Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Reflection / Emit / AssemblyBuilder.cs / 1 / AssemblyBuilder.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //************************************************************************************************************** // For each dynamic assembly there will be two AssemblyBuilder objects: the "internal" // AssemblyBuilder object and the "external" AssemblyBuilder object. // 1. The "internal" object is the real assembly object that the VM creates and knows about. However, // you can perform RefEmit operations on it only if you have its granted permission. From the AppDomain // and other "internal" objects like the "internal" ModuleBuilders and runtime types, you can only // get the "internal" objects. This is to prevent low-trust code from getting a hold of the dynamic // AssemblyBuilder/ModuleBuilder/TypeBuilder/MethodBuilder/etc other people have created by simply // enumerating the AppDomain and inject code in it. // 2. The "external" object is merely an wrapper of the "internal" object and all operations on it // are directed to the internal object. This is the one you get by calling DefineDynamicAssembly // on AppDomain and the one you can always perform RefEmit operations on. You can get other "external" // objects from the "external" AssemblyBuilder, ModuleBuilder, TypeBuilder, MethodBuilder, etc. Note // that VM doesn't know about this object. So every time we call into the VM we need to pass in the // "internal" object. // // "internal" and "external" ModuleBuilders are similar //************************************************************************************************************* namespace System.Reflection.Emit { using System; using System.IO; using System.Diagnostics.SymbolStore; using System.Reflection; using System.Diagnostics; using System.Resources; using System.Security.Permissions; using System.Runtime.Remoting.Activation; using CultureInfo = System.Globalization.CultureInfo; using System.Runtime.Serialization; using System.Security; using System.Threading; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Runtime.Versioning; using System.Collections.Generic; // These must match the definitions in Assembly.hpp [Flags] internal enum DynamicAssemblyFlags { None = 0x00000000, Transparent = 0x00000001, } // AssemblyBuilder class. // deliberately not [serializable] [HostProtection(MayLeakOnAbort = true)] [ClassInterface(ClassInterfaceType.None)] [ComDefaultInterface(typeof(_AssemblyBuilder))] [ComVisible(true)] public sealed class AssemblyBuilder : Assembly, _AssemblyBuilder { #region Intenral Data Members private AssemblyBuilder m_internalAssemblyBuilder; private PermissionSet m_grantedPermissionSet; private PermissionSet GrantedPermissionSet { get { AssemblyBuilder internalAssemblyBuilder = (AssemblyBuilder)InternalAssembly; if (internalAssemblyBuilder.m_grantedPermissionSet == null) { PermissionSet denied = null; InternalAssembly.nGetGrantSet(out internalAssemblyBuilder.m_grantedPermissionSet, out denied); // The grantset is null for any dynamic assemblies created by mscorlib // e.g. the Anonymously Hosted DynamicMethods Assembly if (internalAssemblyBuilder.m_grantedPermissionSet == null) { // set it to full trust internalAssemblyBuilder.m_grantedPermissionSet = new PermissionSet(PermissionState.Unrestricted); } } return internalAssemblyBuilder.m_grantedPermissionSet; } } internal void DemandGrantedPermission() { GrantedPermissionSet.Demand(); } // m_internalAssemblyBuilder is null iff this is a "internal" AssemblyBuilder private bool IsInternal { get { return (m_internalAssemblyBuilder == null); } } internal override Assembly InternalAssembly { get { if (IsInternal) return this; else return m_internalAssemblyBuilder; } } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] internal override Module[] nGetModules(bool loadIfNotFound, bool getResourceModules) { // Retrieving the "internal" modules Module[] modules = InternalAssembly._nGetModules(loadIfNotFound, getResourceModules); if (!IsInternal) { // For an external AssemblyBuilder, We should return the "external" ModuleBuilders for (int i = 0; i < modules.Length; i++) { modules[i] = ModuleBuilder.GetModuleBuilder(modules[i]); } } return modules; } internal override Module GetModuleInternal(String name) { Module module = InternalAssembly._GetModule(name); if (module == null) return null; if (!IsInternal) return ModuleBuilder.GetModuleBuilder(module); else return module; } #endregion #region Constructor internal AssemblyBuilder(AssemblyBuilder internalAssemblyBuilder) { m_internalAssemblyBuilder = internalAssemblyBuilder; } #endregion /********************************************** * * Defines a named dynamic module. It is an error to define multiple * modules within an Assembly with the same name. This dynamic module is * a transient module. * **********************************************/ [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public ModuleBuilder DefineDynamicModule( String name) { if (IsInternal) DemandGrantedPermission(); StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return DefineDynamicModuleInternal(name, false, ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public ModuleBuilder DefineDynamicModule( String name, bool emitSymbolInfo) // specify if emit symbol info or not { if (IsInternal) DemandGrantedPermission(); StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return DefineDynamicModuleInternal( name, emitSymbolInfo, ref stackMark ); } private ModuleBuilder DefineDynamicModuleInternal( String name, bool emitSymbolInfo, // specify if emit symbol info or not ref StackCrawlMark stackMark) { if (m_assemblyData.m_isSynchronized) { lock(m_assemblyData) { return DefineDynamicModuleInternalNoLock(name, emitSymbolInfo, ref stackMark); } } else { return DefineDynamicModuleInternalNoLock(name, emitSymbolInfo, ref stackMark); } } private ModuleBuilder DefineDynamicModuleInternalNoLock( String name, bool emitSymbolInfo, // specify if emit symbol info or not ref StackCrawlMark stackMark) { BCLDebug.Log("DYNIL","## DYNIL LOGGING: AssemblyBuilder.DefineDynamicModule( " + name + " )"); BCLDebug.Assert(m_assemblyData != null, "m_assemblyData is null in DefineDynamicModuleInternal"); if (name == null) throw new ArgumentNullException("name"); if (name.Length == 0) throw new ArgumentException(Environment.GetResourceString("Argument_EmptyName"), "name"); if (name[0] == '\0') throw new ArgumentException(Environment.GetResourceString("Argument_InvalidName"), "name"); m_assemblyData.CheckNameConflict(name); // create the dynamic module // this gets the "internal" ModuleBuilder ModuleBuilder dynModule = (ModuleBuilder)nDefineDynamicModule(this, emitSymbolInfo, name, ref stackMark); // this gets the "external" ModuleBuilder dynModule = new ModuleBuilder(this, dynModule); ISymbolWriter writer = null; if (emitSymbolInfo) { // create the default SymWriter Assembly assem = LoadISymWrapper(); Type symWriter = assem.GetType("System.Diagnostics.SymbolStore.SymWriter", true, false); if (symWriter != null && !symWriter.IsVisible) symWriter = null; if (symWriter == null) { // cannot find SymWriter throw new ExecutionEngineException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString(ResId.MissingType), "SymWriter")); } new ReflectionPermission(ReflectionPermissionFlag.ReflectionEmit).Demand(); try { (new PermissionSet(PermissionState.Unrestricted)).Assert(); writer = (ISymbolWriter) Activator.CreateInstance(symWriter); } finally { CodeAccessPermission.RevertAssert(); } } dynModule.Init(name, null, writer); m_assemblyData.AddModule(dynModule); return dynModule; } private Assembly LoadISymWrapper() { if (m_assemblyData.m_ISymWrapperAssembly != null) return m_assemblyData.m_ISymWrapperAssembly; Assembly assem = Assembly.Load("ISymWrapper, Version=" + ThisAssembly.Version + ", Culture=neutral, PublicKeyToken=" + AssemblyRef.MicrosoftPublicKeyToken); m_assemblyData.m_ISymWrapperAssembly = assem; return