Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Server / System / Data / Services / Providers / ServiceOperation.cs / 2 / ServiceOperation.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a type to represent custom operations on services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { using System; using System.Diagnostics; using System.Reflection; ///Use this class to represent a custom service operation. [DebuggerVisualizer("ServiceOperation={Name}")] internal class ServiceOperation { ///Whether this is an update method or a GET method. ///Might need to be more specific than this when we design service operation updates. private readonly bool invoke; ///private readonly MethodInfo method; /// for the service operation. MIME type specified on primitive results, possibly null. private readonly string mimeType; ///In-order parameters for this operation. private readonly ServiceOperationParameter[] parameters; ///Kind of result expected from this operation. private readonly ServiceOperationResultKind resultKind; ///Type of element of the method result. private readonly Type resultType; ///Entity set from which entities are read, if applicable. private ResourceContainer entitySet; ///Access rights to this service operation. private ServiceOperationRights rights; ////// Initializes a new ///instance. /// for the service operation. /// Kind of result expected from this operation. /// Type of element of the method result. /// MIME type specified on primitive results, possibly null. /// Whether this is an update method or a GET method. /// In-order parameters for this operation. public ServiceOperation( MethodInfo method, ServiceOperationResultKind resultKind, Type resultType, string mimeType, bool invoke, ServiceOperationParameter[] parameters) { Debug.Assert(method != null, "method != null"); Debug.Assert(resultType != null, "resultType != null"); Debug.Assert(parameters != null, "parameters != null"); WebUtil.DebugEnumIsDefined(resultKind); if (mimeType != null && !WebUtil.IsValidMimeType(mimeType)) { string message = Strings.ServiceOperation_MimeTypeNotValid(mimeType, method.Name, method.DeclaringType); throw new InvalidOperationException(message); } this.method = method; this.resultKind = resultKind; this.resultType = resultType; this.mimeType = mimeType; this.invoke = invoke; this.parameters = parameters; } /// Entity set from which entities are read (possibly null). public ResourceContainer EntitySet { get { return this.entitySet; } set { this.entitySet = value; } } ///Whether this is an update method or a GET method. public bool Invoke { get { return this.invoke; } } ///public MethodInfo Method { get { return this.method; } } /// for the service operation. MIME type specified on primitive results, possibly null. public string MimeType { get { return this.mimeType; } } ///Name of the service operation. public string Name { get { return this.method.Name; } } ///In-order parameters for this operation. public ServiceOperationParameter[] Parameters { get { return this.parameters; } } ///Kind of result expected from this operation. public ServiceOperationResultKind ResultKind { get { return this.resultKind; } } ///Element of result type. ////// Note that if the method returns an IEnumerable<string>, /// this property will be typeof(string). /// public Type ResultType { get { return this.resultType; } } ///Whether the operation is visible to service consumers. internal bool IsHidden { get { return (this.rights & ~ServiceOperationRights.OverrideEntitySetRights) == ServiceOperationRights.None; } } ///Access rights to this service operation. internal ServiceOperationRights Rights { get { return this.rights; } set { this.rights = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a type to represent custom operations on services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { using System; using System.Diagnostics; using System.Reflection; ///Use this class to represent a custom service operation. [DebuggerVisualizer("ServiceOperation={Name}")] internal class ServiceOperation { ///Whether this is an update method or a GET method. ///Might need to be more specific than this when we design service operation updates. private readonly bool invoke; ///private readonly MethodInfo method; /// for the service operation. MIME type specified on primitive results, possibly null. private readonly string mimeType; ///In-order parameters for this operation. private readonly ServiceOperationParameter[] parameters; ///Kind of result expected from this operation. private readonly ServiceOperationResultKind resultKind; ///Type of element of the method result. private readonly Type resultType; ///Entity set from which entities are read, if applicable. private ResourceContainer entitySet; ///Access rights to this service operation. private ServiceOperationRights rights; ////// Initializes a new ///instance. /// for the