Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / EntityTypeBase.cs / 1 / EntityTypeBase.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Diagnostics; using System.Text; namespace System.Data.Metadata.Edm { ////// Represents the Entity Type /// public abstract class EntityTypeBase : StructuralType { #region Constructors ////// Initializes a new instance of Entity Type /// /// name of the entity type /// namespace of the entity type /// version of the entity type /// dataSpace in which this edmtype belongs to ///Thrown if either name, namespace or version arguments are null internal EntityTypeBase(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { _keyMembers = new ReadOnlyMetadataCollection(new MetadataCollection ()); } #endregion #region Fields private readonly ReadOnlyMetadataCollection _keyMembers; private string[] _keyMemberNames; #endregion #region Properties /// /// Returns the list of all the key members for this entity type /// [MetadataProperty(BuiltInTypeKind.EdmMember, true)] public ReadOnlyMetadataCollectionKeyMembers { get { // Since we allow entity types with no keys, we should first check if there are // keys defined on the base class. If yes, then return the keys otherwise, return // the keys defined on this class if (this.BaseType != null && ((EntityTypeBase)this.BaseType).KeyMembers.Count != 0) { Debug.Assert(_keyMembers.Count == 0, "Since the base type have keys, current type cannot have keys defined"); return ((EntityTypeBase)this.BaseType).KeyMembers; } else { return _keyMembers; } } } /// /// Returns the list of the member names that form the key for this entity type /// Perf Bug #529294: To cache the list of member names that form the key for the entity type /// internal string[] KeyMemberNames { get { String[] keyNames = _keyMemberNames; if (keyNames == null) { keyNames = new string[this.KeyMembers.Count]; for (int i = 0; i < keyNames.Length; i++) { keyNames[i] = this.KeyMembers[i].Name; } _keyMemberNames = keyNames; } Debug.Assert(_keyMemberNames.Length == this.KeyMembers.Count, "This list is out of [....] with the key members count. This property was called before all the keymembers were added"); return _keyMemberNames; } } #endregion #region Methods ////// Returns the list of all the key members for this entity type /// ///if member argument is null ///Thrown if the EntityType has a base type of another EntityTypeBase. In this case KeyMembers should be added to the base type ///If the EntityType instance is in ReadOnly state internal void AddKeyMember(EdmMember member) { EntityUtil.GenericCheckArgumentNull(member, "member"); Util.ThrowIfReadOnly(this); Debug.Assert(this.BaseType == null || ((EntityTypeBase)this.BaseType).KeyMembers.Count == 0, "Key cannot be added if there is a basetype with keys"); if (!Members.Contains(member)) { this.AddMember(member); } _keyMembers.Source.Add(member); } ////// Makes this property readonly /// internal override void SetReadOnly() { if (!IsReadOnly) { _keyMembers.Source.SetReadOnly(); base.SetReadOnly(); } } ////// Checks for each property to be non-null and then adds it to the member collection /// /// members for this type /// the membersCollection to which the members should be added internal static void CheckAndAddMembers(IEnumerablemembers, EntityType entityType) { foreach (EdmMember member in members) { // Check for each property to be non-null if (null == member) throw EntityUtil.CollectionParameterElementIsNull("members"); // Add the property to the member collection entityType.AddMember(member); } } /// /// Checks for each key member to be non-null /// also check for it to be present in the members collection /// and then adds it to the KeyMembers collection. /// /// Throw if the key member is not already in the members /// collection. Cannot do much other than that as the /// Key members is just an