Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Synthesis / TTSEvent.cs / 1 / TTSEvent.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Contains either a reference to an audio audioStream or a list of // bookmark fragments. // // History: // 2/1/2005 jeanfp Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Speech.Internal.ObjectTokens; using System.Speech.Synthesis; using System.Speech.Synthesis.TtsEngine; namespace System.Speech.Internal.Synthesis { ////// /// internal class TTSEvent { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal TTSEvent (TtsEventId id, Prompt prompt, Exception exception, VoiceInfo voice) { _evtId = id; _prompt = prompt; _exception = exception; _voice = voice; } internal TTSEvent (TtsEventId id, Prompt prompt, Exception exception, VoiceInfo voice, TimeSpan audioPosition, long streamPosition, string bookmark, uint wParam, IntPtr lParam) : this (id, prompt, exception, voice) { _audioPosition = audioPosition; _bookmark = bookmark; _wParam = wParam; _lParam = lParam; #if SPEECHSERVER _streamPosition = streamPosition; #endif } #if !SPEECHSERVER private TTSEvent() { } static internal TTSEvent CreatePhonemeEvent(string phoneme, string nextPhoneme, TimeSpan duration, SynthesizerEmphasis emphasis, Prompt prompt, TimeSpan audioPosition) { TTSEvent ttsEvent = new TTSEvent(); ttsEvent._evtId = TtsEventId.Phoneme; ttsEvent._audioPosition = audioPosition; ttsEvent._prompt = prompt; ttsEvent._phoneme = phoneme; ttsEvent._nextPhoneme = nextPhoneme; ttsEvent._phonemeDuration = duration; ttsEvent._phonemeEmphasis = emphasis; return ttsEvent; } #endif #endregion //******************************************************************** // // Internal Properties // //******************************************************************* #region Internal Properties internal TtsEventId Id { get { return _evtId; } } internal Exception Exception { get { return _exception; } } internal Prompt Prompt { get { return _prompt; } } internal VoiceInfo Voice { get { return _voice; } } internal TimeSpan AudioPosition { get { return _audioPosition; } } internal string Bookmark { get { return _bookmark; } } internal IntPtr LParam { get { return _lParam; } } internal uint WParam { get { return _wParam; } } #if !SPEECHSERVER internal SynthesizerEmphasis PhonemeEmphasis { get { return _phonemeEmphasis; } } internal string Phoneme { get { return _phoneme; } } internal string NextPhoneme { get { return _nextPhoneme; } set { _nextPhoneme = value; } } internal TimeSpan PhonemeDuration { get { return _phonemeDuration; } } #else internal long StreamPosition { get { return _streamPosition; } } #endif #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region private Fields private TtsEventId _evtId; private Exception _exception; private VoiceInfo _voice; private TimeSpan _audioPosition; private string _bookmark; private uint _wParam; private IntPtr _lParam; private Prompt _prompt; #if !SPEECHSERVER // // Data for phoneme event // private string _phoneme; private string _nextPhoneme; private TimeSpan _phonemeDuration; private SynthesizerEmphasis _phonemeEmphasis; #else private long _streamPosition; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Contains either a reference to an audio audioStream or a list of // bookmark fragments. // // History: // 2/1/2005 jeanfp Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Speech.Internal.ObjectTokens; using System.Speech.Synthesis; using System.Speech.Synthesis.TtsEngine; namespace System.Speech.Internal.Synthesis { ////// /// internal class TTSEvent { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal TTSEvent (TtsEventId id, Prompt prompt, Exception exception, VoiceInfo voice) { _evtId = id; _prompt = prompt; _exception = exception; _voice = voice; } internal TTSEvent (TtsEventId id, Prompt prompt, Exception exception, VoiceInfo voice, TimeSpan audioPosition, long streamPosition, string bookmark, uint wParam, IntPtr lParam) : this (id, prompt, exception, voice) { _audioPosition = audioPosition; _bookmark = bookmark; _wParam = wParam; _lParam = lParam; #if SPEECHSERVER _streamPosition = streamPosition; #endif } #if !SPEECHSERVER private TTSEvent() { } static internal TTSEvent CreatePhonemeEvent(string phoneme, string nextPhoneme, TimeSpan duration, SynthesizerEmphasis emphasis, Prompt prompt, TimeSpan