assem; } /********************************************** * * Defines a named dynamic module. It is an error to define multiple * modules within an Assembly with the same name. No symbol information * will be emitted. * **********************************************/ [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public ModuleBuilder DefineDynamicModule( String name, String fileName) { if (IsInternal) DemandGrantedPermission(); StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; // delegate to the next DefineDynamicModule return DefineDynamicModuleInternal(name, fileName, false, ref stackMark); } /********************************************** * * Emit symbol information if emitSymbolInfo is true using the * default symbol writer interface. * An exception will be thrown if the assembly is transient. * **********************************************/ [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public ModuleBuilder DefineDynamicModule( String name, // module name String fileName, // module file name bool emitSymbolInfo) // specify if emit symbol info or not { if (IsInternal) DemandGrantedPermission(); StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return DefineDynamicModuleInternal(name, fileName, emitSymbolInfo, ref stackMark); } private ModuleBuilder DefineDynamicModuleInternal( String name, // module name String fileName, // module file name bool emitSymbolInfo, // specify if emit symbol info or not ref StackCrawlMark stackMark) // stack crawl mark used to find caller { if (m_assemblyData.m_isSynchronized) { lock(m_assemblyData) { return DefineDynamicModuleInternalNoLock(name, fileName, emitSymbolInfo, ref stackMark); } } else { return DefineDynamicModuleInternalNoLock(name, fileName, emitSymbolInfo, ref stackMark); } } internal void CheckContext(params Type[][] typess) { if (typess == null) return; foreach(Type[] types in typess) if (types != null) CheckContext(types); } internal void CheckContext(params Type[] types) { if (types == null) return; foreach (Type type in types) { if (type == null || type.Module.Assembly == typeof(object).Module.Assembly) return; if (type.Module.Assembly.ReflectionOnly && !ReflectionOnly) throw new InvalidOperationException(String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString("Arugment_EmitMixedContext1"), type.AssemblyQualifiedName)); if (!type.Module.Assembly.ReflectionOnly && ReflectionOnly) throw new InvalidOperationException(String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString("Arugment_EmitMixedContext2"), type.AssemblyQualifiedName)); } } private ModuleBuilder DefineDynamicModuleInternalNoLock( String name, // module name String fileName, // module file name bool emitSymbolInfo, // specify if emit symbol info or not ref StackCrawlMark stackMark) // stack crawl mark used to find caller { BCLDebug.Log("DYNIL","## DYNIL LOGGING: AssemblyBuilder.DefineDynamicModule( " + name + ", " + fileName + ", " + emitSymbolInfo + " )"); if (m_assemblyData.m_access == AssemblyBuilderAccess.Run) { // Error! You cannot define a persistable module within a transient data. throw new NotSupportedException(Environment.GetResourceString("Argument_BadPersistableModuleInTransientAssembly")); } if (m_assemblyData.m_isSaved == true) { // assembly has been saved before! throw new InvalidOperationException(Environment.GetResourceString( "InvalidOperation_CannotAlterAssembly")); } if (name == null) throw new ArgumentNullException("name"); if (name.Length == 0) throw new ArgumentException(Environment.GetResourceString("Argument_EmptyName"), "name"); if (name[0] == '\0') throw new ArgumentException(Environment.GetResourceString("Argument_InvalidName"), "name"); if (fileName == null) throw new ArgumentNullException("fileName"); if (fileName.Length == 0) throw new ArgumentException(Environment.GetResourceString("Argument_EmptyFileName"), "fileName"); if (!String.Equals(fileName, Path.GetFileName(fileName))) throw new