Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Server / System / Data / Services / ExpandSegment.cs / 2 / ExpandSegment.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a description of a segment in an $expand query option // for an ADO.NET Data Service. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Collections.Generic; using System.Linq.Expressions; using System.Data.Services.Providers; using System.Diagnostics; #endregion Namespaces. ////// Provides a description of a segment in an $expand query option for an ADO.NET Data Service. /// ////// INTERNAL /// Expand providers may replace segments to indicate a different expansion shape. However, they are /// unable to set the MaxResultsExpected. The value for the instances created by external providers /// will always be Int32.MaxValue, but the value enforced by the serializers will be the one declared /// by the data service in the configuration. /// /// When the configuration supports a more granular value, we should overload the constructor to make /// the MaxResultsExpected property settable as well. /// [DebuggerDisplay("ExpandSegment ({name},Filter={filter})]")] public class ExpandSegment { #region Private fields. ///Container to which the segment belongs; possibly null. private readonly ResourceContainer container; ///Filter expression for this segment on an $expand path. private readonly Expression filter; ///Name for this segment on an $expand path. private readonly string name; ////// The maximum number of results expected for this property; Int32.MaxValue if no limit is expected. /// private readonly int maxResultsExpected; #endregion Private fields. #region Constructors. ///Initializes a new /// Segment name. /// Filter expression for segment, possibly null. public ExpandSegment(string name, Expression filter) : this(name, filter, Int32.MaxValue, null) { } ///instance. Initializes a new /// Segment name. /// Filter expression for segment, possibly null. /// /// Expand providers may choose to return at most MaxResultsExpected + 1 elements to allow the /// data service to detect a failure to meet this constraint. /// /// Container to which the segment belongs; possibly null. internal ExpandSegment(string name, Expression filter, int maxResultsExpected, ResourceContainer container) { WebUtil.CheckArgumentNull(name, "name"); CheckFilterType(filter); this.name = name; this.filter = filter; this.container = container; this.maxResultsExpected = maxResultsExpected; } #endregion Constructors. #region Public properties. ///instance. Filter expression for this segment on an $expand path. public Expression Filter { get { return this.filter; } } ///Whether this segment has a filter. public bool HasFilter { get { return this.Filter != null; } } ////// The maximum number of results expected for this property; Int32.MaxValue if no limit is expected. /// ////// Expand providers may choose to return at most MaxResultsExpected + 1 elements to allow the /// data service to detect a failure to meet this constraint. /// public int MaxResultsExpected { get { return this.maxResultsExpected; } } ///Name for this segment on an $expand path. public string Name { get { return this.name; } } #endregion Public properties. #region Internal properties. ///Gets the container to which this segment belongs; possibly null. internal ResourceContainer Container { get { return this.container; } } ///Whether this segment has a filter or a constraint on max results returns. internal bool HasFilterOrMaxResults { get { return this.Filter != null || this.MaxResultsExpected != Int32.MaxValue; } } #endregion Internal properties. #region Public methods. ///Checks whether any segments in the specified /// Path with segments to check. ///have a filter. true if any of the segments in public static bool PathHasFilter(IEnumerablehas a filter; false otherwise. path) { WebUtil.CheckArgumentNull(path, "path"); foreach (ExpandSegment segment in path) { if (segment.HasFilter) { return true; } } return false; } #endregion