Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / SrgsGrammar / SrgsGrammar.cs / 1 / SrgsGrammar.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Globalization; using System.Speech.Internal; using System.Speech.Internal.SrgsCompiler; using System.Speech.Internal.SrgsParser; using System.Xml; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. #pragma warning disable 56500 // Remove all the catch all statements warnings used by the interop layer namespace System.Speech.Recognition.SrgsGrammar { /// |summary| /// Summary description for Grammar. /// |/summary| [Serializable] internal sealed class SrgsGrammar : IGrammar { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// Initializes a new instance of the Grammar class. /// internal SrgsGrammar () { _rules = new SrgsRulesCollection (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods /// |summary| /// Write the XML fragment describing the object. /// |/summary| /// |param name="writer"|XmlWriter to which to write the XML fragment.|/param| internal void WriteSrgs (XmlWriter writer) { // Writewriter.WriteStartElement ("grammar", XmlParser.srgsNamespace); writer.WriteAttributeString ("xml", "lang", null, _culture.ToString ()); if (_root != null) { writer.WriteAttributeString ("root", _root.Id); } #if !NO_STG // Write the attributes for strongly typed grammars WriteSTGAttributes (writer); #endif if (_isModeSet) { switch (_mode) { case SrgsGrammarMode.Voice: writer.WriteAttributeString ("mode", "voice"); break; case SrgsGrammarMode.Dtmf: writer.WriteAttributeString ("mode", "dtmf"); break; } } // Write the tag format if any string tagFormat = null; switch (_tagFormat) { case SrgsTagFormat.Default: // Nothing to do break; case SrgsTagFormat.MssV1: tagFormat = "semantics-ms/1.0"; break; case SrgsTagFormat.W3cV1: tagFormat = "semantics/1.0"; break; case SrgsTagFormat.KeyValuePairs: tagFormat = "properties-ms/1.0"; break; default: System.Diagnostics.Debug.Assert (false, "Unknown Tag Format!!!"); break; } if (tagFormat != null) { writer.WriteAttributeString ("tag-format", tagFormat); } // Write the Alphabet type if not SAPI if (_hasPhoneticAlphabetBeenSet || (_phoneticAlphabet != SrgsPhoneticAlphabet.Sapi && HasPronunciation)) { string alphabet = _phoneticAlphabet == SrgsPhoneticAlphabet.Ipa ? "ipa" : _phoneticAlphabet == SrgsPhoneticAlphabet.Ups ? "x-microsoft-ups" : "x-microsoft-sapi"; writer.WriteAttributeString ("sapi", "alphabet", XmlParser.sapiNamespace, alphabet); } if (_xmlBase != null) { writer.WriteAttributeString ("xml:base", _xmlBase.ToString ()); } writer.WriteAttributeString ("version", "1.0"); writer.WriteAttributeString ("xmlns", XmlParser.srgsNamespace); if (_isSapiExtensionUsed) { writer.WriteAttributeString ("xmlns", "sapi", null, XmlParser.sapiNamespace); } foreach (SrgsRule rule in _rules) { // Validate child _rules rule.Validate (this); } // Write the tag elements if any foreach (string tag in _globalTags) { writer.WriteElementString ("tag", tag); } //Write the the references to the referenced assemblies and the various scripts WriteGrammarElements (writer); writer.WriteEndElement (); } /// |summary| /// Validate the SRGS element. /// |/summary| internal void Validate () { // Validation set the pronunciation so reset it to zero HasPronunciation = HasSapiExtension = false; // validate all the rules foreach (SrgsRule rule in _rules) { // Validate child _rules rule.Validate (this); } // Initial values for ContainsCOde and SapiExtensionUsed. _isSapiExtensionUsed |= HasPronunciation; #if !NO_STG _fContainsCode |= _language != null || _script.Length > 0 || _usings.Count > 0 || _assemblyReferences.Count > 0 || _codebehind.Count > 0 || _namespace != null || _fDebug; _isSapiExtensionUsed |= _fContainsCode; #endif // If the grammar contains no pronunciations, set the phonetic alphabet to SAPI. // This way, the CFG data can be loaded by SAPI 5.1. if (!HasPronunciation) { PhoneticAlphabet = AlphabetType.Sapi; } // Validate root rule reference if (_root != null) { if (!