Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / DataSourceViewSchemaConverter.cs / 1 / DataSourceViewSchemaConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Globalization; using System.Runtime.InteropServices; using System.Web.UI; ////// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class DataSourceViewSchemaConverter : TypeConverter { ////// Provides design-time support for getting schema from an object /// ////// /// public DataSourceViewSchemaConverter() { } ////// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return false; } ////// Gets a value indicating whether this converter can /// convert an object in the given source type to the native type of the converter /// using the context. /// ////// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { return String.Empty; } else if (value.GetType() == typeof(string)) { return (string)value; } throw GetConvertFromException(value); } ////// Converts the given object to the converter's native type. /// ////// /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { return GetStandardValues(context, null); } public virtual StandardValuesCollection GetStandardValues(ITypeDescriptorContext context, Type typeFilter) { string[] names = null; if (context != null) { IDataSourceViewSchemaAccessor schemaAccessor = context.Instance as IDataSourceViewSchemaAccessor; if (schemaAccessor != null) { IDataSourceViewSchema schema = schemaAccessor.DataSourceViewSchema as IDataSourceViewSchema; if (schema != null) { IDataSourceFieldSchema[] fields = schema.GetFields(); string[] tempNames = new string[fields.Length]; int fieldCount = 0; for (int i = 0; i < fields.Length; i++) { if ((typeFilter != null && fields[i].DataType == typeFilter) || typeFilter == null) { tempNames[fieldCount] = fields[i].Name; fieldCount++; } } names = new string[fieldCount]; Array.Copy(tempNames, names, fieldCount); } } if (names == null) { names = new string[0]; } Array.Sort(names, Comparer.Default); } return new StandardValuesCollection(names); } ////// Gets the fields present within the selected object's schema /// ////// /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// Gets a value indicating whether the collection of standard values returned from /// ///is an exclusive /// list of possible values, using the specified context. /// /// /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { if ((context != null) && (context.Instance is IDataSourceViewSchemaAccessor)) { return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Gets a value indicating whether this object supports a standard set of values /// that can be picked from a list. /// ///
Link Menu
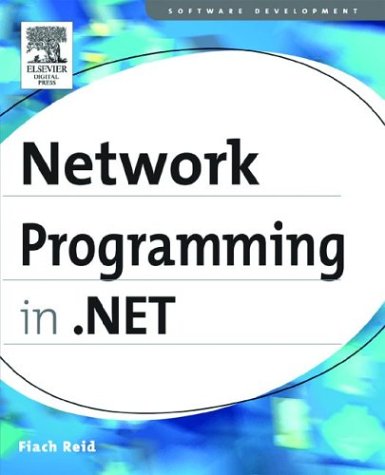
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AspNetPartialTrustHelpers.cs
- CultureSpecificCharacterBufferRange.cs
- CultureSpecificStringDictionary.cs
- TreeView.cs
- RunWorkerCompletedEventArgs.cs
- BooleanSwitch.cs
- documentsequencetextcontainer.cs
- AutoGeneratedField.cs
- TemplateControlBuildProvider.cs
- XmlChildNodes.cs
- MouseButton.cs
- ToolStripDropDown.cs
- SQLString.cs
- ExternalException.cs
- EnumUnknown.cs
- ValidatorCompatibilityHelper.cs
- NotifyCollectionChangedEventArgs.cs
- PathSegment.cs
- NullableLongMinMaxAggregationOperator.cs
- EntityDataSourceWrapperCollection.cs
- Viewport3DVisual.cs
- SignatureDescription.cs
- VisualProxy.cs
- TabRenderer.cs
- GlyphsSerializer.cs
- DesignerVerbCollection.cs
- FlatButtonAppearance.cs
- MarshalDirectiveException.cs
- RecordBuilder.cs
- SolidBrush.cs
- AsyncPostBackErrorEventArgs.cs
- __ComObject.cs
- Substitution.cs
- MemberMaps.cs
- Mutex.cs
- RequestCachePolicy.cs
- XXXInfos.cs
- DockPanel.cs
- MergePropertyDescriptor.cs
- MenuBindingsEditorForm.cs
- TargetConverter.cs
- PageFunction.cs
- securitymgrsite.cs
- SecurityCriticalDataForSet.cs
- AggregateNode.cs
- X509Extension.cs
- Rect3DValueSerializer.cs
- QueueProcessor.cs
- TTSEvent.cs
- MethodAccessException.cs
- EmptyElement.cs
- TextDecorationUnitValidation.cs
- AdCreatedEventArgs.cs
- Parameter.cs
- Processor.cs
- SqlWebEventProvider.cs
- XmlSchemaSimpleContent.cs
- Misc.cs
- columnmapfactory.cs
- ComponentConverter.cs
- SqlConnectionPoolGroupProviderInfo.cs
- SortAction.cs
- WindowsSysHeader.cs
- RequestStatusBarUpdateEventArgs.cs
- InputLanguageProfileNotifySink.cs
- EntityDescriptor.cs
- XmlSchemaAttributeGroupRef.cs
- DBSchemaRow.cs
- MenuItemBinding.cs
- TextEditorDragDrop.cs
- OleDbErrorCollection.cs
- ByteRangeDownloader.cs
- DataServiceQueryContinuation.cs
- HitTestParameters3D.cs
- BitmapMetadataEnumerator.cs
- TagPrefixAttribute.cs
- WebBrowserUriTypeConverter.cs
- DataGridTextBoxColumn.cs
- DSASignatureFormatter.cs
- EntityContainer.cs
- MailAddressCollection.cs
- DPCustomTypeDescriptor.cs
- SecurityManager.cs
- Policy.cs
- Stacktrace.cs
- ReflectEventDescriptor.cs
- SettingsPropertyIsReadOnlyException.cs
- Button.cs
- WindowsSlider.cs
- MULTI_QI.cs
- NameSpaceExtractor.cs
- AdRotator.cs
- OleDbRowUpdatedEvent.cs
- _Events.cs
- Emitter.cs
- Latin1Encoding.cs
- SmtpAuthenticationManager.cs
- Item.cs
- MeshGeometry3D.cs
- HtmlToClrEventProxy.cs