Public methods. #region Private methods. /// Checks that the specified filter is of the right type. /// Filter to check. private static void CheckFilterType(Expression filter) { if (filter == null) { return; } if (filter.NodeType != ExpressionType.Lambda) { throw new ArgumentException(Strings.ExpandSegment_FilterShouldBeLambda(filter.NodeType), "filter"); } LambdaExpression lambda = (LambdaExpression)filter; if (lambda.Body.Type != typeof(bool) && lambda.Body.Type != typeof(bool?)) { throw new ArgumentException( Strings.ExpandSegment_FilterBodyShouldReturnBool(lambda.Body.Type), "filter"); } if (lambda.Parameters.Count != 1) { throw new ArgumentException( Strings.ExpandSegment_FilterBodyShouldTakeOneParameter(lambda.Parameters.Count), "filter"); } } #endregion Private methods. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a description of a segment in an $expand query option // for an ADO.NET Data Service. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Collections.Generic; using System.Linq.Expressions; using System.Data.Services.Providers; using System.Diagnostics; #endregion Namespaces. ////// Provides a description of a segment in an $expand query option for an ADO.NET Data Service. /// ////// INTERNAL /// Expand providers may replace segments to indicate a different expansion shape. However, they are /// unable to set the MaxResultsExpected. The value for the instances created by external providers /// will always be Int32.MaxValue, but the value enforced by the serializers will be the one declared /// by the data service in the configuration. /// /// When the configuration supports a more granular value, we should overload the constructor to make /// the MaxResultsExpected property settable as well. /// [DebuggerDisplay("ExpandSegment ({name},Filter={filter})]")] public class ExpandSegment { #region Private fields. ///Container to which the segment belongs; possibly null. private readonly ResourceContainer container; ///Filter expression for this segment on an $expand path. private readonly Expression filter; ///Name for this segment on an $expand path. private readonly string name; ////// The maximum number of results expected for this property; Int32.MaxValue if no limit is expected. /// private readonly int maxResultsExpected; #endregion Private fields. #region Constructors. ///Initializes a new /// Segment name. /// Filter expression for segment, possibly null. public ExpandSegment(string name, Expression filter) : this(name, filter, Int32.MaxValue, null) { } ///instance. Initializes a new /// Segment name. /// Filter expression for segment, possibly null. /// /// Expand providers may choose to return at most MaxResultsExpected + 1 elements to allow the /// data service to detect a failure to meet this constraint. /// /// Container to which the segment belongs; possibly null. internal ExpandSegment(string name, Expression filter, int maxResultsExpected, ResourceContainer container) { WebUtil.CheckArgumentNull(name, "name"); CheckFilterType(filter); this.name = name; this.filter = filter; this.container = container; this.maxResultsExpected = maxResultsExpected; } #endregion Constructors. #region Public properties. ///instance. Filter expression for this segment on an $expand path. public Expression Filter { get { return this.filter; } } ///Whether this segment has a filter. public bool HasFilter { get { return this.Filter != null; } } ////// The maximum number of results expected for this property; Int32.MaxValue if no limit is expected. /// ////// Expand providers may choose to return at most MaxResultsExpected + 1 elements to allow the /// data service to detect a failure to meet this constraint. /// public int MaxResultsExpected { get { return this.maxResultsExpected; } } ///Name for this segment on an $expand path. public string Name { get { return this.name; } } #endregion Public properties. #region Internal properties. ///Gets the container to which this segment belongs; possibly null. internal