_rules.Contains (_root)) { XmlParser.ThrowSrgsException (SRID.RootNotDefined, _root.Id); } } if (_globalTags.Count > 0) { _tagFormat = SrgsTagFormat.W3cV1; } #if !NO_STG // Force the tag format to Sapi fprperties if .Net semantics are used. if (_fContainsCode) { if (_tagFormat == SrgsTagFormat.Default) { _tagFormat = SrgsTagFormat.KeyValuePairs; } // SAPI semantics only for .Net Semantics if (_tagFormat != SrgsTagFormat.KeyValuePairs) { XmlParser.ThrowSrgsException (SRID.InvalidSemanticProcessingType); } } #endif } IRule IGrammar.CreateRule (string id, RulePublic publicRule, RuleDynamic dynamic, bool hasScript) { SrgsRule rule = new SrgsRule (id); if (publicRule != RulePublic.NotSet) { rule.Scope = publicRule == RulePublic.True ? SrgsRuleScope.Public : SrgsRuleScope.Private; } rule.Dynamic = dynamic; return (IRule) rule; } void IElement.PostParse (IElement parent) { // Check that the root rule is defined if (_sRoot != null) { bool found = false; foreach (SrgsRule rule in Rules) { if (rule.Id == _sRoot) { Root = rule; found = true; break; } } if (!found) { // "Root rule ""%s"" is undefined." XmlParser.ThrowSrgsException (SRID.RootNotDefined, _sRoot); } } #if !NO_STG // Resolve the references to the scripts foreach (XmlParser.ForwardReference script in _scriptsForwardReference) { SrgsRule rule = Rules [script._name]; if (rule != null) { rule.Script = rule.Script + script._value; } else { XmlParser.ThrowSrgsException (SRID.InvalidScriptDefinition); } } #endif // Validate the whole grammar Validate (); } #if !NO_STG #pragma warning disable 56507 // check for null or empty strings // Add a script to this grammar or to a rule internal void AddScript (string rule, string code) { if (rule == null) { _script += code; } else { _scriptsForwardReference.Add (new XmlParser.ForwardReference (rule, code)); } } #endif #pragma warning restore 56507 #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties /// /// Sets the Root element /// ///string IGrammar.Root { set { _sRoot = value; } get { return _sRoot; } } /// |summary| /// Base URI of grammar (xml:base) /// |/summary| /// |remarks| /// public Uri XmlBase { get { return _xmlBase; } set { _xmlBase = value; } } /// |summary| /// Grammar language (xml:lang) /// |/summary| public CultureInfo Culture { get { return _culture; } set { Helpers.ThrowIfNull (value, "value"); _culture = value; } } /// |summary| /// Grammar mode. voice or dtmf /// |/summary| public GrammarType Mode { get { return _mode == SrgsGrammarMode.Voice ? GrammarType.VoiceGrammar : GrammarType.DtmfGrammar; } set { _mode = value == GrammarType.VoiceGrammar ? SrgsGrammarMode.Voice : SrgsGrammarMode.Dtmf; _isModeSet = true; } } /// |summary| /// Pronunciation Alphabet, IPA or SAPI or UPS /// |/summary| public AlphabetType PhoneticAlphabet { get { return (AlphabetType) _phoneticAlphabet; } set { _phoneticAlphabet = (SrgsPhoneticAlphabet) value; } } /// |summary|root /// Root rule (srgs:root) /// |/summary| public SrgsRule Root { get { return _root; } set { _root = value; } } /// |summary| /// Tag format (srgs:tag-format) /// |/summary| public SrgsTagFormat TagFormat { get { return _tagFormat; } set { _tagFormat = value; } } /// |summary| /// Tag format (srgs:tag-format) /// |/summary| public Collection GlobalTags { get { return _globalTags; } set { _globalTags = value; } } #if !NO_STG /// |summary| /// language /// |/summary| public string Language { get { return _language; } set { _language = value; } } /// |summary| /// namespace /// |/summary| public string Namespace { get { return _namespace; } set { _namespace = value; } } /// |summary| /// CodeBehind /// |/summary| public Collection CodeBehind { set { throw new InvalidOperationException (); } get { return _codebehind; } } /// |summary| /// Add #line statements to the inline scripts if set /// |/summary| public bool Debug { get { return _fDebug; } set { _fDebug = value; } } /// |summary| /// Scripts /// |/summary| public string Script { set { Helpers.ThrowIfEmptyOrNull (value, "value"); _script = value; } get { return _script; } } /// |summary| /// ImportNameSpaces /// |/summary| public Collection ImportNamespaces { set { throw new InvalidOperationException (); } get { return _usings; } } /// |summary| /// ImportNameSpaces /// |/summary| public Collection AssemblyReferences { set { throw new InvalidOperationException (); } get { return _assemblyReferences; } } #endif #endregion //******************************************************************* // // Internal Properties // //******************************************************************** #region Internal Properties /// |summary| /// A collection of _rules that this grammar houses. /// |/summary| internal SrgsRulesCollection Rules { get { return _rules; } } /// |summary| /// A collection of _rules that this grammar houses. /// |/summary| internal bool HasPronunciation { set { _hasPronunciation = value; } get { return _hasPronunciation; } } /// |summary| /// A collection of _rules that this grammar houses. /// |/summary| internal bool HasPhoneticAlphabetBeenSet { set { _hasPhoneticAlphabetBeenSet = value; } } /// |summary| /// A collection of _rules that this grammar houses. /// |/summary| internal bool HasSapiExtension { set { _isSapiExtensionUsed = value; } get { return _isSapiExtensionUsed; } } #endregion //******************************************************************* // // Private Methods // //******************************************************************* #region Private Methods #if !NO_STG /// /// Write the attributes of the grammar element for strongly typed grammars /// /// private void WriteSTGAttributes (XmlWriter writer) { // Write the 'language' attribute if (_language != null) { writer.WriteAttributeString ("sapi", "language", XmlParser.sapiNamespace, _language); } // Write the 'namespace' attribute if (_namespace != null) { writer.WriteAttributeString ("sapi", "namespace", XmlParser.sapiNamespace, _namespace); } // Write the 'codebehind' attribute foreach (string sFile in _codebehind) { if (!string.IsNullOrEmpty (sFile)) { writer.WriteAttributeString ("sapi", "codebehind", XmlParser.sapiNamespace, sFile); } } // Write the 'debug' attribute if (_fDebug) { writer.WriteAttributeString ("sapi", "debug", XmlParser.sapiNamespace, "True"); } } #endif ////// Write the the references to the referenced assemblies and the various scripts /// /// private void WriteGrammarElements (XmlWriter writer) { #if !NO_STG // Writeall theentries foreach (string sAssembly in _assemblyReferences) { writer.WriteStartElement ("sapi", "assemblyReference", XmlParser.sapiNamespace); writer.WriteAttributeString ("sapi", "assembly", XmlParser.sapiNamespace, sAssembly); writer.WriteEndElement (); } // Writeall the entries foreach (string sNamespace in _usings) { if (!string.IsNullOrEmpty (sNamespace)) { writer.WriteStartElement ("sapi", "importNamespace", XmlParser.sapiNamespace); writer.WriteAttributeString ("sapi", "namespace", XmlParser.sapiNamespace, sNamespace); writer.WriteEndElement (); } } #endif // Then write the rules WriteRules (writer); #if !NO_STG // At the very bottom write the scripts shared by all the rules WriteGlobalScripts (writer); #endif } /// /// Write all Rules. /// /// private void WriteRules (XmlWriter writer) { // Writebody and footer. foreach (SrgsRule rule in _rules) { rule.WriteSrgs (writer); } } #if !NO_STG /// /// Write the script that are global to this grammar /// /// private void WriteGlobalScripts (XmlWriter writer) { if (_script.Length > 0) { writer.WriteStartElement ("sapi", "script", XmlParser.sapiNamespace); writer.WriteCData (_script); writer.WriteEndElement (); } } #endif #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private bool _isSapiExtensionUsed; // Set in *.Validate() private Uri _xmlBase; private CultureInfo _culture = CultureInfo.CurrentUICulture; private SrgsGrammarMode _mode = SrgsGrammarMode.Voice; private SrgsPhoneticAlphabet _phoneticAlphabet = SrgsPhoneticAlphabet.Ipa; private bool _hasPhoneticAlphabetBeenSet; private bool _hasPronunciation; private SrgsRule _root; private SrgsTagFormat _tagFormat = SrgsTagFormat.Default; private Collection_globalTags = new Collection (); private bool _isModeSet; private SrgsRulesCollection _rules; private string _sRoot; #if !NO_STG internal bool _fContainsCode; // Set in *.Validate() // .Net Language for this grammar private string _language; // .Net Language for this grammar private Collection _codebehind = new Collection (); // namespace for the code behind private string _namespace; // Insert #line statements in the sources code if set internal bool _fDebug; // .Net language script private string _script = string.Empty; // .Net language script private List _scriptsForwardReference = new List (); // .Net Namespaces to import private Collection _usings = new Collection (); // .Net Namespaces to import private Collection _assemblyReferences = new Collection (); #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Globalization; using System.Speech.Internal; using System.Speech.Internal.SrgsCompiler; using System.Speech.Internal.SrgsParser; using System.Xml; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. #pragma warning disable 56500 // Remove all the catch all statements warnings used by the interop layer namespace System.Speech.Recognition.SrgsGrammar { /// |summary| /// Summary description for Grammar. /// |/summary| [Serializable] internal sealed class SrgsGrammar : IGrammar { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// Initializes a new instance of the Grammar class. /// internal SrgsGrammar () { _rules = new SrgsRulesCollection (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods /// |summary| /// Write the XML fragment describing the object. /// |/summary| /// |param name="writer"|XmlWriter to which to write the XML fragment.|/param| internal void WriteSrgs (XmlWriter writer) { // Writewriter.WriteStartElement ("grammar", XmlParser.srgsNamespace); writer.WriteAttributeString ("xml", "lang", null, _culture.ToString ()); if (_root != null) { writer.WriteAttributeString ("root", _root.Id); } #if !NO_STG // Write the attributes for strongly typed grammars WriteSTGAttributes (writer); #endif if (_isModeSet) { switch (_mode) { case SrgsGrammarMode.Voice: writer.WriteAttributeString ("mode", "voice"); break; case SrgsGrammarMode.Dtmf: writer.WriteAttributeString ("mode", "dtmf"); break; } } // Write the tag format if any string tagFormat = null; switch (_tagFormat) { case SrgsTagFormat.Default: // Nothing to do break; case SrgsTagFormat.MssV1: tagFormat = "semantics-ms/1.0"; break; case SrgsTagFormat.W3cV1: tagFormat = "semantics/1.0"; break; case SrgsTagFormat.KeyValuePairs: tagFormat = "properties-ms/1.0"; break; default: System.Diagnostics.Debug.Assert (false, "Unknown Tag Format!!!"); break; } if (tagFormat != null) { writer.WriteAttributeString ("tag-format", tagFormat); } // Write the Alphabet type if not SAPI if (_hasPhoneticAlphabetBeenSet || (_phoneticAlphabet != SrgsPhoneticAlphabet.Sapi && HasPronunciation)) { string alphabet = _phoneticAlphabet == SrgsPhoneticAlphabet.Ipa ? "ipa" : _phoneticAlphabet == SrgsPhoneticAlphabet.Ups ? "x-microsoft-ups" : "x-microsoft-sapi"; writer.WriteAttributeString ("sapi", "alphabet", XmlParser.sapiNamespace, alphabet); } if (_xmlBase != null) { writer.WriteAttributeString ("xml:base", _xmlBase.ToString ()); } writer.WriteAttributeString ("version", "1.0"); writer.WriteAttributeString ("xmlns", XmlParser.srgsNamespace); if (_isSapiExtensionUsed) { writer.WriteAttributeString ("xmlns", "sapi", null, XmlParser.sapiNamespace); } foreach (SrgsRule rule in _rules) { // Validate child _rules rule.Validate (this); } // Write the tag elements if any foreach (string tag in _globalTags) { writer.WriteElementString ("tag", tag); } //Write the the references to the referenced assemblies and the various scripts WriteGrammarElements (writer); writer.WriteEndElement (); } /// |summary| /// Validate the SRGS element. /// |/summary| internal void Validate () { // Validation set the pronunciation so reset it to zero