Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Validation / KeyConstraint.cs / 1 / KeyConstraint.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Text; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.Validation { // Class representing a key constraint for particular cellrelation internal class KeyConstraint: InternalBase where TCellRelation : CellRelation { #region Constructor // Constructs a key constraint for the given relation and keyslots // with comparer being the comparison operator for comparing various // keyslots in Implies, etc internal KeyConstraint(TCellRelation relation, IEnumerable keySlots, IEqualityComparer comparer) { m_relation = relation; m_keySlots = new Set (keySlots, comparer).MakeReadOnly(); Debug.Assert(m_keySlots.Count > 0, "Key constraint being created without any keyslots?"); } #endregion #region Fields private TCellRelation m_relation; private Set m_keySlots; #endregion #region Properties protected TCellRelation CellRelation { get { return m_relation; } } protected Set KeySlots { get { return m_keySlots;} } #endregion #region Methods internal override void ToCompactString(StringBuilder builder) { StringUtil.FormatStringBuilder(builder, "Key (V{0}) - ", m_relation.CellNumber); StringUtil.ToSeparatedStringSorted(builder, KeySlots, ", "); // The slots contain the name of the relation: So we skip // printing the CellRelation } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Text; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.Validation { // Class representing a key constraint for particular cellrelation internal class KeyConstraint: InternalBase where TCellRelation : CellRelation { #region Constructor // Constructs a key constraint for the given relation and keyslots // with comparer being the comparison operator for comparing various // keyslots in Implies, etc internal KeyConstraint(TCellRelation relation, IEnumerable keySlots, IEqualityComparer comparer) { m_relation = relation; m_keySlots = new Set (keySlots, comparer).MakeReadOnly(); Debug.Assert(m_keySlots.Count > 0, "Key constraint being created without any keyslots?"); } #endregion #region Fields private TCellRelation m_relation; private Set m_keySlots; #endregion #region Properties protected TCellRelation CellRelation { get { return m_relation; } } protected Set KeySlots { get { return m_keySlots;} } #endregion #region Methods internal override void ToCompactString(StringBuilder builder) { StringUtil.FormatStringBuilder(builder, "Key (V{0}) - ", m_relation.CellNumber); StringUtil.ToSeparatedStringSorted(builder, KeySlots, ", "); // The slots contain the name of the relation: So we skip // printing the CellRelation } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
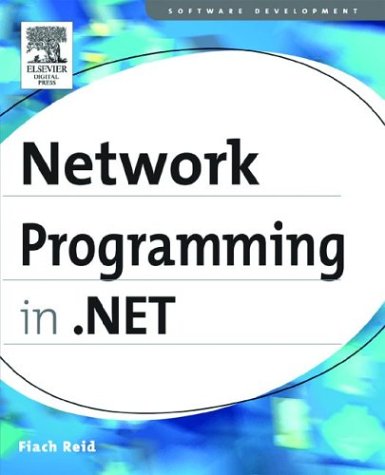
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompensationTokenData.cs
- GenericTextProperties.cs
- XmlText.cs
- IdentityNotMappedException.cs
- TableItemStyle.cs
- Type.cs
- MexNamedPipeBindingCollectionElement.cs
- Source.cs
- CollectionsUtil.cs
- ToolTipService.cs
- DbMetaDataColumnNames.cs
- ApplicationServiceManager.cs
- PropertyGrid.cs
- Rule.cs
- TypeDependencyAttribute.cs
- SoapExtensionTypeElementCollection.cs
- ChannelTracker.cs
- ADConnectionHelper.cs
- PropertyInformation.cs
- PDBReader.cs
- ComboBoxDesigner.cs
- CharConverter.cs
- NetworkInterface.cs
- TextFormatterImp.cs
- AccessibleObject.cs
- DateTimeStorage.cs
- EventToken.cs
- DbParameterHelper.cs
- HtmlAnchor.cs
- OverflowException.cs
- FigureParagraph.cs
- BitmapMetadataEnumerator.cs
- HostProtectionPermission.cs
- Model3DGroup.cs
- baseshape.cs
- DBAsyncResult.cs
- SecureEnvironment.cs
- FillRuleValidation.cs
- Encoder.cs
- linebase.cs
- BindingExpressionUncommonField.cs
- TextCharacters.cs
- DSACryptoServiceProvider.cs
- FormConverter.cs
- UpdatePanelTrigger.cs
- MatrixCamera.cs
- TimeStampChecker.cs
- HotSpot.cs
- EditorZoneBase.cs
- PersonalizationAdministration.cs
- MultiTrigger.cs
- CompilerResults.cs
- DataGridViewElement.cs
- ProfileSettingsCollection.cs
- MediaScriptCommandRoutedEventArgs.cs
- DbProviderSpecificTypePropertyAttribute.cs
- FixedTextSelectionProcessor.cs
- _SecureChannel.cs
- BinaryObjectInfo.cs
- FlowDocumentPage.cs
- Geometry3D.cs
- SerializationAttributes.cs
- HybridDictionary.cs
- XmlSchemaObjectCollection.cs
- InvalidPrinterException.cs
- TileBrush.cs
- UniqueSet.cs
- TypeToTreeConverter.cs
- Code.cs
- UpDownEvent.cs
- BaseDataBoundControl.cs
- CellConstantDomain.cs
- EventWaitHandleSecurity.cs
- X509ChainPolicy.cs
- ObjectStateFormatter.cs
- CurrencyWrapper.cs
- FixedHighlight.cs
- ServiceNotStartedException.cs
- MessageBodyMemberAttribute.cs
- ConnectionPointCookie.cs
- GlobalEventManager.cs
- XmlAttributeProperties.cs
- ServerProtocol.cs
- RequestCache.cs
- MSG.cs
- BinaryReader.cs
- CharEntityEncoderFallback.cs
- ReflectPropertyDescriptor.cs
- iisPickupDirectory.cs
- EncryptedXml.cs
- DtrList.cs
- AlignmentYValidation.cs
- QuaternionKeyFrameCollection.cs
- TypeGeneratedEventArgs.cs
- DataGridTextBox.cs
- ButtonPopupAdapter.cs
- DesignerActionVerbList.cs
- CodeActivityContext.cs
- VectorCollection.cs
- _NegoStream.cs