service operation. /// Kind of result expected from this operation. /// Type of element of the method result. /// MIME type specified on primitive results, possibly null. /// Whether this is an update method or a GET method. /// In-order parameters for this operation. public ServiceOperation( MethodInfo method, ServiceOperationResultKind resultKind, Type resultType, string mimeType, bool invoke, ServiceOperationParameter[] parameters) { Debug.Assert(method != null, "method != null"); Debug.Assert(resultType != null, "resultType != null"); Debug.Assert(parameters != null, "parameters != null"); WebUtil.DebugEnumIsDefined(resultKind); if (mimeType != null && !WebUtil.IsValidMimeType(mimeType)) { string message = Strings.ServiceOperation_MimeTypeNotValid(mimeType, method.Name, method.DeclaringType); throw new InvalidOperationException(message); } this.method = method; this.resultKind = resultKind; this.resultType = resultType; this.mimeType = mimeType; this.invoke = invoke; this.parameters = parameters; } /// Entity set from which entities are read (possibly null). public ResourceContainer EntitySet { get { return this.entitySet; } set { this.entitySet = value; } } ///Whether this is an update method or a GET method. public bool Invoke { get { return this.invoke; } } ///public MethodInfo Method { get { return this.method; } } /// for the service operation. MIME type specified on primitive results, possibly null. public string MimeType { get { return this.mimeType; } } ///Name of the service operation. public string Name { get { return this.method.Name; } } ///In-order parameters for this operation. public ServiceOperationParameter[] Parameters { get { return this.parameters; } } ///Kind of result expected from this operation. public ServiceOperationResultKind ResultKind { get { return this.resultKind; } } ///Element of result type. ////// Note that if the method returns an IEnumerable<string>, /// this property will be typeof(string). /// public Type ResultType { get { return this.resultType; } } ///Whether the operation is visible to service consumers. internal bool IsHidden { get { return (this.rights & ~ServiceOperationRights.OverrideEntitySetRights) == ServiceOperationRights.None; } } ///Access rights to this service operation. internal ServiceOperationRights Rights { get { return this.rights; } set { this.rights = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
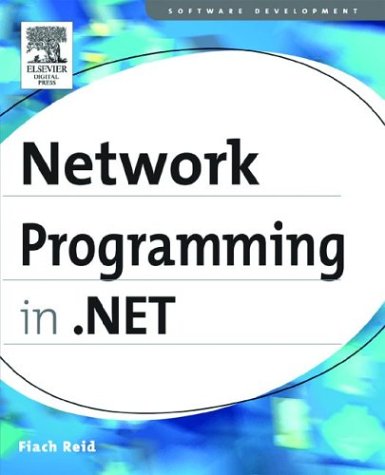
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewContainer.cs
- HeaderUtility.cs
- NativeStructs.cs
- ButtonChrome.cs
- WebPartMenuStyle.cs
- ContentType.cs
- DataBindingCollection.cs
- ReadOnlyHierarchicalDataSourceView.cs
- BigInt.cs
- LowerCaseStringConverter.cs
- Schedule.cs
- BufferedWebEventProvider.cs
- ProjectionPlan.cs
- AuthenticateEventArgs.cs
- VirtualizingStackPanel.cs
- SqlUserDefinedAggregateAttribute.cs
- NamespaceMapping.cs
- StatusBarDrawItemEvent.cs
- ObjectContextServiceProvider.cs
- EdmFunction.cs
- PersianCalendar.cs
- InvalidPrinterException.cs
- Size.cs
- activationcontext.cs
- ProxyGenerationError.cs
- RawAppCommandInputReport.cs
- Native.cs
- DefaultBindingPropertyAttribute.cs
- XmlSubtreeReader.cs
- Int32AnimationUsingKeyFrames.cs
- SystemEvents.cs
- BooleanExpr.cs
- NativeRightsManagementAPIsStructures.cs
- SqlTriggerAttribute.cs
- RijndaelManagedTransform.cs
- IndexObject.cs
- SystemWebSectionGroup.cs
- Parameter.cs
- BindUriHelper.cs
- SQLSingle.cs
- StrongNameKeyPair.cs
- ObjectItemCachedAssemblyLoader.cs
- FixedDocument.cs
- OutputCacheSettingsSection.cs
- RelationshipWrapper.cs
- ChangeBlockUndoRecord.cs
- HttpDictionary.cs
- ListBox.cs
- WebConfigurationManager.cs
- Tag.cs
- DropDownList.cs
- GlyphRun.cs
- WindowsTokenRoleProvider.cs
- XPathNodePointer.cs
- SafeCryptoHandles.cs
- PasswordDeriveBytes.cs
- ReadOnlyHierarchicalDataSourceView.cs
- Int32AnimationUsingKeyFrames.cs
- SynchronizedInputAdaptor.cs
- HwndStylusInputProvider.cs
- QueryableDataSource.cs
- Empty.cs
- ApplicationCommands.cs
- DesignerSerializationOptionsAttribute.cs
- TextSelectionProcessor.cs
- CustomAttribute.cs
- ProcessHostMapPath.cs
- ScriptReference.cs
- TextParagraph.cs
- PageBreakRecord.cs
- ToolStripOverflow.cs
- NotCondition.cs
- MulticastDelegate.cs
- ParseNumbers.cs
- Listener.cs
- RoleService.cs
- AttributeCollection.cs
- PixelFormat.cs
- ToolStripContentPanelRenderEventArgs.cs
- SecurityAlgorithmSuite.cs
- Window.cs
- DesigntimeLicenseContext.cs
- Qualifier.cs
- TogglePatternIdentifiers.cs
- CheckableControlBaseAdapter.cs
- DecimalConverter.cs
- SpellerError.cs
- DesignOnlyAttribute.cs
- WorkflowApplication.cs
- ImportCatalogPart.cs
- Variable.cs
- IndependentAnimationStorage.cs
- AppliedDeviceFiltersDialog.cs
- DataGridCommandEventArgs.cs
- Win32.cs
- FieldMetadata.cs
- TypeConverterMarkupExtension.cs
- DesignSurfaceEvent.cs
- SimpleFieldTemplateUserControl.cs
- WebServiceErrorEvent.cs