Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / WindowCollection.cs / 1 / WindowCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // WindowCollection can be used to interate over all the windows // that have been opened in the current application. // // History: // 08/10/04: kusumav Moved out of Application.cs to its own separate file. // //--------------------------------------------------------------------------- using System.Collections; using System.Diagnostics; namespace System.Windows { #region WindowCollection class ////// WindowCollection can be used to interate over all the windows that have been /// opened in the current application. /// // public sealed class WindowCollection : ICollection { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Default Constructor /// public WindowCollection() { _list = new ArrayList(1); } internal WindowCollection(int count) { Debug.Assert(count >= 0, "count must not be less than zero"); _list = new ArrayList(count); } #endregion Public Methods //------------------------------------------------------ // // Operator overload // //----------------------------------------------------- #region Operator overload ////// Overloaded [] operator to access the WindowCollection list /// public Window this[int index] { get { return _list[index] as Window; } } #endregion Operator overload //------------------------------------------------------ // // IEnumerable implementation // //------------------------------------------------------ #region IEnumerable implementation ////// GetEnumerator /// ///public IEnumerator GetEnumerator() { return _list.GetEnumerator(); } #endregion IEnumerable implementation //------------------------------------------------------- // // ICollection implementation (derives from IEnumerable) // //-------------------------------------------------------- #region ICollection implementation /// /// CopyTo /// /// /// void ICollection.CopyTo(Array array, int index) { _list.CopyTo(array, index); } ////// CopyTo /// /// /// public void CopyTo(Window[] array, int index) { _list.CopyTo(array, index); } ////// Count property /// public int Count { get { return _list.Count; } } ////// IsSynchronized /// public bool IsSynchronized { get { return _list.IsSynchronized; } } ////// SyncRoot /// public Object SyncRoot { get { return _list.SyncRoot; } } #endregion ICollection implementation //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal WindowCollection Clone() { WindowCollection clone; lock (_list.SyncRoot) { clone = new WindowCollection(_list.Count); for (int i = 0; i < _list.Count; i++) { clone._list.Add(_list[i]); } } return clone; } internal void Remove(Window win) { lock (_list.SyncRoot) { _list.Remove(win); } } internal void RemoveAt(int index) { lock (_list.SyncRoot) { _list.Remove(index); } } internal int Add (Window win) { lock (_list.SyncRoot) { return _list.Add(win); } } internal bool HasItem(Window win) { lock (_list.SyncRoot) { for (int i = 0; i < _list.Count; i++) { if (_list[i] == win) { return true; } } } return false; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private ArrayList _list; #endregion Private Fields } #endregion WindowCollection class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // WindowCollection can be used to interate over all the windows // that have been opened in the current application. // // History: // 08/10/04: kusumav Moved out of Application.cs to its own separate file. // //--------------------------------------------------------------------------- using System.Collections; using System.Diagnostics; namespace System.Windows { #region WindowCollection class ////// WindowCollection can be used to interate over all the windows that have been /// opened in the current application. /// // public sealed class WindowCollection : ICollection { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Default Constructor /// public WindowCollection() { _list = new ArrayList(1); } internal WindowCollection(int count) { Debug.Assert(count >= 0, "count must not be less than zero"); _list = new ArrayList(count); } #endregion Public Methods //------------------------------------------------------ // // Operator overload // //----------------------------------------------------- #region Operator overload ////// Overloaded [] operator to access the WindowCollection list /// public Window