Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / UseAttributeSetsAction.cs / 1 / UseAttributeSetsAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; using System.Collections; internal class UseAttributeSetsAction : CompiledAction { private XmlQualifiedName[] useAttributeSets; private string useString; private const int ProcessingSets = 2; internal XmlQualifiedName[] UsedSets { get { return this.useAttributeSets; } } internal override void Compile(Compiler compiler) { Debug.Assert(Keywords.Equals(compiler.Input.LocalName, compiler.Atoms.UseAttributeSets)); this.useString = compiler.Input.Value; Debug.Assert(this.useAttributeSets == null); if (this.useString.Length == 0) { // Split creates empty node is spliting empty string this.useAttributeSets = new XmlQualifiedName[0]; return; } string[] qnames = XmlConvert.SplitString(this.useString); try { this.useAttributeSets = new XmlQualifiedName[qnames.Length]; { for (int i = 0; i < qnames.Length; i++) { this.useAttributeSets[i] = compiler.CreateXPathQName(qnames[i]); } } } catch (XsltException) { if (!compiler.ForwardCompatibility) { // Rethrow the exception if we're not in forwards-compatible mode throw; } // Ignore the whole list in forwards-compatible mode this.useAttributeSets = new XmlQualifiedName[0]; } } internal override void Execute(Processor processor, ActionFrame frame) { switch(frame.State) { case Initialized: frame.Counter = 0; frame.State = ProcessingSets; goto case ProcessingSets; case ProcessingSets: if (frame.Counter < this.useAttributeSets.Length) { AttributeSetAction action = processor.RootAction.GetAttributeSet(this.useAttributeSets[frame.Counter]); frame.IncrementCounter(); processor.PushActionFrame(action, frame.NodeSet); } else { frame.Finished(); } break; default: Debug.Fail("Invalid Container action execution state"); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; using System.Collections; internal class UseAttributeSetsAction : CompiledAction { private XmlQualifiedName[] useAttributeSets; private string useString; private const int ProcessingSets = 2; internal XmlQualifiedName[] UsedSets { get { return this.useAttributeSets; } } internal override void Compile(Compiler compiler) { Debug.Assert(Keywords.Equals(compiler.Input.LocalName, compiler.Atoms.UseAttributeSets)); this.useString = compiler.Input.Value; Debug.Assert(this.useAttributeSets == null); if (this.useString.Length == 0) { // Split creates empty node is spliting empty string this.useAttributeSets = new XmlQualifiedName[0]; return; } string[] qnames = XmlConvert.SplitString(this.useString); try { this.useAttributeSets = new XmlQualifiedName[qnames.Length]; { for (int i = 0; i < qnames.Length; i++) { this.useAttributeSets[i] = compiler.CreateXPathQName(qnames[i]); } } } catch (XsltException) { if (!compiler.ForwardCompatibility) { // Rethrow the exception if we're not in forwards-compatible mode throw; } // Ignore the whole list in forwards-compatible mode this.useAttributeSets = new XmlQualifiedName[0]; } } internal override void Execute(Processor processor, ActionFrame frame) { switch(frame.State) { case Initialized: frame.Counter = 0; frame.State = ProcessingSets; goto case ProcessingSets; case ProcessingSets: if (frame.Counter < this.useAttributeSets.Length) { AttributeSetAction action = processor.RootAction.GetAttributeSet(this.useAttributeSets[frame.Counter]); frame.IncrementCounter(); processor.PushActionFrame(action, frame.NodeSet); } else { frame.Finished(); } break; default: Debug.Fail("Invalid Container action execution state"); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
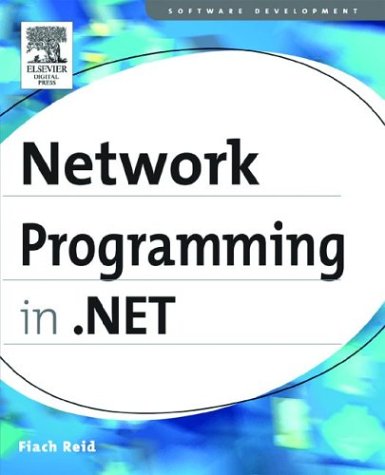
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceHost.cs
- TextSpan.cs
- ToolboxItem.cs
- WebControlsSection.cs
- EnumBuilder.cs
- ResourcePart.cs
- RegistryDataKey.cs
- ProfileGroupSettingsCollection.cs
- BufferModeSettings.cs
- GACIdentityPermission.cs
- Substitution.cs
- WebMessageFormatHelper.cs
- WindowPattern.cs
- OdbcUtils.cs
- UrlEncodedParameterWriter.cs
- RenderingEventArgs.cs
- SharedDp.cs
- IPPacketInformation.cs
- _SecureChannel.cs
- SqlTrackingService.cs
- GrammarBuilderWildcard.cs
- StrokeNodeEnumerator.cs
- VisualStates.cs
- CatchBlock.cs
- BadImageFormatException.cs
- FileSystemWatcher.cs
- OracleSqlParser.cs
- LicenseException.cs
- NodeFunctions.cs
- RequestQueue.cs
- DataGridViewRow.cs
- X509CertificateInitiatorServiceCredential.cs
- OrderedDictionary.cs
- ExceptionRoutedEventArgs.cs
- FileChangesMonitor.cs
- XmlReturnReader.cs
- MatrixCamera.cs
- SubstitutionList.cs
- PeerTransportListenAddressValidatorAttribute.cs
- TableLayoutPanelResizeGlyph.cs
- Attributes.cs
- DeflateStream.cs
- DelayedRegex.cs
- Variable.cs
- DashStyles.cs
- PasswordTextNavigator.cs
- NumericPagerField.cs
- ToggleButton.cs
- EllipseGeometry.cs
- CodeComment.cs
- TrackingSection.cs
- ArgumentValidation.cs
- ContainerParagraph.cs
- ThicknessAnimationUsingKeyFrames.cs
- QueryException.cs
- VirtualDirectoryMappingCollection.cs
- ListBase.cs
- precedingsibling.cs
- DataGridViewSelectedCellCollection.cs
- ZipArchive.cs
- RuntimeConfigurationRecord.cs
- SelectionWordBreaker.cs
- StrokeRenderer.cs
- ErrorRuntimeConfig.cs
- StringCollectionMarkupSerializer.cs
- XmlDownloadManager.cs
- _TransmitFileOverlappedAsyncResult.cs
- BasicHttpMessageCredentialType.cs
- KnownAssemblyEntry.cs
- FileDialogCustomPlace.cs
- DataGridItemEventArgs.cs
- CopyAttributesAction.cs
- DBAsyncResult.cs
- StringFreezingAttribute.cs
- XPathQueryGenerator.cs
- BitmapEffect.cs
- QuaternionRotation3D.cs
- TextRange.cs
- TreeViewDesigner.cs
- _StreamFramer.cs
- DbParameterCollectionHelper.cs
- UDPClient.cs
- Stroke.cs
- XmlTextWriter.cs
- PropertyDescriptor.cs
- InputScope.cs
- _ChunkParse.cs
- MailAddress.cs
- Vector.cs
- RegistryDataKey.cs
- XmlWellformedWriterHelpers.cs
- DocumentXPathNavigator.cs
- DelegatingStream.cs
- DataTrigger.cs
- TextRangeProviderWrapper.cs
- OdbcConnectionOpen.cs
- SemaphoreSlim.cs
- QueryServiceConfigHandle.cs
- MethodBuilderInstantiation.cs
- Scene3D.cs