HasPronunciation = HasSapiExtension = false; // validate all the rules foreach (SrgsRule rule in _rules) { // Validate child _rules rule.Validate (this); } // Initial values for ContainsCOde and SapiExtensionUsed. _isSapiExtensionUsed |= HasPronunciation; #if !NO_STG _fContainsCode |= _language != null || _script.Length > 0 || _usings.Count > 0 || _assemblyReferences.Count > 0 || _codebehind.Count > 0 || _namespace != null || _fDebug; _isSapiExtensionUsed |= _fContainsCode; #endif // If the grammar contains no pronunciations, set the phonetic alphabet to SAPI. // This way, the CFG data can be loaded by SAPI 5.1. if (!HasPronunciation) { PhoneticAlphabet = AlphabetType.Sapi; } // Validate root rule reference if (_root != null) { if (!_rules.Contains (_root)) { XmlParser.ThrowSrgsException (SRID.RootNotDefined, _root.Id); } } if (_globalTags.Count > 0) { _tagFormat = SrgsTagFormat.W3cV1; } #if !NO_STG // Force the tag format to Sapi fprperties if .Net semantics are used. if (_fContainsCode) { if (_tagFormat == SrgsTagFormat.Default) { _tagFormat = SrgsTagFormat.KeyValuePairs; } // SAPI semantics only for .Net Semantics if (_tagFormat != SrgsTagFormat.KeyValuePairs) { XmlParser.ThrowSrgsException (SRID.InvalidSemanticProcessingType); } } #endif } IRule IGrammar.CreateRule (string id, RulePublic publicRule, RuleDynamic dynamic, bool hasScript) { SrgsRule rule = new SrgsRule (id); if (publicRule != RulePublic.NotSet) { rule.Scope = publicRule == RulePublic.True ? SrgsRuleScope.Public : SrgsRuleScope.Private; } rule.Dynamic = dynamic; return (IRule) rule; } void IElement.PostParse (IElement parent) { // Check that the root rule is defined if (_sRoot != null) { bool found = false; foreach (SrgsRule rule in Rules) { if (rule.Id == _sRoot) { Root = rule; found = true; break; } } if (!found) { // "Root rule ""%s"" is undefined." XmlParser.ThrowSrgsException (SRID.RootNotDefined, _sRoot); } } #if !NO_STG // Resolve the references to the scripts foreach (XmlParser.ForwardReference script in _scriptsForwardReference) { SrgsRule rule = Rules [script._name]; if (rule != null) { rule.Script = rule.Script + script._value; } else { XmlParser.ThrowSrgsException (SRID.InvalidScriptDefinition); } } #endif // Validate the whole grammar Validate (); } #if !NO_STG #pragma warning disable 56507 // check for null or empty strings // Add a script to this grammar or to a rule internal void AddScript (string rule, string code) { if (rule == null) { _script += code; } else { _scriptsForwardReference.Add (new XmlParser.ForwardReference (rule, code)); } } #endif #pragma warning restore 56507 #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties /// /// Sets the Root element /// ///string IGrammar.Root { set { _sRoot = value; } get { return _sRoot; } } /// |summary| /// Base URI of grammar (xml:base) /// |/summary| /// |remarks| /// public Uri XmlBase { get { return _xmlBase; } set { _xmlBase = value; } } /// |summary| /// Grammar language (xml:lang) /// |/summary| public CultureInfo Culture { get { return _culture; } set { Helpers.ThrowIfNull (value, "value"); _culture = value; } } /// |summary| /// Grammar mode. voice or dtmf /// |/summary| public GrammarType Mode { get { return _mode == SrgsGrammarMode.Voice ? GrammarType.VoiceGrammar : GrammarType.DtmfGrammar; } set { _mode = value == GrammarType.VoiceGrammar ? SrgsGrammarMode.Voice : SrgsGrammarMode.Dtmf; _isModeSet = true; } } /// |summary| /// Pronunciation Alphabet, IPA or SAPI or UPS /// |/summary| public AlphabetType PhoneticAlphabet { get { return (AlphabetType) _phoneticAlphabet; } set { _phoneticAlphabet = (SrgsPhoneticAlphabet) value; } } /// |summary|root /// Root rule (srgs:root) /// |/summary| public SrgsRule Root { get { return _root; } set { _root = value; } } /// |summary| /// Tag format (srgs:tag-format) /// |/summary| public SrgsTagFormat TagFormat { get { return _tagFormat; } set { _tagFormat = value; } } /// |summary| /// Tag format (srgs:tag-format) /// |/summary| public Collection GlobalTags { get { return _globalTags; } set { _globalTags = value; } } #if !NO_STG /// |summary| /// language /// |/summary| public string Language { get { return _language; } set { _language = value; } } /// |summary| /// namespace /// |/summary| public string Namespace { get { return _namespace; } set { _namespace = value; } } /// |summary| /// CodeBehind /// |/summary| public Collection CodeBehind { set { throw new InvalidOperationException (); } get { return _codebehind; } } /// |summary| /// Add #line statements to the inline scripts if set /// |/summary| public bool Debug { get { return _fDebug; } set { _fDebug = value; } } /// |summary| /// Scripts /// |/summary| public string Script { set { Helpers.ThrowIfEmptyOrNull (value, "value"); _script = value; } get { return _script; } } /// |summary| /// ImportNameSpaces /// |/summary| public Collection ImportNamespaces { set { throw new InvalidOperationException (); } get { return _usings; } } /// |summary| /// ImportNameSpaces /// |/summary| public Collection AssemblyReferences { set { throw new InvalidOperationException (); } get { return _assemblyReferences; } } #endif #endregion //******************************************************************* // // Internal Properties // //******************************************************************** #region Internal Properties /// |summary| /// A collection of _rules that this grammar houses. /// |/summary| internal SrgsRulesCollection Rules { get { return _rules; } } /// |summary| /// A collection of _rules that this grammar houses. /// |/summary| internal bool HasPronunciation { set { _hasPronunciation = value; } get { return _hasPronunciation; } } /// |summary| /// A collection of _rules that this grammar houses. /// |/summary| internal bool HasPhoneticAlphabetBeenSet { set { _hasPhoneticAlphabetBeenSet = value; } } /// |summary| /// A collection of _rules that this grammar houses. /// |/summary| internal bool HasSapiExtension { set { _isSapiExtensionUsed = value; } get { return _isSapiExtensionUsed; } } #endregion //******************************************************************* // // Private Methods // //******************************************************************* #region Private Methods #if !NO_STG /// /// Write the attributes of the grammar element for strongly typed grammars /// /// private void WriteSTGAttributes (XmlWriter writer) { // Write the 'language' attribute if (_language != null) { writer.WriteAttributeString ("sapi", "language", XmlParser.sapiNamespace, _language); } // Write the 'namespace' attribute if (_namespace != null) { writer.WriteAttributeString ("sapi", "namespace", XmlParser.sapiNamespace, _namespace); } // Write the 'codebehind' attribute foreach (string sFile in _codebehind) { if (!string.IsNullOrEmpty (sFile)) { writer.WriteAttributeString ("sapi", "codebehind", XmlParser.sapiNamespace, sFile); } } // Write the 'debug' attribute if (_fDebug) { writer.WriteAttributeString ("sapi", "debug", XmlParser.sapiNamespace, "True"); } } #endif ////// Write the the references to the referenced assemblies and the various scripts /// /// private void WriteGrammarElements (XmlWriter writer) { #if !NO_STG // Writeall theentries foreach (string sAssembly in _assemblyReferences) { writer.WriteStartElement ("sapi", "assemblyReference", XmlParser.sapiNamespace); writer.WriteAttributeString ("sapi", "assembly", XmlParser.sapiNamespace, sAssembly); writer.WriteEndElement (); } // Writeall the entries foreach (string sNamespace in _usings) { if (!string.IsNullOrEmpty (sNamespace)) { writer.WriteStartElement ("sapi", "importNamespace", XmlParser.sapiNamespace); writer.WriteAttributeString ("sapi", "namespace", XmlParser.sapiNamespace, sNamespace); writer.WriteEndElement (); } } #endif // Then write the rules WriteRules (writer); #if !NO_STG // At the very bottom write the scripts shared by all the rules WriteGlobalScripts (writer); #endif } /// /// Write all Rules. /// /// private void WriteRules (XmlWriter writer) { // Writebody and footer. foreach (SrgsRule