ArgumentException(Environment.GetResourceString("Argument_NotSimpleFileName"), "fileName"); m_assemblyData.CheckNameConflict(name); m_assemblyData.CheckFileNameConflict(fileName); // ecall to create the dynamic module // this gets the "internal" ModuleBuilder ModuleBuilder dynModule = (ModuleBuilder)nDefineDynamicModule(this, emitSymbolInfo, fileName, ref stackMark); // this gets the "external" ModuleBuilder dynModule = new ModuleBuilder(this, dynModule); ISymbolWriter writer = null; if (emitSymbolInfo) { // create the default SymWriter Assembly assem = LoadISymWrapper(); Type symWriter = assem.GetType("System.Diagnostics.SymbolStore.SymWriter", true, false); if (symWriter != null && !symWriter.IsVisible) symWriter = null; if (symWriter == null) { // cannot find SymWriter throw new ExecutionEngineException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("MissingType"), "SymWriter")); } new ReflectionPermission(ReflectionPermissionFlag.ReflectionEmit).Demand(); try { (new PermissionSet(PermissionState.Unrestricted)).Assert(); writer = (ISymbolWriter) Activator.CreateInstance(symWriter); } finally { CodeAccessPermission.RevertAssert(); } } // initialize the dynamic module's managed side information dynModule.Init(name, fileName, writer); m_assemblyData.AddModule(dynModule); return dynModule; } /********************************************** * * Define stand alone managed resource for Assembly * **********************************************/ [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public IResourceWriter DefineResource( String name, String description, String fileName) { return DefineResource(name, description, fileName, ResourceAttributes.Public); } /********************************************** * * Define stand alone managed resource for Assembly * **********************************************/ [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public IResourceWriter DefineResource( String name, String description, String fileName, ResourceAttributes attribute) { if (IsInternal) DemandGrantedPermission(); if (m_assemblyData.m_isSynchronized) { lock(m_assemblyData) { return DefineResourceNoLock(name, description, fileName, attribute); } } else { return DefineResourceNoLock(name, description, fileName, attribute); } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] private IResourceWriter DefineResourceNoLock( String name, String description, String fileName, ResourceAttributes attribute) { BCLDebug.Log("DYNIL","## DYNIL LOGGING: AssemblyBuilder.DefineResource( " + name + ", " + fileName + ")"); if (name == null) throw new ArgumentNullException("name"); if (name.Length == 0) throw new ArgumentException(Environment.GetResourceString("Argument_EmptyName"), name); if (fileName == null) throw new ArgumentNullException("fileName"); if (fileName.Length == 0) throw new ArgumentException(Environment.GetResourceString("Argument_EmptyFileName"), "fileName"); if (!String.Equals(fileName, Path.GetFileName(fileName))) throw new ArgumentException(Environment.GetResourceString("Argument_NotSimpleFileName"), "fileName"); m_assemblyData.CheckResNameConflict(name); m_assemblyData.CheckFileNameConflict(fileName); ResourceWriter resWriter; String fullFileName; if (m_assemblyData.m_strDir == null) { // If assembly directory is null, use current directory fullFileName = Path.Combine(Environment.CurrentDirectory, fileName); resWriter = new ResourceWriter(fullFileName); } else { // Form the full path given the directory provided by user fullFileName = Path.Combine(m_assemblyData.m_strDir, fileName); resWriter = new ResourceWriter(fullFileName); } // get the full path fullFileName = Path.GetFullPath(fullFileName); // retrieve just the file name fileName = Path.GetFileName(fullFileName); m_assemblyData.AddResWriter( new ResWriterData( resWriter, null, name, fileName, fullFileName, attribute) ); return resWriter; } /********************************************** * * Add an existing resource file to the Assembly * **********************************************/ [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public