this[int index] { get { return _list[index] as Window; } } #endregion Operator overload //------------------------------------------------------ // // IEnumerable implementation // //------------------------------------------------------ #region IEnumerable implementation ////// GetEnumerator /// ///public IEnumerator GetEnumerator() { return _list.GetEnumerator(); } #endregion IEnumerable implementation //------------------------------------------------------- // // ICollection implementation (derives from IEnumerable) // //-------------------------------------------------------- #region ICollection implementation /// /// CopyTo /// /// /// void ICollection.CopyTo(Array array, int index) { _list.CopyTo(array, index); } ////// CopyTo /// /// /// public void CopyTo(Window[] array, int index) { _list.CopyTo(array, index); } ////// Count property /// public int Count { get { return _list.Count; } } ////// IsSynchronized /// public bool IsSynchronized { get { return _list.IsSynchronized; } } ////// SyncRoot /// public Object SyncRoot { get { return _list.SyncRoot; } } #endregion ICollection implementation //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal WindowCollection Clone() { WindowCollection clone; lock (_list.SyncRoot) { clone = new WindowCollection(_list.Count); for (int i = 0; i < _list.Count; i++) { clone._list.Add(_list[i]); } } return clone; } internal void Remove(Window win) { lock (_list.SyncRoot) { _list.Remove(win); } } internal void RemoveAt(int index) { lock (_list.SyncRoot) { _list.Remove(index); } } internal int Add (Window win) { lock (_list.SyncRoot) { return _list.Add(win); } } internal bool HasItem(Window win) { lock (_list.SyncRoot) { for (int i = 0; i < _list.Count; i++) { if (_list[i] == win) { return true; } } } return false; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private ArrayList _list; #endregion Private Fields } #endregion WindowCollection class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
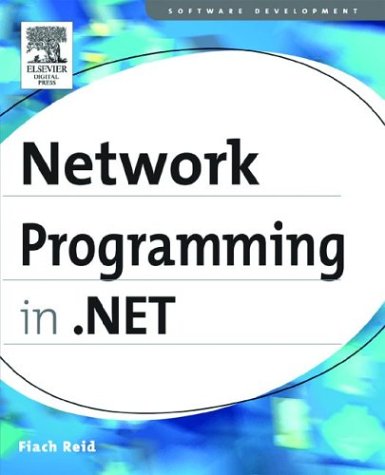
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AxisAngleRotation3D.cs
- HttpCachePolicy.cs
- CodeArgumentReferenceExpression.cs
- StateMachine.cs
- Publisher.cs
- ParameterToken.cs
- BitmapEffectInputData.cs
- Serializer.cs
- WebPartConnectionsConnectVerb.cs
- DoubleUtil.cs
- SecurityUniqueId.cs
- MultiTrigger.cs
- GPRECT.cs
- DebugView.cs
- StringUtil.cs
- XmlUtil.cs
- DatePickerAutomationPeer.cs
- AssemblyBuilder.cs
- DeclarativeCatalogPart.cs
- ListControlBuilder.cs
- WebBrowserSiteBase.cs
- WSHttpBindingElement.cs
- ThreadPool.cs
- IPGlobalProperties.cs
- ApplicationException.cs
- Setter.cs
- SchemaManager.cs
- CultureSpecificCharacterBufferRange.cs
- CrossSiteScriptingValidation.cs
- Pool.cs
- BindingManagerDataErrorEventArgs.cs
- OraclePermissionAttribute.cs
- QueryResponse.cs
- HeaderCollection.cs
- Simplifier.cs
- BufferModesCollection.cs
- FontInfo.cs
- UserControl.cs
- AccessedThroughPropertyAttribute.cs
- ServiceProviders.cs
- Bits.cs
- NotImplementedException.cs
- ListViewItemSelectionChangedEvent.cs
- CheckBoxStandardAdapter.cs
- SerializationEventsCache.cs
- ToolStripOverflow.cs
- MetadataItemSerializer.cs
- MessageLoggingElement.cs
- CompositeCollection.cs
- IMembershipProvider.cs
- XamlHostingSectionGroup.cs
- RevocationPoint.cs
- SortQuery.cs
- DelegateSerializationHolder.cs
- ObjectQueryState.cs
- WebPartDisplayMode.cs
- SoapFaultCodes.cs
- Visual3D.cs
- WorkflowMarkupSerializationProvider.cs
- DoubleUtil.cs
- GacUtil.cs
- ContentPresenter.cs
- Permission.cs
- LiteralDesigner.cs
- XmlSubtreeReader.cs
- StylesEditorDialog.cs
- RelatedEnd.cs
- Keywords.cs
- PauseStoryboard.cs
- FixedPosition.cs
- BuildTopDownAttribute.cs
- MenuItemBinding.cs
- AxisAngleRotation3D.cs
- BezierSegment.cs
- XPathDocumentBuilder.cs
- ColumnResizeAdorner.cs
- CompilationPass2TaskInternal.cs
- WinFormsUtils.cs
- XamlStream.cs
- PrtTicket_Public.cs
- ButtonAutomationPeer.cs
- CacheMemory.cs
- ObjectDataSourceEventArgs.cs
- DecoderFallbackWithFailureFlag.cs
- Button.cs
- CollectionType.cs
- XmlTypeMapping.cs
- OracleConnectionFactory.cs
- SafeThemeHandle.cs
- DispatcherExceptionFilterEventArgs.cs
- CompareValidator.cs
- DbCommandTree.cs
- EFColumnProvider.cs
- Size.cs
- FilteredDataSetHelper.cs
- LinkUtilities.cs
- DataSourceXmlAttributeAttribute.cs
- ResourceDefaultValueAttribute.cs
- Image.cs
- ChangeDirector.cs