Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Runtime / InteropServices / RuntimeEnvironment.cs / 1 / RuntimeEnvironment.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: RuntimeEnvironment ** ** ** Purpose: Runtime information ** ** =============================================================================*/ using System; using System.Text; using System.IO; using System.Runtime.CompilerServices; using System.Security.Permissions; using System.Reflection; using Microsoft.Win32; namespace System.Runtime.InteropServices { [System.Runtime.InteropServices.ComVisible(true)] public class RuntimeEnvironment { [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern String GetModuleFileName(); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern String GetDeveloperPath(); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern String GetHostBindingFile(); [DllImport(Win32Native.SHIM, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.StdCall)] private static extern int GetCORVersion(StringBuilder sb, int BufferLength, ref int retLength); [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern bool FromGlobalAccessCache(Assembly a); public static String GetSystemVersion() { StringBuilder s = new StringBuilder(256); int retLength = 0; if(GetCORVersion(s, 256, ref retLength) == 0) return s.ToString(); else return null; } public static String GetRuntimeDirectory() { String dir = GetRuntimeDirectoryImpl(); new FileIOPermission(FileIOPermissionAccess.PathDiscovery, dir).Demand(); return dir; } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern String GetRuntimeDirectoryImpl(); // Returns the system ConfigurationFile public static String SystemConfigurationFile { get { StringBuilder sb = new StringBuilder(Path.MAX_PATH); sb.Append(GetRuntimeDirectory()); sb.Append(AppDomainSetup.RuntimeConfigurationFile); String path = sb.ToString(); // Do security check new FileIOPermission(FileIOPermissionAccess.PathDiscovery, path).Demand(); return path; } } } }
Link Menu
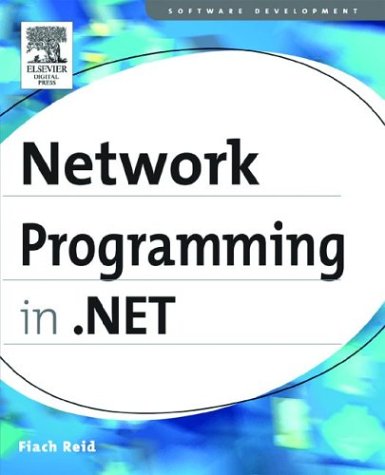
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CheckBoxField.cs
- ClockController.cs
- SafeNativeMethodsMilCoreApi.cs
- ExecutionContext.cs
- TextEditorCopyPaste.cs
- HandleExceptionArgs.cs
- ViewStateException.cs
- Query.cs
- MasterPageCodeDomTreeGenerator.cs
- LiteralSubsegment.cs
- EditorPart.cs
- TdsValueSetter.cs
- FixedFlowMap.cs
- FontFaceLayoutInfo.cs
- CommandBinding.cs
- DesignerHost.cs
- ConfigUtil.cs
- DesignerDataStoredProcedure.cs
- SelectedGridItemChangedEvent.cs
- SerialPort.cs
- ClientProtocol.cs
- PointAnimationClockResource.cs
- DeviceContexts.cs
- EntityTypeBase.cs
- SimpleRecyclingCache.cs
- DataFieldEditor.cs
- Matrix.cs
- EntityDataSourceSelectedEventArgs.cs
- Directory.cs
- ValidationResult.cs
- CustomExpression.cs
- DelimitedListTraceListener.cs
- RetriableClipboard.cs
- TreeView.cs
- HatchBrush.cs
- CursorConverter.cs
- UserControlDesigner.cs
- _UriSyntax.cs
- KeyboardEventArgs.cs
- MenuTracker.cs
- ActivityCodeDomSerializer.cs
- StylusButtonEventArgs.cs
- SystemUdpStatistics.cs
- ButtonField.cs
- OdbcConnectionPoolProviderInfo.cs
- SQLMoney.cs
- VisualStyleRenderer.cs
- ToolStripItem.cs
- FullTrustAssembliesSection.cs
- ApplicationId.cs
- DataGridSortCommandEventArgs.cs
- infer.cs
- CommandField.cs
- BaseTemplateBuildProvider.cs
- InvalidDataException.cs
- securitycriticaldataClass.cs
- Metafile.cs
- EntitySet.cs
- JapaneseLunisolarCalendar.cs
- CompressStream.cs
- StringArrayConverter.cs
- VisualBrush.cs
- ConvertTextFrag.cs
- ValidationSummary.cs
- SelfIssuedAuthAsymmetricKey.cs
- LineBreak.cs
- Roles.cs
- Console.cs
- ObjectStateEntry.cs
- ErrorHandler.cs
- Wizard.cs
- ConditionalAttribute.cs
- Binding.cs
- ScopedKnownTypes.cs
- MenuBase.cs
- PointF.cs
- IndexedGlyphRun.cs
- CmsInterop.cs
- TextAdaptor.cs
- NotCondition.cs
- SoapReflectionImporter.cs
- TypeSystem.cs
- TextElementAutomationPeer.cs
- MethodBuilderInstantiation.cs
- ThicknessKeyFrameCollection.cs
- StrokeCollection2.cs
- NullableLongSumAggregationOperator.cs
- Trace.cs
- WindowsListViewItemStartMenu.cs
- AccessViolationException.cs
- CodeNamespace.cs
- DesignTableCollection.cs
- ColorContext.cs
- CmsInterop.cs
- DependentList.cs
- EngineSiteSapi.cs
- ImageListStreamer.cs
- MatrixAnimationUsingPath.cs
- CustomErrorCollection.cs
- VersionPair.cs