Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / DesignerExtenders.cs / 1 / DesignerExtenders.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System; using System.CodeDom; using System.CodeDom.Compiler; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Diagnostics.CodeAnalysis; ////// This class provides the Modifiers property to components. It is shared between /// the document designer and the component document designer. /// internal class DesignerExtenders { private IExtenderProvider[] providers; private IExtenderProviderService extenderService; ////// /// This is called by a root designer to add the correct extender providers. /// public DesignerExtenders(IExtenderProviderService ex) { this.extenderService = ex; if (providers == null) { providers = new IExtenderProvider[] { new NameExtenderProvider(), new NameInheritedExtenderProvider() }; } for (int i = 0; i < providers.Length; i++) { ex.AddExtenderProvider(providers[i]); } } ////// /// This is called at the appropriate time to remove any extra extender /// providers previously added to the designer host. /// public void Dispose() { if (extenderService != null && providers != null) { for (int i = 0; i < providers.Length; i++) { extenderService.RemoveExtenderProvider(providers[i]); } providers = null; extenderService = null; } } ////// This is the base extender provider for all winform document /// designers. It provides the "Name" property. /// [ ProvideProperty("Name", typeof(IComponent)) ] private class NameExtenderProvider : IExtenderProvider { private IComponent baseComponent; ////// Creates a new DocumentExtenderProvider. /// internal NameExtenderProvider() { } protected IComponent GetBaseComponent(object o) { if (baseComponent == null) { ISite site = ((IComponent)o).Site; if (site != null) { IDesignerHost host = (IDesignerHost)site.GetService(typeof(IDesignerHost)); if (host != null) { baseComponent = host.RootComponent; } } } return baseComponent; } ////// Determines if ths extender provider can extend the given object. We extend /// all objects, so we always return true. /// public virtual bool CanExtend(object o) { // We always extend the root // IComponent baseComp = GetBaseComponent(o); if (baseComp == o) { return true; } // See if this object is inherited. If so, then we don't want to // extend. // if (!TypeDescriptor.GetAttributes(o)[typeof(InheritanceAttribute)].Equals(InheritanceAttribute.NotInherited)) { return false; } return true; } ////// This is an extender property that we offer to all components /// on the form. It implements the "Name" property. /// [ DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ParenthesizePropertyName(true), MergableProperty(false), SRDescriptionAttribute(SR.DesignerPropName), Category("Design") ] [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] public virtual string GetName(IComponent comp) { ISite site = comp.Site; if (site != null) { return site.Name; } return null; } ////// This is an extender property that we offer to all components /// on the form. It implements the "Name" property. /// [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] public void SetName(IComponent comp, string newName) { ISite site = comp.Site; if (site != null) { site.Name = newName; } } } ////// This extender provider offers up read-only versions of "Name" property /// for inherited components. /// private class NameInheritedExtenderProvider : NameExtenderProvider { ////// Creates a new DocumentInheritedExtenderProvider. /// internal NameInheritedExtenderProvider() { } ////// Determines if ths extender provider can extend the given object. We extend /// all objects, so we always return true. /// public override bool CanExtend(object o) { // We never extend the root // IComponent baseComp = GetBaseComponent(o); if (baseComp == o) { return false; } // See if this object is inherited. If so, then we are interested in it. // if (!TypeDescriptor.GetAttributes(o)[typeof(InheritanceAttribute)].Equals(InheritanceAttribute.NotInherited)) { return true; } return false; } [ReadOnly(true)] public override string GetName(IComponent comp) { return base.GetName(comp); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
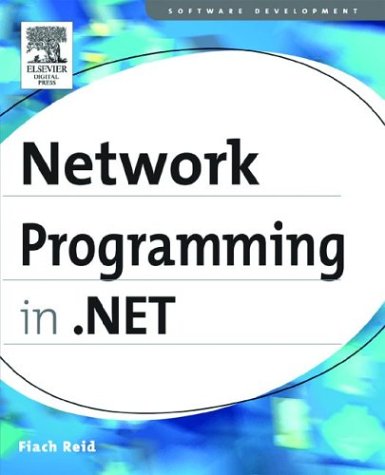
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LockedAssemblyCache.cs
- SQLGuid.cs
- SessionStateContainer.cs
- ConfigurationElementCollection.cs
- MutexSecurity.cs
- RandomNumberGenerator.cs
- ArcSegment.cs
- RuleRefElement.cs
- CaseInsensitiveHashCodeProvider.cs
- TypeExtensionConverter.cs
- HostedHttpContext.cs
- StringConcat.cs
- DaylightTime.cs
- MDIControlStrip.cs
- TdsParserSafeHandles.cs
- FastEncoder.cs
- RunWorkerCompletedEventArgs.cs
- DockPanel.cs
- CodeGeneratorOptions.cs
- DataGridSortCommandEventArgs.cs
- PresentationAppDomainManager.cs
- ApplicationActivator.cs
- MetaForeignKeyColumn.cs
- InstancePersistence.cs
- NestedContainer.cs
- ClickablePoint.cs
- OdbcException.cs
- XmlEntityReference.cs
- PipelineModuleStepContainer.cs
- SupportingTokenProviderSpecification.cs
- MenuItem.cs
- XsdSchemaFileEditor.cs
- SplineKeyFrames.cs
- CustomErrorsSection.cs
- Line.cs
- LightweightEntityWrapper.cs
- templategroup.cs
- Variable.cs
- AuthorizationRule.cs
- DesignerDataSchemaClass.cs
- XhtmlBasicPageAdapter.cs
- XmlDataSourceNodeDescriptor.cs
- CompressedStack.cs
- AvTraceFormat.cs
- ParagraphResult.cs
- AppearanceEditorPart.cs
- AppSettingsSection.cs
- DateTime.cs
- StyleSheetRefUrlEditor.cs
- StringReader.cs
- Thread.cs
- COSERVERINFO.cs
- _NtlmClient.cs
- SrgsRulesCollection.cs
- AvtEvent.cs
- _HeaderInfo.cs
- DefaultEventAttribute.cs
- PhysicalAddress.cs
- XamlTreeBuilderBamlRecordWriter.cs
- __Error.cs
- Ref.cs
- WebBrowserEvent.cs
- XmlNodeList.cs
- New.cs
- DataBoundControlHelper.cs
- StringUtil.cs
- Environment.cs
- OleDbReferenceCollection.cs
- PermissionAttributes.cs
- VirtualizingStackPanel.cs
- DataGridViewRowEventArgs.cs
- SqlProviderServices.cs
- FileSystemInfo.cs
- CursorConverter.cs
- RuleConditionDialog.Designer.cs
- ZipIOCentralDirectoryBlock.cs
- XmlSchemaAnnotated.cs
- ReadWriteObjectLock.cs
- DataGridViewColumnHeaderCell.cs
- SignedPkcs7.cs
- HelpPage.cs
- ObjectCacheHost.cs
- PeerNameRecord.cs
- IUnknownConstantAttribute.cs
- DataTableNewRowEvent.cs
- XmlObjectSerializer.cs
- Matrix3D.cs
- CreationContext.cs
- SafeCryptHandles.cs
- PreservationFileWriter.cs
- VersionValidator.cs
- TransactionState.cs
- SQLBinaryStorage.cs
- XmlChildNodes.cs
- XamlRtfConverter.cs
- PrimitiveXmlSerializers.cs
- DateTime.cs
- DefaultClaimSet.cs
- ImageUrlEditor.cs
- DecimalAnimationUsingKeyFrames.cs