Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / PaintEvent.cs / 1 / PaintEvent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Windows.Forms.Internal; using System.Drawing.Drawing2D; using System.Runtime.InteropServices; ////// /// // NOTE: Please keep this class consistent with PrintPageEventArgs. public class PaintEventArgs : EventArgs, IDisposable { ////// Provides data for the ////// event. /// /// Graphics object with which painting should be done. /// private Graphics graphics = null; // See ResetGraphics() private GraphicsState savedGraphicsState = null; ////// DC (Display context) for obtaining the graphics object. Used to delay getting the graphics /// object until absolutely necessary (for perf reasons) /// private readonly IntPtr dc = IntPtr.Zero; IntPtr oldPal = IntPtr.Zero; ////// Rectangle into which all painting should be done. /// private readonly Rectangle clipRect; //private Control paletteSource; ////// /// public PaintEventArgs(Graphics graphics, Rectangle clipRect) { if( graphics == null ){ throw new ArgumentNullException("graphics"); } this.graphics = graphics; this.clipRect = clipRect; } // Internal version of constructor for performance // We try to avoid getting the graphics object until needed internal PaintEventArgs(IntPtr dc, Rectangle clipRect) { Debug.Assert(dc != IntPtr.Zero, "dc is not initialized."); this.dc = dc; this.clipRect = clipRect; } ////// Initializes a new instance of the ///class with the specified graphics and /// clipping rectangle. /// ~PaintEventArgs() { Dispose(false); } /// /// /// public Rectangle ClipRectangle { get { return clipRect; } } ////// Gets the /// rectangle in which to paint. /// ////// Gets the HDC this paint event is connected to. If there is no associated /// HDC, or the GDI+ Graphics object has been created (meaning GDI+ now owns the /// HDC), 0 is returned. /// /// internal IntPtr HDC { get { if (graphics == null) return dc; else return IntPtr.Zero; } } ////// /// public System.Drawing.Graphics Graphics { get { if (graphics == null && dc != IntPtr.Zero) { oldPal = Control.SetUpPalette(dc, false /*force*/, false /*realize*/); graphics = Graphics.FromHdcInternal(dc); graphics.PageUnit = GraphicsUnit.Pixel; savedGraphicsState = graphics.Save(); // See ResetGraphics() below } return graphics; } } // We want a way to dispose the GDI+ Graphics, but we don't want to create one // simply to dispose it // cpr: should be internal ////// Gets the ////// object used to /// paint. /// /// /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Disposes /// of the resources (other than memory) used by the ///. /// protected virtual void Dispose(bool disposing) { if (disposing) { //only dispose the graphics object if we created it via the dc. if (graphics != null && dc != IntPtr.Zero) { graphics.Dispose(); } } if (oldPal != IntPtr.Zero && dc != IntPtr.Zero) { SafeNativeMethods.SelectPalette(new HandleRef(this, dc), new HandleRef(this, oldPal), 0); oldPal = IntPtr.Zero; } } // If ControlStyles.AllPaintingInWmPaint, we call this method // after OnPaintBackground so it appears to OnPaint that it's getting a fresh // Graphics. We want to make sure AllPaintingInWmPaint is purely an optimization, // and doesn't change behavior, so we need to make sure any clipping regions established // in OnPaintBackground don't apply to OnPaint. See ASURT 44682. internal void ResetGraphics() { if (graphics != null) { Debug.Assert(dc == IntPtr.Zero || savedGraphicsState != null, "Called ResetGraphics more than once?"); if (savedGraphicsState != null) { graphics.Restore(savedGraphicsState); savedGraphicsState = null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
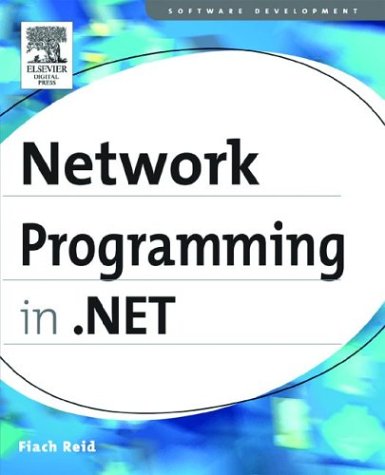
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StatusBarPanel.cs
- Light.cs
- SemanticKeyElement.cs
- COM2PropertyBuilderUITypeEditor.cs
- ResizeGrip.cs
- PictureBox.cs
- FixedPage.cs
- BrowserDefinitionCollection.cs
- IndentedWriter.cs
- SoapCodeExporter.cs
- XmlBinaryReader.cs
- PathNode.cs
- ExpressionEditorAttribute.cs
- XmlSchemaExternal.cs
- DbModificationCommandTree.cs
- InstanceDataCollectionCollection.cs
- GraphicsState.cs
- ListControlDataBindingHandler.cs
- PanelDesigner.cs
- CodeRegionDirective.cs
- TextDecorationCollectionConverter.cs
- CustomErrorCollection.cs
- EntityProviderFactory.cs
- PropertyOverridesTypeEditor.cs
- TiffBitmapDecoder.cs
- DispatcherHooks.cs
- CardSpaceSelector.cs
- AbstractSvcMapFileLoader.cs
- PlatformNotSupportedException.cs
- FileDialogCustomPlacesCollection.cs
- DrawListViewItemEventArgs.cs
- SortedList.cs
- ForeignConstraint.cs
- HtmlImageAdapter.cs
- RemoteWebConfigurationHost.cs
- Convert.cs
- ServiceDescriptionImporter.cs
- CodeNamespace.cs
- MarkerProperties.cs
- XpsImageSerializationService.cs
- PlanCompilerUtil.cs
- DocumentGrid.cs
- CqlBlock.cs
- InstanceLockTracking.cs
- WinInetCache.cs
- DateBoldEvent.cs
- BamlCollectionHolder.cs
- GetMemberBinder.cs
- TextEffectCollection.cs
- FileFormatException.cs
- RecognizeCompletedEventArgs.cs
- HostVisual.cs
- ModulesEntry.cs
- SqlProviderServices.cs
- FilterableAttribute.cs
- StringKeyFrameCollection.cs
- WebPartTransformer.cs
- PointValueSerializer.cs
- NumericUpDownAccelerationCollection.cs
- ProcessInfo.cs
- WebPartAuthorizationEventArgs.cs
- StoryFragments.cs
- CompositeDesignerAccessibleObject.cs
- Directory.cs
- ConsoleKeyInfo.cs
- ConstraintConverter.cs
- DbProviderFactories.cs
- InstancePersistenceEvent.cs
- DataGridItemCollection.cs
- Options.cs
- WebPartZoneCollection.cs
- SecurityDescriptor.cs
- HierarchicalDataBoundControlAdapter.cs
- AlternateView.cs
- URLAttribute.cs
- SecurityRuntime.cs
- DbConnectionFactory.cs
- webclient.cs
- BaseComponentEditor.cs
- ValidatedControlConverter.cs
- ProfileGroupSettings.cs
- SystemTcpStatistics.cs
- NavigatorInput.cs
- DrawingBrush.cs
- SchemaReference.cs
- InternalCache.cs
- XmlDeclaration.cs
- ParameterCollection.cs
- SmiEventStream.cs
- ObjectDataSourceSelectingEventArgs.cs
- ScrollItemPatternIdentifiers.cs
- CompositeDataBoundControl.cs
- ItemCheckedEvent.cs
- PaperSize.cs
- HyperLinkField.cs
- NativeActivityMetadata.cs
- PageAsyncTaskManager.cs
- LoginView.cs
- DataGridAutomationPeer.cs
- Random.cs