Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Collections / Concurrent / IProducerConsumerCollection.cs / 1305376 / IProducerConsumerCollection.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // IProducerConsumerCollection.cs // //[....] // // A common interface for all concurrent collections. // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections; using System.Collections.Generic; using System.Diagnostics; namespace System.Collections.Concurrent { ////// Defines methods to manipulate thread-safe collections intended for producer/consumer usage. /// ///Specifies the type of elements in the collection. ////// All implementations of this interface must enable all members of this interface /// to be used concurrently from multiple threads. /// public interface IProducerConsumerCollection: IEnumerable , ICollection { /// /// Copies the elements of the /// The one-dimensionalto /// an /// , starting at a specified index. /// that is the destination of /// the elements copied from the . /// The array must have zero-based indexing. /// The zero-based index in at which copying /// begins. /// /// is a null reference (Nothing in /// Visual Basic). /// is less than /// zero. void CopyTo(T[] array, int index); /// is equal to or greater than the /// length of the /// -or- The number of elements in the source is greater than the /// available space from to the end of the destination . /// /// Attempts to add an object to the /// The object to add to the. /// . /// true if the object was added successfully; otherwise, false. ///The bool TryAdd(T item); ///was invalid for this collection. /// Attempts to remove and return an object from the /// /// When this method returns, if the object was removed and returned successfully,. /// contains the removed object. If no object was available to be removed, the value is /// unspecified. /// /// true if an object was removed and returned successfully; otherwise, false. bool TryTake(out T item); ////// Copies the elements contained in the ///to a new array. /// A new array containing the elements copied from the T[] ToArray(); } ///. /// A debugger view of the IProducerConsumerCollection that makes it simple to browse the /// collection's contents at a point in time. /// ///The type of elements stored within. internal sealed class SystemCollectionsConcurrent_ProducerConsumerCollectionDebugView{ private IProducerConsumerCollection m_collection; // The collection being viewed. /// /// Constructs a new debugger view object for the provided collection object. /// /// A collection to browse in the debugger. public SystemCollectionsConcurrent_ProducerConsumerCollectionDebugView(IProducerConsumerCollectioncollection) { if (collection == null) { throw new ArgumentNullException("collection"); } m_collection = collection; } /// /// Returns a snapshot of the underlying collection's elements. /// [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { return m_collection.ToArray(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // IProducerConsumerCollection.cs // //[....] // // A common interface for all concurrent collections. // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections; using System.Collections.Generic; using System.Diagnostics; namespace System.Collections.Concurrent { ////// Defines methods to manipulate thread-safe collections intended for producer/consumer usage. /// ///Specifies the type of elements in the collection. ////// All implementations of this interface must enable all members of this interface /// to be used concurrently from multiple threads. /// public interface IProducerConsumerCollection: IEnumerable , ICollection { /// /// Copies the elements of the /// The one-dimensionalto /// an /// , starting at a specified index. /// that is the destination of /// the elements copied from the . /// The array must have zero-based indexing. /// The zero-based index in at which copying /// begins. /// /// is a null reference (Nothing in /// Visual Basic). /// is less than /// zero. void CopyTo(T[] array, int index); /// is equal to or greater than the /// length of the /// -or- The number of elements in the source is greater than the /// available