Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / UserControlDocumentDesigner.cs / 1 / UserControlDocumentDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Collections; using System.ComponentModel; using System.Runtime.Serialization; using System.Diagnostics; using System; using System.Design; using System.ComponentModel.Design; using Microsoft.Win32; using System.Drawing; using System.Drawing.Design; using System.IO; using System.Windows.Forms; ////// /// [ ToolboxItemFilter("System.Windows.Forms.UserControl", ToolboxItemFilterType.Custom), ToolboxItemFilter("System.Windows.Forms.MainMenu", ToolboxItemFilterType.Prevent) ] internal class UserControlDocumentDesigner : DocumentDesigner { public UserControlDocumentDesigner() { AutoResizeHandles = true; } ///Provides a base implementation of a designer for user controls. ////// On user controls, size == client size. We do this so we can mess around /// with the non-client area of the user control when editing menus and not /// mess up the size property. /// private Size Size { get { return Control.ClientSize; } set { Control.ClientSize = value; } } internal override bool CanDropComponents(DragEventArgs de) { bool canDrop = base.CanDropComponents(de); if (canDrop) { // Figure out if any of the components in a main menu item. // We don't like main menus on UserControlDocumentDesigner. // OleDragDropHandler ddh = GetOleDragHandler(); object[] dragComps = ddh.GetDraggingObjects(de); if (dragComps != null) { IDesignerHost host = (IDesignerHost)GetService(typeof(IDesignerHost)); for (int i = 0; i < dragComps.Length; i++) { if (host == null || dragComps[i] == null || !(dragComps[i] is IComponent)) { continue; } if (dragComps[i] is MainMenu) return false; } } } return canDrop; } ////// /// Allows a designer to filter the set of properties /// the component it is designing will expose through the /// TypeDescriptor object. This method is called /// immediately before its corresponding "Post" method. /// If you are overriding this method you should call /// the base implementation before you perform your own /// filtering. /// protected override void PreFilterProperties(IDictionary properties) { PropertyDescriptor prop; base.PreFilterProperties(properties); // Handle shadowed properties // string[] shadowProps = new string[] { "Size" }; Attribute[] empty = new Attribute[0]; for (int i = 0; i < shadowProps.Length; i++) { prop = (PropertyDescriptor)properties[shadowProps[i]]; if (prop != null) { properties[shadowProps[i]] = TypeDescriptor.CreateProperty(typeof(UserControlDocumentDesigner), prop, empty); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
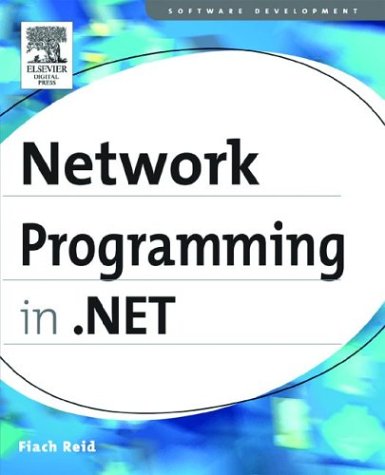
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SingleQueryOperator.cs
- PKCS1MaskGenerationMethod.cs
- Serializer.cs
- ResolveMatches11.cs
- TrackingMemoryStreamFactory.cs
- TdsRecordBufferSetter.cs
- SmiXetterAccessMap.cs
- RegexInterpreter.cs
- FrameworkContentElement.cs
- DBDataPermissionAttribute.cs
- DataColumnChangeEvent.cs
- SqlNodeAnnotations.cs
- OuterGlowBitmapEffect.cs
- BasicAsyncResult.cs
- SystemNetworkInterface.cs
- PrivateFontCollection.cs
- ClientTargetSection.cs
- UserPersonalizationStateInfo.cs
- AttributeQuery.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- TdsParserSafeHandles.cs
- ContainerFilterService.cs
- ClientSettingsSection.cs
- ServerIdentity.cs
- SqlDependencyListener.cs
- Pen.cs
- SQLDateTime.cs
- TreePrinter.cs
- UInt64Storage.cs
- SourceElementsCollection.cs
- DelegatedStream.cs
- sqlnorm.cs
- ManagementInstaller.cs
- CachedPathData.cs
- NumberFormatInfo.cs
- COM2ExtendedUITypeEditor.cs
- SecurityKeyUsage.cs
- NetworkInterface.cs
- xsdvalidator.cs
- NeutralResourcesLanguageAttribute.cs
- ElementAtQueryOperator.cs
- IfAction.cs
- basecomparevalidator.cs
- AuthenticationModuleElement.cs
- OperationResponse.cs
- InputBuffer.cs
- VariableElement.cs
- FileInfo.cs
- PowerEase.cs
- Container.cs
- ObjectQuery.cs
- DataAdapter.cs
- TextServicesContext.cs
- TerminateDesigner.cs
- CodeConstructor.cs
- XmlSchemaComplexContentExtension.cs
- GridViewCancelEditEventArgs.cs
- DynamicEntity.cs
- RMPublishingDialog.cs
- NavigatorInput.cs
- PropertyEntry.cs
- SrgsItemList.cs
- FromRequest.cs
- AdvancedBindingEditor.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- EditorZoneBase.cs
- NotifyParentPropertyAttribute.cs
- BlockUIContainer.cs
- TextServicesProperty.cs
- DrawingCollection.cs
- SHA256.cs
- WrappedReader.cs
- SecurityElementBase.cs
- WebPartDescriptionCollection.cs
- AsymmetricKeyExchangeFormatter.cs
- TypeSystemProvider.cs
- ReachUIElementCollectionSerializer.cs
- QuaternionKeyFrameCollection.cs
- AccessViolationException.cs
- TextViewBase.cs
- SoapObjectInfo.cs
- EnumerableRowCollection.cs
- NavigationPropertyEmitter.cs
- XPathSelectionIterator.cs
- ErrorWrapper.cs
- PropertyInformation.cs
- CqlQuery.cs
- TypeUtils.cs
- EntityDataSourceUtil.cs
- SwitchLevelAttribute.cs
- XmlQueryRuntime.cs
- ValuePattern.cs
- SqlBuffer.cs
- HierarchicalDataBoundControl.cs
- __FastResourceComparer.cs
- SectionInput.cs
- TypeConverter.cs
- TwoPhaseCommit.cs
- SystemIPInterfaceProperties.cs
- Expressions.cs