Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / XamlBuildTask / Microsoft / Build / Tasks / Xaml / CompilationPass2TaskInternal.cs / 1606072 / CompilationPass2TaskInternal.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace Microsoft.Build.Tasks.Xaml { using System; using System.Collections.Generic; using System.IO; using System.Xaml; using System.Xaml.Schema; using System.Xml; using System.Reflection; using System.Runtime; internal class CompilationPass2TaskInternal : MarshalByRefObject, IExceptionLogger { IListapplicationMarkup; IList references; IList logData; public IList ApplicationMarkup { get { if (this.applicationMarkup == null) { this.applicationMarkup = new List (); } return this.applicationMarkup; } set { this.applicationMarkup = value; } } public string AssemblyName { get; set; } public string LocalAssemblyReference { get; set; } public string RootNamespace { get; set; } public IList LogData { get { if (this.logData == null) { this.logData = new List (); } return this.logData; } } public IList References { get { if (this.references == null) { this.references = new List (); } return this.references; } set { this.references = value; } } public bool Execute() { try { if (this.ApplicationMarkup == null || this.ApplicationMarkup.Count == 0) { return true; } IList loadedAssemblyList = null; if (this.References != null) { loadedAssemblyList = XamlBuildTaskServices.Load(this.References); } Assembly localAssembly = null; if (LocalAssemblyReference != null) { try { localAssembly = XamlBuildTaskServices.Load(LocalAssemblyReference); loadedAssemblyList.Add(localAssembly); } catch (FileNotFoundException e) { LogException(e, e.FileName); return false; } } AppDomain.CurrentDomain.ReflectionOnlyAssemblyResolve += new ResolveEventHandler(XamlBuildTaskServices.ReflectionOnlyAssemblyResolve); XamlNsReplacingContext wxsc = new XamlNsReplacingContext(loadedAssemblyList, localAssembly.GetName().Name, this.AssemblyName); bool foundValidationErrors = false; foreach (string app in ApplicationMarkup) { try { if (!ProcessMarkupItem(app, wxsc, localAssembly)) { foundValidationErrors = true; } } catch (Exception e) { if (Fx.IsFatal(e)) { throw; } LogException(e, app); return false; } } return !foundValidationErrors; } catch (Exception e) { if (Fx.IsFatal(e)) { throw; } // Log unknown errors that do not originate from the task. // Assumes that all known errors are logged when the exception is thrown. if (!(e is LoggedException)) { LogException(e); } return false; } } public Exception LogException(Exception exception, string fileName) { return LogException(exception, fileName, 0, 0); } public Exception LogException(Exception exception, string fileName, int lineNumber, int linePosition) { this.LogData.Add(new LogData() { Message = exception.Message, FileName = fileName, LineNumber = lineNumber, LinePosition = linePosition }); return new LoggedException(exception); } public Exception LogException(Exception exception) { this.LogData.Add(new LogData() { Message = exception.Message, LineNumber = 0, LinePosition = 0 }); return new LoggedException(exception); } bool ProcessMarkupItem(string markupItem, XamlNsReplacingContext