rule in _rules) { rule.WriteSrgs (writer); } } #if !NO_STG /// /// Write the script that are global to this grammar /// /// private void WriteGlobalScripts (XmlWriter writer) { if (_script.Length > 0) { writer.WriteStartElement ("sapi", "script", XmlParser.sapiNamespace); writer.WriteCData (_script); writer.WriteEndElement (); } } #endif #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private bool _isSapiExtensionUsed; // Set in *.Validate() private Uri _xmlBase; private CultureInfo _culture = CultureInfo.CurrentUICulture; private SrgsGrammarMode _mode = SrgsGrammarMode.Voice; private SrgsPhoneticAlphabet _phoneticAlphabet = SrgsPhoneticAlphabet.Ipa; private bool _hasPhoneticAlphabetBeenSet; private bool _hasPronunciation; private SrgsRule _root; private SrgsTagFormat _tagFormat = SrgsTagFormat.Default; private Collection_globalTags = new Collection (); private bool _isModeSet; private SrgsRulesCollection _rules; private string _sRoot; #if !NO_STG internal bool _fContainsCode; // Set in *.Validate() // .Net Language for this grammar private string _language; // .Net Language for this grammar private Collection _codebehind = new Collection (); // namespace for the code behind private string _namespace; // Insert #line statements in the sources code if set internal bool _fDebug; // .Net language script private string _script = string.Empty; // .Net language script private List _scriptsForwardReference = new List (); // .Net Namespaces to import private Collection _usings = new Collection (); // .Net Namespaces to import private Collection _assemblyReferences = new Collection (); #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
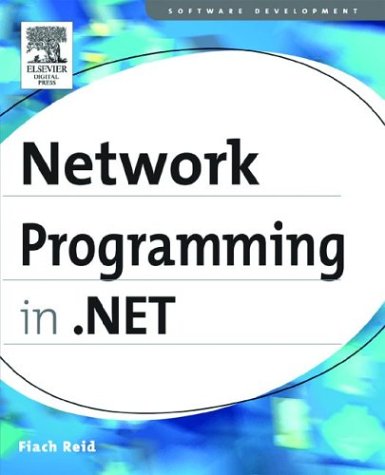
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewUpdatedEventArgs.cs
- xml.cs
- SecurityRuntime.cs
- ExpandSegment.cs
- XsltSettings.cs
- Base64Stream.cs
- DictionaryBase.cs
- CapabilitiesUse.cs
- WebServiceHandler.cs
- Track.cs
- PnrpPeerResolver.cs
- SevenBitStream.cs
- ProfilePropertySettings.cs
- RoutedEventHandlerInfo.cs
- TextRangeAdaptor.cs
- BufferModeSettings.cs
- XmlSchemaExternal.cs
- DesignerDataTableBase.cs
- FieldReference.cs
- DesignerActionHeaderItem.cs
- DynamicILGenerator.cs
- DSASignatureDeformatter.cs
- FieldToken.cs
- DbParameterCollectionHelper.cs
- Style.cs
- TypedElement.cs
- HtmlElementErrorEventArgs.cs
- AdapterUtil.cs
- SHA384.cs
- CacheHelper.cs
- DayRenderEvent.cs
- UpdateException.cs
- InstanceDataCollectionCollection.cs
- ClrPerspective.cs
- AnnotationComponentChooser.cs
- ResourceContainer.cs
- SmiEventSink_DeferedProcessing.cs
- RegexWriter.cs
- StringConverter.cs
- ReliableChannelListener.cs
- UriTemplateQueryValue.cs
- MaskInputRejectedEventArgs.cs
- StylusButton.cs
- BamlBinaryWriter.cs
- FlatButtonAppearance.cs
- OdbcHandle.cs
- WebServiceBindingAttribute.cs
- DesignOnlyAttribute.cs
- ListenerChannelContext.cs
- XmlChildNodes.cs
- PKCS1MaskGenerationMethod.cs
- TcpChannelHelper.cs
- SafeNativeMethods.cs
- DtrList.cs
- StyleHelper.cs
- InternalTransaction.cs
- SecurityTokenAuthenticator.cs
- UInt64Converter.cs
- StrongNamePublicKeyBlob.cs
- OutputCacheSection.cs
- COAUTHIDENTITY.cs
- XmlEnumAttribute.cs
- PersonalizableAttribute.cs
- AppDomainManager.cs
- BamlTreeNode.cs
- XamlStyleSerializer.cs
- UnsafeNativeMethodsTablet.cs
- SqlException.cs
- TransactionException.cs
- CryptoConfig.cs
- PlainXmlWriter.cs
- XPathScanner.cs
- HttpRuntimeSection.cs
- FixedLineResult.cs
- Select.cs
- _ListenerAsyncResult.cs
- HttpListenerRequest.cs
- SectionXmlInfo.cs
- SpecularMaterial.cs
- WebRequest.cs
- TypeDescriptionProvider.cs
- Point3DAnimationBase.cs
- UInt16.cs
- _HTTPDateParse.cs
- String.cs
- RecognizerInfo.cs
- SQLDateTime.cs
- codemethodreferenceexpression.cs
- ExpressionStringBuilder.cs
- ObjectSecurityT.cs
- GrabHandleGlyph.cs
- RewritingPass.cs
- TextCompositionManager.cs
- ServerValidateEventArgs.cs
- SecurityTokenReferenceStyle.cs
- DbCommandTree.cs
- ToolStripLabel.cs
- NgenServicingAttributes.cs
- XmlDictionaryString.cs
- InstanceKey.cs