void AddResourceFile( String name, String fileName) { AddResourceFile(name, fileName, ResourceAttributes.Public); } /********************************************** * * Add an existing resource file to the Assembly * **********************************************/ [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public void AddResourceFile( String name, String fileName, ResourceAttributes attribute) { if (IsInternal) DemandGrantedPermission(); if (m_assemblyData.m_isSynchronized) { lock(m_assemblyData) { AddResourceFileNoLock(name, fileName, attribute); } } else { AddResourceFileNoLock(name, fileName, attribute); } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] private void AddResourceFileNoLock( String name, String fileName, ResourceAttributes attribute) { BCLDebug.Log("DYNIL","## DYNIL LOGGING: AssemblyBuilder.AddResourceFile( " + name + ", " + fileName + ")"); if (name == null) throw new ArgumentNullException("name"); if (name.Length == 0) throw new ArgumentException(Environment.GetResourceString("Argument_EmptyName"), name); if (fileName == null) throw new ArgumentNullException("fileName"); if (fileName.Length == 0) throw new ArgumentException(Environment.GetResourceString("Argument_EmptyFileName"), fileName); if (!String.Equals(fileName, Path.GetFileName(fileName))) throw new ArgumentException(Environment.GetResourceString("Argument_NotSimpleFileName"), "fileName"); m_assemblyData.CheckResNameConflict(name); m_assemblyData.CheckFileNameConflict(fileName); String fullFileName; if (m_assemblyData.m_strDir == null) { // If assembly directory is null, use current directory fullFileName = Path.Combine(Environment.CurrentDirectory, fileName); } else { // Form the full path given the directory provided by user fullFileName = Path.Combine(m_assemblyData.m_strDir, fileName); } // get the full path fullFileName = Path.GetFullPath(fullFileName); // retrieve just the file name fileName = Path.GetFileName(fullFileName); if (File.Exists(fullFileName) == false) throw new FileNotFoundException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString( "IO.FileNotFound_FileName"), fileName), fileName); m_assemblyData.AddResWriter( new ResWriterData( null, null, name, fileName, fullFileName, attribute) ); } #region Assembly overrides // Returns the names of all the resources public override String[] GetManifestResourceNames() { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicAssembly")); } public override FileStream GetFile(String name) { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicAssembly")); } public override FileStream[] GetFiles(bool getResourceModules) { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicAssembly")); } public override Stream GetManifestResourceStream(Type type, String name) { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicAssembly")); } public override Stream GetManifestResourceStream(String name) { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicAssembly")); } public override ManifestResourceInfo GetManifestResourceInfo(String resourceName) { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicAssembly")); } public override String Location { get { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicAssembly")); } } public override String ImageRuntimeVersion { get { return RuntimeEnvironment.GetSystemVersion(); } } public override String CodeBase { get { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicAssembly")); } } // Override the EntryPoint method on Assembly. // This doesn't need to be synchronized because it is simple enough public override MethodInfo EntryPoint { get { if (IsInternal) { // We only return MethodBuilder if this is an "external" AssemblyBuilder or we have its granted permission DemandGrantedPermission(); } return m_assemblyData.m_entryPointMethod; } } // Get an array of all the public types defined in this assembly public override Type[] GetExportedTypes() { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicAssembly")); } #endregion #if !FEATURE_PAL /********************************************** * * Add an unmanaged Version resource to the * assembly * **********************************************/ public void DefineVersionInfoResource( String product, String productVersion, String company, String copyright, String trademark) { if (IsInternal) DemandGrantedPermission(); if (m_assemblyData.m_isSynchronized) { lock (m_assemblyData) { DefineVersionInfoResourceNoLock( product, productVersion, company, copyright, trademark); } } else { DefineVersionInfoResourceNoLock( product, productVersion, company, copyright, trademark); } } private void DefineVersionInfoResourceNoLock( String product, String productVersion, String company, String copyright, String trademark) { if (m_assemblyData.m_strResourceFileName != null || m_assemblyData.m_resourceBytes != null || m_assemblyData.m_nativeVersion != null) throw new ArgumentException(Environment.GetResourceString("Argument_NativeResourceAlreadyDefined")); m_assemblyData.m_nativeVersion = new NativeVersionInfo(); m_assemblyData.m_nativeVersion.m_strCopyright = copyright; m_assemblyData.m_nativeVersion.m_strTrademark = trademark; m_assemblyData.m_nativeVersion.m_strCompany = company; m_assemblyData.m_nativeVersion.m_strProduct = product; m_assemblyData.m_nativeVersion.m_strProductVersion = productVersion; m_assemblyData.m_hasUnmanagedVersionInfo = true; m_assemblyData.m_OverrideUnmanagedVersionInfo = true; } public void DefineVersionInfoResource() { if (IsInternal) DemandGrantedPermission(); if (m_assemblyData.m_isSynchronized) { lock (m_assemblyData) { DefineVersionInfoResourceNoLock(); } } else { DefineVersionInfoResourceNoLock(); } } private void DefineVersionInfoResourceNoLock() { if (m_assemblyData.m_strResourceFileName != null || m_assemblyData.m_resourceBytes != null || m_assemblyData.m_nativeVersion != null) throw new ArgumentException(Environment.GetResourceString("Argument_NativeResourceAlreadyDefined")); m_assemblyData.m_hasUnmanagedVersionInfo = true; m_assemblyData.m_nativeVersion = new NativeVersionInfo(); } public void DefineUnmanagedResource(Byte[] resource) { if (IsInternal) DemandGrantedPermission(); if (resource == null) throw new ArgumentNullException("resource"); if (m_assemblyData.m_isSynchronized) { lock (m_assemblyData) { DefineUnmanagedResourceNoLock(resource); } } else { DefineUnmanagedResourceNoLock(resource); } } private void DefineUnmanagedResourceNoLock(Byte[] resource) { if (m_assemblyData.m_strResourceFileName != null || m_assemblyData.m_resourceBytes != null || m_assemblyData.m_nativeVersion != null) throw new ArgumentException(Environment.GetResourceString("Argument_NativeResourceAlreadyDefined")); m_assemblyData.m_resourceBytes = new byte[resource.Length]; System.Array.Copy(resource, m_assemblyData.m_resourceBytes, resource.Length); } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public void DefineUnmanagedResource(String resourceFileName) { if (IsInternal) DemandGrantedPermission(); if (resourceFileName == null) throw new ArgumentNullException("resourceFileName"); if (m_assemblyData.m_isSynchronized) { lock (m_assemblyData) { DefineUnmanagedResourceNoLock(resourceFileName); } } else { DefineUnmanagedResourceNoLock(resourceFileName); } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] private void DefineUnmanagedResourceNoLock(String resourceFileName) { if (m_assemblyData.m_strResourceFileName != null || m_assemblyData.m_resourceBytes != null || m_assemblyData.m_nativeVersion != null) throw new ArgumentException(Environment.GetResourceString("Argument_NativeResourceAlreadyDefined")); // Check caller has the right to read the file. string strFullFileName; if (m_assemblyData.m_strDir == null) { // If assembly directory is null, use current directory strFullFileName = Path.Combine(Environment.CurrentDirectory, resourceFileName); } else { // Form the full path given the directory provided by user strFullFileName = Path.Combine(m_assemblyData.m_strDir, resourceFileName); } strFullFileName = Path.GetFullPath(resourceFileName); new FileIOPermission(FileIOPermissionAccess.Read, strFullFileName).Demand(); if (File.Exists(strFullFileName) == false) throw new FileNotFoundException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString( "IO.FileNotFound_FileName"), resourceFileName), resourceFileName); m_assemblyData.m_strResourceFileName = strFullFileName; } #endif // !FEATURE_PAL /********************************************** * * return a dynamic module with the specified name. * **********************************************/ public ModuleBuilder GetDynamicModule( String name) // the name of module for the look up { // We cannot allow this operation on internal AssemblyBuilders // because this method always returns an external ModuleBuilder if (IsInternal) DemandGrantedPermission(); if (m_assemblyData.m_isSynchronized) { lock(m_assemblyData) { return GetDynamicModuleNoLock(name); } } else { return GetDynamicModuleNoLock(name); } } private ModuleBuilder GetDynamicModuleNoLock( String name) // the name of module for the look up { BCLDebug.Log("DYNIL","## DYNIL LOGGING: AssemblyBuilder.GetDynamicModule( " + name + " )"); if (name == null) throw new ArgumentNullException("name"); if (name.Length == 0) throw new ArgumentException(Environment.GetResourceString("Argument_EmptyName"), "name"); int size = m_assemblyData.m_moduleBuilderList.Count; for (int i=0;i
Link Menu
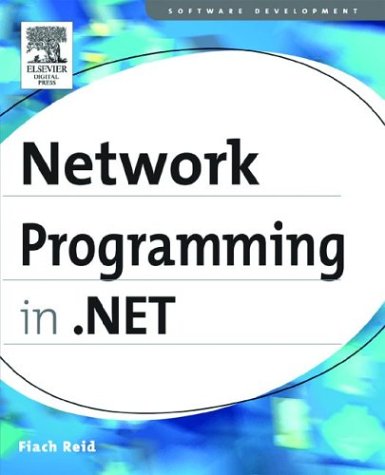
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UniqueID.cs
- NumberFunctions.cs
- CommandSet.cs
- ClientSettingsProvider.cs
- NotifyCollectionChangedEventArgs.cs
- DummyDataSource.cs
- VectorValueSerializer.cs
- AttributeCollection.cs
- CoreSwitches.cs
- KeyedHashAlgorithm.cs
- ZipIOBlockManager.cs
- TextDecoration.cs
- ChangeInterceptorAttribute.cs
- DbParameterCollectionHelper.cs
- ArrayWithOffset.cs
- SerializationException.cs
- UserControlBuildProvider.cs
- bindurihelper.cs
- PermissionAttributes.cs
- ComboBoxAutomationPeer.cs
- DiscoveryReferences.cs
- DataGridViewTextBoxEditingControl.cs
- AspNetHostingPermission.cs
- XmlUtil.cs
- ImpersonationOption.cs
- ExpressionConverter.cs
- Property.cs
- RenamedEventArgs.cs
- DataShape.cs
- EventDescriptorCollection.cs
- BindingContext.cs
- HiddenField.cs
- RoutedEventHandlerInfo.cs
- DataGridViewColumnCollectionDialog.cs
- DefinitionUpdate.cs
- HeaderedItemsControl.cs
- MatrixAnimationBase.cs
- SortFieldComparer.cs
- CodeArrayCreateExpression.cs
- LogRecordSequence.cs
- KeyNotFoundException.cs
- XmlSerializationWriter.cs
- DynamicFilterExpression.cs
- JapaneseLunisolarCalendar.cs
- DataControlCommands.cs
- BrushMappingModeValidation.cs
- BitmapImage.cs
- RegexCaptureCollection.cs
- HTTPNotFoundHandler.cs
- X509ChainPolicy.cs
- HttpWriter.cs
- _PooledStream.cs
- COM2ICategorizePropertiesHandler.cs
- TypedAsyncResult.cs
- FixedSOMFixedBlock.cs
- ImpersonateTokenRef.cs
- DataObjectFieldAttribute.cs
- DesignerDataView.cs
- RoleManagerModule.cs
- XsltConvert.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- HttpModuleActionCollection.cs
- MouseGesture.cs
- DiscoveryClientChannelFactory.cs
- ReflectionTypeLoadException.cs
- DbConnectionPoolOptions.cs
- CodeParameterDeclarationExpression.cs
- TypeConverterHelper.cs
- WebPartConnectionsEventArgs.cs
- BaseTemplateBuildProvider.cs
- RetriableClipboard.cs
- CachedCompositeFamily.cs
- DataGridViewRowCancelEventArgs.cs
- ApplicationException.cs
- tabpagecollectioneditor.cs
- PKCS1MaskGenerationMethod.cs
- HttpWebRequest.cs
- RegisteredScript.cs
- ListMarkerLine.cs
- EntityWrapperFactory.cs
- InvariantComparer.cs
- CopyOnWriteList.cs
- BaseProcessor.cs
- glyphs.cs
- NameValueFileSectionHandler.cs
- LocatorPartList.cs
- FormViewRow.cs
- StringFunctions.cs
- ImageField.cs
- WebDisplayNameAttribute.cs
- CodeDirectoryCompiler.cs
- TextTreeTextNode.cs
- ConnectionPointGlyph.cs
- BufferBuilder.cs
- NotifyIcon.cs
- MetaDataInfo.cs
- WarningException.cs
- xamlnodes.cs
- shaper.cs
- BamlLocalizerErrorNotifyEventArgs.cs