Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / OleDb / OleDbError.cs / 1 / OleDbError.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.OleDb { using System; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; [Serializable] #if WINFSInternalOnly internal #else public #endif sealed class OleDbError { readonly private string message; readonly private string source; readonly private string sqlState; readonly private int nativeError; internal OleDbError(UnsafeNativeMethods.IErrorRecords errorRecords, int index) { OleDbHResult hr; int lcid = System.Globalization.CultureInfo.CurrentCulture.LCID; Bid.Trace("\n"); UnsafeNativeMethods.IErrorInfo errorInfo = errorRecords.GetErrorInfo(index, lcid); if (null != errorInfo) { Bid.Trace(" \n"); hr = errorInfo.GetDescription(out this.message); Bid.Trace(" Message='%ls'\n", this.message); if (OleDbHResult.DB_E_NOLOCALE == hr) { // MDAC 87303 Bid.Trace(" ErrorInfo\n"); Marshal.ReleaseComObject(errorInfo); Bid.Trace(" \n"); lcid = SafeNativeMethods.GetUserDefaultLCID(); Bid.Trace(" LCID=%d\n", lcid); errorInfo = errorRecords.GetErrorInfo(index, lcid); if (null != errorInfo) { Bid.Trace(" \n"); hr = errorInfo.GetDescription(out this.message); Bid.Trace(" Message='%ls'\n", this.message); } } if ((hr < 0) && ADP.IsEmpty(this.message)) { this.message = ODB.FailedGetDescription(hr); } if (null != errorInfo) { Bid.Trace(" \n"); hr = errorInfo.GetSource(out this.source); Bid.Trace(" Source='%ls'\n", this.source); if (OleDbHResult.DB_E_NOLOCALE == hr) { // MDAC 87303 Marshal.ReleaseComObject(errorInfo); Bid.Trace(" \n"); lcid = SafeNativeMethods.GetUserDefaultLCID(); Bid.Trace(" LCID=%d\n", lcid); errorInfo = errorRecords.GetErrorInfo(index, lcid); if (null != errorInfo) { Bid.Trace(" \n"); hr = errorInfo.GetSource(out this.source); Bid.Trace(" Source='%ls'\n", this.source); } } if ((hr < 0) && ADP.IsEmpty(this.source)) { this.source = ODB.FailedGetSource(hr); } Bid.Trace(" ErrorInfo\n"); Marshal.ReleaseComObject(errorInfo); } } UnsafeNativeMethods.ISQLErrorInfo sqlErrorInfo; Bid.Trace(" IID_ISQLErrorInfo\n"); hr = errorRecords.GetCustomErrorObject(index, ref ODB.IID_ISQLErrorInfo, out sqlErrorInfo); if (null != sqlErrorInfo) { Bid.Trace(" \n"); this.nativeError = sqlErrorInfo.GetSQLInfo(out this.sqlState); Bid.Trace(" SQLErrorInfo\n"); Marshal.ReleaseComObject(sqlErrorInfo); } } public string Message { get { string message = this.message; return ((null != message) ? message : ADP.StrEmpty); } } public int NativeError { get { return this.nativeError; } } public string Source { get { string source = this.source; return ((null != source) ? source : ADP.StrEmpty); } } public string SQLState { get { string sqlState = this.sqlState; return ((null != sqlState) ? sqlState : ADP.StrEmpty); } } override public string ToString() { return Message; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.OleDb { using System; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; [Serializable] #if WINFSInternalOnly internal #else public #endif sealed class OleDbError { readonly private string message; readonly private string source; readonly private string sqlState; readonly private int nativeError; internal OleDbError(UnsafeNativeMethods.IErrorRecords errorRecords, int index) { OleDbHResult hr; int lcid = System.Globalization.CultureInfo.CurrentCulture.LCID; Bid.Trace("\n"); UnsafeNativeMethods.IErrorInfo errorInfo = errorRecords.GetErrorInfo(index, lcid); if (null != errorInfo) { Bid.Trace(" \n"); hr = errorInfo.GetDescription(out this.message); Bid.Trace(" Message='%ls'\n", this.message); if (OleDbHResult.DB_E_NOLOCALE == hr) { // MDAC 87303 Bid.Trace(" ErrorInfo\n"); Marshal.ReleaseComObject(errorInfo); Bid.Trace(" \n"); lcid = SafeNativeMethods.GetUserDefaultLCID(); Bid.Trace(" LCID=%d\n", lcid); errorInfo = errorRecords.GetErrorInfo(index, lcid); if (null != errorInfo) { Bid.Trace(" \n"); hr = errorInfo.GetDescription(out this.message); Bid.Trace(" Message='%ls'\n", this.message); } } if ((hr < 