Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SrgsCompiler / RuleRef.cs / 1 / RuleRef.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- #region Using directives using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Speech.Internal.SrgsParser; using System.Text; #endregion namespace System.Speech.Internal.SrgsCompiler { internal class RuleRef : ParseElement, IRuleRef { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// Special private constructor for Special Rulerefs /// /// /// private RuleRef (SpecialRuleRefType type, Rule rule) : base (rule) { _type = type; } ////// Add transition corresponding to Special or Uri. /// /// /// /// /// /// /// internal RuleRef (ParseElementCollection parent, Backend backend, Uri uri, ListundefRules, string semanticKey, string initParameters) :base (parent._rule) { string id = uri.OriginalString; Rule ruleRef = null; int posPound = id.IndexOf ('#'); // Get the initial state for the RuleRef. if (posPound == 0) { // Internal RuleRef. Get InitialState of RuleRef. // GetRuleRef() may temporarily create a Rule placeholder for later resolution. ruleRef = GetRuleRef (backend, id.Substring (1), undefRules); } else { // External RuleRef. Build URL:GrammarUri#RuleName StringBuilder sbExternalRuleUri = new StringBuilder ("URL:"); // Add the parameters to initialize a rule if (!string.IsNullOrEmpty (initParameters)) { // look for the # and insert the parameters sbExternalRuleUri.Append (posPound > 0 ? id.Substring (0, posPound) : id); sbExternalRuleUri.Append ('>'); sbExternalRuleUri.Append (initParameters); if (posPound > 0) { sbExternalRuleUri.Append (id.Substring (posPound)); } } else { sbExternalRuleUri.Append (id); } // Get InitialState of external RuleRef. string sExternalRuleUri = sbExternalRuleUri.ToString (); ruleRef = backend.FindRule (sExternalRuleUri); if (ruleRef == null) { ruleRef = backend.CreateRule (sExternalRuleUri, SPCFGRULEATTRIBUTES.SPRAF_Import); } } Arc rulerefArc = backend.RuleTransition (ruleRef, _rule, 1.0f); if (!string.IsNullOrEmpty (semanticKey)) { CfgGrammar.CfgProperty propertyInfo = new CfgGrammar.CfgProperty (); propertyInfo._pszName = "SemanticKey"; propertyInfo._comValue = semanticKey; propertyInfo._comType = VarEnum.VT_EMPTY; backend.AddPropertyTag (rulerefArc, rulerefArc, propertyInfo); } parent.AddArc (rulerefArc); } #endregion //******************************************************************** // // Internal Method // //******************************************************************* #region Internal Method /// /// Returns the initial state of a special rule. /// For each type of special rule we make a rule with a numeric id and return a reference to it. /// /// /// ///internal void InitSpecialRuleRef (Backend backend, ParseElementCollection parent) { Rule rule = null; // Create a transition corresponding to Special or Uri switch (_type) { case SpecialRuleRefType.Null: parent.AddArc (backend.EpsilonTransition (1.0f)); break; case SpecialRuleRefType.Void: rule = backend.FindRule (szSpecialVoid); if (rule == null) { rule = backend.CreateRule (szSpecialVoid, 0); // Rule with no transitions is a void rule. ((IRule) rule).PostParse (parent); } parent.AddArc (backend.RuleTransition (rule, parent._rule, 1.0f)); break; case SpecialRuleRefType.Garbage: // Garbage transition is optional whereas Wildcard is not. So we need additional epsilon transition. OneOf oneOf = new OneOf (parent._rule, backend); // Add the garbage transition oneOf.AddArc (backend.RuleTransition (CfgGrammar.SPRULETRANS_WILDCARD, parent._rule, 0.5f)); // Add a parallele epsilon path oneOf.AddArc (backend.EpsilonTransition (0.5f)); ((IOneOf) oneOf).PostParse (parent); break; default: System.Diagnostics.Debug.Assert (false, "Unknown special ruleref type"); break; } } #endregion //******************************************************************** // // Private Methods // //******************************************************************** #region Private Methods /// /// Return the initial state of the rule with the specified name. /// If the rule is not defined yet, create a placeholder Rule. /// /// /// Rule name /// ///private static Rule GetRuleRef (Backend backend, string sRuleId, List undefRules) { System.Diagnostics.Debug.Assert (!string.IsNullOrEmpty (sRuleId)); // Get specified rule. Rule rule = backend.FindRule (sRuleId); if (rule == null) { // Rule doesn't exist. Create a placeholder rule and add StateHandle to UndefinedRules. rule = backend.CreateRule (sRuleId, 0); undefRules.Insert (0, rule); } return rule; } #endregion //******************************************************************* // // Public Properties // //******************************************************************** #region internal Properties static internal IRuleRef Null { get { return new RuleRef (SpecialRuleRefType.Null, null); } } static internal IRuleRef Void { get { return new RuleRef (SpecialRuleRefType.Void, null); } } static internal IRuleRef Garbage { get { return new RuleRef (SpecialRuleRefType.Garbage, null); } } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields //******************************************************************* // // Private Enums // //******************************************************************** #region Private Enums /// TODOC <_include file='doc\SpecialRuleRef.uex' path='docs/doc[@for="SpecialRuleRefType"]/*' /> // Special rule references allow grammars based on CFGs to have powerful // additional features, such as transitions into dictation (both recognized // or not recognized) and word seqeuences from SAPI 5.0. private enum SpecialRuleRefType { /// TODOC <_include file='doc\SpecialRuleRef.uex' path='docs/doc[@for="SpecialRuleRefType.Null"]/*' /> // Defines a rule that is automatically matched that is, matched without // the user speaking any word. Null, /// TODOC <_include file='doc\SpecialRuleRef.uex' path='docs/doc[@for="SpecialRuleRefType.Void"]/*' /> // Defines a rule that can never be spoken. Inserting VOID into a sequence // automatically makes that sequence unspeakable. Void, /// TODOC <_include file='doc\SpecialRuleRef.uex' path='docs/doc[@for="SpecialRuleRefType.Garbage"]/*' /> // Defines a rule that may match any speech up until the next rule match, // the next token or until the end of spoken input. // Designed for applications that would like to recognize some phrases // without failing due to irrelevant, or ignorable words. Garbage, } #endregion private SpecialRuleRefType _type; const string szSpecialVoid = "VOID"; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
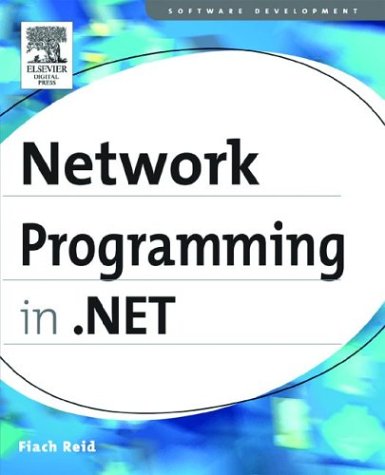
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttributeEmitter.cs
- CompositeCollection.cs
- ActivationWorker.cs
- UIElementCollection.cs
- FontStretches.cs
- XmlWrappingReader.cs
- LoginUtil.cs
- FastEncoderWindow.cs
- FontResourceCache.cs
- UnicodeEncoding.cs
- GeneralTransform3DGroup.cs
- VisualBasicHelper.cs
- DataControlFieldHeaderCell.cs
- ParallelEnumerable.cs
- SpeechRecognizer.cs
- HMAC.cs
- SmtpNetworkElement.cs
- WorkflowNamespace.cs
- SQLStringStorage.cs
- CodeAttributeArgument.cs
- NodeLabelEditEvent.cs
- DbConnectionPoolCounters.cs
- AdapterUtil.cs
- CombinedGeometry.cs
- ping.cs
- TablePattern.cs
- TranslateTransform3D.cs
- HashCoreRequest.cs
- PanelStyle.cs
- PngBitmapDecoder.cs
- BaseDataList.cs
- SetIndexBinder.cs
- DataServiceRequestOfT.cs
- QilXmlWriter.cs
- StateBag.cs
- WaveHeader.cs
- QilInvokeEarlyBound.cs
- CheckBoxPopupAdapter.cs
- ToolBar.cs
- NamespaceDisplay.xaml.cs
- Brush.cs
- RelativeSource.cs
- StandardBindingElementCollection.cs
- ProgramPublisher.cs
- InvalidOperationException.cs
- DBCommand.cs
- QueryExpr.cs
- Menu.cs
- EdgeModeValidation.cs
- InvalidProgramException.cs
- NullableLongMinMaxAggregationOperator.cs
- Model3DCollection.cs
- ResXResourceSet.cs
- FontStyleConverter.cs
- ImplicitInputBrush.cs
- LocalBuilder.cs
- SqlError.cs
- EventData.cs
- StandardOleMarshalObject.cs
- EmptyStringExpandableObjectConverter.cs
- XPathNodeList.cs
- ADMembershipUser.cs
- RequestNavigateEventArgs.cs
- DesignerImageAdapter.cs
- ClientBuildManager.cs
- UnsafeNativeMethods.cs
- SecurityDescriptor.cs
- SoundPlayer.cs
- ButtonChrome.cs
- JsonReaderWriterFactory.cs
- SqlConnection.cs
- MetaModel.cs
- BinaryFormatter.cs
- IResourceProvider.cs
- SyndicationElementExtensionCollection.cs
- TextTreeUndo.cs
- ArrayWithOffset.cs
- VectorCollectionConverter.cs
- MiniLockedBorderGlyph.cs
- TrackBarRenderer.cs
- ContentDisposition.cs
- BulletedListEventArgs.cs
- XmlSchemaComplexContentExtension.cs
- QuaternionAnimation.cs
- ComplexObject.cs
- BufferedStream.cs
- NetSectionGroup.cs
- HttpRuntimeSection.cs
- HttpWebResponse.cs
- TypedReference.cs
- QueryGeneratorBase.cs
- Int32.cs
- D3DImage.cs
- ItemsPanelTemplate.cs
- StatementContext.cs
- TraceHandler.cs
- FontInfo.cs
- ConfigurationStrings.cs
- SignedXmlDebugLog.cs
- DetailsViewModeEventArgs.cs