audioPosition) { TTSEvent ttsEvent = new TTSEvent(); ttsEvent._evtId = TtsEventId.Phoneme; ttsEvent._audioPosition = audioPosition; ttsEvent._prompt = prompt; ttsEvent._phoneme = phoneme; ttsEvent._nextPhoneme = nextPhoneme; ttsEvent._phonemeDuration = duration; ttsEvent._phonemeEmphasis = emphasis; return ttsEvent; } #endif #endregion //******************************************************************** // // Internal Properties // //******************************************************************* #region Internal Properties internal TtsEventId Id { get { return _evtId; } } internal Exception Exception { get { return _exception; } } internal Prompt Prompt { get { return _prompt; } } internal VoiceInfo Voice { get { return _voice; } } internal TimeSpan AudioPosition { get { return _audioPosition; } } internal string Bookmark { get { return _bookmark; } } internal IntPtr LParam { get { return _lParam; } } internal uint WParam { get { return _wParam; } } #if !SPEECHSERVER internal SynthesizerEmphasis PhonemeEmphasis { get { return _phonemeEmphasis; } } internal string Phoneme { get { return _phoneme; } } internal string NextPhoneme { get { return _nextPhoneme; } set { _nextPhoneme = value; } } internal TimeSpan PhonemeDuration { get { return _phonemeDuration; } } #else internal long StreamPosition { get { return _streamPosition; } } #endif #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region private Fields private TtsEventId _evtId; private Exception _exception; private VoiceInfo _voice; private TimeSpan _audioPosition; private string _bookmark; private uint _wParam; private IntPtr _lParam; private Prompt _prompt; #if !SPEECHSERVER // // Data for phoneme event // private string _phoneme; private string _nextPhoneme; private TimeSpan _phonemeDuration; private SynthesizerEmphasis _phonemeEmphasis; #else private long _streamPosition; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
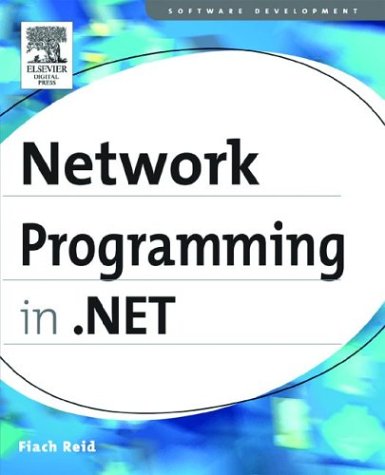
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventLogStatus.cs
- DataAdapter.cs
- ErrorTableItemStyle.cs
- SiteMapProvider.cs
- DesignerContextDescriptor.cs
- NotImplementedException.cs
- SmtpReplyReader.cs
- ToolStripInSituService.cs
- Function.cs
- Encoding.cs
- XmlILAnnotation.cs
- XPathConvert.cs
- MimeTypeMapper.cs
- DbTransaction.cs
- GridView.cs
- IteratorFilter.cs
- CharEntityEncoderFallback.cs
- CellParaClient.cs
- WorkflowDesigner.cs
- XPathPatternParser.cs
- X509Chain.cs
- Thread.cs
- UInt64Converter.cs
- BinHexDecoder.cs
- _UriTypeConverter.cs
- CompositeKey.cs
- CngProvider.cs
- RepeaterItemEventArgs.cs
- XPathAncestorIterator.cs
- StrokeDescriptor.cs
- DbMetaDataColumnNames.cs
- InkPresenter.cs
- DesignTimeTemplateParser.cs
- RegexBoyerMoore.cs
- CodeIdentifiers.cs
- ScrollChrome.cs
- WsatStrings.cs
- ExeConfigurationFileMap.cs
- Substitution.cs
- Unit.cs
- ProgressBarAutomationPeer.cs
- AttachedPropertyBrowsableAttribute.cs
- ClrPerspective.cs
- Cursor.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- DataGridCellInfo.cs
- AutomationPeer.cs
- ScaleTransform3D.cs
- RoleManagerModule.cs
- ListBase.cs
- HtmlInputImage.cs
- Byte.cs
- ParameterCollection.cs
- OperationAbortedException.cs
- ClientFormsAuthenticationCredentials.cs
- DependencyPropertyConverter.cs
- SamlAdvice.cs
- SharingService.cs
- PathSegmentCollection.cs
- HandlerFactoryWrapper.cs
- XPathNodeHelper.cs
- Identity.cs
- NonSerializedAttribute.cs
- SelectionGlyphBase.cs
- ProfileBuildProvider.cs
- DocumentViewerBaseAutomationPeer.cs
- SqlTopReducer.cs
- FilterQuery.cs
- AdornerDecorator.cs
- JapaneseLunisolarCalendar.cs
- PrintController.cs
- SolidColorBrush.cs
- NetworkAddressChange.cs
- PermissionSetEnumerator.cs
- PageOutputColor.cs
- nulltextcontainer.cs
- UxThemeWrapper.cs
- ActiveXContainer.cs
- SequenceNumber.cs
- XamlVector3DCollectionSerializer.cs
- FrameworkTemplate.cs
- PtsCache.cs
- StructuralComparisons.cs
- EventQueueState.cs
- FontFamilyValueSerializer.cs
- TransformGroup.cs
- XmlCharType.cs
- TableRowGroup.cs
- PrefixHandle.cs
- BitmapVisualManager.cs
- XamlFigureLengthSerializer.cs
- DefaultClaimSet.cs
- ListControlDesigner.cs
- _NtlmClient.cs
- DataGridViewCellValueEventArgs.cs
- SecurityContext.cs
- SimpleNameService.cs
- RightsManagementEncryptedStream.cs
- BreakRecordTable.cs
- HandleValueEditor.cs