0) && ADP.IsEmpty(this.message)) { this.message = ODB.FailedGetDescription(hr); } if (null != errorInfo) { Bid.Trace(" \n"); hr = errorInfo.GetSource(out this.source); Bid.Trace(" Source='%ls'\n", this.source); if (OleDbHResult.DB_E_NOLOCALE == hr) { // MDAC 87303 Marshal.ReleaseComObject(errorInfo); Bid.Trace(" \n"); lcid = SafeNativeMethods.GetUserDefaultLCID(); Bid.Trace(" LCID=%d\n", lcid); errorInfo = errorRecords.GetErrorInfo(index, lcid); if (null != errorInfo) { Bid.Trace(" \n"); hr = errorInfo.GetSource(out this.source); Bid.Trace(" Source='%ls'\n", this.source); } } if ((hr < 0) && ADP.IsEmpty(this.source)) { this.source = ODB.FailedGetSource(hr); } Bid.Trace(" ErrorInfo\n"); Marshal.ReleaseComObject(errorInfo); } } UnsafeNativeMethods.ISQLErrorInfo sqlErrorInfo; Bid.Trace(" IID_ISQLErrorInfo\n"); hr = errorRecords.GetCustomErrorObject(index, ref ODB.IID_ISQLErrorInfo, out sqlErrorInfo); if (null != sqlErrorInfo) { Bid.Trace(" \n"); this.nativeError = sqlErrorInfo.GetSQLInfo(out this.sqlState); Bid.Trace(" SQLErrorInfo\n"); Marshal.ReleaseComObject(sqlErrorInfo); } } public string Message { get { string message = this.message; return ((null != message) ? message : ADP.StrEmpty); } } public int NativeError { get { return this.nativeError; } } public string Source { get { string source = this.source; return ((null != source) ? source : ADP.StrEmpty); } } public string SQLState { get { string sqlState = this.sqlState; return ((null != sqlState) ? sqlState : ADP.StrEmpty); } } override public string ToString() { return Message; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
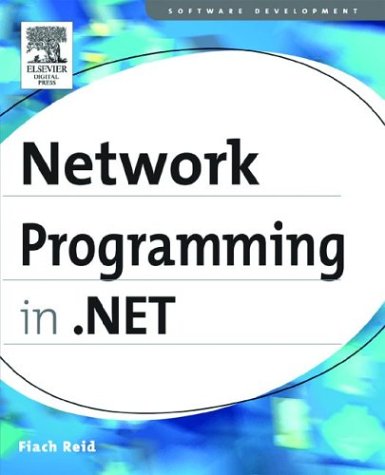
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SetIterators.cs
- Hex.cs
- DynamicField.cs
- HierarchicalDataBoundControlAdapter.cs
- IRCollection.cs
- InvalidEnumArgumentException.cs
- UriTemplateTable.cs
- DesignerForm.cs
- XPathNodeHelper.cs
- LicenseProviderAttribute.cs
- DBAsyncResult.cs
- Update.cs
- CaseInsensitiveHashCodeProvider.cs
- RightsManagementEncryptionTransform.cs
- KeyboardNavigation.cs
- ArgumentValue.cs
- SaveFileDialog.cs
- TreeNodeSelectionProcessor.cs
- InstanceData.cs
- storepermission.cs
- Control.cs
- TemplateContent.cs
- SafeNativeMethods.cs
- ProfileInfo.cs
- DeclarativeExpressionConditionDeclaration.cs
- ObfuscateAssemblyAttribute.cs
- FrameSecurityDescriptor.cs
- XmlILConstructAnalyzer.cs
- VariantWrapper.cs
- CompoundFileStreamReference.cs
- MsmqProcessProtocolHandler.cs
- MulticastDelegate.cs
- PointUtil.cs
- AnonymousIdentificationSection.cs
- TitleStyle.cs
- GridPattern.cs
- Renderer.cs
- Track.cs
- WebConvert.cs
- DispatcherExceptionEventArgs.cs
- Rotation3D.cs
- CollectionAdapters.cs
- BitStack.cs
- DictionaryBase.cs
- cookieexception.cs
- PanelContainerDesigner.cs
- ContextBase.cs
- XNodeValidator.cs
- xamlnodes.cs
- DropDownButton.cs
- SimpleHandlerBuildProvider.cs
- MenuItemStyle.cs
- RetrieveVirtualItemEventArgs.cs
- DesignerEditorPartChrome.cs
- MessageRpc.cs
- remotingproxy.cs
- Manipulation.cs
- ScriptResourceAttribute.cs
- CacheAxisQuery.cs
- XmlEncodedRawTextWriter.cs
- DebugInfoExpression.cs
- Select.cs
- ExternalFile.cs
- Base64Decoder.cs
- QuestionEventArgs.cs
- QilExpression.cs
- PolicyLevel.cs
- ValueSerializerAttribute.cs
- HybridDictionary.cs
- ByteRangeDownloader.cs
- BuildResultCache.cs
- SkinBuilder.cs
- InvalidFilterCriteriaException.cs
- FixedSOMPageConstructor.cs
- ListBoxAutomationPeer.cs
- TemplateXamlTreeBuilder.cs
- SamlSubjectStatement.cs
- ExpandCollapsePattern.cs
- HttpClientCredentialType.cs
- CompilationSection.cs
- InvalidDocumentContentsException.cs
- FieldToken.cs
- XsdDateTime.cs
- XmlCharacterData.cs
- DescendantQuery.cs
- TraceUtility.cs
- OraclePermission.cs
- SHA512Cng.cs
- TemplatePagerField.cs
- WebReferencesBuildProvider.cs
- ListItemsPage.cs
- StreamFormatter.cs
- Transform.cs
- GroupBox.cs
- OleDbRowUpdatedEvent.cs
- transactioncontext.cs
- SystemThemeKey.cs
- JsonClassDataContract.cs
- SoapExtensionTypeElementCollection.cs
- Drawing.cs