Ienumerable of the names /// of the members. /// /// the list of keys (member names) to be added for the given type internal void CheckAndAddKeyMembers(IEnumerablekeyMembers) { foreach (string keyMember in keyMembers) { // Check for each keymember to be non-null if (null == keyMember) { throw EntityUtil.CollectionParameterElementIsNull("keyMembers"); } // Check for whether the key exists in the members collection EdmMember member; if (!Members.TryGetValue(keyMember, false, out member)) { throw EntityUtil.Argument(System.Data.Entity.Strings.InvalidKeyMember(keyMember)); //--- to do, identify the right exception to throw here } // Add the key member to the key member collection AddKeyMember(member); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Diagnostics; using System.Text; namespace System.Data.Metadata.Edm { ////// Represents the Entity Type /// public abstract class EntityTypeBase : StructuralType { #region Constructors ////// Initializes a new instance of Entity Type /// /// name of the entity type /// namespace of the entity type /// version of the entity type /// dataSpace in which this edmtype belongs to ///Thrown if either name, namespace or version arguments are null internal EntityTypeBase(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { _keyMembers = new ReadOnlyMetadataCollection(new MetadataCollection ()); } #endregion #region Fields private readonly ReadOnlyMetadataCollection _keyMembers; private string[] _keyMemberNames; #endregion #region Properties /// /// Returns the list of all the key members for this entity type /// [MetadataProperty(BuiltInTypeKind.EdmMember, true)] public ReadOnlyMetadataCollectionKeyMembers { get { // Since we allow entity types with no keys, we should first check if there are // keys defined on the base class. If yes, then return the keys otherwise, return // the keys defined on this class if (this.BaseType != null && ((EntityTypeBase)this.BaseType).KeyMembers.Count != 0) { Debug.Assert(_keyMembers.Count == 0, "Since the base type have keys, current type cannot have keys defined"); return ((EntityTypeBase)this.BaseType).KeyMembers; } else { return _keyMembers; } } } /// /// Returns the list of the member names that form the key for this entity type /// Perf Bug #529294: To cache the list of member names that form the key for the entity type /// internal string[] KeyMemberNames { get { String[] keyNames = _keyMemberNames; if (keyNames == null) { keyNames = new string[this.KeyMembers.Count]; for (int i = 0; i < keyNames.Length; i++) { keyNames[i] = this.KeyMembers[i].Name; } _keyMemberNames = keyNames; } Debug.Assert(_keyMemberNames.Length == this.KeyMembers.Count, "This list is out of [....] with the key members count. This property was called before all the keymembers were added"); return _keyMemberNames; } } #endregion #region Methods ////// Returns the list of all the key members for this entity type /// ///if member argument is null ///Thrown if the EntityType has a base type of another EntityTypeBase. In this case KeyMembers should be added to the base type ///If the EntityType instance is in ReadOnly state internal void AddKeyMember(EdmMember member) { EntityUtil.GenericCheckArgumentNull(member, "member"); Util.ThrowIfReadOnly(this); Debug.Assert(this.BaseType == null || ((EntityTypeBase)this.BaseType).KeyMembers.Count == 0, "Key cannot be added if there is a basetype with keys"); if (!Members.Contains(member)) { this.AddMember(member); } _keyMembers.Source.Add(member); } ////// Makes this property readonly /// internal override void SetReadOnly() { if (!IsReadOnly) { _keyMembers.Source.SetReadOnly(); base.SetReadOnly(); } } ////// Checks for each property to be non-null and then adds it to the member collection /// /// members for this type /// the membersCollection to which the members should be added internal static void