Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / MainMenu.cs / 1 / MainMenu.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; using System.Security.Permissions; ////// /// [ToolboxItemFilter("System.Windows.Forms.MainMenu")] public class MainMenu : Menu { internal Form form; internal Form ownerForm; // this is the form that created this menu, and is the only form allowed to dispose it. private RightToLeft rightToLeft = System.Windows.Forms.RightToLeft.Inherit; private EventHandler onCollapse; ////// Represents /// a menu structure for a form. ////// /// Creates a new MainMenu control. /// public MainMenu() : base(null) { } ////// /// public MainMenu(IContainer container) : this() { container.Add(this); } ///Initializes a new instance of the ///class with the specified container. /// /// Creates a new MainMenu control with the given items to start /// with. /// public MainMenu(MenuItem[] items) : base(items) { } ////// /// [SRDescription(SR.MainMenuCollapseDescr)] public event EventHandler Collapse { add { onCollapse += value; } remove { onCollapse -= value; } } ///[To be supplied.] ////// /// This is used for international applications where the language /// is written from RightToLeft. When this property is true, /// text alignment and reading order will be from right to left. /// // VSWhidbey 94189: Add an AmbientValue attribute so that the Reset context menu becomes available in the Property Grid. [ Localizable(true), AmbientValue(RightToLeft.Inherit), SRDescription(SR.MenuRightToLeftDescr) ] public virtual RightToLeft RightToLeft { get { if (System.Windows.Forms.RightToLeft.Inherit == rightToLeft) { if (form != null) { return form.RightToLeft; } else { return RightToLeft.Inherit; } } else { return rightToLeft; } } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)RightToLeft.No, (int)RightToLeft.Inherit)){ throw new InvalidEnumArgumentException("RightToLeft", (int)value, typeof(RightToLeft)); } if (rightToLeft != value) { rightToLeft = value; UpdateRtl((value == System.Windows.Forms.RightToLeft.Yes)); } } } internal override bool RenderIsRightToLeft { get { return (RightToLeft == System.Windows.Forms.RightToLeft.Yes && (form == null || !form.IsMirrored)); } } ////// /// Creates a new MainMenu object which is a dupliate of this one. /// public virtual MainMenu CloneMenu() { MainMenu newMenu = new MainMenu(); newMenu.CloneMenu(this); return newMenu; } ////// /// ///protected override IntPtr CreateMenuHandle() { return UnsafeNativeMethods.CreateMenu(); } /// /// /// Clears out this MainMenu object and discards all of it's resources. /// If the menu is parented in a form, it is disconnected from that as /// well. /// protected override void Dispose(bool disposing) { if (disposing) { if (form != null && (ownerForm == null || form == ownerForm)) { form.Menu = null; } } base.Dispose(disposing); } ////// /// Indicates which form in which we are currently residing [if any] /// [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] public Form GetForm() { return form; } internal Form GetFormUnsafe() { return form; } ////// /// ///internal override void ItemsChanged(int change) { base.ItemsChanged(change); if (form != null) form.MenuChanged(change, this); } /// /// /// ///internal virtual void ItemsChanged(int change, Menu menu) { if (form != null) form.MenuChanged(change, menu); } /// /// /// Fires the collapse event /// protected internal virtual void OnCollapse(EventArgs e) { if (onCollapse != null) { onCollapse(this, e); } } ////// /// Returns true if the RightToLeft should be persisted in code gen. /// internal virtual bool ShouldSerializeRightToLeft() { if (System.Windows.Forms.RightToLeft.Inherit == RightToLeft) { return false; } return true; } ////// /// Returns a string representation for this control. /// ///public override string ToString() { // VSWhidbey 495300: removing GetForm information return base.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
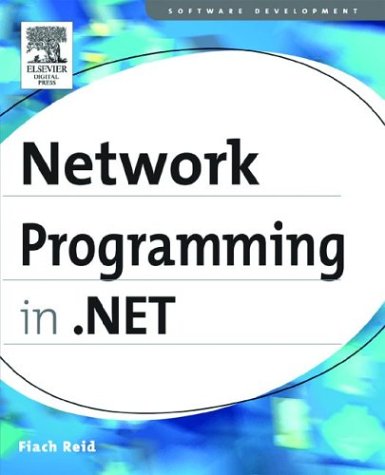
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AnnotationAdorner.cs
- Material.cs
- ValidatorCompatibilityHelper.cs
- DocumentOrderQuery.cs
- IIS7ConfigurationLoader.cs
- UIElementIsland.cs
- UserUseLicenseDictionaryLoader.cs
- QuaternionValueSerializer.cs
- RuleRef.cs
- GlyphInfoList.cs
- XDRSchema.cs
- StreamingContext.cs
- EdmToObjectNamespaceMap.cs
- XmlCountingReader.cs
- ScrollContentPresenter.cs
- CustomMenuItemCollection.cs
- TimelineGroup.cs
- COAUTHIDENTITY.cs
- LZCodec.cs
- Size3D.cs
- PeerNameRegistration.cs
- TemplateParser.cs
- ThousandthOfEmRealPoints.cs
- SqlMethodTransformer.cs
- ToolboxComponentsCreatedEventArgs.cs
- DateTimeOffsetStorage.cs
- DiscoveryClientDuplexChannel.cs
- PerformanceCounterCategory.cs
- HttpDictionary.cs
- ControlPropertyNameConverter.cs
- NotImplementedException.cs
- shaper.cs
- Perspective.cs
- AsymmetricSignatureFormatter.cs
- GreaterThan.cs
- SurrogateSelector.cs
- DataGridViewComboBoxColumn.cs
- ValidationRule.cs
- CngAlgorithm.cs
- SelectionRange.cs
- SystemIPInterfaceStatistics.cs
- ComplexLine.cs
- BinarySerializer.cs
- DataViewManager.cs
- DataGridViewCellCollection.cs
- Select.cs
- MouseGestureConverter.cs
- AssertHelper.cs
- ItemList.cs
- Scene3D.cs
- JsonEnumDataContract.cs
- IdentifierService.cs
- GacUtil.cs
- WebPartDisplayModeCollection.cs
- JsonByteArrayDataContract.cs
- RuleConditionDialog.Designer.cs
- LocationUpdates.cs
- TrackingLocationCollection.cs
- PatternMatcher.cs
- ComplexBindingPropertiesAttribute.cs
- BidOverLoads.cs
- Vector.cs
- BulletedList.cs
- FixUp.cs
- DesignSurfaceManager.cs
- errorpatternmatcher.cs
- RegexCapture.cs
- Operator.cs
- SqlUtils.cs
- DataGridViewHitTestInfo.cs
- _SpnDictionary.cs
- CodeCommentStatementCollection.cs
- ObjectViewEntityCollectionData.cs
- ColorKeyFrameCollection.cs
- WebPartConnectionsCancelEventArgs.cs
- PerformanceCounterPermissionAttribute.cs
- SqlRetyper.cs
- CodePageUtils.cs
- XmlIlTypeHelper.cs
- ResourcesChangeInfo.cs
- wgx_commands.cs
- DataGrid.cs
- UInt64.cs
- DeclarationUpdate.cs
- CodeBlockBuilder.cs
- DrawItemEvent.cs
- BitmapSizeOptions.cs
- HttpMethodAttribute.cs
- TrackingMemoryStream.cs
- WebPartMenuStyle.cs
- MobileComponentEditorPage.cs
- CodeSnippetStatement.cs
- SQlBooleanStorage.cs
- SchemaObjectWriter.cs
- WebPartChrome.cs
- AstNode.cs
- SchemaImporterExtensionsSection.cs
- ActionItem.cs
- ActiveXContainer.cs
- Formatter.cs