Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / EditorServiceContext.cs / 1 / EditorServiceContext.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms.Design.Behavior; using System.Drawing; using System.Drawing.Design; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; ////// /// internal class EditorServiceContext : IWindowsFormsEditorService, ITypeDescriptorContext { private ComponentDesigner _designer; private IComponentChangeService _componentChangeSvc; private PropertyDescriptor _targetProperty; [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal EditorServiceContext(ComponentDesigner designer) { this._designer = designer; } internal EditorServiceContext(ComponentDesigner designer, PropertyDescriptor prop) { this._designer = designer; this._targetProperty = prop; if (prop == null) { prop = TypeDescriptor.GetDefaultProperty(designer.Component); if (prop != null && typeof(ICollection).IsAssignableFrom(prop.PropertyType)) { _targetProperty = prop; } } Debug.Assert(_targetProperty != null, "Need PropertyDescriptor for ICollection property to associate collectoin edtior with."); } internal EditorServiceContext(ComponentDesigner designer, PropertyDescriptor prop, string newVerbText) : this(designer, prop) { Debug.Assert(!string.IsNullOrEmpty(newVerbText), "newVerbText cannot be null or empty"); _designer.Verbs.Add(new DesignerVerb(newVerbText, new EventHandler(this.OnEditItems))); } public static object EditValue(ComponentDesigner designer, object objectToChange, string propName) { // Get PropertyDescriptor PropertyDescriptor descriptor = TypeDescriptor.GetProperties(objectToChange)[propName]; // Create a Context EditorServiceContext context = new EditorServiceContext(designer, descriptor); // Get Editor UITypeEditor editor = descriptor.GetEditor(typeof(UITypeEditor)) as UITypeEditor; // Get value to edit object value = descriptor.GetValue(objectToChange); // Edit value object newValue = editor.EditValue(context, context, value); if (newValue != value) { try { descriptor.SetValue(objectToChange, newValue); } catch (CheckoutException) { } } return newValue; } ////// Provides an implementation of IWindowsFormsEditorService and ITypeDescriptorContext. /// Also provides a static method to invoke a UITypeEditor given a designer, an object /// and a property name. /// ////// Our caching property for the IComponentChangeService /// private IComponentChangeService ChangeService { get { if (_componentChangeSvc == null) { _componentChangeSvc = (IComponentChangeService)((IServiceProvider)this).GetService(typeof(IComponentChangeService)); } return _componentChangeSvc; } } ////// Self-explanitory interface impl. /// IContainer ITypeDescriptorContext.Container { get { if (_designer.Component.Site != null) { return _designer.Component.Site.Container; } return null; } } ////// Self-explanitory interface impl. /// void ITypeDescriptorContext.OnComponentChanged() { ChangeService.OnComponentChanged(_designer.Component, _targetProperty, null, null); } ////// Self-explanitory interface impl. /// bool ITypeDescriptorContext.OnComponentChanging() { try { ChangeService.OnComponentChanging(_designer.Component, _targetProperty); } catch (CheckoutException checkoutException) { if (checkoutException == CheckoutException.Canceled) { return false; } throw; } return true; } ////// Self-explanitory interface impl. /// object ITypeDescriptorContext.Instance { get { return _designer.Component; } } ////// Self-explanitory interface impl. /// PropertyDescriptor ITypeDescriptorContext.PropertyDescriptor { get { return _targetProperty; } } ////// Self-explanitory interface impl. /// object IServiceProvider.GetService(Type serviceType) { if (serviceType == typeof(ITypeDescriptorContext) || serviceType == typeof(IWindowsFormsEditorService)) { return this; } if (_designer.Component.Site != null) { return _designer.Component.Site.GetService(serviceType); } return null; } ////// Self-explanitory interface impl. /// void IWindowsFormsEditorService.CloseDropDown() { // we'll never be called to do this. // Debug.Fail("NOTIMPL"); return; } ////// Self-explanitory interface impl. /// void IWindowsFormsEditorService.DropDownControl(Control control) { // nope, sorry // Debug.Fail("NOTIMPL"); return; } ////// Self-explanitory interface impl. /// System.Windows.Forms.DialogResult IWindowsFormsEditorService.ShowDialog(Form dialog) { IUIService uiSvc = (IUIService)((IServiceProvider)this).GetService(typeof(IUIService)); if (uiSvc != null) { return uiSvc.ShowDialog(dialog); } else { return dialog.ShowDialog(_designer.Component as IWin32Window); } } ////// When the verb is invoked, use all the stuff above to show the dialog, etc. /// private void OnEditItems(object sender, EventArgs e) { object propertyValue = _targetProperty.GetValue(_designer.Component); if (propertyValue == null) { return; } CollectionEditor itemsEditor = TypeDescriptor.GetEditor(propertyValue, typeof(UITypeEditor)) as CollectionEditor; Debug.Assert(itemsEditor != null, "Didn't get a collection editor for type '" + _targetProperty.PropertyType.FullName + "'"); if (itemsEditor != null) { itemsEditor.EditValue(this, this, propertyValue); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
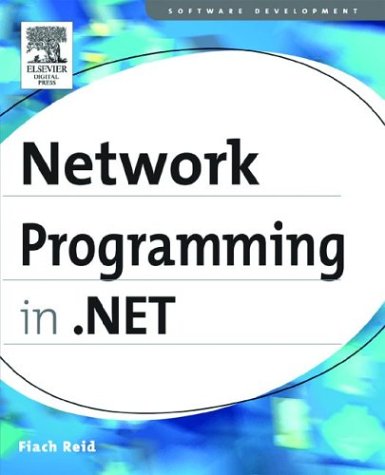
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PenContexts.cs
- KeyNotFoundException.cs
- NotConverter.cs
- ListViewDeleteEventArgs.cs
- EventMappingSettingsCollection.cs
- AssemblySettingAttributes.cs
- IdentityNotMappedException.cs
- SamlAssertion.cs
- MediaScriptCommandRoutedEventArgs.cs
- UIElementPropertyUndoUnit.cs
- LogReserveAndAppendState.cs
- GacUtil.cs
- SmuggledIUnknown.cs
- SmtpSection.cs
- DSASignatureDeformatter.cs
- ToolStripItemEventArgs.cs
- ActivityExecutionFilter.cs
- TextWriterTraceListener.cs
- VsPropertyGrid.cs
- ScrollBarRenderer.cs
- ClipboardData.cs
- LinearKeyFrames.cs
- RangeValuePattern.cs
- CFGGrammar.cs
- SevenBitStream.cs
- CallbackValidator.cs
- FlowDocumentReaderAutomationPeer.cs
- PageThemeParser.cs
- StyleBamlRecordReader.cs
- ListControl.cs
- Label.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- HwndSourceParameters.cs
- BooleanProjectedSlot.cs
- _HTTPDateParse.cs
- ImageMap.cs
- DateBoldEvent.cs
- ICspAsymmetricAlgorithm.cs
- ExpressionPrefixAttribute.cs
- SQLInt64Storage.cs
- RootBrowserWindowAutomationPeer.cs
- CompleteWizardStep.cs
- NumberFormatter.cs
- CssClassPropertyAttribute.cs
- Point4DValueSerializer.cs
- SpecularMaterial.cs
- MetadataPropertyvalue.cs
- HTTPNotFoundHandler.cs
- PlainXmlSerializer.cs
- FacetDescriptionElement.cs
- SafeWaitHandle.cs
- PeerApplicationLaunchInfo.cs
- TextDecorations.cs
- ImplicitInputBrush.cs
- Highlights.cs
- TrustManager.cs
- EllipseGeometry.cs
- MailMessage.cs
- ConfigPathUtility.cs
- MediaPlayer.cs
- AttributeUsageAttribute.cs
- RefreshEventArgs.cs
- Triangle.cs
- Panel.cs
- Schema.cs
- ContentPropertyAttribute.cs
- EdmItemCollection.cs
- MediaContext.cs
- IChannel.cs
- ReplyChannelAcceptor.cs
- BeginStoryboard.cs
- MailSettingsSection.cs
- RootBrowserWindowProxy.cs
- NativeActivityAbortContext.cs
- SerialReceived.cs
- PageTrueTypeFont.cs
- Transform.cs
- MaskInputRejectedEventArgs.cs
- SmtpFailedRecipientsException.cs
- OpacityConverter.cs
- QilScopedVisitor.cs
- ImportContext.cs
- TextRunTypographyProperties.cs
- HttpModuleCollection.cs
- XmlElementAttributes.cs
- BuildManager.cs
- WebPartConnectionsCancelVerb.cs
- SiteMapDataSourceView.cs
- SchemaAttDef.cs
- IfAction.cs
- Parameter.cs
- sqlnorm.cs
- SqlMethodAttribute.cs
- NestedContainer.cs
- InputLanguageEventArgs.cs
- ButtonPopupAdapter.cs
- ProxyHelper.cs
- ConfigurationSchemaErrors.cs
- DesignerTransactionCloseEvent.cs
- SslStreamSecurityBindingElement.cs