wxsc, Assembly localAssembly) { XamlXmlReaderSettings settings = new XamlXmlReaderSettings() { LocalAssembly = localAssembly, ProvideLineInfo = true, AllowProtectedMembersOnRoot = true }; using (StreamReader streamReader = new StreamReader(markupItem)) { var xamlReader = new XamlXmlReader(XmlReader.Create(streamReader), wxsc, settings); ClassValidator validator = new ClassValidator(this, markupItem, localAssembly, this.RootNamespace); IList validationErrors = null; if (validator.ValidateXaml(xamlReader, false, this.AssemblyName, out validationErrors)) { return true; } else { foreach (LogData logData in validationErrors) { this.LogData.Add(logData); } return false; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
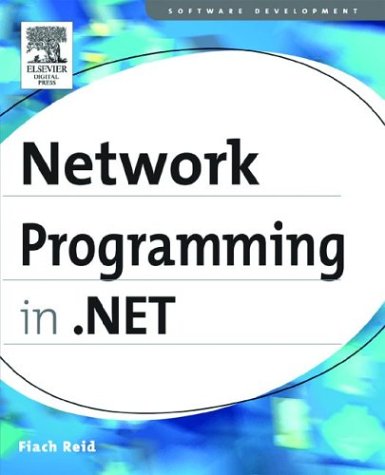
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlErrorCollection.cs
- ImageMap.cs
- ExitEventArgs.cs
- SqlSelectStatement.cs
- SimpleTypeResolver.cs
- XmlQualifiedName.cs
- DataBindingHandlerAttribute.cs
- RuntimeResourceSet.cs
- DeobfuscatingStream.cs
- ActivityBuilder.cs
- TargetPerspective.cs
- ControlPager.cs
- PriorityBinding.cs
- MissingSatelliteAssemblyException.cs
- TreeNodeBindingCollection.cs
- DataViewSetting.cs
- Drawing.cs
- AuthenticationService.cs
- EventProxy.cs
- EventRouteFactory.cs
- ObjectDataSource.cs
- ComponentEditorPage.cs
- XmlNodeComparer.cs
- VerificationAttribute.cs
- ItemsPanelTemplate.cs
- CompiledQueryCacheKey.cs
- WindowsUpDown.cs
- Baml2006Reader.cs
- ResourceDisplayNameAttribute.cs
- UIntPtr.cs
- QueryStringParameter.cs
- InertiaTranslationBehavior.cs
- TraceEventCache.cs
- MethodBody.cs
- ExtractCollection.cs
- PublishLicense.cs
- Vector3DAnimationUsingKeyFrames.cs
- XmlEncodedRawTextWriter.cs
- HttpListenerPrefixCollection.cs
- MobileTemplatedControlDesigner.cs
- ClientTarget.cs
- uribuilder.cs
- JobPageOrder.cs
- FixedBufferAttribute.cs
- BaseWebProxyFinder.cs
- sqlstateclientmanager.cs
- AnimationClock.cs
- TimeSpanConverter.cs
- ParenthesizePropertyNameAttribute.cs
- CombinedGeometry.cs
- AssemblyName.cs
- DirectoryObjectSecurity.cs
- LicenseProviderAttribute.cs
- XPathSingletonIterator.cs
- EmptyStringExpandableObjectConverter.cs
- Constants.cs
- XmlChildNodes.cs
- RowType.cs
- _HTTPDateParse.cs
- Size.cs
- ToolStripContentPanelDesigner.cs
- WebPartDescriptionCollection.cs
- TypeBrowserDialog.cs
- ComponentConverter.cs
- WindowsToolbarAsMenu.cs
- EntityDataSourceQueryBuilder.cs
- SqlAliaser.cs
- Item.cs
- WebPartDescription.cs
- UriSectionData.cs
- CallId.cs
- ClaimTypes.cs
- safelink.cs
- TcpClientSocketManager.cs
- FastPropertyAccessor.cs
- BulletedListEventArgs.cs
- CheckBoxPopupAdapter.cs
- IPAddressCollection.cs
- DesigntimeLicenseContext.cs
- WindowsPrincipal.cs
- ProfessionalColorTable.cs
- FormViewRow.cs
- Command.cs
- TransformerTypeCollection.cs
- GridLengthConverter.cs
- JoinGraph.cs
- ResourcePermissionBaseEntry.cs
- Message.cs
- PartialTrustVisibleAssembliesSection.cs
- Point.cs
- SemanticBasicElement.cs
- HttpResponse.cs
- PartialArray.cs
- StylusDownEventArgs.cs
- DuplicateWaitObjectException.cs
- ToolBarOverflowPanel.cs
- FlowDocumentView.cs
- HMACRIPEMD160.cs
- FixedSOMLineCollection.cs
- ProcessExitedException.cs