjectAssociationEndMapping.cs
- DragEventArgs.cs
- LinkLabelLinkClickedEvent.cs
- QueueException.cs
- ReferenceService.cs
- BasePropertyDescriptor.cs
- StrongNameKeyPair.cs
- CodeAttachEventStatement.cs
- AnimationClock.cs
- ServiceEndpointElement.cs
- UInt32Converter.cs
- DataTemplate.cs
- UDPClient.cs
- CodeDesigner.cs
- TextDecorationCollectionConverter.cs
- PropertySet.cs
- IntSecurity.cs
- PlatformNotSupportedException.cs
- DebugView.cs
- DiscoveryExceptionDictionary.cs
- TransformationRules.cs
- DependencyPropertyChangedEventArgs.cs
- UserNameSecurityTokenProvider.cs
- StylusDevice.cs
- VerificationAttribute.cs
- DefaultTraceListener.cs
- OdbcError.cs
- NegotiateStream.cs
- BoundsDrawingContextWalker.cs
- FieldValue.cs
- StorageInfo.cs
- CompensatableSequenceActivity.cs
- SelectionEditor.cs
- ImageListStreamer.cs
- EncodingNLS.cs
- ComNativeDescriptor.cs
- Timer.cs
- TerminateWorkflow.cs
- StylusEventArgs.cs
- CompositeActivityMarkupSerializer.cs
- BindingCollection.cs
- DataGridViewLayoutData.cs
- OracleBinary.cs
- PrintPreviewDialog.cs
- SmiSettersStream.cs
- BookmarkEventArgs.cs
- ObjectStateFormatter.cs
- ContentPropertyAttribute.cs
- NetworkInterface.cs
- SingletonChannelAcceptor.cs
- StreamSecurityUpgradeAcceptor.cs
- MgmtResManager.cs
- BuilderPropertyEntry.cs
- PipeStream.cs
- Operator.cs
- HierarchicalDataSourceIDConverter.cs
- TableItemProviderWrapper.cs
- GridViewDeleteEventArgs.cs
- ReadWriteControlDesigner.cs
- ServiceOperation.cs
- OracleSqlParser.cs
- SendKeys.cs
- SocketElement.cs
- SmiRequestExecutor.cs
- input.cs
- InputMethodStateChangeEventArgs.cs
- NameScopePropertyAttribute.cs
- Baml6ConstructorInfo.cs
- QilParameter.cs
- TypeExtensionSerializer.cs
- UriTemplateLiteralQueryValue.cs
- HttpInputStream.cs
- DragDeltaEventArgs.cs
- RepeaterItem.cs
- FileInfo.cs
- DocumentGridContextMenu.cs
- ToolZone.cs
- Memoizer.cs
- SizeConverter.cs
- TableCell.cs
- TlsnegoTokenAuthenticator.cs
- SelectionChangedEventArgs.cs
- TextFormatter.cs
- AsmxEndpointPickerExtension.cs