CheckAndAddMembers(IEnumerablemembers, EntityType entityType) { foreach (EdmMember member in members) { // Check for each property to be non-null if (null == member) throw EntityUtil.CollectionParameterElementIsNull("members"); // Add the property to the member collection entityType.AddMember(member); } } /// /// Checks for each key member to be non-null /// also check for it to be present in the members collection /// and then adds it to the KeyMembers collection. /// /// Throw if the key member is not already in the members /// collection. Cannot do much other than that as the /// Key members is just an Ienumerable of the names /// of the members. /// /// the list of keys (member names) to be added for the given type internal void CheckAndAddKeyMembers(IEnumerablekeyMembers) { foreach (string keyMember in keyMembers) { // Check for each keymember to be non-null if (null == keyMember) { throw EntityUtil.CollectionParameterElementIsNull("keyMembers"); } // Check for whether the key exists in the members collection EdmMember member; if (!Members.TryGetValue(keyMember, false, out member)) { throw EntityUtil.Argument(System.Data.Entity.Strings.InvalidKeyMember(keyMember)); //--- to do, identify the right exception to throw here } // Add the key member to the key member collection AddKeyMember(member); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
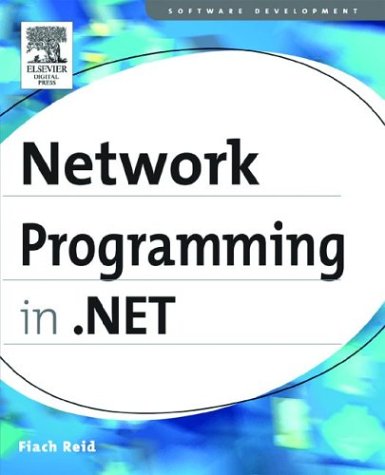
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StandardBindingElementCollection.cs
- GlyphRunDrawing.cs
- SiblingIterators.cs
- ObservableDictionary.cs
- AppliedDeviceFiltersDialog.cs
- xamlnodes.cs
- DataGridTable.cs
- cookiecontainer.cs
- HandleTable.cs
- RowToParametersTransformer.cs
- ToolZone.cs
- Group.cs
- BatchParser.cs
- AssociationTypeEmitter.cs
- ToolStripItemEventArgs.cs
- DataGridColumn.cs
- MenuBindingsEditorForm.cs
- StandardOleMarshalObject.cs
- WindowsSlider.cs
- StaticTextPointer.cs
- ThumbAutomationPeer.cs
- PersistChildrenAttribute.cs
- CurrentChangingEventManager.cs
- Switch.cs
- ColorAnimationBase.cs
- DrawingServices.cs
- XamlToRtfWriter.cs
- LocalizableResourceBuilder.cs
- KeyManager.cs
- HtmlTableCellCollection.cs
- CultureInfo.cs
- PublisherIdentityPermission.cs
- MetadataArtifactLoaderFile.cs
- ListControl.cs
- ContainerFilterService.cs
- InvalidPropValue.cs
- ContextStaticAttribute.cs
- EntityDataSourceViewSchema.cs
- ProcessModelInfo.cs
- GridPatternIdentifiers.cs
- SQlBooleanStorage.cs
- CodeSnippetStatement.cs
- hebrewshape.cs
- ValidatedControlConverter.cs
- NamedPermissionSet.cs
- AnnotationAdorner.cs
- ColorContextHelper.cs
- ZoneMembershipCondition.cs
- PageCodeDomTreeGenerator.cs
- ValueQuery.cs
- TextReader.cs
- DesignerAutoFormatStyle.cs
- HtmlInputSubmit.cs
- PageClientProxyGenerator.cs
- RectangleGeometry.cs
- TraceHwndHost.cs
- PostBackTrigger.cs
- CompositeControl.cs
- RunInstallerAttribute.cs
- EntityTypeBase.cs
- SyndicationItem.cs
- GridViewDeletedEventArgs.cs
- MexBindingElement.cs
- TextDecoration.cs
- FontNameEditor.cs
- ProxyHwnd.cs
- UriWriter.cs
- _IPv4Address.cs
- shaperfactoryquerycachekey.cs
- DocumentGridContextMenu.cs
- SoapElementAttribute.cs
- Aggregates.cs
- ReaderContextStackData.cs
- HtmlFormWrapper.cs
- LocationChangedEventArgs.cs
- AnnotationObservableCollection.cs
- MarshalByRefObject.cs
- DocumentGridContextMenu.cs
- PropertyCollection.cs
- ActivityDesignerAccessibleObject.cs
- WebService.cs
- XmlQueryTypeFactory.cs
- TreeNodeEventArgs.cs
- FilterException.cs
- StrokeRenderer.cs
- base64Transforms.cs
- InstanceDescriptor.cs
- TimeSpanFormat.cs
- TimeSpanStorage.cs
- Gdiplus.cs
- SHA384Managed.cs
- XamlValidatingReader.cs
- XXXOnTypeBuilderInstantiation.cs
- SqlLiftWhereClauses.cs
- unsafenativemethodsother.cs
- ResourceDescriptionAttribute.cs
- TrackingProfileSerializer.cs
- DataGridRow.cs
- WindowsFormsHelpers.cs
- XmlWriterSettings.cs