ResourceContainer Container { get { return this.container; } } ///Whether this segment has a filter or a constraint on max results returns. internal bool HasFilterOrMaxResults { get { return this.Filter != null || this.MaxResultsExpected != Int32.MaxValue; } } #endregion Internal properties. #region Public methods. ///Checks whether any segments in the specified /// Path with segments to check. ///have a filter. true if any of the segments in public static bool PathHasFilter(IEnumerablehas a filter; false otherwise. path) { WebUtil.CheckArgumentNull(path, "path"); foreach (ExpandSegment segment in path) { if (segment.HasFilter) { return true; } } return false; } #endregion Public methods. #region Private methods. /// Checks that the specified filter is of the right type. /// Filter to check. private static void CheckFilterType(Expression filter) { if (filter == null) { return; } if (filter.NodeType != ExpressionType.Lambda) { throw new ArgumentException(Strings.ExpandSegment_FilterShouldBeLambda(filter.NodeType), "filter"); } LambdaExpression lambda = (LambdaExpression)filter; if (lambda.Body.Type != typeof(bool) && lambda.Body.Type != typeof(bool?)) { throw new ArgumentException( Strings.ExpandSegment_FilterBodyShouldReturnBool(lambda.Body.Type), "filter"); } if (lambda.Parameters.Count != 1) { throw new ArgumentException( Strings.ExpandSegment_FilterBodyShouldTakeOneParameter(lambda.Parameters.Count), "filter"); } } #endregion Private methods. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
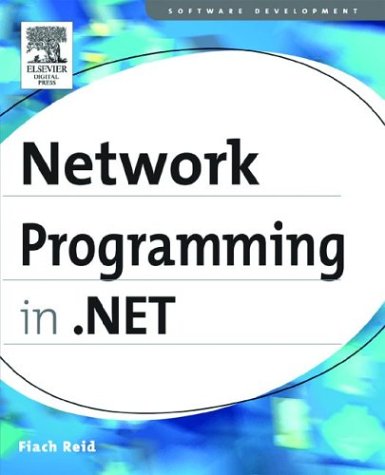
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProtocolsConfigurationEntry.cs
- GeneralTransform.cs
- Match.cs
- ServiceOperationParameter.cs
- SettingsPropertyIsReadOnlyException.cs
- AtomicFile.cs
- FormatterServices.cs
- TextOptionsInternal.cs
- ScopeCompiler.cs
- SchemaConstraints.cs
- DynamicValueConverter.cs
- TypeConverter.cs
- XpsS0ValidatingLoader.cs
- WindowsImpersonationContext.cs
- DataGridLengthConverter.cs
- AutomationPatternInfo.cs
- Config.cs
- InstrumentationTracker.cs
- ImageClickEventArgs.cs
- CodeNamespace.cs
- PageContent.cs
- DateTimeFormatInfoScanner.cs
- TdsParserSafeHandles.cs
- Int32Converter.cs
- WindowPattern.cs
- MailFileEditor.cs
- ImmutableCommunicationTimeouts.cs
- XPathParser.cs
- RenderContext.cs
- PrintControllerWithStatusDialog.cs
- WSSecurityJan2004.cs
- ReferencedAssembly.cs
- DataListGeneralPage.cs
- BuildProvider.cs
- XamlClipboardData.cs
- ReadOnlyTernaryTree.cs
- ReadOnlyDataSource.cs
- Semaphore.cs
- PersonalizationStateQuery.cs
- PermissionToken.cs
- Point3DIndependentAnimationStorage.cs
- Formatter.cs
- GenerateHelper.cs
- DeviceContexts.cs
- ReliableSessionBindingElement.cs
- FaultReason.cs
- WebPartCollection.cs
- FontDriver.cs
- SynchronizedKeyedCollection.cs
- WebPartsPersonalization.cs
- SelectedDatesCollection.cs
- OdbcCommandBuilder.cs
- Underline.cs
- InternalResources.cs
- ACL.cs
- LongPath.cs
- SecureEnvironment.cs
- StylusDevice.cs
- WindowsScrollBar.cs
- AndCondition.cs
- LogRestartAreaEnumerator.cs
- GeometryDrawing.cs
- HttpApplication.cs
- ExpressionContext.cs
- TextCharacters.cs
- DataColumnMapping.cs
- ZipArchive.cs
- __TransparentProxy.cs
- SamlAssertion.cs
- MessageQuerySet.cs
- FloaterParagraph.cs
- SafeRightsManagementSessionHandle.cs
- AutomationFocusChangedEventArgs.cs
- ReadOnlyCollectionBase.cs
- DataViewListener.cs
- Stylesheet.cs
- DefaultValueTypeConverter.cs
- documentsequencetextview.cs
- GregorianCalendarHelper.cs
- BitmapDecoder.cs
- SystemIcmpV4Statistics.cs
- DockAndAnchorLayout.cs
- TypeDescriptionProvider.cs
- XmlMembersMapping.cs
- Matrix.cs
- ObjectDataSourceStatusEventArgs.cs
- DataSourceProvider.cs
- WebConfigurationHostFileChange.cs
- userdatakeys.cs
- MenuAutomationPeer.cs
- MetadataCache.cs
- MultiSelectRootGridEntry.cs
- DictionaryChange.cs
- BufferAllocator.cs
- AttributeUsageAttribute.cs
- ReflectionUtil.cs
- ReferenceConverter.cs
- ISCIIEncoding.cs
- HtmlEncodedRawTextWriter.cs
- ReliabilityContractAttribute.cs