space from to the end of the destination . /// /// Attempts to add an object to the /// The object to add to the. /// . /// true if the object was added successfully; otherwise, false. ///The bool TryAdd(T item); ///was invalid for this collection. /// Attempts to remove and return an object from the /// /// When this method returns, if the object was removed and returned successfully,. /// contains the removed object. If no object was available to be removed, the value is /// unspecified. /// /// true if an object was removed and returned successfully; otherwise, false. bool TryTake(out T item); ////// Copies the elements contained in the ///to a new array. /// A new array containing the elements copied from the T[] ToArray(); } ///. /// A debugger view of the IProducerConsumerCollection that makes it simple to browse the /// collection's contents at a point in time. /// ///The type of elements stored within. internal sealed class SystemCollectionsConcurrent_ProducerConsumerCollectionDebugView{ private IProducerConsumerCollection m_collection; // The collection being viewed. /// /// Constructs a new debugger view object for the provided collection object. /// /// A collection to browse in the debugger. public SystemCollectionsConcurrent_ProducerConsumerCollectionDebugView(IProducerConsumerCollectioncollection) { if (collection == null) { throw new ArgumentNullException("collection"); } m_collection = collection; } /// /// Returns a snapshot of the underlying collection's elements. /// [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { return m_collection.ToArray(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
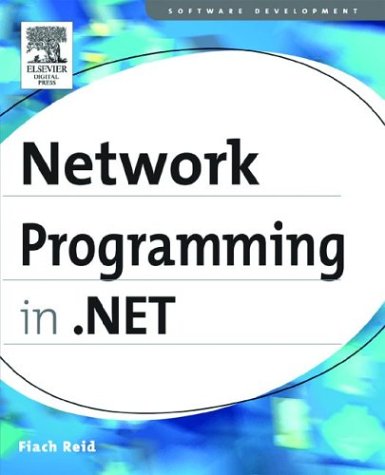
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymbolMethod.cs
- CallbackValidatorAttribute.cs
- ClientBuildManager.cs
- MaterializeFromAtom.cs
- XPathMultyIterator.cs
- SqlExpressionNullability.cs
- SettingsPropertyValue.cs
- SamlAuthorityBinding.cs
- SemaphoreSecurity.cs
- EntityDataSourceValidationException.cs
- AdjustableArrowCap.cs
- ValidatorCollection.cs
- XamlClipboardData.cs
- DataGridViewColumn.cs
- InputBinding.cs
- Paragraph.cs
- CompilerTypeWithParams.cs
- PeerNameRegistration.cs
- Table.cs
- DynamicRendererThreadManager.cs
- HttpErrorTraceRecord.cs
- Accessible.cs
- Lasso.cs
- SecurityHelper.cs
- DataPointer.cs
- AssociatedControlConverter.cs
- ConnectionStringEditor.cs
- CompositeClientFormatter.cs
- WebPartDisplayMode.cs
- TextViewBase.cs
- XmlDictionaryString.cs
- BinaryMessageEncodingElement.cs
- BitmapCodecInfoInternal.cs
- x509utils.cs
- ListParaClient.cs
- EventSinkHelperWriter.cs
- Set.cs
- TrackingMemoryStream.cs
- MethodBody.cs
- ConstantCheck.cs
- DetailsView.cs
- MarkerProperties.cs
- SystemEvents.cs
- _HeaderInfoTable.cs
- ComplexBindingPropertiesAttribute.cs
- PlainXmlSerializer.cs
- LedgerEntryCollection.cs
- SecurityRuntime.cs
- PropertyItemInternal.cs
- EventSource.cs
- IList.cs
- FocusTracker.cs
- WCFModelStrings.Designer.cs
- AtomMaterializerLog.cs
- DataGridViewColumnTypeEditor.cs
- TextTreeFixupNode.cs
- SystemIPAddressInformation.cs
- GB18030Encoding.cs
- DllNotFoundException.cs
- InkCanvasInnerCanvas.cs
- AsyncPostBackTrigger.cs
- IdentityNotMappedException.cs
- ZoneIdentityPermission.cs
- validationstate.cs
- BitConverter.cs
- ResourceReferenceExpressionConverter.cs
- ZipIOExtraFieldZip64Element.cs
- ToolStripTextBox.cs
- ProtectedConfiguration.cs
- SqlClientWrapperSmiStreamChars.cs
- ObjectSpanRewriter.cs
- PropertyMetadata.cs
- ContextInformation.cs
- ExtendedProtectionPolicy.cs
- HtmlControlDesigner.cs
- StylusPointPropertyInfo.cs
- Focus.cs
- ByteAnimation.cs
- SharedPersonalizationStateInfo.cs
- InternalResources.cs
- MetabaseServerConfig.cs
- SafeNativeMethodsMilCoreApi.cs
- ConfigurationElementProperty.cs
- Context.cs
- BindToObject.cs
- RegexCompilationInfo.cs
- Parser.cs
- EditingMode.cs
- AutoGeneratedFieldProperties.cs
- GestureRecognitionResult.cs
- CodeFieldReferenceExpression.cs
- QueryExpr.cs
- XmlSchemaAnnotated.cs
- ExecutionPropertyManager.cs
- SortedList.cs
- XmlAggregates.cs
- ControlEvent.cs
- LinqDataSourceValidationException.cs
- FormViewCommandEventArgs.cs
- EntityClientCacheKey.cs